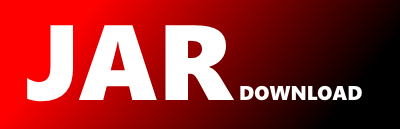
com.pulumi.azurenative.azurestackhci.kotlin.UpdateArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurestackhci.kotlin
import com.pulumi.azurenative.azurestackhci.UpdateArgs.builder
import com.pulumi.azurenative.azurestackhci.kotlin.enums.AvailabilityType
import com.pulumi.azurenative.azurestackhci.kotlin.enums.State
import com.pulumi.azurenative.azurestackhci.kotlin.inputs.UpdatePrerequisiteArgs
import com.pulumi.azurenative.azurestackhci.kotlin.inputs.UpdatePrerequisiteArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Update details
* Azure REST API version: 2023-03-01.
* Other available API versions: 2022-12-15-preview, 2023-06-01, 2023-08-01, 2023-08-01-preview, 2023-11-01-preview, 2024-01-01, 2024-02-15-preview, 2024-04-01.
* ## Example Usage
* ### Put a specific update
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var update = new AzureNative.AzureStackHCI.Update("update", new()
* {
* AdditionalProperties = "additional properties",
* AvailabilityType = AzureNative.AzureStackHCI.AvailabilityType.Local,
* ClusterName = "testcluster",
* Description = "AzS Update 4.2203.2.32",
* DisplayName = "AzS Update - 4.2203.2.32",
* InstalledDate = "2022-04-06T14:08:18.254Z",
* NotifyMessage = "Brief message with instructions for updates of AvailabilityType Notify",
* PackagePath = "\\\\SU1FileServer\\SU1_Infrastructure_2\\Updates\\Packages\\Microsoft4.2203.2.32",
* PackageSizeInMb = 18858,
* PackageType = "Infrastructure",
* Prerequisites = new[]
* {
* new AzureNative.AzureStackHCI.Inputs.UpdatePrerequisiteArgs
* {
* PackageName = "update package name",
* UpdateType = "update type",
* Version = "prerequisite version",
* },
* },
* ProgressPercentage = 0,
* Publisher = "Microsoft",
* ReleaseLink = "https://docs.microsoft.com/azure-stack/operator/release-notes?view=azs-2203",
* ResourceGroupName = "testrg",
* State = AzureNative.AzureStackHCI.State.Installed,
* UpdateName = "Microsoft4.2203.2.32",
* Version = "4.2203.2.32",
* });
* });
* ```
* ```go
* package main
* import (
* azurestackhci "github.com/pulumi/pulumi-azure-native-sdk/azurestackhci/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := azurestackhci.NewUpdate(ctx, "update", &azurestackhci.UpdateArgs{
* AdditionalProperties: pulumi.String("additional properties"),
* AvailabilityType: pulumi.String(azurestackhci.AvailabilityTypeLocal),
* ClusterName: pulumi.String("testcluster"),
* Description: pulumi.String("AzS Update 4.2203.2.32"),
* DisplayName: pulumi.String("AzS Update - 4.2203.2.32"),
* InstalledDate: pulumi.String("2022-04-06T14:08:18.254Z"),
* NotifyMessage: pulumi.String("Brief message with instructions for updates of AvailabilityType Notify"),
* PackagePath: pulumi.String("\\\\SU1FileServer\\SU1_Infrastructure_2\\Updates\\Packages\\Microsoft4.2203.2.32"),
* PackageSizeInMb: pulumi.Float64(18858),
* PackageType: pulumi.String("Infrastructure"),
* Prerequisites: azurestackhci.UpdatePrerequisiteArray{
* &azurestackhci.UpdatePrerequisiteArgs{
* PackageName: pulumi.String("update package name"),
* UpdateType: pulumi.String("update type"),
* Version: pulumi.String("prerequisite version"),
* },
* },
* ProgressPercentage: pulumi.Float64(0),
* Publisher: pulumi.String("Microsoft"),
* ReleaseLink: pulumi.String("https://docs.microsoft.com/azure-stack/operator/release-notes?view=azs-2203"),
* ResourceGroupName: pulumi.String("testrg"),
* State: pulumi.String(azurestackhci.StateInstalled),
* UpdateName: pulumi.String("Microsoft4.2203.2.32"),
* Version: pulumi.String("4.2203.2.32"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.azurestackhci.Update;
* import com.pulumi.azurenative.azurestackhci.UpdateArgs;
* import com.pulumi.azurenative.azurestackhci.inputs.UpdatePrerequisiteArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var update = new Update("update", UpdateArgs.builder()
* .additionalProperties("additional properties")
* .availabilityType("Local")
* .clusterName("testcluster")
* .description("AzS Update 4.2203.2.32")
* .displayName("AzS Update - 4.2203.2.32")
* .installedDate("2022-04-06T14:08:18.254Z")
* .notifyMessage("Brief message with instructions for updates of AvailabilityType Notify")
* .packagePath("\\\\SU1FileServer\\SU1_Infrastructure_2\\Updates\\Packages\\Microsoft4.2203.2.32")
* .packageSizeInMb(18858)
* .packageType("Infrastructure")
* .prerequisites(UpdatePrerequisiteArgs.builder()
* .packageName("update package name")
* .updateType("update type")
* .version("prerequisite version")
* .build())
* .progressPercentage(0)
* .publisher("Microsoft")
* .releaseLink("https://docs.microsoft.com/azure-stack/operator/release-notes?view=azs-2203")
* .resourceGroupName("testrg")
* .state("Installed")
* .updateName("Microsoft4.2203.2.32")
* .version("4.2203.2.32")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:azurestackhci:Update Microsoft4.2203.2.32 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.AzureStackHCI/clusters/{clusterName}/updates/{updateName}
* ```
* @property additionalProperties Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
* @property availabilityType Indicates the way the update content can be downloaded.
* @property clusterName The name of the cluster.
* @property description Description of the update.
* @property displayName Display name of the Update
* @property healthCheckDate Last time the package-specific checks were run.
* @property installedDate Date that the update was installed.
* @property location The geo-location where the resource lives
* @property notifyMessage Brief message with instructions for updates of AvailabilityType Notify.
* @property packagePath Path where the update package is available.
* @property packageSizeInMb Size of the package. This value is a combination of the size from update metadata and size of the payload that results from the live scan operation for OS update content.
* @property packageType Customer-visible type of the update.
* @property prerequisites If update State is HasPrerequisite, this property contains an array of objects describing prerequisite updates before installing this update. Otherwise, it is empty.
* @property progressPercentage Progress percentage of ongoing operation. Currently this property is only valid when the update is in the Downloading state, where it maps to how much of the update content has been downloaded.
* @property publisher Publisher of the update package.
* @property releaseLink Link to release notes for the update.
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property state State of the update as it relates to this stamp.
* @property updateName The name of the Update
* @property version Version of the update.
*/
public data class UpdateArgs(
public val additionalProperties: Output? = null,
public val availabilityType: Output>? = null,
public val clusterName: Output? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val healthCheckDate: Output? = null,
public val installedDate: Output? = null,
public val location: Output? = null,
public val notifyMessage: Output? = null,
public val packagePath: Output? = null,
public val packageSizeInMb: Output? = null,
public val packageType: Output? = null,
public val prerequisites: Output>? = null,
public val progressPercentage: Output? = null,
public val publisher: Output? = null,
public val releaseLink: Output? = null,
public val resourceGroupName: Output? = null,
public val state: Output>? = null,
public val updateName: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurestackhci.UpdateArgs =
com.pulumi.azurenative.azurestackhci.UpdateArgs.builder()
.additionalProperties(additionalProperties?.applyValue({ args0 -> args0 }))
.availabilityType(
availabilityType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.clusterName(clusterName?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.healthCheckDate(healthCheckDate?.applyValue({ args0 -> args0 }))
.installedDate(installedDate?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.notifyMessage(notifyMessage?.applyValue({ args0 -> args0 }))
.packagePath(packagePath?.applyValue({ args0 -> args0 }))
.packageSizeInMb(packageSizeInMb?.applyValue({ args0 -> args0 }))
.packageType(packageType?.applyValue({ args0 -> args0 }))
.prerequisites(
prerequisites?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.progressPercentage(progressPercentage?.applyValue({ args0 -> args0 }))
.publisher(publisher?.applyValue({ args0 -> args0 }))
.releaseLink(releaseLink?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.state(
state?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.updateName(updateName?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [UpdateArgs].
*/
@PulumiTagMarker
public class UpdateArgsBuilder internal constructor() {
private var additionalProperties: Output? = null
private var availabilityType: Output>? = null
private var clusterName: Output? = null
private var description: Output? = null
private var displayName: Output? = null
private var healthCheckDate: Output? = null
private var installedDate: Output? = null
private var location: Output? = null
private var notifyMessage: Output? = null
private var packagePath: Output? = null
private var packageSizeInMb: Output? = null
private var packageType: Output? = null
private var prerequisites: Output>? = null
private var progressPercentage: Output? = null
private var publisher: Output? = null
private var releaseLink: Output? = null
private var resourceGroupName: Output? = null
private var state: Output>? = null
private var updateName: Output? = null
private var version: Output? = null
/**
* @param value Extensible KV pairs serialized as a string. This is currently used to report the stamp OEM family and hardware model information when an update is flagged as Invalid for the stamp based on OEM type.
*/
@JvmName("joospflrsfnsjfka")
public suspend fun additionalProperties(`value`: Output) {
this.additionalProperties = value
}
/**
* @param value Indicates the way the update content can be downloaded.
*/
@JvmName("auvurfxnihmardjb")
public suspend fun availabilityType(`value`: Output>) {
this.availabilityType = value
}
/**
* @param value The name of the cluster.
*/
@JvmName("sboifiofymbfgsgs")
public suspend fun clusterName(`value`: Output) {
this.clusterName = value
}
/**
* @param value Description of the update.
*/
@JvmName("tsqfjeqjqxmewmmk")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Display name of the Update
*/
@JvmName("boqiutonpsijkrvv")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Last time the package-specific checks were run.
*/
@JvmName("moxkjnslcmqqexwr")
public suspend fun healthCheckDate(`value`: Output) {
this.healthCheckDate = value
}
/**
* @param value Date that the update was installed.
*/
@JvmName("ffhqoebfjtcjpygy")
public suspend fun installedDate(`value`: Output) {
this.installedDate = value
}
/**
* @param value The geo-location where the resource lives
*/
@JvmName("pehddbqhwrfxtiii")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Brief message with instructions for updates of AvailabilityType Notify.
*/
@JvmName("xiqqpqgewclxgluq")
public suspend fun notifyMessage(`value`: Output) {
this.notifyMessage = value
}
/**
* @param value Path where the update package is available.
*/
@JvmName("euhvjdcjbpqxduer")
public suspend fun packagePath(`value`: Output) {
this.packagePath = value
}
/**
* @param value Size of the package. This value is a combination of the size from update metadata and size of the payload that results from the live scan operation for OS update content.
*/
@JvmName("krqdduxxnigegncb")
public suspend fun packageSizeInMb(`value`: Output) {
this.packageSizeInMb = value
}
/**
* @param value Customer-visible type of the update.
*/
@JvmName("osmiaouoihscwuyy")
public suspend fun packageType(`value`: Output) {
this.packageType = value
}
/**
* @param value If update State is HasPrerequisite, this property contains an array of objects describing prerequisite updates before installing this update. Otherwise, it is empty.
*/
@JvmName("xrfcklqwmowwoekc")
public suspend fun prerequisites(`value`: Output>) {
this.prerequisites = value
}
@JvmName("aardnkqptywitqtj")
public suspend fun prerequisites(vararg values: Output) {
this.prerequisites = Output.all(values.asList())
}
/**
* @param values If update State is HasPrerequisite, this property contains an array of objects describing prerequisite updates before installing this update. Otherwise, it is empty.
*/
@JvmName("ufjawevbxwupywrp")
public suspend fun prerequisites(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy