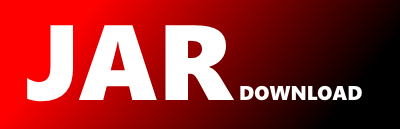
com.pulumi.azurenative.azurearcdata.kotlin.inputs.AvailabilityGroupInfoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurearcdata.kotlin.inputs
import com.pulumi.azurenative.azurearcdata.inputs.AvailabilityGroupInfoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The specifications of the availability group state
* @property basicFeatures Specifies whether this is a basic availability group.
* @property dbFailover Specifies whether the availability group supports failover for database health conditions.
* @property dtcSupport Specifies whether DTC support has been enabled for this availability group.
* @property failureConditionLevel User-defined failure condition level under which an automatic failover must be triggered.
* @property healthCheckTimeout Wait time (in milliseconds) for the sp_server_diagnostics system stored procedure to return server-health information, before the server instance is assumed to be slow or not responding.
* @property isContained SQL Server availability group contained system databases.
* @property isDistributed Specifies whether this is a distributed availability group.
* @property requiredSynchronizedSecondariesToCommit The number of secondary replicas that must be in a synchronized state for a commit to complete.
*/
public data class AvailabilityGroupInfoArgs(
public val basicFeatures: Output? = null,
public val dbFailover: Output? = null,
public val dtcSupport: Output? = null,
public val failureConditionLevel: Output? = null,
public val healthCheckTimeout: Output? = null,
public val isContained: Output? = null,
public val isDistributed: Output? = null,
public val requiredSynchronizedSecondariesToCommit: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurearcdata.inputs.AvailabilityGroupInfoArgs =
com.pulumi.azurenative.azurearcdata.inputs.AvailabilityGroupInfoArgs.builder()
.basicFeatures(basicFeatures?.applyValue({ args0 -> args0 }))
.dbFailover(dbFailover?.applyValue({ args0 -> args0 }))
.dtcSupport(dtcSupport?.applyValue({ args0 -> args0 }))
.failureConditionLevel(failureConditionLevel?.applyValue({ args0 -> args0 }))
.healthCheckTimeout(healthCheckTimeout?.applyValue({ args0 -> args0 }))
.isContained(isContained?.applyValue({ args0 -> args0 }))
.isDistributed(isDistributed?.applyValue({ args0 -> args0 }))
.requiredSynchronizedSecondariesToCommit(
requiredSynchronizedSecondariesToCommit?.applyValue({ args0 ->
args0
}),
).build()
}
/**
* Builder for [AvailabilityGroupInfoArgs].
*/
@PulumiTagMarker
public class AvailabilityGroupInfoArgsBuilder internal constructor() {
private var basicFeatures: Output? = null
private var dbFailover: Output? = null
private var dtcSupport: Output? = null
private var failureConditionLevel: Output? = null
private var healthCheckTimeout: Output? = null
private var isContained: Output? = null
private var isDistributed: Output? = null
private var requiredSynchronizedSecondariesToCommit: Output? = null
/**
* @param value Specifies whether this is a basic availability group.
*/
@JvmName("tmotupjyfwpfvwyb")
public suspend fun basicFeatures(`value`: Output) {
this.basicFeatures = value
}
/**
* @param value Specifies whether the availability group supports failover for database health conditions.
*/
@JvmName("jehncburcacarnjm")
public suspend fun dbFailover(`value`: Output) {
this.dbFailover = value
}
/**
* @param value Specifies whether DTC support has been enabled for this availability group.
*/
@JvmName("bptjcvtfefhdjigh")
public suspend fun dtcSupport(`value`: Output) {
this.dtcSupport = value
}
/**
* @param value User-defined failure condition level under which an automatic failover must be triggered.
*/
@JvmName("uokdekuccwqehgse")
public suspend fun failureConditionLevel(`value`: Output) {
this.failureConditionLevel = value
}
/**
* @param value Wait time (in milliseconds) for the sp_server_diagnostics system stored procedure to return server-health information, before the server instance is assumed to be slow or not responding.
*/
@JvmName("docpejfitemfsaql")
public suspend fun healthCheckTimeout(`value`: Output) {
this.healthCheckTimeout = value
}
/**
* @param value SQL Server availability group contained system databases.
*/
@JvmName("nmcjwdpailtuream")
public suspend fun isContained(`value`: Output) {
this.isContained = value
}
/**
* @param value Specifies whether this is a distributed availability group.
*/
@JvmName("tklenkpfvxmafaix")
public suspend fun isDistributed(`value`: Output) {
this.isDistributed = value
}
/**
* @param value The number of secondary replicas that must be in a synchronized state for a commit to complete.
*/
@JvmName("ieqpudvoouyinvdm")
public suspend fun requiredSynchronizedSecondariesToCommit(`value`: Output) {
this.requiredSynchronizedSecondariesToCommit = value
}
/**
* @param value Specifies whether this is a basic availability group.
*/
@JvmName("dpxkxelbicjppbsa")
public suspend fun basicFeatures(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.basicFeatures = mapped
}
/**
* @param value Specifies whether the availability group supports failover for database health conditions.
*/
@JvmName("pnkivauusbyoqnpu")
public suspend fun dbFailover(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dbFailover = mapped
}
/**
* @param value Specifies whether DTC support has been enabled for this availability group.
*/
@JvmName("bfsojueowumhkmlc")
public suspend fun dtcSupport(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dtcSupport = mapped
}
/**
* @param value User-defined failure condition level under which an automatic failover must be triggered.
*/
@JvmName("xvsylnfdbyuowdad")
public suspend fun failureConditionLevel(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureConditionLevel = mapped
}
/**
* @param value Wait time (in milliseconds) for the sp_server_diagnostics system stored procedure to return server-health information, before the server instance is assumed to be slow or not responding.
*/
@JvmName("grelmesfqmoxfofm")
public suspend fun healthCheckTimeout(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.healthCheckTimeout = mapped
}
/**
* @param value SQL Server availability group contained system databases.
*/
@JvmName("onuhwaoxevkdulyv")
public suspend fun isContained(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isContained = mapped
}
/**
* @param value Specifies whether this is a distributed availability group.
*/
@JvmName("hvhbscqybopbjcif")
public suspend fun isDistributed(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isDistributed = mapped
}
/**
* @param value The number of secondary replicas that must be in a synchronized state for a commit to complete.
*/
@JvmName("aolajyxgaobgqsus")
public suspend fun requiredSynchronizedSecondariesToCommit(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requiredSynchronizedSecondariesToCommit = mapped
}
internal fun build(): AvailabilityGroupInfoArgs = AvailabilityGroupInfoArgs(
basicFeatures = basicFeatures,
dbFailover = dbFailover,
dtcSupport = dtcSupport,
failureConditionLevel = failureConditionLevel,
healthCheckTimeout = healthCheckTimeout,
isContained = isContained,
isDistributed = isDistributed,
requiredSynchronizedSecondariesToCommit = requiredSynchronizedSecondariesToCommit,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy