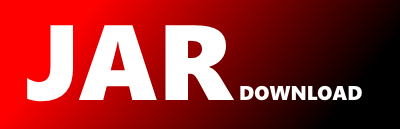
com.pulumi.azurenative.cdn.kotlin.inputs.CacheConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cdn.kotlin.inputs
import com.pulumi.azurenative.cdn.inputs.CacheConfigurationArgs.builder
import com.pulumi.azurenative.cdn.kotlin.enums.RuleCacheBehavior
import com.pulumi.azurenative.cdn.kotlin.enums.RuleIsCompressionEnabled
import com.pulumi.azurenative.cdn.kotlin.enums.RuleQueryStringCachingBehavior
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Caching settings for a caching-type route. To disable caching, do not provide a cacheConfiguration object.
* @property cacheBehavior Caching behavior for the requests
* @property cacheDuration The duration for which the content needs to be cached. Allowed format is [d.]hh:mm:ss
* @property isCompressionEnabled Indicates whether content compression is enabled. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on AzureFrontDoor when requested content is smaller than 1 byte or larger than 1 MB.
* @property queryParameters query parameters to include or exclude (comma separated).
* @property queryStringCachingBehavior Defines how Frontdoor caches requests that include query strings. You can ignore any query strings when caching, ignore specific query strings, cache every request with a unique URL, or cache specific query strings.
*/
public data class CacheConfigurationArgs(
public val cacheBehavior: Output>? = null,
public val cacheDuration: Output? = null,
public val isCompressionEnabled: Output>? = null,
public val queryParameters: Output? = null,
public val queryStringCachingBehavior: Output>? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.cdn.inputs.CacheConfigurationArgs =
com.pulumi.azurenative.cdn.inputs.CacheConfigurationArgs.builder()
.cacheBehavior(
cacheBehavior?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.cacheDuration(cacheDuration?.applyValue({ args0 -> args0 }))
.isCompressionEnabled(
isCompressionEnabled?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.queryParameters(queryParameters?.applyValue({ args0 -> args0 }))
.queryStringCachingBehavior(
queryStringCachingBehavior?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 -> args0.let({ args0 -> args0.toJava() }) })
}),
).build()
}
/**
* Builder for [CacheConfigurationArgs].
*/
@PulumiTagMarker
public class CacheConfigurationArgsBuilder internal constructor() {
private var cacheBehavior: Output>? = null
private var cacheDuration: Output? = null
private var isCompressionEnabled: Output>? = null
private var queryParameters: Output? = null
private var queryStringCachingBehavior: Output>? =
null
/**
* @param value Caching behavior for the requests
*/
@JvmName("sftgbaguibkumpcs")
public suspend fun cacheBehavior(`value`: Output>) {
this.cacheBehavior = value
}
/**
* @param value The duration for which the content needs to be cached. Allowed format is [d.]hh:mm:ss
*/
@JvmName("pxiuituuhgvqhqbc")
public suspend fun cacheDuration(`value`: Output) {
this.cacheDuration = value
}
/**
* @param value Indicates whether content compression is enabled. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on AzureFrontDoor when requested content is smaller than 1 byte or larger than 1 MB.
*/
@JvmName("wdammlhjexqcfesr")
public suspend fun isCompressionEnabled(`value`: Output>) {
this.isCompressionEnabled = value
}
/**
* @param value query parameters to include or exclude (comma separated).
*/
@JvmName("sgepbawvatebuieb")
public suspend fun queryParameters(`value`: Output) {
this.queryParameters = value
}
/**
* @param value Defines how Frontdoor caches requests that include query strings. You can ignore any query strings when caching, ignore specific query strings, cache every request with a unique URL, or cache specific query strings.
*/
@JvmName("blutytlrhgjqshys")
public suspend fun queryStringCachingBehavior(`value`: Output>) {
this.queryStringCachingBehavior = value
}
/**
* @param value Caching behavior for the requests
*/
@JvmName("njelxeocreukqbbw")
public suspend fun cacheBehavior(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cacheBehavior = mapped
}
/**
* @param value Caching behavior for the requests
*/
@JvmName("apqxsyepdtarqljm")
public fun cacheBehavior(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.cacheBehavior = mapped
}
/**
* @param value Caching behavior for the requests
*/
@JvmName("pltefoakrpvhigha")
public fun cacheBehavior(`value`: RuleCacheBehavior) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.cacheBehavior = mapped
}
/**
* @param value The duration for which the content needs to be cached. Allowed format is [d.]hh:mm:ss
*/
@JvmName("lkbwncuimvmekqeg")
public suspend fun cacheDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cacheDuration = mapped
}
/**
* @param value Indicates whether content compression is enabled. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on AzureFrontDoor when requested content is smaller than 1 byte or larger than 1 MB.
*/
@JvmName("mcbyykdlgogdsllo")
public suspend fun isCompressionEnabled(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.isCompressionEnabled = mapped
}
/**
* @param value Indicates whether content compression is enabled. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on AzureFrontDoor when requested content is smaller than 1 byte or larger than 1 MB.
*/
@JvmName("eigqrxqsjxcpofyf")
public fun isCompressionEnabled(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.isCompressionEnabled = mapped
}
/**
* @param value Indicates whether content compression is enabled. If compression is enabled, content will be served as compressed if user requests for a compressed version. Content won't be compressed on AzureFrontDoor when requested content is smaller than 1 byte or larger than 1 MB.
*/
@JvmName("oxmoopvcwdrmmccb")
public fun isCompressionEnabled(`value`: RuleIsCompressionEnabled) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.isCompressionEnabled = mapped
}
/**
* @param value query parameters to include or exclude (comma separated).
*/
@JvmName("vpcfaqaueygofnfr")
public suspend fun queryParameters(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryParameters = mapped
}
/**
* @param value Defines how Frontdoor caches requests that include query strings. You can ignore any query strings when caching, ignore specific query strings, cache every request with a unique URL, or cache specific query strings.
*/
@JvmName("qckhiodbdditqira")
public suspend fun queryStringCachingBehavior(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryStringCachingBehavior = mapped
}
/**
* @param value Defines how Frontdoor caches requests that include query strings. You can ignore any query strings when caching, ignore specific query strings, cache every request with a unique URL, or cache specific query strings.
*/
@JvmName("pjosxkxrxbdjugoi")
public fun queryStringCachingBehavior(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.queryStringCachingBehavior = mapped
}
/**
* @param value Defines how Frontdoor caches requests that include query strings. You can ignore any query strings when caching, ignore specific query strings, cache every request with a unique URL, or cache specific query strings.
*/
@JvmName("euupyrnfkrruobff")
public fun queryStringCachingBehavior(`value`: RuleQueryStringCachingBehavior) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.queryStringCachingBehavior = mapped
}
internal fun build(): CacheConfigurationArgs = CacheConfigurationArgs(
cacheBehavior = cacheBehavior,
cacheDuration = cacheDuration,
isCompressionEnabled = isCompressionEnabled,
queryParameters = queryParameters,
queryStringCachingBehavior = queryStringCachingBehavior,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy