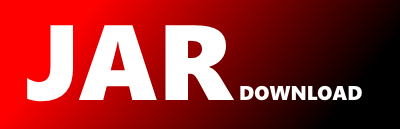
com.pulumi.azurenative.botservice.kotlin.inputs.SkypeChannelPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.botservice.kotlin.inputs
import com.pulumi.azurenative.botservice.inputs.SkypeChannelPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The parameters to provide for the Microsoft Teams channel.
* @property callingWebHook Calling web hook for Skype channel
* @property enableCalling Enable calling for Skype channel
* @property enableGroups Enable groups for Skype channel
* @property enableMediaCards Enable media cards for Skype channel
* @property enableMessaging Enable messaging for Skype channel
* @property enableScreenSharing Enable screen sharing for Skype channel
* @property enableVideo Enable video for Skype channel
* @property groupsMode Group mode for Skype channel
* @property incomingCallRoute Incoming call route for Skype channel
* @property isEnabled Whether this channel is enabled for the bot
*/
public data class SkypeChannelPropertiesArgs(
public val callingWebHook: Output? = null,
public val enableCalling: Output? = null,
public val enableGroups: Output? = null,
public val enableMediaCards: Output? = null,
public val enableMessaging: Output? = null,
public val enableScreenSharing: Output? = null,
public val enableVideo: Output? = null,
public val groupsMode: Output? = null,
public val incomingCallRoute: Output? = null,
public val isEnabled: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.botservice.inputs.SkypeChannelPropertiesArgs =
com.pulumi.azurenative.botservice.inputs.SkypeChannelPropertiesArgs.builder()
.callingWebHook(callingWebHook?.applyValue({ args0 -> args0 }))
.enableCalling(enableCalling?.applyValue({ args0 -> args0 }))
.enableGroups(enableGroups?.applyValue({ args0 -> args0 }))
.enableMediaCards(enableMediaCards?.applyValue({ args0 -> args0 }))
.enableMessaging(enableMessaging?.applyValue({ args0 -> args0 }))
.enableScreenSharing(enableScreenSharing?.applyValue({ args0 -> args0 }))
.enableVideo(enableVideo?.applyValue({ args0 -> args0 }))
.groupsMode(groupsMode?.applyValue({ args0 -> args0 }))
.incomingCallRoute(incomingCallRoute?.applyValue({ args0 -> args0 }))
.isEnabled(isEnabled.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SkypeChannelPropertiesArgs].
*/
@PulumiTagMarker
public class SkypeChannelPropertiesArgsBuilder internal constructor() {
private var callingWebHook: Output? = null
private var enableCalling: Output? = null
private var enableGroups: Output? = null
private var enableMediaCards: Output? = null
private var enableMessaging: Output? = null
private var enableScreenSharing: Output? = null
private var enableVideo: Output? = null
private var groupsMode: Output? = null
private var incomingCallRoute: Output? = null
private var isEnabled: Output? = null
/**
* @param value Calling web hook for Skype channel
*/
@JvmName("misaasdmyssoapdk")
public suspend fun callingWebHook(`value`: Output) {
this.callingWebHook = value
}
/**
* @param value Enable calling for Skype channel
*/
@JvmName("jlqvgkqijifagael")
public suspend fun enableCalling(`value`: Output) {
this.enableCalling = value
}
/**
* @param value Enable groups for Skype channel
*/
@JvmName("wynepkgmgdqarxbv")
public suspend fun enableGroups(`value`: Output) {
this.enableGroups = value
}
/**
* @param value Enable media cards for Skype channel
*/
@JvmName("esvxrxpghwajdlic")
public suspend fun enableMediaCards(`value`: Output) {
this.enableMediaCards = value
}
/**
* @param value Enable messaging for Skype channel
*/
@JvmName("nucytygnynefjasw")
public suspend fun enableMessaging(`value`: Output) {
this.enableMessaging = value
}
/**
* @param value Enable screen sharing for Skype channel
*/
@JvmName("opitjleulhiicmex")
public suspend fun enableScreenSharing(`value`: Output) {
this.enableScreenSharing = value
}
/**
* @param value Enable video for Skype channel
*/
@JvmName("tdgctujbtyjicjfn")
public suspend fun enableVideo(`value`: Output) {
this.enableVideo = value
}
/**
* @param value Group mode for Skype channel
*/
@JvmName("swumxvscbllrrsuw")
public suspend fun groupsMode(`value`: Output) {
this.groupsMode = value
}
/**
* @param value Incoming call route for Skype channel
*/
@JvmName("xiyvxpsmrgxrhyie")
public suspend fun incomingCallRoute(`value`: Output) {
this.incomingCallRoute = value
}
/**
* @param value Whether this channel is enabled for the bot
*/
@JvmName("bwdvffrtmpauuyfu")
public suspend fun isEnabled(`value`: Output) {
this.isEnabled = value
}
/**
* @param value Calling web hook for Skype channel
*/
@JvmName("hemjtvaymluaxqpk")
public suspend fun callingWebHook(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.callingWebHook = mapped
}
/**
* @param value Enable calling for Skype channel
*/
@JvmName("uepplkdttfbkkfew")
public suspend fun enableCalling(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableCalling = mapped
}
/**
* @param value Enable groups for Skype channel
*/
@JvmName("vepskqhooejhobfr")
public suspend fun enableGroups(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableGroups = mapped
}
/**
* @param value Enable media cards for Skype channel
*/
@JvmName("mqdtxuwgwoeawfsn")
public suspend fun enableMediaCards(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableMediaCards = mapped
}
/**
* @param value Enable messaging for Skype channel
*/
@JvmName("doqrhvqlupvytesl")
public suspend fun enableMessaging(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableMessaging = mapped
}
/**
* @param value Enable screen sharing for Skype channel
*/
@JvmName("tdlnwgaobmaybrst")
public suspend fun enableScreenSharing(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableScreenSharing = mapped
}
/**
* @param value Enable video for Skype channel
*/
@JvmName("iuqmrsvdkborgniy")
public suspend fun enableVideo(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableVideo = mapped
}
/**
* @param value Group mode for Skype channel
*/
@JvmName("pdjjvkgqpdktgfle")
public suspend fun groupsMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.groupsMode = mapped
}
/**
* @param value Incoming call route for Skype channel
*/
@JvmName("hyphtehjewnatlyi")
public suspend fun incomingCallRoute(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.incomingCallRoute = mapped
}
/**
* @param value Whether this channel is enabled for the bot
*/
@JvmName("ahvjtnjskoplofvu")
public suspend fun isEnabled(`value`: Boolean) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.isEnabled = mapped
}
internal fun build(): SkypeChannelPropertiesArgs = SkypeChannelPropertiesArgs(
callingWebHook = callingWebHook,
enableCalling = enableCalling,
enableGroups = enableGroups,
enableMediaCards = enableMediaCards,
enableMessaging = enableMessaging,
enableScreenSharing = enableScreenSharing,
enableVideo = enableVideo,
groupsMode = groupsMode,
incomingCallRoute = incomingCallRoute,
isEnabled = isEnabled ?: throw PulumiNullFieldException("isEnabled"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy