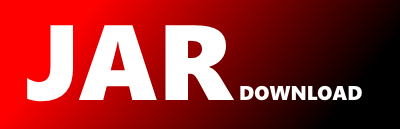
com.pulumi.azurenative.azurefleet.kotlin.inputs.ImageReferenceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurefleet.kotlin.inputs
import com.pulumi.azurenative.azurefleet.inputs.ImageReferenceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Specifies information about the image to use. You can specify information about
* platform images, marketplace images, or virtual machine images. This element is
* required when you want to use a platform image, marketplace image, or virtual
* machine image, but is not used in other creation operations. NOTE: Image
* reference publisher and offer can only be set when you create the scale set.
* @property communityGalleryImageId Specified the community gallery image unique id for vm deployment. This can be
* fetched from community gallery image GET call.
* @property id Resource Id
* @property offer Specifies the offer of the platform image or marketplace image used to create
* the virtual machine.
* @property publisher The image publisher.
* @property sharedGalleryImageId Specified the shared gallery image unique id for vm deployment. This can be
* fetched from shared gallery image GET call.
* @property sku The image SKU.
* @property version Specifies the version of the platform image or marketplace image used to create
* the virtual machine. The allowed formats are Major.Minor.Build or 'latest'.
* Major, Minor, and Build are decimal numbers. Specify 'latest' to use the latest
* version of an image available at deploy time. Even if you use 'latest', the VM
* image will not automatically update after deploy time even if a new version
* becomes available. Please do not use field 'version' for gallery image
* deployment, gallery image should always use 'id' field for deployment, to use 'latest'
* version of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{galleryName}/images/{imageName}'
* in the 'id' field without version input.
*/
public data class ImageReferenceArgs(
public val communityGalleryImageId: Output? = null,
public val id: Output? = null,
public val offer: Output? = null,
public val publisher: Output? = null,
public val sharedGalleryImageId: Output? = null,
public val sku: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurefleet.inputs.ImageReferenceArgs =
com.pulumi.azurenative.azurefleet.inputs.ImageReferenceArgs.builder()
.communityGalleryImageId(communityGalleryImageId?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.offer(offer?.applyValue({ args0 -> args0 }))
.publisher(publisher?.applyValue({ args0 -> args0 }))
.sharedGalleryImageId(sharedGalleryImageId?.applyValue({ args0 -> args0 }))
.sku(sku?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ImageReferenceArgs].
*/
@PulumiTagMarker
public class ImageReferenceArgsBuilder internal constructor() {
private var communityGalleryImageId: Output? = null
private var id: Output? = null
private var offer: Output? = null
private var publisher: Output? = null
private var sharedGalleryImageId: Output? = null
private var sku: Output? = null
private var version: Output? = null
/**
* @param value Specified the community gallery image unique id for vm deployment. This can be
* fetched from community gallery image GET call.
*/
@JvmName("dpvvyxnbunwhodbl")
public suspend fun communityGalleryImageId(`value`: Output) {
this.communityGalleryImageId = value
}
/**
* @param value Resource Id
*/
@JvmName("itgedlvoiuxrykcc")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Specifies the offer of the platform image or marketplace image used to create
* the virtual machine.
*/
@JvmName("ajasnotrkodxejgb")
public suspend fun offer(`value`: Output) {
this.offer = value
}
/**
* @param value The image publisher.
*/
@JvmName("vkvtohpyorluiecw")
public suspend fun publisher(`value`: Output) {
this.publisher = value
}
/**
* @param value Specified the shared gallery image unique id for vm deployment. This can be
* fetched from shared gallery image GET call.
*/
@JvmName("ekjjmerrlgoifruv")
public suspend fun sharedGalleryImageId(`value`: Output) {
this.sharedGalleryImageId = value
}
/**
* @param value The image SKU.
*/
@JvmName("mhmhifquexsbjnqa")
public suspend fun sku(`value`: Output) {
this.sku = value
}
/**
* @param value Specifies the version of the platform image or marketplace image used to create
* the virtual machine. The allowed formats are Major.Minor.Build or 'latest'.
* Major, Minor, and Build are decimal numbers. Specify 'latest' to use the latest
* version of an image available at deploy time. Even if you use 'latest', the VM
* image will not automatically update after deploy time even if a new version
* becomes available. Please do not use field 'version' for gallery image
* deployment, gallery image should always use 'id' field for deployment, to use 'latest'
* version of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{galleryName}/images/{imageName}'
* in the 'id' field without version input.
*/
@JvmName("rvqponijhaaxndjr")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value Specified the community gallery image unique id for vm deployment. This can be
* fetched from community gallery image GET call.
*/
@JvmName("tksidkmkvawenurr")
public suspend fun communityGalleryImageId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.communityGalleryImageId = mapped
}
/**
* @param value Resource Id
*/
@JvmName("iebkhwepsvmcqnvr")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Specifies the offer of the platform image or marketplace image used to create
* the virtual machine.
*/
@JvmName("xqdomnluxwrgtgmn")
public suspend fun offer(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.offer = mapped
}
/**
* @param value The image publisher.
*/
@JvmName("jnqduwakqoaxvsxr")
public suspend fun publisher(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publisher = mapped
}
/**
* @param value Specified the shared gallery image unique id for vm deployment. This can be
* fetched from shared gallery image GET call.
*/
@JvmName("clphtetdrpqenswq")
public suspend fun sharedGalleryImageId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sharedGalleryImageId = mapped
}
/**
* @param value The image SKU.
*/
@JvmName("xjstjohjyefctsyi")
public suspend fun sku(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sku = mapped
}
/**
* @param value Specifies the version of the platform image or marketplace image used to create
* the virtual machine. The allowed formats are Major.Minor.Build or 'latest'.
* Major, Minor, and Build are decimal numbers. Specify 'latest' to use the latest
* version of an image available at deploy time. Even if you use 'latest', the VM
* image will not automatically update after deploy time even if a new version
* becomes available. Please do not use field 'version' for gallery image
* deployment, gallery image should always use 'id' field for deployment, to use 'latest'
* version of gallery image, just set
* '/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/galleries/{galleryName}/images/{imageName}'
* in the 'id' field without version input.
*/
@JvmName("wvgitdvwaxlymdvm")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): ImageReferenceArgs = ImageReferenceArgs(
communityGalleryImageId = communityGalleryImageId,
id = id,
offer = offer,
publisher = publisher,
sharedGalleryImageId = sharedGalleryImageId,
sku = sku,
version = version,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy