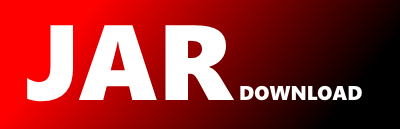
com.pulumi.azurenative.azuresphere.kotlin.AzuresphereFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azuresphere.kotlin
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getCatalogPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getDeploymentPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getDeviceGroupPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getDevicePlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getImagePlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.getProductPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.listCatalogDeploymentsPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.listCatalogDeviceGroupsPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.listCatalogDeviceInsightsPlain
import com.pulumi.azurenative.azuresphere.AzuresphereFunctions.listCatalogDevicesPlain
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetCatalogPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetCatalogPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeploymentPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeploymentPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeviceGroupPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeviceGroupPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDevicePlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDevicePlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetImagePlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetImagePlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetProductPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.GetProductPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeploymentsPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeploymentsPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceGroupsPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceGroupsPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceInsightsPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceInsightsPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDevicesPlainArgs
import com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDevicesPlainArgsBuilder
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetCatalogResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeploymentResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeviceGroupResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeviceResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetImageResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetProductResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeploymentsResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeviceGroupsResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeviceInsightsResult
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDevicesResult
import kotlinx.coroutines.future.await
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetCatalogResult.Companion.toKotlin as getCatalogResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeploymentResult.Companion.toKotlin as getDeploymentResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeviceGroupResult.Companion.toKotlin as getDeviceGroupResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetDeviceResult.Companion.toKotlin as getDeviceResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetImageResult.Companion.toKotlin as getImageResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.GetProductResult.Companion.toKotlin as getProductResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeploymentsResult.Companion.toKotlin as listCatalogDeploymentsResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeviceGroupsResult.Companion.toKotlin as listCatalogDeviceGroupsResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDeviceInsightsResult.Companion.toKotlin as listCatalogDeviceInsightsResultToKotlin
import com.pulumi.azurenative.azuresphere.kotlin.outputs.ListCatalogDevicesResult.Companion.toKotlin as listCatalogDevicesResultToKotlin
public object AzuresphereFunctions {
/**
* Get a Catalog
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An Azure Sphere catalog
*/
public suspend fun getCatalog(argument: GetCatalogPlainArgs): GetCatalogResult =
getCatalogResultToKotlin(getCatalogPlain(argument.toJava()).await())
/**
* @see [getCatalog].
* @param catalogName Name of catalog
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An Azure Sphere catalog
*/
public suspend fun getCatalog(catalogName: String, resourceGroupName: String): GetCatalogResult {
val argument = GetCatalogPlainArgs(
catalogName = catalogName,
resourceGroupName = resourceGroupName,
)
return getCatalogResultToKotlin(getCatalogPlain(argument.toJava()).await())
}
/**
* @see [getCatalog].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetCatalogPlainArgs].
* @return An Azure Sphere catalog
*/
public suspend fun getCatalog(argument: suspend GetCatalogPlainArgsBuilder.() -> Unit): GetCatalogResult {
val builder = GetCatalogPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCatalogResultToKotlin(getCatalogPlain(builtArgument.toJava()).await())
}
/**
* Get a Deployment. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An deployment resource belonging to a device group resource.
*/
public suspend fun getDeployment(argument: GetDeploymentPlainArgs): GetDeploymentResult =
getDeploymentResultToKotlin(getDeploymentPlain(argument.toJava()).await())
/**
* @see [getDeployment].
* @param catalogName Name of catalog
* @param deploymentName Deployment name. Use .default for deployment creation and to get the current deployment for the associated device group.
* @param deviceGroupName Name of device group.
* @param productName Name of product.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An deployment resource belonging to a device group resource.
*/
public suspend fun getDeployment(
catalogName: String,
deploymentName: String,
deviceGroupName: String,
productName: String,
resourceGroupName: String,
): GetDeploymentResult {
val argument = GetDeploymentPlainArgs(
catalogName = catalogName,
deploymentName = deploymentName,
deviceGroupName = deviceGroupName,
productName = productName,
resourceGroupName = resourceGroupName,
)
return getDeploymentResultToKotlin(getDeploymentPlain(argument.toJava()).await())
}
/**
* @see [getDeployment].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeploymentPlainArgs].
* @return An deployment resource belonging to a device group resource.
*/
public suspend fun getDeployment(argument: suspend GetDeploymentPlainArgsBuilder.() -> Unit): GetDeploymentResult {
val builder = GetDeploymentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDeploymentResultToKotlin(getDeploymentPlain(builtArgument.toJava()).await())
}
/**
* Get a Device. Use '.unassigned' or '.default' for the device group and product names when a device does not belong to a device group and product.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An device resource belonging to a device group resource.
*/
public suspend fun getDevice(argument: GetDevicePlainArgs): GetDeviceResult =
getDeviceResultToKotlin(getDevicePlain(argument.toJava()).await())
/**
* @see [getDevice].
* @param catalogName Name of catalog
* @param deviceGroupName Name of device group.
* @param deviceName Device name
* @param productName Name of product.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An device resource belonging to a device group resource.
*/
public suspend fun getDevice(
catalogName: String,
deviceGroupName: String,
deviceName: String,
productName: String,
resourceGroupName: String,
): GetDeviceResult {
val argument = GetDevicePlainArgs(
catalogName = catalogName,
deviceGroupName = deviceGroupName,
deviceName = deviceName,
productName = productName,
resourceGroupName = resourceGroupName,
)
return getDeviceResultToKotlin(getDevicePlain(argument.toJava()).await())
}
/**
* @see [getDevice].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDevicePlainArgs].
* @return An device resource belonging to a device group resource.
*/
public suspend fun getDevice(argument: suspend GetDevicePlainArgsBuilder.() -> Unit): GetDeviceResult {
val builder = GetDevicePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDeviceResultToKotlin(getDevicePlain(builtArgument.toJava()).await())
}
/**
* Get a DeviceGroup. '.default' and '.unassigned' are system defined values and cannot be used for product or device group name.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An device group resource belonging to a product resource.
*/
public suspend fun getDeviceGroup(argument: GetDeviceGroupPlainArgs): GetDeviceGroupResult =
getDeviceGroupResultToKotlin(getDeviceGroupPlain(argument.toJava()).await())
/**
* @see [getDeviceGroup].
* @param catalogName Name of catalog
* @param deviceGroupName Name of device group.
* @param productName Name of product.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An device group resource belonging to a product resource.
*/
public suspend fun getDeviceGroup(
catalogName: String,
deviceGroupName: String,
productName: String,
resourceGroupName: String,
): GetDeviceGroupResult {
val argument = GetDeviceGroupPlainArgs(
catalogName = catalogName,
deviceGroupName = deviceGroupName,
productName = productName,
resourceGroupName = resourceGroupName,
)
return getDeviceGroupResultToKotlin(getDeviceGroupPlain(argument.toJava()).await())
}
/**
* @see [getDeviceGroup].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetDeviceGroupPlainArgs].
* @return An device group resource belonging to a product resource.
*/
public suspend fun getDeviceGroup(argument: suspend GetDeviceGroupPlainArgsBuilder.() -> Unit): GetDeviceGroupResult {
val builder = GetDeviceGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getDeviceGroupResultToKotlin(getDeviceGroupPlain(builtArgument.toJava()).await())
}
/**
* Get a Image
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An image resource belonging to a catalog resource.
*/
public suspend fun getImage(argument: GetImagePlainArgs): GetImageResult =
getImageResultToKotlin(getImagePlain(argument.toJava()).await())
/**
* @see [getImage].
* @param catalogName Name of catalog
* @param imageName Image name. Use an image GUID for GA versions of the API.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An image resource belonging to a catalog resource.
*/
public suspend fun getImage(
catalogName: String,
imageName: String,
resourceGroupName: String,
): GetImageResult {
val argument = GetImagePlainArgs(
catalogName = catalogName,
imageName = imageName,
resourceGroupName = resourceGroupName,
)
return getImageResultToKotlin(getImagePlain(argument.toJava()).await())
}
/**
* @see [getImage].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetImagePlainArgs].
* @return An image resource belonging to a catalog resource.
*/
public suspend fun getImage(argument: suspend GetImagePlainArgsBuilder.() -> Unit): GetImageResult {
val builder = GetImagePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getImageResultToKotlin(getImagePlain(builtArgument.toJava()).await())
}
/**
* Get a Product. '.default' and '.unassigned' are system defined values and cannot be used for product name.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return An product resource belonging to a catalog resource.
*/
public suspend fun getProduct(argument: GetProductPlainArgs): GetProductResult =
getProductResultToKotlin(getProductPlain(argument.toJava()).await())
/**
* @see [getProduct].
* @param catalogName Name of catalog
* @param productName Name of product.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @return An product resource belonging to a catalog resource.
*/
public suspend fun getProduct(
catalogName: String,
productName: String,
resourceGroupName: String,
): GetProductResult {
val argument = GetProductPlainArgs(
catalogName = catalogName,
productName = productName,
resourceGroupName = resourceGroupName,
)
return getProductResultToKotlin(getProductPlain(argument.toJava()).await())
}
/**
* @see [getProduct].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.GetProductPlainArgs].
* @return An product resource belonging to a catalog resource.
*/
public suspend fun getProduct(argument: suspend GetProductPlainArgsBuilder.() -> Unit): GetProductResult {
val builder = GetProductPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getProductResultToKotlin(getProductPlain(builtArgument.toJava()).await())
}
/**
* Lists deployments for catalog.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return The response of a Deployment list operation.
*/
public suspend fun listCatalogDeployments(argument: ListCatalogDeploymentsPlainArgs): ListCatalogDeploymentsResult =
listCatalogDeploymentsResultToKotlin(listCatalogDeploymentsPlain(argument.toJava()).await())
/**
* @see [listCatalogDeployments].
* @param catalogName Name of catalog
* @param filter Filter the result list using the given expression
* @param maxpagesize The maximum number of result items per page.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param skip The number of result items to skip.
* @param top The number of result items to return.
* @return The response of a Deployment list operation.
*/
public suspend fun listCatalogDeployments(
catalogName: String,
filter: String? = null,
maxpagesize: Int? = null,
resourceGroupName: String,
skip: Int? = null,
top: Int? = null,
): ListCatalogDeploymentsResult {
val argument = ListCatalogDeploymentsPlainArgs(
catalogName = catalogName,
filter = filter,
maxpagesize = maxpagesize,
resourceGroupName = resourceGroupName,
skip = skip,
top = top,
)
return listCatalogDeploymentsResultToKotlin(listCatalogDeploymentsPlain(argument.toJava()).await())
}
/**
* @see [listCatalogDeployments].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeploymentsPlainArgs].
* @return The response of a Deployment list operation.
*/
public suspend fun listCatalogDeployments(argument: suspend ListCatalogDeploymentsPlainArgsBuilder.() -> Unit): ListCatalogDeploymentsResult {
val builder = ListCatalogDeploymentsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listCatalogDeploymentsResultToKotlin(listCatalogDeploymentsPlain(builtArgument.toJava()).await())
}
/**
* List the device groups for the catalog.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return The response of a DeviceGroup list operation.
*/
public suspend fun listCatalogDeviceGroups(argument: ListCatalogDeviceGroupsPlainArgs): ListCatalogDeviceGroupsResult =
listCatalogDeviceGroupsResultToKotlin(listCatalogDeviceGroupsPlain(argument.toJava()).await())
/**
* @see [listCatalogDeviceGroups].
* @param catalogName Name of catalog
* @param deviceGroupName Device Group name.
* @param filter Filter the result list using the given expression
* @param maxpagesize The maximum number of result items per page.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param skip The number of result items to skip.
* @param top The number of result items to return.
* @return The response of a DeviceGroup list operation.
*/
public suspend fun listCatalogDeviceGroups(
catalogName: String,
deviceGroupName: String? = null,
filter: String? = null,
maxpagesize: Int? = null,
resourceGroupName: String,
skip: Int? = null,
top: Int? = null,
): ListCatalogDeviceGroupsResult {
val argument = ListCatalogDeviceGroupsPlainArgs(
catalogName = catalogName,
deviceGroupName = deviceGroupName,
filter = filter,
maxpagesize = maxpagesize,
resourceGroupName = resourceGroupName,
skip = skip,
top = top,
)
return listCatalogDeviceGroupsResultToKotlin(listCatalogDeviceGroupsPlain(argument.toJava()).await())
}
/**
* @see [listCatalogDeviceGroups].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceGroupsPlainArgs].
* @return The response of a DeviceGroup list operation.
*/
public suspend fun listCatalogDeviceGroups(argument: suspend ListCatalogDeviceGroupsPlainArgsBuilder.() -> Unit): ListCatalogDeviceGroupsResult {
val builder = ListCatalogDeviceGroupsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listCatalogDeviceGroupsResultToKotlin(listCatalogDeviceGroupsPlain(builtArgument.toJava()).await())
}
/**
* Lists device insights for catalog.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return Paged collection of DeviceInsight items
*/
public suspend fun listCatalogDeviceInsights(argument: ListCatalogDeviceInsightsPlainArgs): ListCatalogDeviceInsightsResult =
listCatalogDeviceInsightsResultToKotlin(listCatalogDeviceInsightsPlain(argument.toJava()).await())
/**
* @see [listCatalogDeviceInsights].
* @param catalogName Name of catalog
* @param filter Filter the result list using the given expression
* @param maxpagesize The maximum number of result items per page.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param skip The number of result items to skip.
* @param top The number of result items to return.
* @return Paged collection of DeviceInsight items
*/
public suspend fun listCatalogDeviceInsights(
catalogName: String,
filter: String? = null,
maxpagesize: Int? = null,
resourceGroupName: String,
skip: Int? = null,
top: Int? = null,
): ListCatalogDeviceInsightsResult {
val argument = ListCatalogDeviceInsightsPlainArgs(
catalogName = catalogName,
filter = filter,
maxpagesize = maxpagesize,
resourceGroupName = resourceGroupName,
skip = skip,
top = top,
)
return listCatalogDeviceInsightsResultToKotlin(listCatalogDeviceInsightsPlain(argument.toJava()).await())
}
/**
* @see [listCatalogDeviceInsights].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDeviceInsightsPlainArgs].
* @return Paged collection of DeviceInsight items
*/
public suspend fun listCatalogDeviceInsights(argument: suspend ListCatalogDeviceInsightsPlainArgsBuilder.() -> Unit): ListCatalogDeviceInsightsResult {
val builder = ListCatalogDeviceInsightsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listCatalogDeviceInsightsResultToKotlin(listCatalogDeviceInsightsPlain(builtArgument.toJava()).await())
}
/**
* Lists devices for catalog.
* Azure REST API version: 2022-09-01-preview.
* Other available API versions: 2024-04-01.
* @param argument null
* @return The response of a Device list operation.
*/
public suspend fun listCatalogDevices(argument: ListCatalogDevicesPlainArgs): ListCatalogDevicesResult =
listCatalogDevicesResultToKotlin(listCatalogDevicesPlain(argument.toJava()).await())
/**
* @see [listCatalogDevices].
* @param catalogName Name of catalog
* @param filter Filter the result list using the given expression
* @param maxpagesize The maximum number of result items per page.
* @param resourceGroupName The name of the resource group. The name is case insensitive.
* @param skip The number of result items to skip.
* @param top The number of result items to return.
* @return The response of a Device list operation.
*/
public suspend fun listCatalogDevices(
catalogName: String,
filter: String? = null,
maxpagesize: Int? = null,
resourceGroupName: String,
skip: Int? = null,
top: Int? = null,
): ListCatalogDevicesResult {
val argument = ListCatalogDevicesPlainArgs(
catalogName = catalogName,
filter = filter,
maxpagesize = maxpagesize,
resourceGroupName = resourceGroupName,
skip = skip,
top = top,
)
return listCatalogDevicesResultToKotlin(listCatalogDevicesPlain(argument.toJava()).await())
}
/**
* @see [listCatalogDevices].
* @param argument Builder for [com.pulumi.azurenative.azuresphere.kotlin.inputs.ListCatalogDevicesPlainArgs].
* @return The response of a Device list operation.
*/
public suspend fun listCatalogDevices(argument: suspend ListCatalogDevicesPlainArgsBuilder.() -> Unit): ListCatalogDevicesResult {
val builder = ListCatalogDevicesPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listCatalogDevicesResultToKotlin(listCatalogDevicesPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy