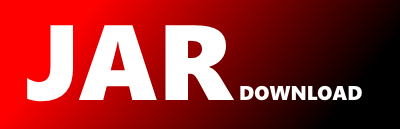
com.pulumi.azurenative.batch.kotlin.outputs.GetBatchAccountResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.batch.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Contains information about an Azure Batch account.
* @property accountEndpoint The account endpoint used to interact with the Batch service.
* @property activeJobAndJobScheduleQuota
* @property allowedAuthenticationModes List of allowed authentication modes for the Batch account that can be used to authenticate with the data plane. This does not affect authentication with the control plane.
* @property autoStorage Contains information about the auto-storage account associated with a Batch account.
* @property dedicatedCoreQuota For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
* @property dedicatedCoreQuotaPerVMFamily A list of the dedicated core quota per Virtual Machine family for the Batch account. For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
* @property dedicatedCoreQuotaPerVMFamilyEnforced If this flag is true, dedicated core quota is enforced via both the dedicatedCoreQuotaPerVMFamily and dedicatedCoreQuota properties on the account. If this flag is false, dedicated core quota is enforced only via the dedicatedCoreQuota property on the account and does not consider Virtual Machine family.
* @property encryption Configures how customer data is encrypted inside the Batch account. By default, accounts are encrypted using a Microsoft managed key. For additional control, a customer-managed key can be used instead.
* @property id The ID of the resource.
* @property identity The identity of the Batch account.
* @property keyVaultReference Identifies the Azure key vault associated with a Batch account.
* @property location The location of the resource.
* @property lowPriorityCoreQuota For accounts with PoolAllocationMode set to UserSubscription, quota is managed on the subscription so this value is not returned.
* @property name The name of the resource.
* @property networkProfile The network profile only takes effect when publicNetworkAccess is enabled.
* @property nodeManagementEndpoint The endpoint used by compute node to connect to the Batch node management service.
* @property poolAllocationMode The allocation mode for creating pools in the Batch account.
* @property poolQuota
* @property privateEndpointConnections List of private endpoint connections associated with the Batch account
* @property provisioningState The provisioned state of the resource
* @property publicNetworkAccess If not specified, the default value is 'enabled'.
* @property tags The tags of the resource.
* @property type The type of the resource.
*/
public data class GetBatchAccountResult(
public val accountEndpoint: String,
public val activeJobAndJobScheduleQuota: Int,
public val allowedAuthenticationModes: List,
public val autoStorage: AutoStoragePropertiesResponse,
public val dedicatedCoreQuota: Int,
public val dedicatedCoreQuotaPerVMFamily: List,
public val dedicatedCoreQuotaPerVMFamilyEnforced: Boolean,
public val encryption: EncryptionPropertiesResponse,
public val id: String,
public val identity: BatchAccountIdentityResponse? = null,
public val keyVaultReference: KeyVaultReferenceResponse,
public val location: String,
public val lowPriorityCoreQuota: Int,
public val name: String,
public val networkProfile: NetworkProfileResponse? = null,
public val nodeManagementEndpoint: String,
public val poolAllocationMode: String,
public val poolQuota: Int,
public val privateEndpointConnections: List,
public val provisioningState: String,
public val publicNetworkAccess: String? = null,
public val tags: Map,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.batch.outputs.GetBatchAccountResult): GetBatchAccountResult = GetBatchAccountResult(
accountEndpoint = javaType.accountEndpoint(),
activeJobAndJobScheduleQuota = javaType.activeJobAndJobScheduleQuota(),
allowedAuthenticationModes = javaType.allowedAuthenticationModes().map({ args0 -> args0 }),
autoStorage = javaType.autoStorage().let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.AutoStoragePropertiesResponse.Companion.toKotlin(args0)
}),
dedicatedCoreQuota = javaType.dedicatedCoreQuota(),
dedicatedCoreQuotaPerVMFamily = javaType.dedicatedCoreQuotaPerVMFamily().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.VirtualMachineFamilyCoreQuotaResponse.Companion.toKotlin(args0)
})
}),
dedicatedCoreQuotaPerVMFamilyEnforced = javaType.dedicatedCoreQuotaPerVMFamilyEnforced(),
encryption = javaType.encryption().let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.EncryptionPropertiesResponse.Companion.toKotlin(args0)
}),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.BatchAccountIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
keyVaultReference = javaType.keyVaultReference().let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.KeyVaultReferenceResponse.Companion.toKotlin(args0)
}),
location = javaType.location(),
lowPriorityCoreQuota = javaType.lowPriorityCoreQuota(),
name = javaType.name(),
networkProfile = javaType.networkProfile().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.NetworkProfileResponse.Companion.toKotlin(args0)
})
}).orElse(null),
nodeManagementEndpoint = javaType.nodeManagementEndpoint(),
poolAllocationMode = javaType.poolAllocationMode(),
poolQuota = javaType.poolQuota(),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.batch.kotlin.outputs.PrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
publicNetworkAccess = javaType.publicNetworkAccess().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy