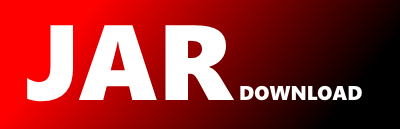
com.pulumi.azurenative.azurestackhci.kotlin.DeploymentSetting.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurestackhci.kotlin
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.DeploymentConfigurationResponse
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.ReportedPropertiesResponse
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.DeploymentConfigurationResponse.Companion.toKotlin as deploymentConfigurationResponseToKotlin
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.ReportedPropertiesResponse.Companion.toKotlin as reportedPropertiesResponseToKotlin
import com.pulumi.azurenative.azurestackhci.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [DeploymentSetting].
*/
@PulumiTagMarker
public class DeploymentSettingResourceBuilder internal constructor() {
public var name: String? = null
public var args: DeploymentSettingArgs = DeploymentSettingArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DeploymentSettingArgsBuilder.() -> Unit) {
val builder = DeploymentSettingArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): DeploymentSetting {
val builtJavaResource =
com.pulumi.azurenative.azurestackhci.DeploymentSetting(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return DeploymentSetting(builtJavaResource)
}
}
/**
* Edge device resource
* Azure REST API version: 2023-08-01-preview.
* Other available API versions: 2023-11-01-preview, 2024-01-01, 2024-02-15-preview, 2024-04-01.
* ## Example Usage
* ### Create Deployment Settings
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var deploymentSetting = new AzureNative.AzureStackHCI.DeploymentSetting("deploymentSetting", new()
* {
* ArcNodeResourceIds = new[]
* {
* "/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1",
* "/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-2",
* },
* ClusterName = "myCluster",
* DeploymentConfiguration = new AzureNative.AzureStackHCI.Inputs.DeploymentConfigurationArgs
* {
* ScaleUnits = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.ScaleUnitsArgs
* {
* DeploymentData = new AzureNative.AzureStackHCI.Inputs.DeploymentDataArgs
* {
* AdouPath = "OU=ms169,DC=ASZ1PLab8,DC=nttest,DC=microsoft,DC=com",
* Cluster = new AzureNative.AzureStackHCI.Inputs.ClusterArgs
* {
* AzureServiceEndpoint = "core.windows.net",
* CloudAccountName = "myasestoragacct",
* Name = "testHCICluster",
* WitnessPath = "Cloud",
* WitnessType = "Cloud",
* },
* DomainFqdn = "ASZ1PLab8.nttest.microsoft.com",
* HostNetwork = new AzureNative.AzureStackHCI.Inputs.HostNetworkArgs
* {
* Intents = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.IntentsArgs
* {
* Adapter = new[]
* {
* "Port2",
* },
* AdapterPropertyOverrides = new AzureNative.AzureStackHCI.Inputs.AdapterPropertyOverridesArgs
* {
* JumboPacket = "1514",
* NetworkDirect = "Enabled",
* NetworkDirectTechnology = "iWARP",
* },
* Name = "Compute_Management",
* OverrideAdapterProperty = false,
* OverrideQosPolicy = false,
* OverrideVirtualSwitchConfiguration = false,
* QosPolicyOverrides = new AzureNative.AzureStackHCI.Inputs.QosPolicyOverridesArgs
* {
* BandwidthPercentageSMB = "50",
* PriorityValue8021ActionCluster = "7",
* PriorityValue8021ActionSMB = "3",
* },
* TrafficType = new[]
* {
* "Compute",
* "Management",
* },
* VirtualSwitchConfigurationOverrides = new AzureNative.AzureStackHCI.Inputs.VirtualSwitchConfigurationOverridesArgs
* {
* EnableIov = "True",
* LoadBalancingAlgorithm = "HyperVPort",
* },
* },
* },
* StorageConnectivitySwitchless = true,
* StorageNetworks = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.StorageNetworksArgs
* {
* Name = "Storage1Network",
* NetworkAdapterName = "Port3",
* VlanId = "5",
* },
* },
* },
* InfrastructureNetwork = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.InfrastructureNetworkArgs
* {
* DnsServers = new[]
* {
* "10.57.50.90",
* },
* Gateway = "255.255.248.0",
* IpPools = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.IpPoolsArgs
* {
* EndingAddress = "10.57.48.66",
* StartingAddress = "10.57.48.60",
* },
* },
* SubnetMask = "255.255.248.0",
* },
* },
* NamingPrefix = "ms169",
* Observability = new AzureNative.AzureStackHCI.Inputs.ObservabilityArgs
* {
* EpisodicDataUpload = true,
* EuLocation = false,
* StreamingDataClient = true,
* },
* OptionalServices = new AzureNative.AzureStackHCI.Inputs.OptionalServicesArgs
* {
* CustomLocation = "customLocationName",
* },
* PhysicalNodes = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.PhysicalNodesArgs
* {
* Ipv4Address = "10.57.51.224",
* Name = "ms169host",
* },
* new AzureNative.AzureStackHCI.Inputs.PhysicalNodesArgs
* {
* Ipv4Address = "10.57.53.236",
* Name = "ms154host",
* },
* },
* SecretsLocation = "/subscriptions/db4e2fdb-6d80-4e6e-b7cd-xxxxxxx/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/abcd123",
* SecuritySettings = new AzureNative.AzureStackHCI.Inputs.SecuritySettingsArgs
* {
* BitlockerBootVolume = true,
* BitlockerDataVolumes = true,
* CredentialGuardEnforced = false,
* DriftControlEnforced = true,
* DrtmProtection = true,
* HvciProtection = true,
* SideChannelMitigationEnforced = true,
* SmbClusterEncryption = false,
* SmbSigningEnforced = true,
* WdacEnforced = true,
* },
* Storage = new AzureNative.AzureStackHCI.Inputs.StorageArgs
* {
* ConfigurationMode = "Express",
* },
* },
* },
* },
* Version = "string",
* },
* DeploymentMode = AzureNative.AzureStackHCI.DeploymentMode.Deploy,
* DeploymentSettingsName = "default",
* ResourceGroupName = "test-rg",
* });
* });
* ```
* ```go
* package main
* import (
* azurestackhci "github.com/pulumi/pulumi-azure-native-sdk/azurestackhci/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azurestackhci.NewDeploymentSetting(ctx, "deploymentSetting", &azurestackhci.DeploymentSettingArgs{
* ArcNodeResourceIds: pulumi.StringArray{
* pulumi.String("/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1"),
* pulumi.String("/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-2"),
* },
* ClusterName: pulumi.String("myCluster"),
* DeploymentConfiguration: &azurestackhci.DeploymentConfigurationArgs{
* ScaleUnits: azurestackhci.ScaleUnitsArray{
* &azurestackhci.ScaleUnitsArgs{
* DeploymentData: &azurestackhci.DeploymentDataArgs{
* AdouPath: pulumi.String("OU=ms169,DC=ASZ1PLab8,DC=nttest,DC=microsoft,DC=com"),
* Cluster: &azurestackhci.ClusterTypeArgs{
* AzureServiceEndpoint: pulumi.String("core.windows.net"),
* CloudAccountName: pulumi.String("myasestoragacct"),
* Name: pulumi.String("testHCICluster"),
* WitnessPath: pulumi.String("Cloud"),
* WitnessType: pulumi.String("Cloud"),
* },
* DomainFqdn: pulumi.String("ASZ1PLab8.nttest.microsoft.com"),
* HostNetwork: &azurestackhci.HostNetworkArgs{
* Intents: azurestackhci.IntentsArray{
* &azurestackhci.IntentsArgs{
* Adapter: pulumi.StringArray{
* pulumi.String("Port2"),
* },
* AdapterPropertyOverrides: &azurestackhci.AdapterPropertyOverridesArgs{
* JumboPacket: pulumi.String("1514"),
* NetworkDirect: pulumi.String("Enabled"),
* NetworkDirectTechnology: pulumi.String("iWARP"),
* },
* Name: pulumi.String("Compute_Management"),
* OverrideAdapterProperty: pulumi.Bool(false),
* OverrideQosPolicy: pulumi.Bool(false),
* OverrideVirtualSwitchConfiguration: pulumi.Bool(false),
* QosPolicyOverrides: &azurestackhci.QosPolicyOverridesArgs{
* BandwidthPercentageSMB: pulumi.String("50"),
* PriorityValue8021ActionCluster: pulumi.String("7"),
* PriorityValue8021ActionSMB: pulumi.String("3"),
* },
* TrafficType: pulumi.StringArray{
* pulumi.String("Compute"),
* pulumi.String("Management"),
* },
* VirtualSwitchConfigurationOverrides: &azurestackhci.VirtualSwitchConfigurationOverridesArgs{
* EnableIov: pulumi.String("True"),
* LoadBalancingAlgorithm: pulumi.String("HyperVPort"),
* },
* },
* },
* StorageConnectivitySwitchless: pulumi.Bool(true),
* StorageNetworks: azurestackhci.StorageNetworksArray{
* &azurestackhci.StorageNetworksArgs{
* Name: pulumi.String("Storage1Network"),
* NetworkAdapterName: pulumi.String("Port3"),
* VlanId: pulumi.String("5"),
* },
* },
* },
* InfrastructureNetwork: azurestackhci.InfrastructureNetworkArray{
* &azurestackhci.InfrastructureNetworkArgs{
* DnsServers: pulumi.StringArray{
* pulumi.String("10.57.50.90"),
* },
* Gateway: pulumi.String("255.255.248.0"),
* IpPools: azurestackhci.IpPoolsArray{
* &azurestackhci.IpPoolsArgs{
* EndingAddress: pulumi.String("10.57.48.66"),
* StartingAddress: pulumi.String("10.57.48.60"),
* },
* },
* SubnetMask: pulumi.String("255.255.248.0"),
* },
* },
* NamingPrefix: pulumi.String("ms169"),
* Observability: &azurestackhci.ObservabilityArgs{
* EpisodicDataUpload: pulumi.Bool(true),
* EuLocation: pulumi.Bool(false),
* StreamingDataClient: pulumi.Bool(true),
* },
* OptionalServices: &azurestackhci.OptionalServicesArgs{
* CustomLocation: pulumi.String("customLocationName"),
* },
* PhysicalNodes: azurestackhci.PhysicalNodesArray{
* &azurestackhci.PhysicalNodesArgs{
* Ipv4Address: pulumi.String("10.57.51.224"),
* Name: pulumi.String("ms169host"),
* },
* &azurestackhci.PhysicalNodesArgs{
* Ipv4Address: pulumi.String("10.57.53.236"),
* Name: pulumi.String("ms154host"),
* },
* },
* SecretsLocation: pulumi.String("/subscriptions/db4e2fdb-6d80-4e6e-b7cd-xxxxxxx/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/abcd123"),
* SecuritySettings: &azurestackhci.SecuritySettingsArgs{
* BitlockerBootVolume: pulumi.Bool(true),
* BitlockerDataVolumes: pulumi.Bool(true),
* CredentialGuardEnforced: pulumi.Bool(false),
* DriftControlEnforced: pulumi.Bool(true),
* DrtmProtection: pulumi.Bool(true),
* HvciProtection: pulumi.Bool(true),
* SideChannelMitigationEnforced: pulumi.Bool(true),
* SmbClusterEncryption: pulumi.Bool(false),
* SmbSigningEnforced: pulumi.Bool(true),
* WdacEnforced: pulumi.Bool(true),
* },
* Storage: &azurestackhci.StorageArgs{
* ConfigurationMode: pulumi.String("Express"),
* },
* },
* },
* },
* Version: pulumi.String("string"),
* },
* DeploymentMode: pulumi.String(azurestackhci.DeploymentModeDeploy),
* DeploymentSettingsName: pulumi.String("default"),
* ResourceGroupName: pulumi.String("test-rg"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.DeploymentSetting;
* import com.pulumi.azurenative.azurestackhci.DeploymentSettingArgs;
* import com.pulumi.azurenative.azurestackhci.inputs.DeploymentConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var deploymentSetting = new DeploymentSetting("deploymentSetting", DeploymentSettingArgs.builder()
* .arcNodeResourceIds(
* "/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1",
* "/subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-2")
* .clusterName("myCluster")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .scaleUnits(ScaleUnitsArgs.builder()
* .deploymentData(DeploymentDataArgs.builder()
* .adouPath("OU=ms169,DC=ASZ1PLab8,DC=nttest,DC=microsoft,DC=com")
* .cluster(ClusterArgs.builder()
* .azureServiceEndpoint("core.windows.net")
* .cloudAccountName("myasestoragacct")
* .name("testHCICluster")
* .witnessPath("Cloud")
* .witnessType("Cloud")
* .build())
* .domainFqdn("ASZ1PLab8.nttest.microsoft.com")
* .hostNetwork(HostNetworkArgs.builder()
* .intents(IntentsArgs.builder()
* .adapter("Port2")
* .adapterPropertyOverrides(AdapterPropertyOverridesArgs.builder()
* .jumboPacket("1514")
* .networkDirect("Enabled")
* .networkDirectTechnology("iWARP")
* .build())
* .name("Compute_Management")
* .overrideAdapterProperty(false)
* .overrideQosPolicy(false)
* .overrideVirtualSwitchConfiguration(false)
* .qosPolicyOverrides(QosPolicyOverridesArgs.builder()
* .bandwidthPercentageSMB("50")
* .priorityValue8021ActionCluster("7")
* .priorityValue8021ActionSMB("3")
* .build())
* .trafficType(
* "Compute",
* "Management")
* .virtualSwitchConfigurationOverrides(VirtualSwitchConfigurationOverridesArgs.builder()
* .enableIov("True")
* .loadBalancingAlgorithm("HyperVPort")
* .build())
* .build())
* .storageConnectivitySwitchless(true)
* .storageNetworks(StorageNetworksArgs.builder()
* .name("Storage1Network")
* .networkAdapterName("Port3")
* .vlanId("5")
* .build())
* .build())
* .infrastructureNetwork(InfrastructureNetworkArgs.builder()
* .dnsServers("10.57.50.90")
* .gateway("255.255.248.0")
* .ipPools(IpPoolsArgs.builder()
* .endingAddress("10.57.48.66")
* .startingAddress("10.57.48.60")
* .build())
* .subnetMask("255.255.248.0")
* .build())
* .namingPrefix("ms169")
* .observability(ObservabilityArgs.builder()
* .episodicDataUpload(true)
* .euLocation(false)
* .streamingDataClient(true)
* .build())
* .optionalServices(OptionalServicesArgs.builder()
* .customLocation("customLocationName")
* .build())
* .physicalNodes(
* PhysicalNodesArgs.builder()
* .ipv4Address("10.57.51.224")
* .name("ms169host")
* .build(),
* PhysicalNodesArgs.builder()
* .ipv4Address("10.57.53.236")
* .name("ms154host")
* .build())
* .secretsLocation("/subscriptions/db4e2fdb-6d80-4e6e-b7cd-xxxxxxx/resourceGroups/test-rg/providers/Microsoft.KeyVault/vaults/abcd123")
* .securitySettings(SecuritySettingsArgs.builder()
* .bitlockerBootVolume(true)
* .bitlockerDataVolumes(true)
* .credentialGuardEnforced(false)
* .driftControlEnforced(true)
* .drtmProtection(true)
* .hvciProtection(true)
* .sideChannelMitigationEnforced(true)
* .smbClusterEncryption(false)
* .smbSigningEnforced(true)
* .wdacEnforced(true)
* .build())
* .storage(StorageArgs.builder()
* .configurationMode("Express")
* .build())
* .build())
* .build())
* .version("string")
* .build())
* .deploymentMode("Deploy")
* .deploymentSettingsName("default")
* .resourceGroupName("test-rg")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:azurestackhci:DeploymentSetting default /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AzureStackHCI/clusters/{clusterName}/deploymentSettings/{deploymentSettingsName}
* ```
*/
public class DeploymentSetting internal constructor(
override val javaResource: com.pulumi.azurenative.azurestackhci.DeploymentSetting,
) : KotlinCustomResource(javaResource, DeploymentSettingMapper) {
/**
* Azure resource ids of Arc machines to be part of cluster.
*/
public val arcNodeResourceIds: Output>
get() = javaResource.arcNodeResourceIds().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Scale units will contains list of deployment data
*/
public val deploymentConfiguration: Output
get() = javaResource.deploymentConfiguration().applyValue({ args0 ->
args0.let({ args0 ->
deploymentConfigurationResponseToKotlin(args0)
})
})
/**
* The deployment mode for cluster deployment.
*/
public val deploymentMode: Output
get() = javaResource.deploymentMode().applyValue({ args0 -> args0 })
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* DeploymentSetting provisioning state
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* Deployment Status reported from cluster.
*/
public val reportedProperties: Output
get() = javaResource.reportedProperties().applyValue({ args0 ->
args0.let({ args0 ->
reportedPropertiesResponseToKotlin(args0)
})
})
/**
* Azure Resource Manager metadata containing createdBy and modifiedBy information.
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object DeploymentSettingMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.azurestackhci.DeploymentSetting::class == javaResource::class
override fun map(javaResource: Resource): DeploymentSetting = DeploymentSetting(
javaResource as
com.pulumi.azurenative.azurestackhci.DeploymentSetting,
)
}
/**
* @see [DeploymentSetting].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [DeploymentSetting].
*/
public suspend fun deploymentSetting(
name: String,
block: suspend DeploymentSettingResourceBuilder.() -> Unit,
): DeploymentSetting {
val builder = DeploymentSettingResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [DeploymentSetting].
* @param name The _unique_ name of the resulting resource.
*/
public fun deploymentSetting(name: String): DeploymentSetting {
val builder = DeploymentSettingResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy