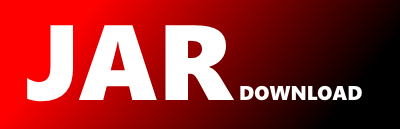
com.pulumi.azurenative.azurearcdata.kotlin.inputs.DataControllerPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurearcdata.kotlin.inputs
import com.pulumi.azurenative.azurearcdata.inputs.DataControllerPropertiesArgs.builder
import com.pulumi.azurenative.azurearcdata.kotlin.enums.Infrastructure
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The data controller properties.
* @property basicLoginInformation Deprecated. Azure Arc Data Services data controller no longer expose any endpoint. All traffic are exposed through Kubernetes native API.
* @property clusterId If a CustomLocation is provided, this contains the ARM id of the connected cluster the custom location belongs to.
* @property extensionId If a CustomLocation is provided, this contains the ARM id of the extension the custom location belongs to.
* @property infrastructure The infrastructure the data controller is running on.
* @property k8sRaw The raw kubernetes information
* @property lastUploadedDate Last uploaded date from Kubernetes cluster. Defaults to current date time
* @property logAnalyticsWorkspaceConfig Log analytics workspace id and primary key
* @property logsDashboardCredential Login credential for logs dashboard on the Kubernetes cluster.
* @property metricsDashboardCredential Login credential for metrics dashboard on the Kubernetes cluster.
* @property onPremiseProperty Properties from the Kubernetes data controller
* @property uploadServicePrincipal Deprecated. Service principal is deprecated in favor of Arc Kubernetes service extension managed identity.
* @property uploadWatermark Properties on upload watermark. Mostly timestamp for each upload data type
*/
public data class DataControllerPropertiesArgs(
public val basicLoginInformation: Output? = null,
public val clusterId: Output? = null,
public val extensionId: Output? = null,
public val infrastructure: Output? = null,
public val k8sRaw: Output? = null,
public val lastUploadedDate: Output? = null,
public val logAnalyticsWorkspaceConfig: Output? = null,
public val logsDashboardCredential: Output? = null,
public val metricsDashboardCredential: Output? = null,
public val onPremiseProperty: Output? = null,
public val uploadServicePrincipal: Output? = null,
public val uploadWatermark: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurearcdata.inputs.DataControllerPropertiesArgs =
com.pulumi.azurenative.azurearcdata.inputs.DataControllerPropertiesArgs.builder()
.basicLoginInformation(
basicLoginInformation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.clusterId(clusterId?.applyValue({ args0 -> args0 }))
.extensionId(extensionId?.applyValue({ args0 -> args0 }))
.infrastructure(infrastructure?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.k8sRaw(k8sRaw?.applyValue({ args0 -> args0 }))
.lastUploadedDate(lastUploadedDate?.applyValue({ args0 -> args0 }))
.logAnalyticsWorkspaceConfig(
logAnalyticsWorkspaceConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.logsDashboardCredential(
logsDashboardCredential?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.metricsDashboardCredential(
metricsDashboardCredential?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.onPremiseProperty(onPremiseProperty?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.uploadServicePrincipal(
uploadServicePrincipal?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.uploadWatermark(
uploadWatermark?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DataControllerPropertiesArgs].
*/
@PulumiTagMarker
public class DataControllerPropertiesArgsBuilder internal constructor() {
private var basicLoginInformation: Output? = null
private var clusterId: Output? = null
private var extensionId: Output? = null
private var infrastructure: Output? = null
private var k8sRaw: Output? = null
private var lastUploadedDate: Output? = null
private var logAnalyticsWorkspaceConfig: Output? = null
private var logsDashboardCredential: Output? = null
private var metricsDashboardCredential: Output? = null
private var onPremiseProperty: Output? = null
private var uploadServicePrincipal: Output? = null
private var uploadWatermark: Output? = null
/**
* @param value Deprecated. Azure Arc Data Services data controller no longer expose any endpoint. All traffic are exposed through Kubernetes native API.
*/
@JvmName("gqsfxwkjbakyymjc")
public suspend fun basicLoginInformation(`value`: Output) {
this.basicLoginInformation = value
}
/**
* @param value If a CustomLocation is provided, this contains the ARM id of the connected cluster the custom location belongs to.
*/
@JvmName("xkbvtyxtauftggrd")
public suspend fun clusterId(`value`: Output) {
this.clusterId = value
}
/**
* @param value If a CustomLocation is provided, this contains the ARM id of the extension the custom location belongs to.
*/
@JvmName("xwmjoyvpgwgmcrry")
public suspend fun extensionId(`value`: Output) {
this.extensionId = value
}
/**
* @param value The infrastructure the data controller is running on.
*/
@JvmName("ebthmvaoykcridmt")
public suspend fun infrastructure(`value`: Output) {
this.infrastructure = value
}
/**
* @param value The raw kubernetes information
*/
@JvmName("kipxivwtytlbdlay")
public suspend fun k8sRaw(`value`: Output) {
this.k8sRaw = value
}
/**
* @param value Last uploaded date from Kubernetes cluster. Defaults to current date time
*/
@JvmName("mfpepbgkdlinwnps")
public suspend fun lastUploadedDate(`value`: Output) {
this.lastUploadedDate = value
}
/**
* @param value Log analytics workspace id and primary key
*/
@JvmName("nkkjxrdyrnesryon")
public suspend fun logAnalyticsWorkspaceConfig(`value`: Output) {
this.logAnalyticsWorkspaceConfig = value
}
/**
* @param value Login credential for logs dashboard on the Kubernetes cluster.
*/
@JvmName("bwkycjivpyqoxbbu")
public suspend fun logsDashboardCredential(`value`: Output) {
this.logsDashboardCredential = value
}
/**
* @param value Login credential for metrics dashboard on the Kubernetes cluster.
*/
@JvmName("edvfrfxdysnvtbdn")
public suspend fun metricsDashboardCredential(`value`: Output) {
this.metricsDashboardCredential = value
}
/**
* @param value Properties from the Kubernetes data controller
*/
@JvmName("veactnjxrewxjcqg")
public suspend fun onPremiseProperty(`value`: Output) {
this.onPremiseProperty = value
}
/**
* @param value Deprecated. Service principal is deprecated in favor of Arc Kubernetes service extension managed identity.
*/
@JvmName("bwmnqdpyththjoxk")
public suspend fun uploadServicePrincipal(`value`: Output) {
this.uploadServicePrincipal = value
}
/**
* @param value Properties on upload watermark. Mostly timestamp for each upload data type
*/
@JvmName("feluotbjnohdqhxp")
public suspend fun uploadWatermark(`value`: Output) {
this.uploadWatermark = value
}
/**
* @param value Deprecated. Azure Arc Data Services data controller no longer expose any endpoint. All traffic are exposed through Kubernetes native API.
*/
@JvmName("mrtagnodhkevimos")
public suspend fun basicLoginInformation(`value`: BasicLoginInformationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.basicLoginInformation = mapped
}
/**
* @param argument Deprecated. Azure Arc Data Services data controller no longer expose any endpoint. All traffic are exposed through Kubernetes native API.
*/
@JvmName("pvadfreyukdsgryv")
public suspend fun basicLoginInformation(argument: suspend BasicLoginInformationArgsBuilder.() -> Unit) {
val toBeMapped = BasicLoginInformationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.basicLoginInformation = mapped
}
/**
* @param value If a CustomLocation is provided, this contains the ARM id of the connected cluster the custom location belongs to.
*/
@JvmName("gxhijseuvcnnfaql")
public suspend fun clusterId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterId = mapped
}
/**
* @param value If a CustomLocation is provided, this contains the ARM id of the extension the custom location belongs to.
*/
@JvmName("douhfmhejlasrmas")
public suspend fun extensionId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.extensionId = mapped
}
/**
* @param value The infrastructure the data controller is running on.
*/
@JvmName("mdvrncwvpthxmvek")
public suspend fun infrastructure(`value`: Infrastructure?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.infrastructure = mapped
}
/**
* @param value The raw kubernetes information
*/
@JvmName("xayvnjyglnqxndrn")
public suspend fun k8sRaw(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.k8sRaw = mapped
}
/**
* @param value Last uploaded date from Kubernetes cluster. Defaults to current date time
*/
@JvmName("knqdmrfopykttpwr")
public suspend fun lastUploadedDate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastUploadedDate = mapped
}
/**
* @param value Log analytics workspace id and primary key
*/
@JvmName("bmkgobpwiiyylmqg")
public suspend fun logAnalyticsWorkspaceConfig(`value`: LogAnalyticsWorkspaceConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logAnalyticsWorkspaceConfig = mapped
}
/**
* @param argument Log analytics workspace id and primary key
*/
@JvmName("fflcqwdoirowjxcb")
public suspend fun logAnalyticsWorkspaceConfig(argument: suspend LogAnalyticsWorkspaceConfigArgsBuilder.() -> Unit) {
val toBeMapped = LogAnalyticsWorkspaceConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.logAnalyticsWorkspaceConfig = mapped
}
/**
* @param value Login credential for logs dashboard on the Kubernetes cluster.
*/
@JvmName("qcmpmvfueklbbxaf")
public suspend fun logsDashboardCredential(`value`: BasicLoginInformationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logsDashboardCredential = mapped
}
/**
* @param argument Login credential for logs dashboard on the Kubernetes cluster.
*/
@JvmName("aslyiutbhkxmomdx")
public suspend fun logsDashboardCredential(argument: suspend BasicLoginInformationArgsBuilder.() -> Unit) {
val toBeMapped = BasicLoginInformationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.logsDashboardCredential = mapped
}
/**
* @param value Login credential for metrics dashboard on the Kubernetes cluster.
*/
@JvmName("yakynlwqcfriiite")
public suspend fun metricsDashboardCredential(`value`: BasicLoginInformationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricsDashboardCredential = mapped
}
/**
* @param argument Login credential for metrics dashboard on the Kubernetes cluster.
*/
@JvmName("adbpjwhbiqcjihqa")
public suspend fun metricsDashboardCredential(argument: suspend BasicLoginInformationArgsBuilder.() -> Unit) {
val toBeMapped = BasicLoginInformationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metricsDashboardCredential = mapped
}
/**
* @param value Properties from the Kubernetes data controller
*/
@JvmName("uxodkihwpdyekulh")
public suspend fun onPremiseProperty(`value`: OnPremisePropertyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.onPremiseProperty = mapped
}
/**
* @param argument Properties from the Kubernetes data controller
*/
@JvmName("anfsvtdyvbtaycxd")
public suspend fun onPremiseProperty(argument: suspend OnPremisePropertyArgsBuilder.() -> Unit) {
val toBeMapped = OnPremisePropertyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.onPremiseProperty = mapped
}
/**
* @param value Deprecated. Service principal is deprecated in favor of Arc Kubernetes service extension managed identity.
*/
@JvmName("qvfxryhqgqbckwhd")
public suspend fun uploadServicePrincipal(`value`: UploadServicePrincipalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uploadServicePrincipal = mapped
}
/**
* @param argument Deprecated. Service principal is deprecated in favor of Arc Kubernetes service extension managed identity.
*/
@JvmName("livhxmpmljsjdfvw")
public suspend fun uploadServicePrincipal(argument: suspend UploadServicePrincipalArgsBuilder.() -> Unit) {
val toBeMapped = UploadServicePrincipalArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.uploadServicePrincipal = mapped
}
/**
* @param value Properties on upload watermark. Mostly timestamp for each upload data type
*/
@JvmName("nejcnbwfktlkevuv")
public suspend fun uploadWatermark(`value`: UploadWatermarkArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.uploadWatermark = mapped
}
/**
* @param argument Properties on upload watermark. Mostly timestamp for each upload data type
*/
@JvmName("omdrppwoqmsvjqio")
public suspend fun uploadWatermark(argument: suspend UploadWatermarkArgsBuilder.() -> Unit) {
val toBeMapped = UploadWatermarkArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.uploadWatermark = mapped
}
internal fun build(): DataControllerPropertiesArgs = DataControllerPropertiesArgs(
basicLoginInformation = basicLoginInformation,
clusterId = clusterId,
extensionId = extensionId,
infrastructure = infrastructure,
k8sRaw = k8sRaw,
lastUploadedDate = lastUploadedDate,
logAnalyticsWorkspaceConfig = logAnalyticsWorkspaceConfig,
logsDashboardCredential = logsDashboardCredential,
metricsDashboardCredential = metricsDashboardCredential,
onPremiseProperty = onPremiseProperty,
uploadServicePrincipal = uploadServicePrincipal,
uploadWatermark = uploadWatermark,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy