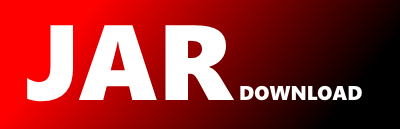
com.pulumi.azurenative.azurestackhci.kotlin.EdgeDeviceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurestackhci.kotlin
import com.pulumi.azurenative.azurestackhci.EdgeDeviceArgs.builder
import com.pulumi.azurenative.azurestackhci.kotlin.inputs.DeviceConfigurationArgs
import com.pulumi.azurenative.azurestackhci.kotlin.inputs.DeviceConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Edge device resource
* Azure REST API version: 2023-08-01-preview.
* Other available API versions: 2023-11-01-preview, 2024-01-01, 2024-02-15-preview, 2024-04-01.
* ## Example Usage
* ### Create Edge Device
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var edgeDevice = new AzureNative.AzureStackHCI.EdgeDevice("edgeDevice", new()
* {
* DeviceConfiguration = new AzureNative.AzureStackHCI.Inputs.DeviceConfigurationArgs
* {
* DeviceMetadata = "",
* NicDetails = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.NicDetailArgs
* {
* AdapterName = "ethernet",
* ComponentId = "VMBUS{f8615163-df3e-46c5-913f-f2d2f965ed0g} ",
* DefaultGateway = "10.10.10.1",
* DefaultIsolationId = "0",
* DnsServers = new[]
* {
* "100.10.10.1",
* },
* DriverVersion = "10.0.20348.1547 ",
* InterfaceDescription = "NDIS 6.70 ",
* Ip4Address = "10.10.10.10",
* SubnetMask = "255.255.255.0",
* },
* },
* },
* EdgeDeviceName = "default",
* ResourceUri = "subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1",
* });
* });
* ```
* ```go
* package main
* import (
* azurestackhci "github.com/pulumi/pulumi-azure-native-sdk/azurestackhci/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azurestackhci.NewEdgeDevice(ctx, "edgeDevice", &azurestackhci.EdgeDeviceArgs{
* DeviceConfiguration: &azurestackhci.DeviceConfigurationArgs{
* DeviceMetadata: pulumi.String(""),
* NicDetails: azurestackhci.NicDetailArray{
* &azurestackhci.NicDetailArgs{
* AdapterName: pulumi.String("ethernet"),
* ComponentId: pulumi.String("VMBUS{f8615163-df3e-46c5-913f-f2d2f965ed0g} "),
* DefaultGateway: pulumi.String("10.10.10.1"),
* DefaultIsolationId: pulumi.String("0"),
* DnsServers: pulumi.StringArray{
* pulumi.String("100.10.10.1"),
* },
* DriverVersion: pulumi.String("10.0.20348.1547 "),
* InterfaceDescription: pulumi.String("NDIS 6.70 "),
* Ip4Address: pulumi.String("10.10.10.10"),
* SubnetMask: pulumi.String("255.255.255.0"),
* },
* },
* },
* EdgeDeviceName: pulumi.String("default"),
* ResourceUri: pulumi.String("subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.EdgeDevice;
* import com.pulumi.azurenative.azurestackhci.EdgeDeviceArgs;
* import com.pulumi.azurenative.azurestackhci.inputs.DeviceConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var edgeDevice = new EdgeDevice("edgeDevice", EdgeDeviceArgs.builder()
* .deviceConfiguration(DeviceConfigurationArgs.builder()
* .deviceMetadata("")
* .nicDetails(NicDetailArgs.builder()
* .adapterName("ethernet")
* .componentId("VMBUS{f8615163-df3e-46c5-913f-f2d2f965ed0g} ")
* .defaultGateway("10.10.10.1")
* .defaultIsolationId("0")
* .dnsServers("100.10.10.1")
* .driverVersion("10.0.20348.1547 ")
* .interfaceDescription("NDIS 6.70 ")
* .ip4Address("10.10.10.10")
* .subnetMask("255.255.255.0")
* .build())
* .build())
* .edgeDeviceName("default")
* .resourceUri("subscriptions/fd3c3665-1729-4b7b-9a38-238e83b0f98b/resourceGroups/ArcInstance-rg/providers/Microsoft.HybridCompute/machines/Node-1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:azurestackhci:EdgeDevice default /{resourceUri}/providers/Microsoft.AzureStackHCI/edgeDevices/{edgeDeviceName}
* ```
* @property deviceConfiguration Device Configuration
* @property edgeDeviceName Name of Device
* @property resourceUri The fully qualified Azure Resource manager identifier of the resource.
*/
public data class EdgeDeviceArgs(
public val deviceConfiguration: Output? = null,
public val edgeDeviceName: Output? = null,
public val resourceUri: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurestackhci.EdgeDeviceArgs =
com.pulumi.azurenative.azurestackhci.EdgeDeviceArgs.builder()
.deviceConfiguration(
deviceConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.edgeDeviceName(edgeDeviceName?.applyValue({ args0 -> args0 }))
.resourceUri(resourceUri?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EdgeDeviceArgs].
*/
@PulumiTagMarker
public class EdgeDeviceArgsBuilder internal constructor() {
private var deviceConfiguration: Output? = null
private var edgeDeviceName: Output? = null
private var resourceUri: Output? = null
/**
* @param value Device Configuration
*/
@JvmName("eefdnpijrdigdrgf")
public suspend fun deviceConfiguration(`value`: Output) {
this.deviceConfiguration = value
}
/**
* @param value Name of Device
*/
@JvmName("esnibeasrxagcnhx")
public suspend fun edgeDeviceName(`value`: Output) {
this.edgeDeviceName = value
}
/**
* @param value The fully qualified Azure Resource manager identifier of the resource.
*/
@JvmName("qqreqnbefyhlgnwe")
public suspend fun resourceUri(`value`: Output) {
this.resourceUri = value
}
/**
* @param value Device Configuration
*/
@JvmName("yrhpdsoeweygutaa")
public suspend fun deviceConfiguration(`value`: DeviceConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deviceConfiguration = mapped
}
/**
* @param argument Device Configuration
*/
@JvmName("wfjiwuuetmpbdmhm")
public suspend fun deviceConfiguration(argument: suspend DeviceConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = DeviceConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.deviceConfiguration = mapped
}
/**
* @param value Name of Device
*/
@JvmName("enctwcitofeqncqp")
public suspend fun edgeDeviceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.edgeDeviceName = mapped
}
/**
* @param value The fully qualified Azure Resource manager identifier of the resource.
*/
@JvmName("hhwretyurccankln")
public suspend fun resourceUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceUri = mapped
}
internal fun build(): EdgeDeviceArgs = EdgeDeviceArgs(
deviceConfiguration = deviceConfiguration,
edgeDeviceName = edgeDeviceName,
resourceUri = resourceUri,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy