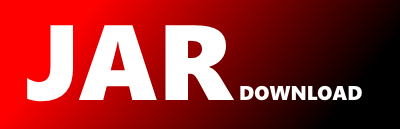
com.pulumi.azurenative.cdn.kotlin.Route.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cdn.kotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.ActivatedResourceReferenceResponse
import com.pulumi.azurenative.cdn.kotlin.outputs.AfdRouteCacheConfigurationResponse
import com.pulumi.azurenative.cdn.kotlin.outputs.ResourceReferenceResponse
import com.pulumi.azurenative.cdn.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.azurenative.cdn.kotlin.outputs.ActivatedResourceReferenceResponse.Companion.toKotlin as activatedResourceReferenceResponseToKotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.AfdRouteCacheConfigurationResponse.Companion.toKotlin as afdRouteCacheConfigurationResponseToKotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.ResourceReferenceResponse.Companion.toKotlin as resourceReferenceResponseToKotlin
import com.pulumi.azurenative.cdn.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [Route].
*/
@PulumiTagMarker
public class RouteResourceBuilder internal constructor() {
public var name: String? = null
public var args: RouteArgs = RouteArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RouteArgsBuilder.() -> Unit) {
val builder = RouteArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Route {
val builtJavaResource = com.pulumi.azurenative.cdn.Route(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Route(builtJavaResource)
}
}
/**
* Friendly Routes name mapping to the any Routes or secret related information.
* Azure REST API version: 2023-05-01. Prior API version in Azure Native 1.x: 2020-09-01.
* Other available API versions: 2020-09-01, 2023-07-01-preview, 2024-02-01, 2024-05-01-preview, 2024-06-01-preview.
* ## Example Usage
* ### Routes_Create
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var route = new AzureNative.Cdn.Route("route", new()
* {
* CacheConfiguration = new AzureNative.Cdn.Inputs.AfdRouteCacheConfigurationArgs
* {
* CompressionSettings = new AzureNative.Cdn.Inputs.CompressionSettingsArgs
* {
* ContentTypesToCompress = new[]
* {
* "text/html",
* "application/octet-stream",
* },
* IsCompressionEnabled = true,
* },
* QueryParameters = "querystring=test",
* QueryStringCachingBehavior = AzureNative.Cdn.AfdQueryStringCachingBehavior.IgnoreSpecifiedQueryStrings,
* },
* CustomDomains = new[]
* {
* new AzureNative.Cdn.Inputs.ActivatedResourceReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/customDomains/domain1",
* },
* },
* EnabledState = AzureNative.Cdn.EnabledState.Enabled,
* EndpointName = "endpoint1",
* ForwardingProtocol = AzureNative.Cdn.ForwardingProtocol.MatchRequest,
* HttpsRedirect = AzureNative.Cdn.HttpsRedirect.Enabled,
* LinkToDefaultDomain = AzureNative.Cdn.LinkToDefaultDomain.Enabled,
* OriginGroup = new AzureNative.Cdn.Inputs.ResourceReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/originGroups/originGroup1",
* },
* PatternsToMatch = new[]
* {
* "/*",
* },
* ProfileName = "profile1",
* ResourceGroupName = "RG",
* RouteName = "route1",
* RuleSets = new[]
* {
* new AzureNative.Cdn.Inputs.ResourceReferenceArgs
* {
* Id = "/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/ruleSets/ruleSet1",
* },
* },
* SupportedProtocols = new[]
* {
* AzureNative.Cdn.AFDEndpointProtocols.Https,
* AzureNative.Cdn.AFDEndpointProtocols.Http,
* },
* });
* });
* ```
* ```go
* package main
* import (
* cdn "github.com/pulumi/pulumi-azure-native-sdk/cdn/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cdn.NewRoute(ctx, "route", &cdn.RouteArgs{
* CacheConfiguration: &cdn.AfdRouteCacheConfigurationArgs{
* CompressionSettings: &cdn.CompressionSettingsArgs{
* ContentTypesToCompress: pulumi.StringArray{
* pulumi.String("text/html"),
* pulumi.String("application/octet-stream"),
* },
* IsCompressionEnabled: pulumi.Bool(true),
* },
* QueryParameters: pulumi.String("querystring=test"),
* QueryStringCachingBehavior: pulumi.String(cdn.AfdQueryStringCachingBehaviorIgnoreSpecifiedQueryStrings),
* },
* CustomDomains: cdn.ActivatedResourceReferenceArray{
* &cdn.ActivatedResourceReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/customDomains/domain1"),
* },
* },
* EnabledState: pulumi.String(cdn.EnabledStateEnabled),
* EndpointName: pulumi.String("endpoint1"),
* ForwardingProtocol: pulumi.String(cdn.ForwardingProtocolMatchRequest),
* HttpsRedirect: pulumi.String(cdn.HttpsRedirectEnabled),
* LinkToDefaultDomain: pulumi.String(cdn.LinkToDefaultDomainEnabled),
* OriginGroup: &cdn.ResourceReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/originGroups/originGroup1"),
* },
* PatternsToMatch: pulumi.StringArray{
* pulumi.String("/*"),
* },
* ProfileName: pulumi.String("profile1"),
* ResourceGroupName: pulumi.String("RG"),
* RouteName: pulumi.String("route1"),
* RuleSets: cdn.ResourceReferenceArray{
* &cdn.ResourceReferenceArgs{
* Id: pulumi.String("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/ruleSets/ruleSet1"),
* },
* },
* SupportedProtocols: pulumi.StringArray{
* pulumi.String(cdn.AFDEndpointProtocolsHttps),
* pulumi.String(cdn.AFDEndpointProtocolsHttp),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.cdn.Route;
* import com.pulumi.azurenative.cdn.RouteArgs;
* import com.pulumi.azurenative.cdn.inputs.AfdRouteCacheConfigurationArgs;
* import com.pulumi.azurenative.cdn.inputs.CompressionSettingsArgs;
* import com.pulumi.azurenative.cdn.inputs.ActivatedResourceReferenceArgs;
* import com.pulumi.azurenative.cdn.inputs.ResourceReferenceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var route = new Route("route", RouteArgs.builder()
* .cacheConfiguration(AfdRouteCacheConfigurationArgs.builder()
* .compressionSettings(CompressionSettingsArgs.builder()
* .contentTypesToCompress(
* "text/html",
* "application/octet-stream")
* .isCompressionEnabled(true)
* .build())
* .queryParameters("querystring=test")
* .queryStringCachingBehavior("IgnoreSpecifiedQueryStrings")
* .build())
* .customDomains(ActivatedResourceReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/customDomains/domain1")
* .build())
* .enabledState("Enabled")
* .endpointName("endpoint1")
* .forwardingProtocol("MatchRequest")
* .httpsRedirect("Enabled")
* .linkToDefaultDomain("Enabled")
* .originGroup(ResourceReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/originGroups/originGroup1")
* .build())
* .patternsToMatch("/*")
* .profileName("profile1")
* .resourceGroupName("RG")
* .routeName("route1")
* .ruleSets(ResourceReferenceArgs.builder()
* .id("/subscriptions/subid/resourceGroups/RG/providers/Microsoft.Cdn/profiles/profile1/ruleSets/ruleSet1")
* .build())
* .supportedProtocols(
* "Https",
* "Http")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:cdn:Route route1 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Cdn/profiles/{profileName}/afdEndpoints/{endpointName}/routes/{routeName}
* ```
* */*/*/
*/
public class Route internal constructor(
override val javaResource: com.pulumi.azurenative.cdn.Route,
) : KotlinCustomResource(javaResource, RouteMapper) {
/**
* The caching configuration for this route. To disable caching, do not provide a cacheConfiguration object.
*/
public val cacheConfiguration: Output?
get() = javaResource.cacheConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> afdRouteCacheConfigurationResponseToKotlin(args0) })
}).orElse(null)
})
/**
* Domains referenced by this endpoint.
*/
public val customDomains: Output>?
get() = javaResource.customDomains().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
activatedResourceReferenceResponseToKotlin(args0)
})
})
}).orElse(null)
})
public val deploymentStatus: Output
get() = javaResource.deploymentStatus().applyValue({ args0 -> args0 })
/**
* Whether to enable use of this rule. Permitted values are 'Enabled' or 'Disabled'
*/
public val enabledState: Output?
get() = javaResource.enabledState().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the endpoint which holds the route.
*/
public val endpointName: Output
get() = javaResource.endpointName().applyValue({ args0 -> args0 })
/**
* Protocol this rule will use when forwarding traffic to backends.
*/
public val forwardingProtocol: Output?
get() = javaResource.forwardingProtocol().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Whether to automatically redirect HTTP traffic to HTTPS traffic. Note that this is a easy way to set up this rule and it will be the first rule that gets executed.
*/
public val httpsRedirect: Output?
get() = javaResource.httpsRedirect().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* whether this route will be linked to the default endpoint domain.
*/
public val linkToDefaultDomain: Output?
get() = javaResource.linkToDefaultDomain().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* A reference to the origin group.
*/
public val originGroup: Output
get() = javaResource.originGroup().applyValue({ args0 ->
args0.let({ args0 ->
resourceReferenceResponseToKotlin(args0)
})
})
/**
* A directory path on the origin that AzureFrontDoor can use to retrieve content from, e.g. contoso.cloudapp.net/originpath.
*/
public val originPath: Output?
get() = javaResource.originPath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The route patterns of the rule.
*/
public val patternsToMatch: Output>?
get() = javaResource.patternsToMatch().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Provisioning status
*/
public val provisioningState: Output
get() = javaResource.provisioningState().applyValue({ args0 -> args0 })
/**
* rule sets referenced by this endpoint.
*/
public val ruleSets: Output>?
get() = javaResource.ruleSets().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> resourceReferenceResponseToKotlin(args0) })
})
}).orElse(null)
})
/**
* List of supported protocols for this route.
*/
public val supportedProtocols: Output>?
get() = javaResource.supportedProtocols().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Read only system data
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* Resource type.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object RouteMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.cdn.Route::class == javaResource::class
override fun map(javaResource: Resource): Route = Route(
javaResource as
com.pulumi.azurenative.cdn.Route,
)
}
/**
* @see [Route].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Route].
*/
public suspend fun route(name: String, block: suspend RouteResourceBuilder.() -> Unit): Route {
val builder = RouteResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Route].
* @param name The _unique_ name of the resulting resource.
*/
public fun route(name: String): Route {
val builder = RouteResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy