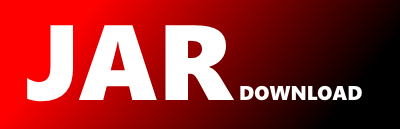
com.pulumi.azurenative.cache.kotlin.outputs.GetRedisResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cache.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A single Redis item in List or Get Operation.
* @property accessKeys The keys of the Redis cache - not set if this object is not the response to Create or Update redis cache
* @property enableNonSslPort Specifies whether the non-ssl Redis server port (6379) is enabled.
* @property hostName Redis host name.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property identity The identity of the resource.
* @property instances List of the Redis instances associated with the cache
* @property linkedServers List of the linked servers associated with the cache
* @property location The geo-location where the resource lives
* @property minimumTlsVersion Optional: requires clients to use a specified TLS version (or higher) to connect (e,g, '1.0', '1.1', '1.2')
* @property name The name of the resource
* @property port Redis non-SSL port.
* @property privateEndpointConnections List of private endpoint connection associated with the specified redis cache
* @property provisioningState Redis instance provisioning status.
* @property publicNetworkAccess Whether or not public endpoint access is allowed for this cache. Value is optional, but if passed in, must be 'Enabled' or 'Disabled'. If 'Disabled', private endpoints are the exclusive access method. Default value is 'Enabled'. Note: This setting is important for caches with private endpoints. It has *no effect* on caches that are joined to, or injected into, a virtual network subnet.
* @property redisConfiguration All Redis Settings. Few possible keys: rdb-backup-enabled,rdb-storage-connection-string,rdb-backup-frequency,maxmemory-delta,maxmemory-policy,notify-keyspace-events,maxmemory-samples,slowlog-log-slower-than,slowlog-max-len,list-max-ziplist-entries,list-max-ziplist-value,hash-max-ziplist-entries,hash-max-ziplist-value,set-max-intset-entries,zset-max-ziplist-entries,zset-max-ziplist-value etc.
* @property redisVersion Redis version. This should be in the form 'major[.minor]' (only 'major' is required) or the value 'latest' which refers to the latest stable Redis version that is available. Supported versions: 4.0, 6.0 (latest). Default value is 'latest'.
* @property replicasPerMaster The number of replicas to be created per primary.
* @property replicasPerPrimary The number of replicas to be created per primary.
* @property shardCount The number of shards to be created on a Premium Cluster Cache.
* @property sku The SKU of the Redis cache to deploy.
* @property sslPort Redis SSL port.
* @property staticIP Static IP address. Optionally, may be specified when deploying a Redis cache inside an existing Azure Virtual Network; auto assigned by default.
* @property subnetId The full resource ID of a subnet in a virtual network to deploy the Redis cache in. Example format: /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/Microsoft.{Network|ClassicNetwork}/VirtualNetworks/vnet1/subnets/subnet1
* @property tags Resource tags.
* @property tenantSettings A dictionary of tenant settings
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
* @property zones A list of availability zones denoting where the resource needs to come from.
*/
public data class GetRedisResult(
public val accessKeys: RedisAccessKeysResponse,
public val enableNonSslPort: Boolean? = null,
public val hostName: String,
public val id: String,
public val identity: ManagedServiceIdentityResponse? = null,
public val instances: List,
public val linkedServers: List,
public val location: String,
public val minimumTlsVersion: String? = null,
public val name: String,
public val port: Int,
public val privateEndpointConnections: List,
public val provisioningState: String,
public val publicNetworkAccess: String? = null,
public val redisConfiguration: RedisCommonPropertiesResponseRedisConfiguration? = null,
public val redisVersion: String? = null,
public val replicasPerMaster: Int? = null,
public val replicasPerPrimary: Int? = null,
public val shardCount: Int? = null,
public val sku: SkuResponse,
public val sslPort: Int,
public val staticIP: String? = null,
public val subnetId: String? = null,
public val tags: Map? = null,
public val tenantSettings: Map? = null,
public val type: String,
public val zones: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.cache.outputs.GetRedisResult): GetRedisResult = GetRedisResult(
accessKeys = javaType.accessKeys().let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.RedisAccessKeysResponse.Companion.toKotlin(args0)
}),
enableNonSslPort = javaType.enableNonSslPort().map({ args0 -> args0 }).orElse(null),
hostName = javaType.hostName(),
id = javaType.id(),
identity = javaType.identity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.ManagedServiceIdentityResponse.Companion.toKotlin(args0)
})
}).orElse(null),
instances = javaType.instances().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.RedisInstanceDetailsResponse.Companion.toKotlin(args0)
})
}),
linkedServers = javaType.linkedServers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.RedisLinkedServerResponse.Companion.toKotlin(args0)
})
}),
location = javaType.location(),
minimumTlsVersion = javaType.minimumTlsVersion().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
port = javaType.port(),
privateEndpointConnections = javaType.privateEndpointConnections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.PrivateEndpointConnectionResponse.Companion.toKotlin(args0)
})
}),
provisioningState = javaType.provisioningState(),
publicNetworkAccess = javaType.publicNetworkAccess().map({ args0 -> args0 }).orElse(null),
redisConfiguration = javaType.redisConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.RedisCommonPropertiesResponseRedisConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
redisVersion = javaType.redisVersion().map({ args0 -> args0 }).orElse(null),
replicasPerMaster = javaType.replicasPerMaster().map({ args0 -> args0 }).orElse(null),
replicasPerPrimary = javaType.replicasPerPrimary().map({ args0 -> args0 }).orElse(null),
shardCount = javaType.shardCount().map({ args0 -> args0 }).orElse(null),
sku = javaType.sku().let({ args0 ->
com.pulumi.azurenative.cache.kotlin.outputs.SkuResponse.Companion.toKotlin(args0)
}),
sslPort = javaType.sslPort(),
staticIP = javaType.staticIP().map({ args0 -> args0 }).orElse(null),
subnetId = javaType.subnetId().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
tenantSettings = javaType.tenantSettings().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
zones = javaType.zones().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy