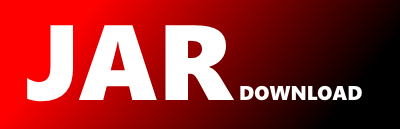
com.pulumi.azurenative.botservice.kotlin.inputs.DirectLineSiteArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.botservice.kotlin.inputs
import com.pulumi.azurenative.botservice.inputs.DirectLineSiteArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A site for the Direct Line channel
* @property appId DirectLine application id
* @property eTag Entity Tag
* @property isBlockUserUploadEnabled Whether this site is enabled for block user upload.
* @property isDetailedLoggingEnabled Whether this site is disabled detailed logging for
* @property isEnabled Whether this site is enabled for DirectLine channel
* @property isEndpointParametersEnabled Whether this site is EndpointParameters enabled for channel
* @property isNoStorageEnabled Whether this no-storage site is disabled detailed logging for
* @property isSecureSiteEnabled Whether this site is enabled for authentication with Bot Framework.
* @property isV1Enabled Whether this site is enabled for Bot Framework V1 protocol.
* @property isV3Enabled Whether this site is enabled for Bot Framework V3 protocol.
* @property isWebChatSpeechEnabled Whether this site is enabled for Webchat Speech
* @property isWebchatPreviewEnabled Whether this site is enabled for preview versions of Webchat
* @property siteName Site name
* @property tenantId Tenant Id
* @property trustedOrigins List of Trusted Origin URLs for this site. This field is applicable only if isSecureSiteEnabled is True.
*/
public data class DirectLineSiteArgs(
public val appId: Output? = null,
public val eTag: Output? = null,
public val isBlockUserUploadEnabled: Output? = null,
public val isDetailedLoggingEnabled: Output? = null,
public val isEnabled: Output,
public val isEndpointParametersEnabled: Output? = null,
public val isNoStorageEnabled: Output? = null,
public val isSecureSiteEnabled: Output? = null,
public val isV1Enabled: Output,
public val isV3Enabled: Output,
public val isWebChatSpeechEnabled: Output? = null,
public val isWebchatPreviewEnabled: Output? = null,
public val siteName: Output,
public val tenantId: Output? = null,
public val trustedOrigins: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.botservice.inputs.DirectLineSiteArgs =
com.pulumi.azurenative.botservice.inputs.DirectLineSiteArgs.builder()
.appId(appId?.applyValue({ args0 -> args0 }))
.eTag(eTag?.applyValue({ args0 -> args0 }))
.isBlockUserUploadEnabled(isBlockUserUploadEnabled?.applyValue({ args0 -> args0 }))
.isDetailedLoggingEnabled(isDetailedLoggingEnabled?.applyValue({ args0 -> args0 }))
.isEnabled(isEnabled.applyValue({ args0 -> args0 }))
.isEndpointParametersEnabled(isEndpointParametersEnabled?.applyValue({ args0 -> args0 }))
.isNoStorageEnabled(isNoStorageEnabled?.applyValue({ args0 -> args0 }))
.isSecureSiteEnabled(isSecureSiteEnabled?.applyValue({ args0 -> args0 }))
.isV1Enabled(isV1Enabled.applyValue({ args0 -> args0 }))
.isV3Enabled(isV3Enabled.applyValue({ args0 -> args0 }))
.isWebChatSpeechEnabled(isWebChatSpeechEnabled?.applyValue({ args0 -> args0 }))
.isWebchatPreviewEnabled(isWebchatPreviewEnabled?.applyValue({ args0 -> args0 }))
.siteName(siteName.applyValue({ args0 -> args0 }))
.tenantId(tenantId?.applyValue({ args0 -> args0 }))
.trustedOrigins(trustedOrigins?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [DirectLineSiteArgs].
*/
@PulumiTagMarker
public class DirectLineSiteArgsBuilder internal constructor() {
private var appId: Output? = null
private var eTag: Output? = null
private var isBlockUserUploadEnabled: Output? = null
private var isDetailedLoggingEnabled: Output? = null
private var isEnabled: Output? = null
private var isEndpointParametersEnabled: Output? = null
private var isNoStorageEnabled: Output? = null
private var isSecureSiteEnabled: Output? = null
private var isV1Enabled: Output? = null
private var isV3Enabled: Output? = null
private var isWebChatSpeechEnabled: Output? = null
private var isWebchatPreviewEnabled: Output? = null
private var siteName: Output? = null
private var tenantId: Output? = null
private var trustedOrigins: Output>? = null
/**
* @param value DirectLine application id
*/
@JvmName("kjjagymmqctnvmui")
public suspend fun appId(`value`: Output) {
this.appId = value
}
/**
* @param value Entity Tag
*/
@JvmName("jifysabeclmhnvpx")
public suspend fun eTag(`value`: Output) {
this.eTag = value
}
/**
* @param value Whether this site is enabled for block user upload.
*/
@JvmName("ondkurltshqagxut")
public suspend fun isBlockUserUploadEnabled(`value`: Output) {
this.isBlockUserUploadEnabled = value
}
/**
* @param value Whether this site is disabled detailed logging for
*/
@JvmName("msppqgxqygrjonbc")
public suspend fun isDetailedLoggingEnabled(`value`: Output) {
this.isDetailedLoggingEnabled = value
}
/**
* @param value Whether this site is enabled for DirectLine channel
*/
@JvmName("hvuhiurjtpkeeywp")
public suspend fun isEnabled(`value`: Output) {
this.isEnabled = value
}
/**
* @param value Whether this site is EndpointParameters enabled for channel
*/
@JvmName("rruppeimpqddvuxo")
public suspend fun isEndpointParametersEnabled(`value`: Output) {
this.isEndpointParametersEnabled = value
}
/**
* @param value Whether this no-storage site is disabled detailed logging for
*/
@JvmName("gmsqabebqbnvuhss")
public suspend fun isNoStorageEnabled(`value`: Output) {
this.isNoStorageEnabled = value
}
/**
* @param value Whether this site is enabled for authentication with Bot Framework.
*/
@JvmName("akawcwpylbnaotly")
public suspend fun isSecureSiteEnabled(`value`: Output) {
this.isSecureSiteEnabled = value
}
/**
* @param value Whether this site is enabled for Bot Framework V1 protocol.
*/
@JvmName("tqrplisarwdddkke")
public suspend fun isV1Enabled(`value`: Output) {
this.isV1Enabled = value
}
/**
* @param value Whether this site is enabled for Bot Framework V3 protocol.
*/
@JvmName("gadbcnixbjkvensm")
public suspend fun isV3Enabled(`value`: Output) {
this.isV3Enabled = value
}
/**
* @param value Whether this site is enabled for Webchat Speech
*/
@JvmName("prwiimbppchjvaed")
public suspend fun isWebChatSpeechEnabled(`value`: Output) {
this.isWebChatSpeechEnabled = value
}
/**
* @param value Whether this site is enabled for preview versions of Webchat
*/
@JvmName("xonhgcouqgetahde")
public suspend fun isWebchatPreviewEnabled(`value`: Output) {
this.isWebchatPreviewEnabled = value
}
/**
* @param value Site name
*/
@JvmName("mpmefpsqqdumsifq")
public suspend fun siteName(`value`: Output) {
this.siteName = value
}
/**
* @param value Tenant Id
*/
@JvmName("bthwuirmugqpejgt")
public suspend fun tenantId(`value`: Output) {
this.tenantId = value
}
/**
* @param value List of Trusted Origin URLs for this site. This field is applicable only if isSecureSiteEnabled is True.
*/
@JvmName("sbympewvawuggini")
public suspend fun trustedOrigins(`value`: Output>) {
this.trustedOrigins = value
}
@JvmName("ahhwaupmcaregiqx")
public suspend fun trustedOrigins(vararg values: Output) {
this.trustedOrigins = Output.all(values.asList())
}
/**
* @param values List of Trusted Origin URLs for this site. This field is applicable only if isSecureSiteEnabled is True.
*/
@JvmName("vaqxbppxsxjrbubj")
public suspend fun trustedOrigins(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy