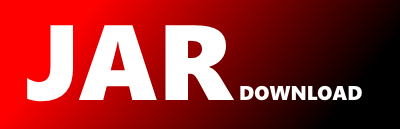
com.pulumi.azurenative.billing.kotlin.BillingProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.billing.kotlin
import com.pulumi.azurenative.billing.BillingProfileArgs.builder
import com.pulumi.azurenative.billing.kotlin.inputs.BillingProfilePropertiesArgs
import com.pulumi.azurenative.billing.kotlin.inputs.BillingProfilePropertiesArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A billing profile.
* Azure REST API version: 2024-04-01.
* ## Example Usage
* ### BillingProfilesCreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var billingProfile = new AzureNative.Billing.BillingProfile("billingProfile", new()
* {
* BillingAccountName = "00000000-0000-0000-0000-000000000000:00000000-0000-0000-0000-000000000000_2019-05-31",
* BillingProfileName = "xxxx-xxxx-xxx-xxx",
* Properties = new AzureNative.Billing.Inputs.BillingProfilePropertiesArgs
* {
* BillTo = new AzureNative.Billing.Inputs.BillingProfilePropertiesBillToArgs
* {
* AddressLine1 = "Test Address1",
* AddressLine2 = "Test Address2",
* AddressLine3 = "Test Address3",
* City = "City",
* CompanyName = "Contoso",
* Country = "US",
* Email = "[email protected]",
* FirstName = "Test",
* IsValidAddress = true,
* LastName = "User",
* PhoneNumber = "000-000-0000",
* PostalCode = "00000",
* Region = "WA",
* },
* DisplayName = "Billing Profile 1",
* EnabledAzurePlans = new[]
* {
* new AzureNative.Billing.Inputs.AzurePlanArgs
* {
* SkuId = "0001",
* },
* new AzureNative.Billing.Inputs.AzurePlanArgs
* {
* SkuId = "0002",
* },
* },
* InvoiceEmailOptIn = true,
* PoNumber = "ABC12345",
* ShipTo = new AzureNative.Billing.Inputs.BillingProfilePropertiesShipToArgs
* {
* AddressLine1 = "Test Address1",
* AddressLine2 = "Test Address2",
* AddressLine3 = "Test Address3",
* City = "City",
* CompanyName = "Contoso",
* Country = "US",
* Email = "[email protected]",
* FirstName = "Test",
* IsValidAddress = true,
* LastName = "User",
* PhoneNumber = "000-000-0000",
* PostalCode = "00000",
* Region = "WA",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* billing "github.com/pulumi/pulumi-azure-native-sdk/billing/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := billing.NewBillingProfile(ctx, "billingProfile", &billing.BillingProfileArgs{
* BillingAccountName: pulumi.String("00000000-0000-0000-0000-000000000000:00000000-0000-0000-0000-000000000000_2019-05-31"),
* BillingProfileName: pulumi.String("xxxx-xxxx-xxx-xxx"),
* Properties: &billing.BillingProfilePropertiesArgs{
* BillTo: &billing.BillingProfilePropertiesBillToArgs{
* AddressLine1: pulumi.String("Test Address1"),
* AddressLine2: pulumi.String("Test Address2"),
* AddressLine3: pulumi.String("Test Address3"),
* City: pulumi.String("City"),
* CompanyName: pulumi.String("Contoso"),
* Country: pulumi.String("US"),
* Email: pulumi.String("[email protected]"),
* FirstName: pulumi.String("Test"),
* IsValidAddress: pulumi.Bool(true),
* LastName: pulumi.String("User"),
* PhoneNumber: pulumi.String("000-000-0000"),
* PostalCode: pulumi.String("00000"),
* Region: pulumi.String("WA"),
* },
* DisplayName: pulumi.String("Billing Profile 1"),
* EnabledAzurePlans: billing.AzurePlanArray{
* &billing.AzurePlanArgs{
* SkuId: pulumi.String("0001"),
* },
* &billing.AzurePlanArgs{
* SkuId: pulumi.String("0002"),
* },
* },
* InvoiceEmailOptIn: pulumi.Bool(true),
* PoNumber: pulumi.String("ABC12345"),
* ShipTo: &billing.BillingProfilePropertiesShipToArgs{
* AddressLine1: pulumi.String("Test Address1"),
* AddressLine2: pulumi.String("Test Address2"),
* AddressLine3: pulumi.String("Test Address3"),
* City: pulumi.String("City"),
* CompanyName: pulumi.String("Contoso"),
* Country: pulumi.String("US"),
* Email: pulumi.String("[email protected]"),
* FirstName: pulumi.String("Test"),
* IsValidAddress: pulumi.Bool(true),
* LastName: pulumi.String("User"),
* PhoneNumber: pulumi.String("000-000-0000"),
* PostalCode: pulumi.String("00000"),
* Region: pulumi.String("WA"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.billing.BillingProfile;
* import com.pulumi.azurenative.billing.BillingProfileArgs;
* import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesArgs;
* import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesBillToArgs;
* import com.pulumi.azurenative.billing.inputs.BillingProfilePropertiesShipToArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var billingProfile = new BillingProfile("billingProfile", BillingProfileArgs.builder()
* .billingAccountName("00000000-0000-0000-0000-000000000000:00000000-0000-0000-0000-000000000000_2019-05-31")
* .billingProfileName("xxxx-xxxx-xxx-xxx")
* .properties(BillingProfilePropertiesArgs.builder()
* .billTo(BillingProfilePropertiesBillToArgs.builder()
* .addressLine1("Test Address1")
* .addressLine2("Test Address2")
* .addressLine3("Test Address3")
* .city("City")
* .companyName("Contoso")
* .country("US")
* .email("[email protected]")
* .firstName("Test")
* .isValidAddress(true)
* .lastName("User")
* .phoneNumber("000-000-0000")
* .postalCode("00000")
* .region("WA")
* .build())
* .displayName("Billing Profile 1")
* .enabledAzurePlans(
* AzurePlanArgs.builder()
* .skuId("0001")
* .build(),
* AzurePlanArgs.builder()
* .skuId("0002")
* .build())
* .invoiceEmailOptIn(true)
* .poNumber("ABC12345")
* .shipTo(BillingProfilePropertiesShipToArgs.builder()
* .addressLine1("Test Address1")
* .addressLine2("Test Address2")
* .addressLine3("Test Address3")
* .city("City")
* .companyName("Contoso")
* .country("US")
* .email("[email protected]")
* .firstName("Test")
* .isValidAddress(true)
* .lastName("User")
* .phoneNumber("000-000-0000")
* .postalCode("00000")
* .region("WA")
* .build())
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:billing:BillingProfile xxxx-xxxx-xxx-xxx /providers/Microsoft.Billing/billingAccounts/{billingAccountName}/billingProfiles/{billingProfileName}
* ```
* @property billingAccountName The ID that uniquely identifies a billing account.
* @property billingProfileName The ID that uniquely identifies a billing profile.
* @property properties A billing profile.
* @property tags Dictionary of metadata associated with the resource. It may not be populated for all resource types. Maximum key/value length supported of 256 characters. Keys/value should not empty value nor null. Keys can not contain < > % & \ ? /
*/
public data class BillingProfileArgs(
public val billingAccountName: Output? = null,
public val billingProfileName: Output? = null,
public val properties: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy