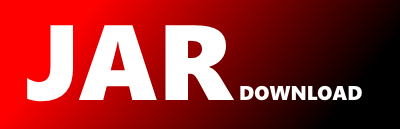
com.pulumi.azurenative.batch.kotlin.inputs.UserAccountArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.batch.kotlin.inputs
import com.pulumi.azurenative.batch.inputs.UserAccountArgs.builder
import com.pulumi.azurenative.batch.kotlin.enums.ElevationLevel
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property elevationLevel nonAdmin - The auto user is a standard user without elevated access. admin - The auto user is a user with elevated access and operates with full Administrator permissions. The default value is nonAdmin.
* @property linuxUserConfiguration This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
* @property name
* @property password
* @property windowsUserConfiguration This property can only be specified if the user is on a Windows pool. If not specified and on a Windows pool, the user is created with the default options.
*/
public data class UserAccountArgs(
public val elevationLevel: Output? = null,
public val linuxUserConfiguration: Output? = null,
public val name: Output,
public val password: Output,
public val windowsUserConfiguration: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.batch.inputs.UserAccountArgs =
com.pulumi.azurenative.batch.inputs.UserAccountArgs.builder()
.elevationLevel(elevationLevel?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.linuxUserConfiguration(
linuxUserConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name.applyValue({ args0 -> args0 }))
.password(password.applyValue({ args0 -> args0 }))
.windowsUserConfiguration(
windowsUserConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [UserAccountArgs].
*/
@PulumiTagMarker
public class UserAccountArgsBuilder internal constructor() {
private var elevationLevel: Output? = null
private var linuxUserConfiguration: Output? = null
private var name: Output? = null
private var password: Output? = null
private var windowsUserConfiguration: Output? = null
/**
* @param value nonAdmin - The auto user is a standard user without elevated access. admin - The auto user is a user with elevated access and operates with full Administrator permissions. The default value is nonAdmin.
*/
@JvmName("dtnmtbwhphqjnhye")
public suspend fun elevationLevel(`value`: Output) {
this.elevationLevel = value
}
/**
* @param value This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
*/
@JvmName("yyqeyjqbguppfrur")
public suspend fun linuxUserConfiguration(`value`: Output) {
this.linuxUserConfiguration = value
}
/**
* @param value
*/
@JvmName("agidswrhjjtqfcoo")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("cpexctshsikvlave")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value This property can only be specified if the user is on a Windows pool. If not specified and on a Windows pool, the user is created with the default options.
*/
@JvmName("wdbprmpldfthobtk")
public suspend fun windowsUserConfiguration(`value`: Output) {
this.windowsUserConfiguration = value
}
/**
* @param value nonAdmin - The auto user is a standard user without elevated access. admin - The auto user is a user with elevated access and operates with full Administrator permissions. The default value is nonAdmin.
*/
@JvmName("rycvufkpdlouicco")
public suspend fun elevationLevel(`value`: ElevationLevel?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.elevationLevel = mapped
}
/**
* @param value This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
*/
@JvmName("lrftxvpwbaamwwyq")
public suspend fun linuxUserConfiguration(`value`: LinuxUserConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.linuxUserConfiguration = mapped
}
/**
* @param argument This property is ignored if specified on a Windows pool. If not specified, the user is created with the default options.
*/
@JvmName("uvxowtrorxhbqarx")
public suspend fun linuxUserConfiguration(argument: suspend LinuxUserConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = LinuxUserConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.linuxUserConfiguration = mapped
}
/**
* @param value
*/
@JvmName("euetqhnhbcjuknku")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("jvlcgdwvfldqltuw")
public suspend fun password(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value This property can only be specified if the user is on a Windows pool. If not specified and on a Windows pool, the user is created with the default options.
*/
@JvmName("igygttfsoqshouvs")
public suspend fun windowsUserConfiguration(`value`: WindowsUserConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.windowsUserConfiguration = mapped
}
/**
* @param argument This property can only be specified if the user is on a Windows pool. If not specified and on a Windows pool, the user is created with the default options.
*/
@JvmName("njxyhrxbnqrynsai")
public suspend fun windowsUserConfiguration(argument: suspend WindowsUserConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = WindowsUserConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.windowsUserConfiguration = mapped
}
internal fun build(): UserAccountArgs = UserAccountArgs(
elevationLevel = elevationLevel,
linuxUserConfiguration = linuxUserConfiguration,
name = name ?: throw PulumiNullFieldException("name"),
password = password ?: throw PulumiNullFieldException("password"),
windowsUserConfiguration = windowsUserConfiguration,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy