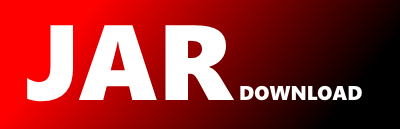
com.pulumi.azurenative.batch.kotlin.inputs.AzureBlobFileSystemConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.batch.kotlin.inputs
import com.pulumi.azurenative.batch.inputs.AzureBlobFileSystemConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property accountKey This property is mutually exclusive with both sasKey and identity; exactly one must be specified.
* @property accountName
* @property blobfuseOptions These are 'net use' options in Windows and 'mount' options in Linux.
* @property containerName
* @property identityReference This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
* @property relativeMountPath All file systems are mounted relative to the Batch mounts directory, accessible via the AZ_BATCH_NODE_MOUNTS_DIR environment variable.
* @property sasKey This property is mutually exclusive with both accountKey and identity; exactly one must be specified.
*/
public data class AzureBlobFileSystemConfigurationArgs(
public val accountKey: Output? = null,
public val accountName: Output,
public val blobfuseOptions: Output? = null,
public val containerName: Output,
public val identityReference: Output? = null,
public val relativeMountPath: Output,
public val sasKey: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.batch.inputs.AzureBlobFileSystemConfigurationArgs =
com.pulumi.azurenative.batch.inputs.AzureBlobFileSystemConfigurationArgs.builder()
.accountKey(accountKey?.applyValue({ args0 -> args0 }))
.accountName(accountName.applyValue({ args0 -> args0 }))
.blobfuseOptions(blobfuseOptions?.applyValue({ args0 -> args0 }))
.containerName(containerName.applyValue({ args0 -> args0 }))
.identityReference(identityReference?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.relativeMountPath(relativeMountPath.applyValue({ args0 -> args0 }))
.sasKey(sasKey?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AzureBlobFileSystemConfigurationArgs].
*/
@PulumiTagMarker
public class AzureBlobFileSystemConfigurationArgsBuilder internal constructor() {
private var accountKey: Output? = null
private var accountName: Output? = null
private var blobfuseOptions: Output? = null
private var containerName: Output? = null
private var identityReference: Output? = null
private var relativeMountPath: Output? = null
private var sasKey: Output? = null
/**
* @param value This property is mutually exclusive with both sasKey and identity; exactly one must be specified.
*/
@JvmName("hurvephhdkkyyxpg")
public suspend fun accountKey(`value`: Output) {
this.accountKey = value
}
/**
* @param value
*/
@JvmName("yhgjdbsqgcujlwlx")
public suspend fun accountName(`value`: Output) {
this.accountName = value
}
/**
* @param value These are 'net use' options in Windows and 'mount' options in Linux.
*/
@JvmName("chjybsyrvchceaoi")
public suspend fun blobfuseOptions(`value`: Output) {
this.blobfuseOptions = value
}
/**
* @param value
*/
@JvmName("rokruhhvhmeyaslb")
public suspend fun containerName(`value`: Output) {
this.containerName = value
}
/**
* @param value This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
*/
@JvmName("vbegthhgfgbfrqrf")
public suspend fun identityReference(`value`: Output) {
this.identityReference = value
}
/**
* @param value All file systems are mounted relative to the Batch mounts directory, accessible via the AZ_BATCH_NODE_MOUNTS_DIR environment variable.
*/
@JvmName("fmkdgonxndxhfbay")
public suspend fun relativeMountPath(`value`: Output) {
this.relativeMountPath = value
}
/**
* @param value This property is mutually exclusive with both accountKey and identity; exactly one must be specified.
*/
@JvmName("ruvcteojioclupuy")
public suspend fun sasKey(`value`: Output) {
this.sasKey = value
}
/**
* @param value This property is mutually exclusive with both sasKey and identity; exactly one must be specified.
*/
@JvmName("rxnqvewtmsyeaidt")
public suspend fun accountKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accountKey = mapped
}
/**
* @param value
*/
@JvmName("uyyjxeiyanahmyue")
public suspend fun accountName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.accountName = mapped
}
/**
* @param value These are 'net use' options in Windows and 'mount' options in Linux.
*/
@JvmName("tywwcvwdfwfkiqnr")
public suspend fun blobfuseOptions(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blobfuseOptions = mapped
}
/**
* @param value
*/
@JvmName("hkcjvqbrbffchjbj")
public suspend fun containerName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.containerName = mapped
}
/**
* @param value This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
*/
@JvmName("thkgrfbqspqaxppd")
public suspend fun identityReference(`value`: ComputeNodeIdentityReferenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identityReference = mapped
}
/**
* @param argument This property is mutually exclusive with both accountKey and sasKey; exactly one must be specified.
*/
@JvmName("ecdirxawtkieishr")
public suspend fun identityReference(argument: suspend ComputeNodeIdentityReferenceArgsBuilder.() -> Unit) {
val toBeMapped = ComputeNodeIdentityReferenceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.identityReference = mapped
}
/**
* @param value All file systems are mounted relative to the Batch mounts directory, accessible via the AZ_BATCH_NODE_MOUNTS_DIR environment variable.
*/
@JvmName("xbfmxrpstltqtvcf")
public suspend fun relativeMountPath(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.relativeMountPath = mapped
}
/**
* @param value This property is mutually exclusive with both accountKey and identity; exactly one must be specified.
*/
@JvmName("yptxjjhiudujmqbh")
public suspend fun sasKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sasKey = mapped
}
internal fun build(): AzureBlobFileSystemConfigurationArgs = AzureBlobFileSystemConfigurationArgs(
accountKey = accountKey,
accountName = accountName ?: throw PulumiNullFieldException("accountName"),
blobfuseOptions = blobfuseOptions,
containerName = containerName ?: throw PulumiNullFieldException("containerName"),
identityReference = identityReference,
relativeMountPath = relativeMountPath ?: throw PulumiNullFieldException("relativeMountPath"),
sasKey = sasKey,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy