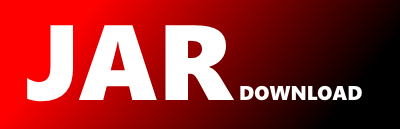
com.pulumi.azurenative.azurefleet.kotlin.inputs.LinuxConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurefleet.kotlin.inputs
import com.pulumi.azurenative.azurefleet.inputs.LinuxConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Specifies the Linux operating system settings on the virtual machine. For a
* list of supported Linux distributions, see [Linux on Azure-Endorsed
* Distributions](https://docs.microsoft.com/azure/virtual-machines/linux/endorsed-distros).
* @property disablePasswordAuthentication Specifies whether password authentication should be disabled.
* @property enableVMAgentPlatformUpdates Indicates whether VMAgent Platform Updates is enabled for the Linux virtual
* machine. Default value is false.
* @property patchSettings [Preview Feature] Specifies settings related to VM Guest Patching on Linux.
* @property provisionVMAgent Indicates whether virtual machine agent should be provisioned on the virtual
* machine. When this property is not specified in the request body, default
* behavior is to set it to true. This will ensure that VM Agent is installed on
* the VM so that extensions can be added to the VM later.
* @property ssh Specifies the ssh key configuration for a Linux OS.
*/
public data class LinuxConfigurationArgs(
public val disablePasswordAuthentication: Output? = null,
public val enableVMAgentPlatformUpdates: Output? = null,
public val patchSettings: Output? = null,
public val provisionVMAgent: Output? = null,
public val ssh: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurefleet.inputs.LinuxConfigurationArgs =
com.pulumi.azurenative.azurefleet.inputs.LinuxConfigurationArgs.builder()
.disablePasswordAuthentication(disablePasswordAuthentication?.applyValue({ args0 -> args0 }))
.enableVMAgentPlatformUpdates(enableVMAgentPlatformUpdates?.applyValue({ args0 -> args0 }))
.patchSettings(patchSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.provisionVMAgent(provisionVMAgent?.applyValue({ args0 -> args0 }))
.ssh(ssh?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [LinuxConfigurationArgs].
*/
@PulumiTagMarker
public class LinuxConfigurationArgsBuilder internal constructor() {
private var disablePasswordAuthentication: Output? = null
private var enableVMAgentPlatformUpdates: Output? = null
private var patchSettings: Output? = null
private var provisionVMAgent: Output? = null
private var ssh: Output? = null
/**
* @param value Specifies whether password authentication should be disabled.
*/
@JvmName("keauprompptkrwgl")
public suspend fun disablePasswordAuthentication(`value`: Output) {
this.disablePasswordAuthentication = value
}
/**
* @param value Indicates whether VMAgent Platform Updates is enabled for the Linux virtual
* machine. Default value is false.
*/
@JvmName("axaqjosoprhamdho")
public suspend fun enableVMAgentPlatformUpdates(`value`: Output) {
this.enableVMAgentPlatformUpdates = value
}
/**
* @param value [Preview Feature] Specifies settings related to VM Guest Patching on Linux.
*/
@JvmName("ehvmnucxoypbknok")
public suspend fun patchSettings(`value`: Output) {
this.patchSettings = value
}
/**
* @param value Indicates whether virtual machine agent should be provisioned on the virtual
* machine. When this property is not specified in the request body, default
* behavior is to set it to true. This will ensure that VM Agent is installed on
* the VM so that extensions can be added to the VM later.
*/
@JvmName("qadtykagbcqcpabv")
public suspend fun provisionVMAgent(`value`: Output) {
this.provisionVMAgent = value
}
/**
* @param value Specifies the ssh key configuration for a Linux OS.
*/
@JvmName("bmyfyxpdpgoqefol")
public suspend fun ssh(`value`: Output) {
this.ssh = value
}
/**
* @param value Specifies whether password authentication should be disabled.
*/
@JvmName("ntnnoljunkgjpjqb")
public suspend fun disablePasswordAuthentication(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disablePasswordAuthentication = mapped
}
/**
* @param value Indicates whether VMAgent Platform Updates is enabled for the Linux virtual
* machine. Default value is false.
*/
@JvmName("eepeargbhsxlewvs")
public suspend fun enableVMAgentPlatformUpdates(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableVMAgentPlatformUpdates = mapped
}
/**
* @param value [Preview Feature] Specifies settings related to VM Guest Patching on Linux.
*/
@JvmName("qcrosbnexmdmvhon")
public suspend fun patchSettings(`value`: LinuxPatchSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.patchSettings = mapped
}
/**
* @param argument [Preview Feature] Specifies settings related to VM Guest Patching on Linux.
*/
@JvmName("gylgajntedxwscie")
public suspend fun patchSettings(argument: suspend LinuxPatchSettingsArgsBuilder.() -> Unit) {
val toBeMapped = LinuxPatchSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.patchSettings = mapped
}
/**
* @param value Indicates whether virtual machine agent should be provisioned on the virtual
* machine. When this property is not specified in the request body, default
* behavior is to set it to true. This will ensure that VM Agent is installed on
* the VM so that extensions can be added to the VM later.
*/
@JvmName("gqljuuthmcmnxkrg")
public suspend fun provisionVMAgent(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.provisionVMAgent = mapped
}
/**
* @param value Specifies the ssh key configuration for a Linux OS.
*/
@JvmName("yyekvbopimtqrcpu")
public suspend fun ssh(`value`: SshConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ssh = mapped
}
/**
* @param argument Specifies the ssh key configuration for a Linux OS.
*/
@JvmName("nvnnemrrgqnnoduj")
public suspend fun ssh(argument: suspend SshConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = SshConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ssh = mapped
}
internal fun build(): LinuxConfigurationArgs = LinuxConfigurationArgs(
disablePasswordAuthentication = disablePasswordAuthentication,
enableVMAgentPlatformUpdates = enableVMAgentPlatformUpdates,
patchSettings = patchSettings,
provisionVMAgent = provisionVMAgent,
ssh = ssh,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy