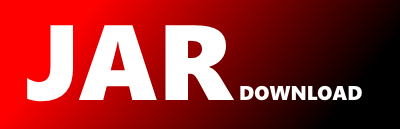
com.pulumi.azurenative.botservice.kotlin.inputs.TelephonyPhoneNumbersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.botservice.kotlin.inputs
import com.pulumi.azurenative.botservice.inputs.TelephonyPhoneNumbersArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A telephone number for the Telephony channel
* @property acsEndpoint The endpoint of ACS.
* @property acsResourceId The resource id of ACS.
* @property acsSecret The secret of ACS.
* @property cognitiveServiceRegion The service region of cognitive service.
* @property cognitiveServiceResourceId The resource id of cognitive service.
* @property cognitiveServiceSubscriptionKey The subscription key of cognitive service.
* @property defaultLocale The default locale of the phone number.
* @property id The element id.
* @property offerType Optional Property that will determine the offering type of the phone.
* @property phoneNumber The phone number.
*/
public data class TelephonyPhoneNumbersArgs(
public val acsEndpoint: Output? = null,
public val acsResourceId: Output? = null,
public val acsSecret: Output? = null,
public val cognitiveServiceRegion: Output? = null,
public val cognitiveServiceResourceId: Output? = null,
public val cognitiveServiceSubscriptionKey: Output? = null,
public val defaultLocale: Output? = null,
public val id: Output? = null,
public val offerType: Output? = null,
public val phoneNumber: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.botservice.inputs.TelephonyPhoneNumbersArgs =
com.pulumi.azurenative.botservice.inputs.TelephonyPhoneNumbersArgs.builder()
.acsEndpoint(acsEndpoint?.applyValue({ args0 -> args0 }))
.acsResourceId(acsResourceId?.applyValue({ args0 -> args0 }))
.acsSecret(acsSecret?.applyValue({ args0 -> args0 }))
.cognitiveServiceRegion(cognitiveServiceRegion?.applyValue({ args0 -> args0 }))
.cognitiveServiceResourceId(cognitiveServiceResourceId?.applyValue({ args0 -> args0 }))
.cognitiveServiceSubscriptionKey(cognitiveServiceSubscriptionKey?.applyValue({ args0 -> args0 }))
.defaultLocale(defaultLocale?.applyValue({ args0 -> args0 }))
.id(id?.applyValue({ args0 -> args0 }))
.offerType(offerType?.applyValue({ args0 -> args0 }))
.phoneNumber(phoneNumber?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [TelephonyPhoneNumbersArgs].
*/
@PulumiTagMarker
public class TelephonyPhoneNumbersArgsBuilder internal constructor() {
private var acsEndpoint: Output? = null
private var acsResourceId: Output? = null
private var acsSecret: Output? = null
private var cognitiveServiceRegion: Output? = null
private var cognitiveServiceResourceId: Output? = null
private var cognitiveServiceSubscriptionKey: Output? = null
private var defaultLocale: Output? = null
private var id: Output? = null
private var offerType: Output? = null
private var phoneNumber: Output? = null
/**
* @param value The endpoint of ACS.
*/
@JvmName("sbemiewflbekgojw")
public suspend fun acsEndpoint(`value`: Output) {
this.acsEndpoint = value
}
/**
* @param value The resource id of ACS.
*/
@JvmName("nvkljcrmrrtpmsxg")
public suspend fun acsResourceId(`value`: Output) {
this.acsResourceId = value
}
/**
* @param value The secret of ACS.
*/
@JvmName("ieuxjrujbtmxcnwr")
public suspend fun acsSecret(`value`: Output) {
this.acsSecret = value
}
/**
* @param value The service region of cognitive service.
*/
@JvmName("cupbfjugryoqfcve")
public suspend fun cognitiveServiceRegion(`value`: Output) {
this.cognitiveServiceRegion = value
}
/**
* @param value The resource id of cognitive service.
*/
@JvmName("ybvwuudjftvjwlgi")
public suspend fun cognitiveServiceResourceId(`value`: Output) {
this.cognitiveServiceResourceId = value
}
/**
* @param value The subscription key of cognitive service.
*/
@JvmName("wimpadxgrvfmfona")
public suspend fun cognitiveServiceSubscriptionKey(`value`: Output) {
this.cognitiveServiceSubscriptionKey = value
}
/**
* @param value The default locale of the phone number.
*/
@JvmName("lvcfhowooawigxwp")
public suspend fun defaultLocale(`value`: Output) {
this.defaultLocale = value
}
/**
* @param value The element id.
*/
@JvmName("flqblvagicquxibl")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value Optional Property that will determine the offering type of the phone.
*/
@JvmName("pqwtjphqtmpnpibs")
public suspend fun offerType(`value`: Output) {
this.offerType = value
}
/**
* @param value The phone number.
*/
@JvmName("ewdbycnxnualxcpu")
public suspend fun phoneNumber(`value`: Output) {
this.phoneNumber = value
}
/**
* @param value The endpoint of ACS.
*/
@JvmName("mualqbcxixfgxivy")
public suspend fun acsEndpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acsEndpoint = mapped
}
/**
* @param value The resource id of ACS.
*/
@JvmName("ykhpbltnsjykfdot")
public suspend fun acsResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acsResourceId = mapped
}
/**
* @param value The secret of ACS.
*/
@JvmName("qjfmqcbxxcftpnes")
public suspend fun acsSecret(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acsSecret = mapped
}
/**
* @param value The service region of cognitive service.
*/
@JvmName("mbbjyneubcaipmmh")
public suspend fun cognitiveServiceRegion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cognitiveServiceRegion = mapped
}
/**
* @param value The resource id of cognitive service.
*/
@JvmName("dwrdlynebexjoiba")
public suspend fun cognitiveServiceResourceId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cognitiveServiceResourceId = mapped
}
/**
* @param value The subscription key of cognitive service.
*/
@JvmName("gflkmonpdsrqbdye")
public suspend fun cognitiveServiceSubscriptionKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cognitiveServiceSubscriptionKey = mapped
}
/**
* @param value The default locale of the phone number.
*/
@JvmName("ududwhjrbrotabfm")
public suspend fun defaultLocale(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.defaultLocale = mapped
}
/**
* @param value The element id.
*/
@JvmName("mfguouavsualscsr")
public suspend fun id(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.id = mapped
}
/**
* @param value Optional Property that will determine the offering type of the phone.
*/
@JvmName("xefclcqyhlgevxps")
public suspend fun offerType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.offerType = mapped
}
/**
* @param value The phone number.
*/
@JvmName("yumqskwfmgjfjlgk")
public suspend fun phoneNumber(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.phoneNumber = mapped
}
internal fun build(): TelephonyPhoneNumbersArgs = TelephonyPhoneNumbersArgs(
acsEndpoint = acsEndpoint,
acsResourceId = acsResourceId,
acsSecret = acsSecret,
cognitiveServiceRegion = cognitiveServiceRegion,
cognitiveServiceResourceId = cognitiveServiceResourceId,
cognitiveServiceSubscriptionKey = cognitiveServiceSubscriptionKey,
defaultLocale = defaultLocale,
id = id,
offerType = offerType,
phoneNumber = phoneNumber,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy