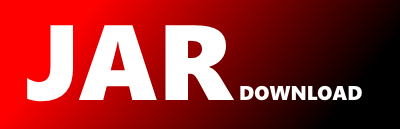
com.pulumi.azurenative.billing.kotlin.inputs.AssociatedTenantPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.billing.kotlin.inputs
import com.pulumi.azurenative.billing.inputs.AssociatedTenantPropertiesArgs.builder
import com.pulumi.azurenative.billing.kotlin.enums.BillingManagementTenantState
import com.pulumi.azurenative.billing.kotlin.enums.ProvisioningTenantState
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* An associated tenant.
* @property billingManagementState The state determines whether users from the associated tenant can be assigned roles for commerce activities like viewing and downloading invoices, managing payments, and making purchases.
* @property displayName The name of the associated tenant.
* @property provisioningManagementState The state determines whether subscriptions and licenses can be provisioned in the associated tenant. It can be set to 'Pending' to initiate a billing request.
* @property tenantId The ID that uniquely identifies a tenant.
*/
public data class AssociatedTenantPropertiesArgs(
public val billingManagementState: Output>? = null,
public val displayName: Output? = null,
public val provisioningManagementState: Output>? = null,
public val tenantId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.billing.inputs.AssociatedTenantPropertiesArgs =
com.pulumi.azurenative.billing.inputs.AssociatedTenantPropertiesArgs.builder()
.billingManagementState(
billingManagementState?.applyValue({ args0 ->
args0.transform({ args0 ->
args0
}, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.displayName(displayName?.applyValue({ args0 -> args0 }))
.provisioningManagementState(
provisioningManagementState?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
)
.tenantId(tenantId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AssociatedTenantPropertiesArgs].
*/
@PulumiTagMarker
public class AssociatedTenantPropertiesArgsBuilder internal constructor() {
private var billingManagementState: Output>? = null
private var displayName: Output? = null
private var provisioningManagementState: Output>? = null
private var tenantId: Output? = null
/**
* @param value The state determines whether users from the associated tenant can be assigned roles for commerce activities like viewing and downloading invoices, managing payments, and making purchases.
*/
@JvmName("jgldluqxwixbcllc")
public suspend fun billingManagementState(`value`: Output>) {
this.billingManagementState = value
}
/**
* @param value The name of the associated tenant.
*/
@JvmName("fgxetbtjfnenfoad")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value The state determines whether subscriptions and licenses can be provisioned in the associated tenant. It can be set to 'Pending' to initiate a billing request.
*/
@JvmName("ynstkoyyfvhunkgf")
public suspend fun provisioningManagementState(`value`: Output>) {
this.provisioningManagementState = value
}
/**
* @param value The ID that uniquely identifies a tenant.
*/
@JvmName("nrsabbktmjgleeck")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value The state determines whether users from the associated tenant can be assigned roles for commerce activities like viewing and downloading invoices, managing payments, and making purchases.
*/
@JvmName("gtkjxwpxhasogomf")
public suspend fun billingManagementState(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.billingManagementState = mapped
}
/**
* @param value The state determines whether users from the associated tenant can be assigned roles for commerce activities like viewing and downloading invoices, managing payments, and making purchases.
*/
@JvmName("dwihmijurswfmvgx")
public fun billingManagementState(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.billingManagementState = mapped
}
/**
* @param value The state determines whether users from the associated tenant can be assigned roles for commerce activities like viewing and downloading invoices, managing payments, and making purchases.
*/
@JvmName("dkeingbbmpdumbtn")
public fun billingManagementState(`value`: BillingManagementTenantState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.billingManagementState = mapped
}
/**
* @param value The name of the associated tenant.
*/
@JvmName("phhdpwmkscixbfwd")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value The state determines whether subscriptions and licenses can be provisioned in the associated tenant. It can be set to 'Pending' to initiate a billing request.
*/
@JvmName("lbapqtivhduinqbh")
public suspend fun provisioningManagementState(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.provisioningManagementState = mapped
}
/**
* @param value The state determines whether subscriptions and licenses can be provisioned in the associated tenant. It can be set to 'Pending' to initiate a billing request.
*/
@JvmName("wuffswrfveswbnoc")
public fun provisioningManagementState(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningManagementState = mapped
}
/**
* @param value The state determines whether subscriptions and licenses can be provisioned in the associated tenant. It can be set to 'Pending' to initiate a billing request.
*/
@JvmName("edwriqeuvtumxjfs")
public fun provisioningManagementState(`value`: ProvisioningTenantState) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.provisioningManagementState = mapped
}
/**
* @param value The ID that uniquely identifies a tenant.
*/
@JvmName("tftpdnfvktujwwla")
public suspend fun tenantId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tenantId = mapped
}
internal fun build(): AssociatedTenantPropertiesArgs = AssociatedTenantPropertiesArgs(
billingManagementState = billingManagementState,
displayName = displayName,
provisioningManagementState = provisioningManagementState,
tenantId = tenantId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy