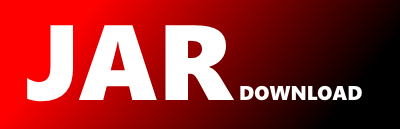
com.pulumi.azurenative.cdn.kotlin.AFDOrigin.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cdn.kotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.ResourceReferenceResponse
import com.pulumi.azurenative.cdn.kotlin.outputs.SharedPrivateLinkResourcePropertiesResponse
import com.pulumi.azurenative.cdn.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.cdn.kotlin.outputs.ResourceReferenceResponse.Companion.toKotlin as resourceReferenceResponseToKotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.SharedPrivateLinkResourcePropertiesResponse.Companion.toKotlin as sharedPrivateLinkResourcePropertiesResponseToKotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [AFDOrigin].
*/
@PulumiTagMarker
public class AFDOriginResourceBuilder internal constructor() {
public var name: String? = null
public var args: AFDOriginArgs = AFDOriginArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AFDOriginArgsBuilder.() -> Unit) {
val builder = AFDOriginArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AFDOrigin {
val builtJavaResource = com.pulumi.azurenative.cdn.AFDOrigin(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AFDOrigin(builtJavaResource)
}
}
/**
* Azure Front Door origin is the source of the content being delivered via Azure Front Door. When the edge nodes represented by an endpoint do not have the requested content cached, they attempt to fetch it from one or more of the configured origins.
* Azure REST API version: 2023-05-01. Prior API version in Azure Native 1.x: 2020-09-01.
* Other available API versions: 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview.
* ## Example Usage
* ### AFDOrigins_Create
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var afdOrigin = new AzureNative.Cdn.AFDOrigin("afdOrigin", new()
* {
* EnabledState = AzureNative.Cdn.EnabledState.Enabled,
* HostName = "host1.blob.core.windows.net",
* HttpPort = 80,
* HttpsPort = 443,
* OriginGroupName = "origingroup1",
* OriginHostHeader = "host1.foo.com",
* OriginName = "origin1",
* ProfileName = "profile1",
* ResourceGroupName = "RG",
* });
* });
* ```
* ```go
* package main
* import (
* cdn "github.com/pulumi/pulumi-azure-native-sdk/cdn/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cdn.NewAFDOrigin(ctx, "afdOrigin", &cdn.AFDOriginArgs{
* EnabledState: pulumi.String(cdn.EnabledStateEnabled),
* HostName: pulumi.String("host1.blob.core.windows.net"),
* HttpPort: pulumi.Int(80),
* HttpsPort: pulumi.Int(443),
* OriginGroupName: pulumi.String("origingroup1"),
* OriginHostHeader: pulumi.String("host1.foo.com"),
* OriginName: pulumi.String("origin1"),
* ProfileName: pulumi.String("profile1"),
* ResourceGroupName: pulumi.String("RG"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cdn.AFDOrigin;
* import com.pulumi.azurenative.cdn.AFDOriginArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var afdOrigin = new AFDOrigin("afdOrigin", AFDOriginArgs.builder()
* .enabledState("Enabled")
* .hostName("host1.blob.core.windows.net")
* .httpPort(80)
* .httpsPort(443)
* .originGroupName("origingroup1")
* .originHostHeader("host1.foo.com")
* .originName("origin1")
* .profileName("profile1")
* .resourceGroupName("RG")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:cdn:AFDOrigin origin1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Cdn/profiles/{profileName}/originGroups/{originGroupName}/origins/{originName}
* ```
*/
public class AFDOrigin internal constructor(
override val javaResource: com.pulumi.azurenative.cdn.AFDOrigin,
) : KotlinCustomResource(javaResource, AFDOriginMapper) {
/**
* Resource reference to the Azure origin resource.
*/
public val azureOrigin: Output?
get() = javaResource.azureOrigin().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
resourceReferenceResponseToKotlin(args0)
})
}).orElse(null)
})
public val deploymentStatus: Output
get() = javaResource.deploymentStatus().applyValue({ args0 -> args0 })
/**
* Whether to enable health probes to be made against backends defined under backendPools. Health probes can only be disabled if there is a single enabled backend in single enabled backend pool.
*/
public val enabledState: Output?
get() = javaResource.enabledState().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether to enable certificate name check at origin level
*/
public val enforceCertificateNameCheck: Output?
get() = javaResource.enforceCertificateNameCheck().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The address of the origin. Domain names, IPv4 addresses, and IPv6 addresses are supported.This should be unique across all origins in an endpoint.
*/
public val hostName: Output
get() = javaResource.hostName().applyValue({ args0 -> args0 })
/**
* The value of the HTTP port. Must be between 1 and 65535.
*/
public val httpPort: Output?
get() = javaResource.httpPort().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The value of the HTTPS port. Must be between 1 and 65535.
*/
public val httpsPort: Output?
get() = javaResource.httpsPort().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The name of the origin group which contains this origin.
*/
public val originGroupName: Output
get() = javaResource.originGroupName().applyValue({ args0 -> args0 })
/**
* The host header value sent to the origin with each request. If you leave this blank, the request hostname determines this value. Azure Front Door origins, such as Web Apps, Blob Storage, and Cloud Services require this host header value to match the origin hostname by default. This overrides the host header defined at Endpoint
*/
public val originHostHeader: Output?
get() = javaResource.originHostHeader().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Priority of origin in given origin group for load balancing. Higher priorities will not be used for load balancing if any lower priority origin is healthy.Must be between 1 and 5
*/
public val priority: Output?
get() = javaResource.priority().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Provisioning status
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* The properties of the private link resource for private origin.
*/
public val sharedPrivateLinkResource: Output?
get() = javaResource.sharedPrivateLinkResource().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
sharedPrivateLinkResourcePropertiesResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Read only system data
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* Resource type.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* Weight of the origin in given origin group for load balancing. Must be between 1 and 1000
*/
public val weight: Output?
get() = javaResource.weight().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object AFDOriginMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.cdn.AFDOrigin::class == javaResource::class
override fun map(javaResource: Resource): AFDOrigin = AFDOrigin(
javaResource as
com.pulumi.azurenative.cdn.AFDOrigin,
)
}
/**
* @see [AFDOrigin].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AFDOrigin].
*/
public suspend fun afdOrigin(name: String, block: suspend AFDOriginResourceBuilder.() -> Unit): AFDOrigin {
val builder = AFDOriginResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AFDOrigin].
* @param name The _unique_ name of the resulting resource.
*/
public fun afdOrigin(name: String): AFDOrigin {
val builder = AFDOriginResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy