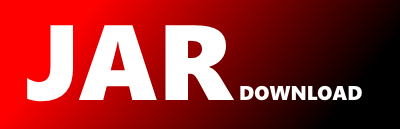
com.pulumi.azurenative.batch.kotlin.PoolArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.batch.kotlin
import com.pulumi.azurenative.batch.PoolArgs.builder
import com.pulumi.azurenative.batch.kotlin.enums.InterNodeCommunicationState
import com.pulumi.azurenative.batch.kotlin.enums.NodeCommunicationMode
import com.pulumi.azurenative.batch.kotlin.inputs.ApplicationPackageReferenceArgs
import com.pulumi.azurenative.batch.kotlin.inputs.ApplicationPackageReferenceArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.BatchPoolIdentityArgs
import com.pulumi.azurenative.batch.kotlin.inputs.BatchPoolIdentityArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.CertificateReferenceArgs
import com.pulumi.azurenative.batch.kotlin.inputs.CertificateReferenceArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.DeploymentConfigurationArgs
import com.pulumi.azurenative.batch.kotlin.inputs.DeploymentConfigurationArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.MetadataItemArgs
import com.pulumi.azurenative.batch.kotlin.inputs.MetadataItemArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.MountConfigurationArgs
import com.pulumi.azurenative.batch.kotlin.inputs.MountConfigurationArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.NetworkConfigurationArgs
import com.pulumi.azurenative.batch.kotlin.inputs.NetworkConfigurationArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.ScaleSettingsArgs
import com.pulumi.azurenative.batch.kotlin.inputs.ScaleSettingsArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.StartTaskArgs
import com.pulumi.azurenative.batch.kotlin.inputs.StartTaskArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.TaskSchedulingPolicyArgs
import com.pulumi.azurenative.batch.kotlin.inputs.TaskSchedulingPolicyArgsBuilder
import com.pulumi.azurenative.batch.kotlin.inputs.UserAccountArgs
import com.pulumi.azurenative.batch.kotlin.inputs.UserAccountArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Contains information about a pool.
* Azure REST API version: 2023-05-01. Prior API version in Azure Native 1.x: 2021-01-01.
* Other available API versions: 2020-05-01, 2023-11-01, 2024-02-01, 2024-07-01.
* ## Example Usage
* ### CreatePool - Custom Image
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1",
* },
* NodeAgentSkuId = "batch.node.ubuntu 18.04",
* },
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* ImageReference: &batch.ImageReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.ubuntu 18.04"),
* },
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .imageReference(ImageReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1")
* .build())
* .nodeAgentSkuId("batch.node.ubuntu 18.04")
* .build())
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - Full CloudServiceConfiguration
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* ApplicationLicenses = new[]
* {
* "app-license0",
* "app-license1",
* },
* ApplicationPackages = new[]
* {
* new AzureNative.Batch.Inputs.ApplicationPackageReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/applications/app_1234",
* Version = "asdf",
* },
* },
* Certificates = new[]
* {
* new AzureNative.Batch.Inputs.CertificateReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/certificates/sha1-1234567",
* StoreLocation = AzureNative.Batch.CertificateStoreLocation.LocalMachine,
* StoreName = "MY",
* Visibility = new[]
* {
* AzureNative.Batch.CertificateVisibility.RemoteUser,
* },
* },
* },
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* CloudServiceConfiguration = new AzureNative.Batch.Inputs.CloudServiceConfigurationArgs
* {
* OsFamily = "4",
* OsVersion = "WA-GUEST-OS-4.45_201708-01",
* },
* },
* DisplayName = "my-pool-name",
* InterNodeCommunication = AzureNative.Batch.InterNodeCommunicationState.Enabled,
* Metadata = new[]
* {
* new AzureNative.Batch.Inputs.MetadataItemArgs
* {
* Name = "metadata-1",
* Value = "value-1",
* },
* new AzureNative.Batch.Inputs.MetadataItemArgs
* {
* Name = "metadata-2",
* Value = "value-2",
* },
* },
* NetworkConfiguration = new AzureNative.Batch.Inputs.NetworkConfigurationArgs
* {
* PublicIPAddressConfiguration = new AzureNative.Batch.Inputs.PublicIPAddressConfigurationArgs
* {
* IpAddressIds = new[]
* {
* "/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135",
* "/subscriptions/subid2/resourceGroups/rg24/providers/Microsoft.Network/publicIPAddresses/ip268",
* },
* Provision = AzureNative.Batch.IPAddressProvisioningType.UserManaged,
* },
* SubnetId = "/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123",
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* FixedScale = new AzureNative.Batch.Inputs.FixedScaleSettingsArgs
* {
* NodeDeallocationOption = AzureNative.Batch.ComputeNodeDeallocationOption.TaskCompletion,
* ResizeTimeout = "PT8M",
* TargetDedicatedNodes = 6,
* TargetLowPriorityNodes = 28,
* },
* },
* StartTask = new AzureNative.Batch.Inputs.StartTaskArgs
* {
* CommandLine = "cmd /c SET",
* EnvironmentSettings = new[]
* {
* new AzureNative.Batch.Inputs.EnvironmentSettingArgs
* {
* Name = "MYSET",
* Value = "1234",
* },
* },
* MaxTaskRetryCount = 6,
* ResourceFiles = new[]
* {
* new AzureNative.Batch.Inputs.ResourceFileArgs
* {
* FileMode = "777",
* FilePath = "c:\\temp\\gohere",
* HttpUrl = "https://testaccount.blob.core.windows.net/example-blob-file",
* },
* },
* UserIdentity = new AzureNative.Batch.Inputs.UserIdentityArgs
* {
* AutoUser = new AzureNative.Batch.Inputs.AutoUserSpecificationArgs
* {
* ElevationLevel = AzureNative.Batch.ElevationLevel.Admin,
* Scope = AzureNative.Batch.AutoUserScope.Pool,
* },
* },
* WaitForSuccess = true,
* },
* TaskSchedulingPolicy = new AzureNative.Batch.Inputs.TaskSchedulingPolicyArgs
* {
* NodeFillType = AzureNative.Batch.ComputeNodeFillType.Pack,
* },
* TaskSlotsPerNode = 13,
* UserAccounts = new[]
* {
* new AzureNative.Batch.Inputs.UserAccountArgs
* {
* ElevationLevel = AzureNative.Batch.ElevationLevel.Admin,
* LinuxUserConfiguration = new AzureNative.Batch.Inputs.LinuxUserConfigurationArgs
* {
* Gid = 4567,
* SshPrivateKey = "sshprivatekeyvalue",
* Uid = 1234,
* },
* Name = "username1",
* Password = "",
* },
* },
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* ApplicationLicenses: pulumi.StringArray{
* pulumi.String("app-license0"),
* pulumi.String("app-license1"),
* },
* ApplicationPackages: batch.ApplicationPackageReferenceArray{
* &batch.ApplicationPackageReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/applications/app_1234"),
* Version: pulumi.String("asdf"),
* },
* },
* Certificates: batch.CertificateReferenceArray{
* &batch.CertificateReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/certificates/sha1-1234567"),
* StoreLocation: batch.CertificateStoreLocationLocalMachine,
* StoreName: pulumi.String("MY"),
* Visibility: batch.CertificateVisibilityArray{
* batch.CertificateVisibilityRemoteUser,
* },
* },
* },
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* CloudServiceConfiguration: &batch.CloudServiceConfigurationArgs{
* OsFamily: pulumi.String("4"),
* OsVersion: pulumi.String("WA-GUEST-OS-4.45_201708-01"),
* },
* },
* DisplayName: pulumi.String("my-pool-name"),
* InterNodeCommunication: batch.InterNodeCommunicationStateEnabled,
* Metadata: batch.MetadataItemArray{
* &batch.MetadataItemArgs{
* Name: pulumi.String("metadata-1"),
* Value: pulumi.String("value-1"),
* },
* &batch.MetadataItemArgs{
* Name: pulumi.String("metadata-2"),
* Value: pulumi.String("value-2"),
* },
* },
* NetworkConfiguration: &batch.NetworkConfigurationArgs{
* PublicIPAddressConfiguration: &batch.PublicIPAddressConfigurationArgs{
* IpAddressIds: pulumi.StringArray{
* pulumi.String("/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135"),
* pulumi.String("/subscriptions/subid2/resourceGroups/rg24/providers/Microsoft.Network/publicIPAddresses/ip268"),
* },
* Provision: batch.IPAddressProvisioningTypeUserManaged,
* },
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123"),
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* FixedScale: &batch.FixedScaleSettingsArgs{
* NodeDeallocationOption: batch.ComputeNodeDeallocationOptionTaskCompletion,
* ResizeTimeout: pulumi.String("PT8M"),
* TargetDedicatedNodes: pulumi.Int(6),
* TargetLowPriorityNodes: pulumi.Int(28),
* },
* },
* StartTask: &batch.StartTaskArgs{
* CommandLine: pulumi.String("cmd /c SET"),
* EnvironmentSettings: batch.EnvironmentSettingArray{
* &batch.EnvironmentSettingArgs{
* Name: pulumi.String("MYSET"),
* Value: pulumi.String("1234"),
* },
* },
* MaxTaskRetryCount: pulumi.Int(6),
* ResourceFiles: batch.ResourceFileArray{
* &batch.ResourceFileArgs{
* FileMode: pulumi.String("777"),
* FilePath: pulumi.String("c:\\temp\\gohere"),
* HttpUrl: pulumi.String("https://testaccount.blob.core.windows.net/example-blob-file"),
* },
* },
* UserIdentity: &batch.UserIdentityArgs{
* AutoUser: &batch.AutoUserSpecificationArgs{
* ElevationLevel: batch.ElevationLevelAdmin,
* Scope: batch.AutoUserScopePool,
* },
* },
* WaitForSuccess: pulumi.Bool(true),
* },
* TaskSchedulingPolicy: &batch.TaskSchedulingPolicyArgs{
* NodeFillType: batch.ComputeNodeFillTypePack,
* },
* TaskSlotsPerNode: pulumi.Int(13),
* UserAccounts: batch.UserAccountArray{
* &batch.UserAccountArgs{
* ElevationLevel: batch.ElevationLevelAdmin,
* LinuxUserConfiguration: &batch.LinuxUserConfigurationArgs{
* Gid: pulumi.Int(4567),
* SshPrivateKey: pulumi.String("sshprivatekeyvalue"),
* Uid: pulumi.Int(1234),
* },
* Name: pulumi.String("username1"),
* Password: pulumi.String(""),
* },
* },
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.ApplicationPackageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.CertificateReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.CloudServiceConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.MetadataItemArgs;
* import com.pulumi.azurenative.batch.inputs.NetworkConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.PublicIPAddressConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.FixedScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.StartTaskArgs;
* import com.pulumi.azurenative.batch.inputs.UserIdentityArgs;
* import com.pulumi.azurenative.batch.inputs.AutoUserSpecificationArgs;
* import com.pulumi.azurenative.batch.inputs.TaskSchedulingPolicyArgs;
* import com.pulumi.azurenative.batch.inputs.UserAccountArgs;
* import com.pulumi.azurenative.batch.inputs.LinuxUserConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .applicationLicenses(
* "app-license0",
* "app-license1")
* .applicationPackages(ApplicationPackageReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/applications/app_1234")
* .version("asdf")
* .build())
* .certificates(CertificateReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/default-azurebatch-japaneast/providers/Microsoft.Batch/batchAccounts/sampleacct/pools/testpool/certificates/sha1-1234567")
* .storeLocation("LocalMachine")
* .storeName("MY")
* .visibility("RemoteUser")
* .build())
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .cloudServiceConfiguration(CloudServiceConfigurationArgs.builder()
* .osFamily("4")
* .osVersion("WA-GUEST-OS-4.45_201708-01")
* .build())
* .build())
* .displayName("my-pool-name")
* .interNodeCommunication("Enabled")
* .metadata(
* MetadataItemArgs.builder()
* .name("metadata-1")
* .value("value-1")
* .build(),
* MetadataItemArgs.builder()
* .name("metadata-2")
* .value("value-2")
* .build())
* .networkConfiguration(NetworkConfigurationArgs.builder()
* .publicIPAddressConfiguration(PublicIPAddressConfigurationArgs.builder()
* .ipAddressIds(
* "/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135",
* "/subscriptions/subid2/resourceGroups/rg24/providers/Microsoft.Network/publicIPAddresses/ip268")
* .provision("UserManaged")
* .build())
* .subnetId("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123")
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .fixedScale(FixedScaleSettingsArgs.builder()
* .nodeDeallocationOption("TaskCompletion")
* .resizeTimeout("PT8M")
* .targetDedicatedNodes(6)
* .targetLowPriorityNodes(28)
* .build())
* .build())
* .startTask(StartTaskArgs.builder()
* .commandLine("cmd /c SET")
* .environmentSettings(EnvironmentSettingArgs.builder()
* .name("MYSET")
* .value("1234")
* .build())
* .maxTaskRetryCount(6)
* .resourceFiles(ResourceFileArgs.builder()
* .fileMode("777")
* .filePath("c:\\temp\\gohere")
* .httpUrl("https://testaccount.blob.core.windows.net/example-blob-file")
* .build())
* .userIdentity(UserIdentityArgs.builder()
* .autoUser(AutoUserSpecificationArgs.builder()
* .elevationLevel("Admin")
* .scope("Pool")
* .build())
* .build())
* .waitForSuccess(true)
* .build())
* .taskSchedulingPolicy(TaskSchedulingPolicyArgs.builder()
* .nodeFillType("Pack")
* .build())
* .taskSlotsPerNode(13)
* .userAccounts(UserAccountArgs.builder()
* .elevationLevel("Admin")
* .linuxUserConfiguration(LinuxUserConfigurationArgs.builder()
* .gid(4567)
* .sshPrivateKey("sshprivatekeyvalue")
* .uid(1234)
* .build())
* .name("username1")
* .password("")
* .build())
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - Full VirtualMachineConfiguration
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* DataDisks = new[]
* {
* new AzureNative.Batch.Inputs.DataDiskArgs
* {
* Caching = AzureNative.Batch.CachingType.ReadWrite,
* DiskSizeGB = 30,
* Lun = 0,
* StorageAccountType = AzureNative.Batch.StorageAccountType.Premium_LRS,
* },
* new AzureNative.Batch.Inputs.DataDiskArgs
* {
* Caching = AzureNative.Batch.CachingType.None,
* DiskSizeGB = 200,
* Lun = 1,
* StorageAccountType = AzureNative.Batch.StorageAccountType.Standard_LRS,
* },
* },
* DiskEncryptionConfiguration = new AzureNative.Batch.Inputs.DiskEncryptionConfigurationArgs
* {
* Targets = new[]
* {
* AzureNative.Batch.DiskEncryptionTarget.OsDisk,
* AzureNative.Batch.DiskEncryptionTarget.TemporaryDisk,
* },
* },
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Offer = "WindowsServer",
* Publisher = "MicrosoftWindowsServer",
* Sku = "2016-Datacenter-SmallDisk",
* Version = "latest",
* },
* LicenseType = "Windows_Server",
* NodeAgentSkuId = "batch.node.windows amd64",
* NodePlacementConfiguration = new AzureNative.Batch.Inputs.NodePlacementConfigurationArgs
* {
* Policy = AzureNative.Batch.NodePlacementPolicyType.Zonal,
* },
* OsDisk = new AzureNative.Batch.Inputs.OSDiskArgs
* {
* EphemeralOSDiskSettings = new AzureNative.Batch.Inputs.DiffDiskSettingsArgs
* {
* Placement = AzureNative.Batch.DiffDiskPlacement.CacheDisk,
* },
* },
* WindowsConfiguration = new AzureNative.Batch.Inputs.WindowsConfigurationArgs
* {
* EnableAutomaticUpdates = false,
* },
* },
* },
* NetworkConfiguration = new AzureNative.Batch.Inputs.NetworkConfigurationArgs
* {
* EndpointConfiguration = new AzureNative.Batch.Inputs.PoolEndpointConfigurationArgs
* {
* InboundNatPools = new[]
* {
* new AzureNative.Batch.Inputs.InboundNatPoolArgs
* {
* BackendPort = 12001,
* FrontendPortRangeEnd = 15100,
* FrontendPortRangeStart = 15000,
* Name = "testnat",
* NetworkSecurityGroupRules = new[]
* {
* new AzureNative.Batch.Inputs.NetworkSecurityGroupRuleArgs
* {
* Access = AzureNative.Batch.NetworkSecurityGroupRuleAccess.Allow,
* Priority = 150,
* SourceAddressPrefix = "192.100.12.45",
* SourcePortRanges = new[]
* {
* "1",
* "2",
* },
* },
* new AzureNative.Batch.Inputs.NetworkSecurityGroupRuleArgs
* {
* Access = AzureNative.Batch.NetworkSecurityGroupRuleAccess.Deny,
* Priority = 3500,
* SourceAddressPrefix = "*",
* SourcePortRanges = new[]
* {
* "*",
* },
* },
* },
* Protocol = AzureNative.Batch.InboundEndpointProtocol.TCP,
* },
* },
* },
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* AutoScale = new AzureNative.Batch.Inputs.AutoScaleSettingsArgs
* {
* EvaluationInterval = "PT5M",
* Formula = "$TargetDedicatedNodes=1",
* },
* },
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* DataDisks: batch.DataDiskArray{
* &batch.DataDiskArgs{
* Caching: batch.CachingTypeReadWrite,
* DiskSizeGB: pulumi.Int(30),
* Lun: pulumi.Int(0),
* StorageAccountType: batch.StorageAccountType_Premium_LRS,
* },
* &batch.DataDiskArgs{
* Caching: batch.CachingTypeNone,
* DiskSizeGB: pulumi.Int(200),
* Lun: pulumi.Int(1),
* StorageAccountType: batch.StorageAccountType_Standard_LRS,
* },
* },
* DiskEncryptionConfiguration: &batch.DiskEncryptionConfigurationArgs{
* Targets: batch.DiskEncryptionTargetArray{
* batch.DiskEncryptionTargetOsDisk,
* batch.DiskEncryptionTargetTemporaryDisk,
* },
* },
* ImageReference: &batch.ImageReferenceArgs{
* Offer: pulumi.String("WindowsServer"),
* Publisher: pulumi.String("MicrosoftWindowsServer"),
* Sku: pulumi.String("2016-Datacenter-SmallDisk"),
* Version: pulumi.String("latest"),
* },
* LicenseType: pulumi.String("Windows_Server"),
* NodeAgentSkuId: pulumi.String("batch.node.windows amd64"),
* NodePlacementConfiguration: &batch.NodePlacementConfigurationArgs{
* Policy: batch.NodePlacementPolicyTypeZonal,
* },
* OsDisk: &batch.OSDiskArgs{
* EphemeralOSDiskSettings: &batch.DiffDiskSettingsArgs{
* Placement: batch.DiffDiskPlacementCacheDisk,
* },
* },
* WindowsConfiguration: &batch.WindowsConfigurationArgs{
* EnableAutomaticUpdates: pulumi.Bool(false),
* },
* },
* },
* NetworkConfiguration: &batch.NetworkConfigurationArgs{
* EndpointConfiguration: &batch.PoolEndpointConfigurationArgs{
* InboundNatPools: batch.InboundNatPoolArray{
* &batch.InboundNatPoolArgs{
* BackendPort: pulumi.Int(12001),
* FrontendPortRangeEnd: pulumi.Int(15100),
* FrontendPortRangeStart: pulumi.Int(15000),
* Name: pulumi.String("testnat"),
* NetworkSecurityGroupRules: batch.NetworkSecurityGroupRuleArray{
* &batch.NetworkSecurityGroupRuleArgs{
* Access: batch.NetworkSecurityGroupRuleAccessAllow,
* Priority: pulumi.Int(150),
* SourceAddressPrefix: pulumi.String("192.100.12.45"),
* SourcePortRanges: pulumi.StringArray{
* pulumi.String("1"),
* pulumi.String("2"),
* },
* },
* &batch.NetworkSecurityGroupRuleArgs{
* Access: batch.NetworkSecurityGroupRuleAccessDeny,
* Priority: pulumi.Int(3500),
* SourceAddressPrefix: pulumi.String("*"),
* SourcePortRanges: pulumi.StringArray{
* pulumi.String("*"),
* },
* },
* },
* Protocol: batch.InboundEndpointProtocolTCP,
* },
* },
* },
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* AutoScale: &batch.AutoScaleSettingsArgs{
* EvaluationInterval: pulumi.String("PT5M"),
* Formula: pulumi.String("$TargetDedicatedNodes=1"),
* },
* },
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.DiskEncryptionConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.NodePlacementConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.OSDiskArgs;
* import com.pulumi.azurenative.batch.inputs.DiffDiskSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.WindowsConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.NetworkConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.PoolEndpointConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.AutoScaleSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .dataDisks(
* DataDiskArgs.builder()
* .caching("ReadWrite")
* .diskSizeGB(30)
* .lun(0)
* .storageAccountType("Premium_LRS")
* .build(),
* DataDiskArgs.builder()
* .caching("None")
* .diskSizeGB(200)
* .lun(1)
* .storageAccountType("Standard_LRS")
* .build())
* .diskEncryptionConfiguration(DiskEncryptionConfigurationArgs.builder()
* .targets(
* "OsDisk",
* "TemporaryDisk")
* .build())
* .imageReference(ImageReferenceArgs.builder()
* .offer("WindowsServer")
* .publisher("MicrosoftWindowsServer")
* .sku("2016-Datacenter-SmallDisk")
* .version("latest")
* .build())
* .licenseType("Windows_Server")
* .nodeAgentSkuId("batch.node.windows amd64")
* .nodePlacementConfiguration(NodePlacementConfigurationArgs.builder()
* .policy("Zonal")
* .build())
* .osDisk(OSDiskArgs.builder()
* .ephemeralOSDiskSettings(DiffDiskSettingsArgs.builder()
* .placement("CacheDisk")
* .build())
* .build())
* .windowsConfiguration(WindowsConfigurationArgs.builder()
* .enableAutomaticUpdates(false)
* .build())
* .build())
* .build())
* .networkConfiguration(NetworkConfigurationArgs.builder()
* .endpointConfiguration(PoolEndpointConfigurationArgs.builder()
* .inboundNatPools(InboundNatPoolArgs.builder()
* .backendPort(12001)
* .frontendPortRangeEnd(15100)
* .frontendPortRangeStart(15000)
* .name("testnat")
* .networkSecurityGroupRules(
* NetworkSecurityGroupRuleArgs.builder()
* .access("Allow")
* .priority(150)
* .sourceAddressPrefix("192.100.12.45")
* .sourcePortRanges(
* "1",
* "2")
* .build(),
* NetworkSecurityGroupRuleArgs.builder()
* .access("Deny")
* .priority(3500)
* .sourceAddressPrefix("*")
* .sourcePortRanges("*")
* .build())
* .protocol("TCP")
* .build())
* .build())
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .autoScale(AutoScaleSettingsArgs.builder()
* .evaluationInterval("PT5M")
* .formula("$TargetDedicatedNodes=1")
* .build())
* .build())
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - Minimal CloudServiceConfiguration
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* CloudServiceConfiguration = new AzureNative.Batch.Inputs.CloudServiceConfigurationArgs
* {
* OsFamily = "5",
* },
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* FixedScale = new AzureNative.Batch.Inputs.FixedScaleSettingsArgs
* {
* TargetDedicatedNodes = 3,
* },
* },
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* CloudServiceConfiguration: &batch.CloudServiceConfigurationArgs{
* OsFamily: pulumi.String("5"),
* },
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* FixedScale: &batch.FixedScaleSettingsArgs{
* TargetDedicatedNodes: pulumi.Int(3),
* },
* },
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.CloudServiceConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.FixedScaleSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .cloudServiceConfiguration(CloudServiceConfigurationArgs.builder()
* .osFamily("5")
* .build())
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .fixedScale(FixedScaleSettingsArgs.builder()
* .targetDedicatedNodes(3)
* .build())
* .build())
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - Minimal VirtualMachineConfiguration
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Offer = "UbuntuServer",
* Publisher = "Canonical",
* Sku = "18.04-LTS",
* Version = "latest",
* },
* NodeAgentSkuId = "batch.node.ubuntu 18.04",
* },
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* AutoScale = new AzureNative.Batch.Inputs.AutoScaleSettingsArgs
* {
* EvaluationInterval = "PT5M",
* Formula = "$TargetDedicatedNodes=1",
* },
* },
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* ImageReference: &batch.ImageReferenceArgs{
* Offer: pulumi.String("UbuntuServer"),
* Publisher: pulumi.String("Canonical"),
* Sku: pulumi.String("18.04-LTS"),
* Version: pulumi.String("latest"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.ubuntu 18.04"),
* },
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* AutoScale: &batch.AutoScaleSettingsArgs{
* EvaluationInterval: pulumi.String("PT5M"),
* Formula: pulumi.String("$TargetDedicatedNodes=1"),
* },
* },
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.AutoScaleSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .imageReference(ImageReferenceArgs.builder()
* .offer("UbuntuServer")
* .publisher("Canonical")
* .sku("18.04-LTS")
* .version("latest")
* .build())
* .nodeAgentSkuId("batch.node.ubuntu 18.04")
* .build())
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .autoScale(AutoScaleSettingsArgs.builder()
* .evaluationInterval("PT5M")
* .formula("$TargetDedicatedNodes=1")
* .build())
* .build())
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - No public IP
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1",
* },
* NodeAgentSkuId = "batch.node.ubuntu 18.04",
* },
* },
* NetworkConfiguration = new AzureNative.Batch.Inputs.NetworkConfigurationArgs
* {
* PublicIPAddressConfiguration = new AzureNative.Batch.Inputs.PublicIPAddressConfigurationArgs
* {
* Provision = AzureNative.Batch.IPAddressProvisioningType.NoPublicIPAddresses,
* },
* SubnetId = "/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123",
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* ImageReference: &batch.ImageReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.ubuntu 18.04"),
* },
* },
* NetworkConfiguration: &batch.NetworkConfigurationArgs{
* PublicIPAddressConfiguration: &batch.PublicIPAddressConfigurationArgs{
* Provision: batch.IPAddressProvisioningTypeNoPublicIPAddresses,
* },
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123"),
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.NetworkConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.PublicIPAddressConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .imageReference(ImageReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1")
* .build())
* .nodeAgentSkuId("batch.node.ubuntu 18.04")
* .build())
* .build())
* .networkConfiguration(NetworkConfigurationArgs.builder()
* .publicIPAddressConfiguration(PublicIPAddressConfigurationArgs.builder()
* .provision("NoPublicIPAddresses")
* .build())
* .subnetId("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123")
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - Public IPs
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1",
* },
* NodeAgentSkuId = "batch.node.ubuntu 18.04",
* },
* },
* NetworkConfiguration = new AzureNative.Batch.Inputs.NetworkConfigurationArgs
* {
* PublicIPAddressConfiguration = new AzureNative.Batch.Inputs.PublicIPAddressConfigurationArgs
* {
* IpAddressIds = new[]
* {
* "/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135",
* },
* Provision = AzureNative.Batch.IPAddressProvisioningType.UserManaged,
* },
* SubnetId = "/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123",
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* ImageReference: &batch.ImageReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.ubuntu 18.04"),
* },
* },
* NetworkConfiguration: &batch.NetworkConfigurationArgs{
* PublicIPAddressConfiguration: &batch.PublicIPAddressConfigurationArgs{
* IpAddressIds: pulumi.StringArray{
* pulumi.String("/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135"),
* },
* Provision: batch.IPAddressProvisioningTypeUserManaged,
* },
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123"),
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.NetworkConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.PublicIPAddressConfigurationArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .imageReference(ImageReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/networking-group/providers/Microsoft.Compute/galleries/testgallery/images/testimagedef/versions/0.0.1")
* .build())
* .nodeAgentSkuId("batch.node.ubuntu 18.04")
* .build())
* .build())
* .networkConfiguration(NetworkConfigurationArgs.builder()
* .publicIPAddressConfiguration(PublicIPAddressConfigurationArgs.builder()
* .ipAddressIds("/subscriptions/subid1/resourceGroups/rg13/providers/Microsoft.Network/publicIPAddresses/ip135")
* .provision("UserManaged")
* .build())
* .subnetId("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123")
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - VirtualMachineConfiguration Extensions
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* Extensions = new[]
* {
* new AzureNative.Batch.Inputs.VMExtensionArgs
* {
* AutoUpgradeMinorVersion = true,
* EnableAutomaticUpgrade = true,
* Name = "batchextension1",
* Publisher = "Microsoft.Azure.KeyVault",
* Settings = new Dictionary
* {
* ["authenticationSettingsKey"] = "authenticationSettingsValue",
* ["secretsManagementSettingsKey"] = "secretsManagementSettingsValue",
* },
* Type = "KeyVaultForLinux",
* TypeHandlerVersion = "2.0",
* },
* },
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Offer = "0001-com-ubuntu-server-focal",
* Publisher = "Canonical",
* Sku = "20_04-lts",
* },
* NodeAgentSkuId = "batch.node.ubuntu 20.04",
* },
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* AutoScale = new AzureNative.Batch.Inputs.AutoScaleSettingsArgs
* {
* EvaluationInterval = "PT5M",
* Formula = "$TargetDedicatedNodes=1",
* },
* },
* TargetNodeCommunicationMode = AzureNative.Batch.NodeCommunicationMode.Default,
* VmSize = "STANDARD_D4",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* Extensions: batch.VMExtensionArray{
* &batch.VMExtensionArgs{
* AutoUpgradeMinorVersion: pulumi.Bool(true),
* EnableAutomaticUpgrade: pulumi.Bool(true),
* Name: pulumi.String("batchextension1"),
* Publisher: pulumi.String("Microsoft.Azure.KeyVault"),
* Settings: pulumi.Any(map[string]interface{}{
* "authenticationSettingsKey": "authenticationSettingsValue",
* "secretsManagementSettingsKey": "secretsManagementSettingsValue",
* }),
* Type: pulumi.String("KeyVaultForLinux"),
* TypeHandlerVersion: pulumi.String("2.0"),
* },
* },
* ImageReference: &batch.ImageReferenceArgs{
* Offer: pulumi.String("0001-com-ubuntu-server-focal"),
* Publisher: pulumi.String("Canonical"),
* Sku: pulumi.String("20_04-lts"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.ubuntu 20.04"),
* },
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* AutoScale: &batch.AutoScaleSettingsArgs{
* EvaluationInterval: pulumi.String("PT5M"),
* Formula: pulumi.String("$TargetDedicatedNodes=1"),
* },
* },
* TargetNodeCommunicationMode: batch.NodeCommunicationModeDefault,
* VmSize: pulumi.String("STANDARD_D4"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.AutoScaleSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .extensions(VMExtensionArgs.builder()
* .autoUpgradeMinorVersion(true)
* .enableAutomaticUpgrade(true)
* .name("batchextension1")
* .publisher("Microsoft.Azure.KeyVault")
* .settings(Map.ofEntries(
* Map.entry("authenticationSettingsKey", "authenticationSettingsValue"),
* Map.entry("secretsManagementSettingsKey", "secretsManagementSettingsValue")
* ))
* .type("KeyVaultForLinux")
* .typeHandlerVersion("2.0")
* .build())
* .imageReference(ImageReferenceArgs.builder()
* .offer("0001-com-ubuntu-server-focal")
* .publisher("Canonical")
* .sku("20_04-lts")
* .build())
* .nodeAgentSkuId("batch.node.ubuntu 20.04")
* .build())
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .autoScale(AutoScaleSettingsArgs.builder()
* .evaluationInterval("PT5M")
* .formula("$TargetDedicatedNodes=1")
* .build())
* .build())
* .targetNodeCommunicationMode("Default")
* .vmSize("STANDARD_D4")
* .build());
* }
* }
* ```
* ### CreatePool - accelerated networking
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var pool = new AzureNative.Batch.Pool("pool", new()
* {
* AccountName = "sampleacct",
* DeploymentConfiguration = new AzureNative.Batch.Inputs.DeploymentConfigurationArgs
* {
* VirtualMachineConfiguration = new AzureNative.Batch.Inputs.VirtualMachineConfigurationArgs
* {
* ImageReference = new AzureNative.Batch.Inputs.ImageReferenceArgs
* {
* Offer = "WindowsServer",
* Publisher = "MicrosoftWindowsServer",
* Sku = "2016-datacenter-smalldisk",
* Version = "latest",
* },
* NodeAgentSkuId = "batch.node.windows amd64",
* },
* },
* NetworkConfiguration = new AzureNative.Batch.Inputs.NetworkConfigurationArgs
* {
* EnableAcceleratedNetworking = true,
* SubnetId = "/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123",
* },
* PoolName = "testpool",
* ResourceGroupName = "default-azurebatch-japaneast",
* ScaleSettings = new AzureNative.Batch.Inputs.ScaleSettingsArgs
* {
* FixedScale = new AzureNative.Batch.Inputs.FixedScaleSettingsArgs
* {
* TargetDedicatedNodes = 1,
* TargetLowPriorityNodes = 0,
* },
* },
* VmSize = "STANDARD_D1_V2",
* });
* });
* ```
* ```go
* package main
* import (
* batch "github.com/pulumi/pulumi-azure-native-sdk/batch/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := batch.NewPool(ctx, "pool", &batch.PoolArgs{
* AccountName: pulumi.String("sampleacct"),
* DeploymentConfiguration: &batch.DeploymentConfigurationArgs{
* VirtualMachineConfiguration: &batch.VirtualMachineConfigurationArgs{
* ImageReference: &batch.ImageReferenceArgs{
* Offer: pulumi.String("WindowsServer"),
* Publisher: pulumi.String("MicrosoftWindowsServer"),
* Sku: pulumi.String("2016-datacenter-smalldisk"),
* Version: pulumi.String("latest"),
* },
* NodeAgentSkuId: pulumi.String("batch.node.windows amd64"),
* },
* },
* NetworkConfiguration: &batch.NetworkConfigurationArgs{
* EnableAcceleratedNetworking: pulumi.Bool(true),
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123"),
* },
* PoolName: pulumi.String("testpool"),
* ResourceGroupName: pulumi.String("default-azurebatch-japaneast"),
* ScaleSettings: &batch.ScaleSettingsArgs{
* FixedScale: &batch.FixedScaleSettingsArgs{
* TargetDedicatedNodes: pulumi.Int(1),
* TargetLowPriorityNodes: pulumi.Int(0),
* },
* },
* VmSize: pulumi.String("STANDARD_D1_V2"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.batch.Pool;
* import com.pulumi.azurenative.batch.PoolArgs;
* import com.pulumi.azurenative.batch.inputs.DeploymentConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.VirtualMachineConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ImageReferenceArgs;
* import com.pulumi.azurenative.batch.inputs.NetworkConfigurationArgs;
* import com.pulumi.azurenative.batch.inputs.ScaleSettingsArgs;
* import com.pulumi.azurenative.batch.inputs.FixedScaleSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var pool = new Pool("pool", PoolArgs.builder()
* .accountName("sampleacct")
* .deploymentConfiguration(DeploymentConfigurationArgs.builder()
* .virtualMachineConfiguration(VirtualMachineConfigurationArgs.builder()
* .imageReference(ImageReferenceArgs.builder()
* .offer("WindowsServer")
* .publisher("MicrosoftWindowsServer")
* .sku("2016-datacenter-smalldisk")
* .version("latest")
* .build())
* .nodeAgentSkuId("batch.node.windows amd64")
* .build())
* .build())
* .networkConfiguration(NetworkConfigurationArgs.builder()
* .enableAcceleratedNetworking(true)
* .subnetId("/subscriptions/subid/resourceGroups/rg1234/providers/Microsoft.Network/virtualNetworks/network1234/subnets/subnet123")
* .build())
* .poolName("testpool")
* .resourceGroupName("default-azurebatch-japaneast")
* .scaleSettings(ScaleSettingsArgs.builder()
* .fixedScale(FixedScaleSettingsArgs.builder()
* .targetDedicatedNodes(1)
* .targetLowPriorityNodes(0)
* .build())
* .build())
* .vmSize("STANDARD_D1_V2")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:batch:Pool testpool /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Batch/batchAccounts/{accountName}/pools/{poolName}
* ```
* @property accountName The name of the Batch account.
* @property applicationLicenses The list of application licenses must be a subset of available Batch service application licenses. If a license is requested which is not supported, pool creation will fail.
* @property applicationPackages Changes to application package references affect all new compute nodes joining the pool, but do not affect compute nodes that are already in the pool until they are rebooted or reimaged. There is a maximum of 10 application package references on any given pool.
* @property certificates For Windows compute nodes, the Batch service installs the certificates to the specified certificate store and location. For Linux compute nodes, the certificates are stored in a directory inside the task working directory and an environment variable AZ_BATCH_CERTIFICATES_DIR is supplied to the task to query for this location. For certificates with visibility of 'remoteUser', a 'certs' directory is created in the user's home directory (e.g., /home/{user-name}/certs) and certificates are placed in that directory.
* Warning: This property is deprecated and will be removed after February, 2024. Please use the [Azure KeyVault Extension](https://learn.microsoft.com/azure/batch/batch-certificate-migration-guide) instead.
* @property deploymentConfiguration Using CloudServiceConfiguration specifies that the nodes should be creating using Azure Cloud Services (PaaS), while VirtualMachineConfiguration uses Azure Virtual Machines (IaaS).
* @property displayName The display name need not be unique and can contain any Unicode characters up to a maximum length of 1024.
* @property identity The type of identity used for the Batch Pool.
* @property interNodeCommunication This imposes restrictions on which nodes can be assigned to the pool. Enabling this value can reduce the chance of the requested number of nodes to be allocated in the pool. If not specified, this value defaults to 'Disabled'.
* @property metadata The Batch service does not assign any meaning to metadata; it is solely for the use of user code.
* @property mountConfiguration This supports Azure Files, NFS, CIFS/SMB, and Blobfuse.
* @property networkConfiguration The network configuration for a pool.
* @property poolName The pool name. This must be unique within the account.
* @property resourceGroupName The name of the resource group that contains the Batch account.
* @property scaleSettings Defines the desired size of the pool. This can either be 'fixedScale' where the requested targetDedicatedNodes is specified, or 'autoScale' which defines a formula which is periodically reevaluated. If this property is not specified, the pool will have a fixed scale with 0 targetDedicatedNodes.
* @property startTask In an PATCH (update) operation, this property can be set to an empty object to remove the start task from the pool.
* @property targetNodeCommunicationMode If omitted, the default value is Default.
* @property taskSchedulingPolicy If not specified, the default is spread.
* @property taskSlotsPerNode The default value is 1. The maximum value is the smaller of 4 times the number of cores of the vmSize of the pool or 256.
* @property userAccounts
* @property vmSize For information about available sizes of virtual machines for Cloud Services pools (pools created with cloudServiceConfiguration), see Sizes for Cloud Services (https://azure.microsoft.com/documentation/articles/cloud-services-sizes-specs/). Batch supports all Cloud Services VM sizes except ExtraSmall. For information about available VM sizes for pools using images from the Virtual Machines Marketplace (pools created with virtualMachineConfiguration) see Sizes for Virtual Machines (Linux) (https://azure.microsoft.com/documentation/articles/virtual-machines-linux-sizes/) or Sizes for Virtual Machines (Windows) (https://azure.microsoft.com/documentation/articles/virtual-machines-windows-sizes/). Batch supports all Azure VM sizes except STANDARD_A0 and those with premium storage (STANDARD_GS, STANDARD_DS, and STANDARD_DSV2 series).
*/
public data class PoolArgs(
public val accountName: Output? = null,
public val applicationLicenses: Output>? = null,
public val applicationPackages: Output>? = null,
public val certificates: Output>? = null,
public val deploymentConfiguration: Output? = null,
public val displayName: Output? = null,
public val identity: Output? = null,
public val interNodeCommunication: Output? = null,
public val metadata: Output>? = null,
public val mountConfiguration: Output>? = null,
public val networkConfiguration: Output? = null,
public val poolName: Output? = null,
public val resourceGroupName: Output? = null,
public val scaleSettings: Output? = null,
public val startTask: Output? = null,
public val targetNodeCommunicationMode: Output? = null,
public val taskSchedulingPolicy: Output? = null,
public val taskSlotsPerNode: Output? = null,
public val userAccounts: Output>? = null,
public val vmSize: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.batch.PoolArgs =
com.pulumi.azurenative.batch.PoolArgs.builder()
.accountName(accountName?.applyValue({ args0 -> args0 }))
.applicationLicenses(applicationLicenses?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.applicationPackages(
applicationPackages?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.certificates(
certificates?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.deploymentConfiguration(
deploymentConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.displayName(displayName?.applyValue({ args0 -> args0 }))
.identity(identity?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.interNodeCommunication(
interNodeCommunication?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.metadata(
metadata?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.mountConfiguration(
mountConfiguration?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.networkConfiguration(
networkConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.poolName(poolName?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.scaleSettings(scaleSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.startTask(startTask?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.targetNodeCommunicationMode(
targetNodeCommunicationMode?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.taskSchedulingPolicy(
taskSchedulingPolicy?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.taskSlotsPerNode(taskSlotsPerNode?.applyValue({ args0 -> args0 }))
.userAccounts(
userAccounts?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.vmSize(vmSize?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PoolArgs].
*/
@PulumiTagMarker
public class PoolArgsBuilder internal constructor() {
private var accountName: Output? = null
private var applicationLicenses: Output>? = null
private var applicationPackages: Output>? = null
private var certificates: Output>? = null
private var deploymentConfiguration: Output? = null
private var displayName: Output? = null
private var identity: Output? = null
private var interNodeCommunication: Output? = null
private var metadata: Output>? = null
private var mountConfiguration: Output>? = null
private var networkConfiguration: Output? = null
private var poolName: Output? = null
private var resourceGroupName: Output? = null
private var scaleSettings: Output? = null
private var startTask: Output? = null
private var targetNodeCommunicationMode: Output? = null
private var taskSchedulingPolicy: Output? = null
private var taskSlotsPerNode: Output? = null
private var userAccounts: Output>? = null
private var vmSize: Output? = null
/**
* @param value The name of the Batch account.
*/
@JvmName("mggfpwuvvnbqlwtl")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value The list of application licenses must be a subset of available Batch service application licenses. If a license is requested which is not supported, pool creation will fail.
*/
@JvmName("xpmcnkcplvcrxpvs")
public suspend fun applicationLicenses(`value`: Output>) {
this.applicationLicenses = value
}
@JvmName("gunsnpmvwpkjjmdj")
public suspend fun applicationLicenses(vararg values: Output) {
this.applicationLicenses = Output.all(values.asList())
}
/**
* @param values The list of application licenses must be a subset of available Batch service application licenses. If a license is requested which is not supported, pool creation will fail.
*/
@JvmName("vdkvvgidfmdvqmyd")
public suspend fun applicationLicenses(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy