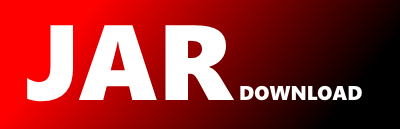
com.pulumi.azurenative.compute.kotlin.DiskAccessArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin
import com.pulumi.azurenative.compute.DiskAccessArgs.builder
import com.pulumi.azurenative.compute.kotlin.inputs.ExtendedLocationArgs
import com.pulumi.azurenative.compute.kotlin.inputs.ExtendedLocationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* disk access resource.
* Azure REST API version: 2022-07-02. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2023-01-02, 2023-04-02, 2023-10-02, 2024-03-02.
* ## Example Usage
* ### Create a disk access resource.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var diskAccess = new AzureNative.Compute.DiskAccess("diskAccess", new()
* {
* DiskAccessName = "myDiskAccess",
* Location = "West US",
* ResourceGroupName = "myResourceGroup",
* });
* });
* ```
* ```go
* package main
* import (
* compute "github.com/pulumi/pulumi-azure-native-sdk/compute/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewDiskAccess(ctx, "diskAccess", &compute.DiskAccessArgs{
* DiskAccessName: pulumi.String("myDiskAccess"),
* Location: pulumi.String("West US"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.DiskAccess;
* import com.pulumi.azurenative.compute.DiskAccessArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var diskAccess = new DiskAccess("diskAccess", DiskAccessArgs.builder()
* .diskAccessName("myDiskAccess")
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:compute:DiskAccess myDiskAccess /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/diskAccesses/{diskAccessName}
* ```
* @property diskAccessName The name of the disk access resource that is being created. The name can't be changed after the disk encryption set is created. Supported characters for the name are a-z, A-Z, 0-9, _ and -. The maximum name length is 80 characters.
* @property extendedLocation The extended location where the disk access will be created. Extended location cannot be changed.
* @property location Resource location
* @property resourceGroupName The name of the resource group.
* @property tags Resource tags
*/
public data class DiskAccessArgs(
public val diskAccessName: Output? = null,
public val extendedLocation: Output? = null,
public val location: Output? = null,
public val resourceGroupName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy