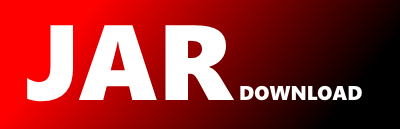
com.pulumi.azurenative.cognitiveservices.kotlin.inputs.AccountPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.cognitiveservices.kotlin.inputs
import com.pulumi.azurenative.cognitiveservices.inputs.AccountPropertiesArgs.builder
import com.pulumi.azurenative.cognitiveservices.kotlin.enums.PublicNetworkAccess
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Properties of Cognitive Services account.
* @property allowedFqdnList
* @property apiProperties The api properties for special APIs.
* @property customSubDomainName Optional subdomain name used for token-based authentication.
* @property disableLocalAuth
* @property dynamicThrottlingEnabled The flag to enable dynamic throttling.
* @property encryption The encryption properties for this resource.
* @property locations The multiregion settings of Cognitive Services account.
* @property migrationToken Resource migration token.
* @property networkAcls A collection of rules governing the accessibility from specific network locations.
* @property publicNetworkAccess Whether or not public endpoint access is allowed for this account.
* @property restore
* @property restrictOutboundNetworkAccess
* @property userOwnedStorage The storage accounts for this resource.
*/
public data class AccountPropertiesArgs(
public val allowedFqdnList: Output>? = null,
public val apiProperties: Output? = null,
public val customSubDomainName: Output? = null,
public val disableLocalAuth: Output? = null,
public val dynamicThrottlingEnabled: Output? = null,
public val encryption: Output? = null,
public val locations: Output? = null,
public val migrationToken: Output? = null,
public val networkAcls: Output? = null,
public val publicNetworkAccess: Output>? = null,
public val restore: Output? = null,
public val restrictOutboundNetworkAccess: Output? = null,
public val userOwnedStorage: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.cognitiveservices.inputs.AccountPropertiesArgs =
com.pulumi.azurenative.cognitiveservices.inputs.AccountPropertiesArgs.builder()
.allowedFqdnList(allowedFqdnList?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.apiProperties(apiProperties?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.customSubDomainName(customSubDomainName?.applyValue({ args0 -> args0 }))
.disableLocalAuth(disableLocalAuth?.applyValue({ args0 -> args0 }))
.dynamicThrottlingEnabled(dynamicThrottlingEnabled?.applyValue({ args0 -> args0 }))
.encryption(encryption?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.locations(locations?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.migrationToken(migrationToken?.applyValue({ args0 -> args0 }))
.networkAcls(networkAcls?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.publicNetworkAccess(
publicNetworkAccess?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
)
.restore(restore?.applyValue({ args0 -> args0 }))
.restrictOutboundNetworkAccess(restrictOutboundNetworkAccess?.applyValue({ args0 -> args0 }))
.userOwnedStorage(
userOwnedStorage?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [AccountPropertiesArgs].
*/
@PulumiTagMarker
public class AccountPropertiesArgsBuilder internal constructor() {
private var allowedFqdnList: Output>? = null
private var apiProperties: Output? = null
private var customSubDomainName: Output? = null
private var disableLocalAuth: Output? = null
private var dynamicThrottlingEnabled: Output? = null
private var encryption: Output? = null
private var locations: Output? = null
private var migrationToken: Output? = null
private var networkAcls: Output? = null
private var publicNetworkAccess: Output>? = null
private var restore: Output? = null
private var restrictOutboundNetworkAccess: Output? = null
private var userOwnedStorage: Output>? = null
/**
* @param value
*/
@JvmName("yfrbehatupfiksda")
public suspend fun allowedFqdnList(`value`: Output>) {
this.allowedFqdnList = value
}
@JvmName("ukgnndhxdieghasn")
public suspend fun allowedFqdnList(vararg values: Output) {
this.allowedFqdnList = Output.all(values.asList())
}
/**
* @param values
*/
@JvmName("ugpoirsowdyixpui")
public suspend fun allowedFqdnList(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy