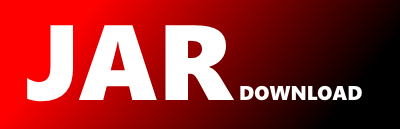
com.pulumi.azurenative.azurestackhci.kotlin.inputs.HostNetworkArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.azurestackhci.kotlin.inputs
import com.pulumi.azurenative.azurestackhci.inputs.HostNetworkArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The HostNetwork of a cluster.
* @property enableStorageAutoIp Optional parameter required only for 3 Nodes Switchless deployments. This allows users to specify IPs and Mask for Storage NICs when Network ATC is not assigning the IPs for storage automatically.
* @property intents The network intents assigned to the network reference pattern used for the deployment. Each intent will define its own name, traffic type, adapter names, and overrides as recommended by your OEM.
* @property storageConnectivitySwitchless Defines how the storage adapters between nodes are connected either switch or switch less..
* @property storageNetworks List of StorageNetworks config to deploy AzureStackHCI Cluster.
*/
public data class HostNetworkArgs(
public val enableStorageAutoIp: Output? = null,
public val intents: Output>? = null,
public val storageConnectivitySwitchless: Output? = null,
public val storageNetworks: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.azurestackhci.inputs.HostNetworkArgs =
com.pulumi.azurenative.azurestackhci.inputs.HostNetworkArgs.builder()
.enableStorageAutoIp(enableStorageAutoIp?.applyValue({ args0 -> args0 }))
.intents(
intents?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.storageConnectivitySwitchless(storageConnectivitySwitchless?.applyValue({ args0 -> args0 }))
.storageNetworks(
storageNetworks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [HostNetworkArgs].
*/
@PulumiTagMarker
public class HostNetworkArgsBuilder internal constructor() {
private var enableStorageAutoIp: Output? = null
private var intents: Output>? = null
private var storageConnectivitySwitchless: Output? = null
private var storageNetworks: Output>? = null
/**
* @param value Optional parameter required only for 3 Nodes Switchless deployments. This allows users to specify IPs and Mask for Storage NICs when Network ATC is not assigning the IPs for storage automatically.
*/
@JvmName("gtbrmtxbtgelrdiv")
public suspend fun enableStorageAutoIp(`value`: Output) {
this.enableStorageAutoIp = value
}
/**
* @param value The network intents assigned to the network reference pattern used for the deployment. Each intent will define its own name, traffic type, adapter names, and overrides as recommended by your OEM.
*/
@JvmName("kdxrrrxcebgulmrr")
public suspend fun intents(`value`: Output>) {
this.intents = value
}
@JvmName("lyyjgqcfkysplccq")
public suspend fun intents(vararg values: Output) {
this.intents = Output.all(values.asList())
}
/**
* @param values The network intents assigned to the network reference pattern used for the deployment. Each intent will define its own name, traffic type, adapter names, and overrides as recommended by your OEM.
*/
@JvmName("dluagwdwpsfbxsyb")
public suspend fun intents(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy