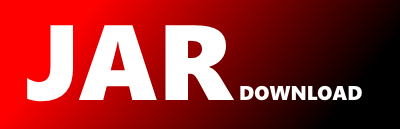
com.pulumi.azurenative.cache.kotlin.RedisArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cache.kotlin
import com.pulumi.azurenative.cache.RedisArgs.builder
import com.pulumi.azurenative.cache.kotlin.enums.PublicNetworkAccess
import com.pulumi.azurenative.cache.kotlin.enums.TlsVersion
import com.pulumi.azurenative.cache.kotlin.inputs.ManagedServiceIdentityArgs
import com.pulumi.azurenative.cache.kotlin.inputs.ManagedServiceIdentityArgsBuilder
import com.pulumi.azurenative.cache.kotlin.inputs.RedisCommonPropertiesRedisConfigurationArgs
import com.pulumi.azurenative.cache.kotlin.inputs.RedisCommonPropertiesRedisConfigurationArgsBuilder
import com.pulumi.azurenative.cache.kotlin.inputs.SkuArgs
import com.pulumi.azurenative.cache.kotlin.inputs.SkuArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* A single Redis item in List or Get Operation.
* Azure REST API version: 2023-04-01. Prior API version in Azure Native 1.x: 2020-06-01.
* Other available API versions: 2015-08-01, 2017-02-01, 2019-07-01, 2020-06-01, 2023-05-01-preview, 2023-08-01, 2024-03-01, 2024-04-01-preview.
* ## Example Usage
* ### RedisCacheCreate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var redis = new AzureNative.Cache.Redis("redis", new()
* {
* EnableNonSslPort = true,
* Location = "West US",
* MinimumTlsVersion = AzureNative.Cache.TlsVersion.TlsVersion_1_2,
* Name = "cache1",
* RedisConfiguration = new AzureNative.Cache.Inputs.RedisCommonPropertiesRedisConfigurationArgs
* {
* MaxmemoryPolicy = "allkeys-lru",
* },
* RedisVersion = "4",
* ReplicasPerPrimary = 2,
* ResourceGroupName = "rg1",
* ShardCount = 2,
* Sku = new AzureNative.Cache.Inputs.SkuArgs
* {
* Capacity = 1,
* Family = AzureNative.Cache.SkuFamily.P,
* Name = "Premium",
* },
* StaticIP = "192.168.0.5",
* SubnetId = "/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1",
* Zones = new[]
* {
* "1",
* },
* });
* });
* ```
* ```go
* package main
* import (
* cache "github.com/pulumi/pulumi-azure-native-sdk/cache/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cache.NewRedis(ctx, "redis", &cache.RedisArgs{
* EnableNonSslPort: pulumi.Bool(true),
* Location: pulumi.String("West US"),
* MinimumTlsVersion: pulumi.String(cache.TlsVersion_1_2),
* Name: pulumi.String("cache1"),
* RedisConfiguration: &cache.RedisCommonPropertiesRedisConfigurationArgs{
* MaxmemoryPolicy: pulumi.String("allkeys-lru"),
* },
* RedisVersion: pulumi.String("4"),
* ReplicasPerPrimary: pulumi.Int(2),
* ResourceGroupName: pulumi.String("rg1"),
* ShardCount: pulumi.Int(2),
* Sku: &cache.SkuArgs{
* Capacity: pulumi.Int(1),
* Family: pulumi.String(cache.SkuFamilyP),
* Name: pulumi.String("Premium"),
* },
* StaticIP: pulumi.String("192.168.0.5"),
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1"),
* Zones: pulumi.StringArray{
* pulumi.String("1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .redisVersion("4")
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
* }
* }
* ```
* ### RedisCacheCreateDefaultVersion
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var redis = new AzureNative.Cache.Redis("redis", new()
* {
* EnableNonSslPort = true,
* Location = "West US",
* MinimumTlsVersion = AzureNative.Cache.TlsVersion.TlsVersion_1_2,
* Name = "cache1",
* RedisConfiguration = new AzureNative.Cache.Inputs.RedisCommonPropertiesRedisConfigurationArgs
* {
* MaxmemoryPolicy = "allkeys-lru",
* },
* ReplicasPerPrimary = 2,
* ResourceGroupName = "rg1",
* ShardCount = 2,
* Sku = new AzureNative.Cache.Inputs.SkuArgs
* {
* Capacity = 1,
* Family = AzureNative.Cache.SkuFamily.P,
* Name = "Premium",
* },
* StaticIP = "192.168.0.5",
* SubnetId = "/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1",
* Zones = new[]
* {
* "1",
* },
* });
* });
* ```
* ```go
* package main
* import (
* cache "github.com/pulumi/pulumi-azure-native-sdk/cache/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cache.NewRedis(ctx, "redis", &cache.RedisArgs{
* EnableNonSslPort: pulumi.Bool(true),
* Location: pulumi.String("West US"),
* MinimumTlsVersion: pulumi.String(cache.TlsVersion_1_2),
* Name: pulumi.String("cache1"),
* RedisConfiguration: &cache.RedisCommonPropertiesRedisConfigurationArgs{
* MaxmemoryPolicy: pulumi.String("allkeys-lru"),
* },
* ReplicasPerPrimary: pulumi.Int(2),
* ResourceGroupName: pulumi.String("rg1"),
* ShardCount: pulumi.Int(2),
* Sku: &cache.SkuArgs{
* Capacity: pulumi.Int(1),
* Family: pulumi.String(cache.SkuFamilyP),
* Name: pulumi.String("Premium"),
* },
* StaticIP: pulumi.String("192.168.0.5"),
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1"),
* Zones: pulumi.StringArray{
* pulumi.String("1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
* }
* }
* ```
* ### RedisCacheCreateLatestVersion
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var redis = new AzureNative.Cache.Redis("redis", new()
* {
* EnableNonSslPort = true,
* Location = "West US",
* MinimumTlsVersion = AzureNative.Cache.TlsVersion.TlsVersion_1_2,
* Name = "cache1",
* RedisConfiguration = new AzureNative.Cache.Inputs.RedisCommonPropertiesRedisConfigurationArgs
* {
* MaxmemoryPolicy = "allkeys-lru",
* },
* RedisVersion = "Latest",
* ReplicasPerPrimary = 2,
* ResourceGroupName = "rg1",
* ShardCount = 2,
* Sku = new AzureNative.Cache.Inputs.SkuArgs
* {
* Capacity = 1,
* Family = AzureNative.Cache.SkuFamily.P,
* Name = "Premium",
* },
* StaticIP = "192.168.0.5",
* SubnetId = "/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1",
* Zones = new[]
* {
* "1",
* },
* });
* });
* ```
* ```go
* package main
* import (
* cache "github.com/pulumi/pulumi-azure-native-sdk/cache/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cache.NewRedis(ctx, "redis", &cache.RedisArgs{
* EnableNonSslPort: pulumi.Bool(true),
* Location: pulumi.String("West US"),
* MinimumTlsVersion: pulumi.String(cache.TlsVersion_1_2),
* Name: pulumi.String("cache1"),
* RedisConfiguration: &cache.RedisCommonPropertiesRedisConfigurationArgs{
* MaxmemoryPolicy: pulumi.String("allkeys-lru"),
* },
* RedisVersion: pulumi.String("Latest"),
* ReplicasPerPrimary: pulumi.Int(2),
* ResourceGroupName: pulumi.String("rg1"),
* ShardCount: pulumi.Int(2),
* Sku: &cache.SkuArgs{
* Capacity: pulumi.Int(1),
* Family: pulumi.String(cache.SkuFamilyP),
* Name: pulumi.String("Premium"),
* },
* StaticIP: pulumi.String("192.168.0.5"),
* SubnetId: pulumi.String("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1"),
* Zones: pulumi.StringArray{
* pulumi.String("1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cache.Redis;
* import com.pulumi.azurenative.cache.RedisArgs;
* import com.pulumi.azurenative.cache.inputs.RedisCommonPropertiesRedisConfigurationArgs;
* import com.pulumi.azurenative.cache.inputs.SkuArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var redis = new Redis("redis", RedisArgs.builder()
* .enableNonSslPort(true)
* .location("West US")
* .minimumTlsVersion("1.2")
* .name("cache1")
* .redisConfiguration(RedisCommonPropertiesRedisConfigurationArgs.builder()
* .maxmemoryPolicy("allkeys-lru")
* .build())
* .redisVersion("Latest")
* .replicasPerPrimary(2)
* .resourceGroupName("rg1")
* .shardCount(2)
* .sku(SkuArgs.builder()
* .capacity(1)
* .family("P")
* .name("Premium")
* .build())
* .staticIP("192.168.0.5")
* .subnetId("/subscriptions/subid/resourceGroups/rg2/providers/Microsoft.Network/virtualNetworks/network1/subnets/subnet1")
* .zones("1")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:cache:Redis cache1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Cache/redis/{name}
* ```
* @property enableNonSslPort Specifies whether the non-ssl Redis server port (6379) is enabled.
* @property identity The identity of the resource.
* @property location The geo-location where the resource lives
* @property minimumTlsVersion Optional: requires clients to use a specified TLS version (or higher) to connect (e,g, '1.0', '1.1', '1.2')
* @property name The name of the Redis cache.
* @property publicNetworkAccess Whether or not public endpoint access is allowed for this cache. Value is optional, but if passed in, must be 'Enabled' or 'Disabled'. If 'Disabled', private endpoints are the exclusive access method. Default value is 'Enabled'. Note: This setting is important for caches with private endpoints. It has *no effect* on caches that are joined to, or injected into, a virtual network subnet.
* @property redisConfiguration All Redis Settings. Few possible keys: rdb-backup-enabled,rdb-storage-connection-string,rdb-backup-frequency,maxmemory-delta,maxmemory-policy,notify-keyspace-events,maxmemory-samples,slowlog-log-slower-than,slowlog-max-len,list-max-ziplist-entries,list-max-ziplist-value,hash-max-ziplist-entries,hash-max-ziplist-value,set-max-intset-entries,zset-max-ziplist-entries,zset-max-ziplist-value etc.
* @property redisVersion Redis version. This should be in the form 'major[.minor]' (only 'major' is required) or the value 'latest' which refers to the latest stable Redis version that is available. Supported versions: 4.0, 6.0 (latest). Default value is 'latest'.
* @property replicasPerMaster The number of replicas to be created per primary.
* @property replicasPerPrimary The number of replicas to be created per primary.
* @property resourceGroupName The name of the resource group.
* @property shardCount The number of shards to be created on a Premium Cluster Cache.
* @property sku The SKU of the Redis cache to deploy.
* @property staticIP Static IP address. Optionally, may be specified when deploying a Redis cache inside an existing Azure Virtual Network; auto assigned by default.
* @property subnetId The full resource ID of a subnet in a virtual network to deploy the Redis cache in. Example format: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/Microsoft.{Network|ClassicNetwork}/VirtualNetworks/vnet1/subnets/subnet1
* @property tags Resource tags.
* @property tenantSettings A dictionary of tenant settings
* @property zones A list of availability zones denoting where the resource needs to come from.
*/
public data class RedisArgs(
public val enableNonSslPort: Output? = null,
public val identity: Output? = null,
public val location: Output? = null,
public val minimumTlsVersion: Output>? = null,
public val name: Output? = null,
public val publicNetworkAccess: Output>? = null,
public val redisConfiguration: Output? = null,
public val redisVersion: Output? = null,
public val replicasPerMaster: Output? = null,
public val replicasPerPrimary: Output? = null,
public val resourceGroupName: Output? = null,
public val shardCount: Output? = null,
public val sku: Output? = null,
public val staticIP: Output? = null,
public val subnetId: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy