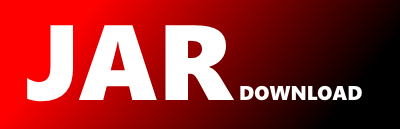
com.pulumi.azurenative.compute.kotlin.DiskEncryptionSetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin
import com.pulumi.azurenative.compute.DiskEncryptionSetArgs.builder
import com.pulumi.azurenative.compute.kotlin.enums.DiskEncryptionSetType
import com.pulumi.azurenative.compute.kotlin.inputs.EncryptionSetIdentityArgs
import com.pulumi.azurenative.compute.kotlin.inputs.EncryptionSetIdentityArgsBuilder
import com.pulumi.azurenative.compute.kotlin.inputs.KeyForDiskEncryptionSetArgs
import com.pulumi.azurenative.compute.kotlin.inputs.KeyForDiskEncryptionSetArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* disk encryption set resource.
* Azure REST API version: 2022-07-02. Prior API version in Azure Native 1.x: 2020-12-01.
* Other available API versions: 2020-06-30, 2023-01-02, 2023-04-02, 2023-10-02, 2024-03-02.
* ## Example Usage
* ### Create a disk encryption set with key vault from a different subscription.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var diskEncryptionSet = new AzureNative.Compute.DiskEncryptionSet("diskEncryptionSet", new()
* {
* ActiveKey = new AzureNative.Compute.Inputs.KeyForDiskEncryptionSetArgs
* {
* KeyUrl = "https://myvaultdifferentsub.vault-int.azure-int.net/keys/{key}",
* },
* DiskEncryptionSetName = "myDiskEncryptionSet",
* EncryptionType = AzureNative.Compute.DiskEncryptionSetType.EncryptionAtRestWithCustomerKey,
* Identity = new AzureNative.Compute.Inputs.EncryptionSetIdentityArgs
* {
* Type = AzureNative.Compute.DiskEncryptionSetIdentityType.SystemAssigned,
* },
* Location = "West US",
* ResourceGroupName = "myResourceGroup",
* });
* });
* ```
* ```go
* package main
* import (
* compute "github.com/pulumi/pulumi-azure-native-sdk/compute/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewDiskEncryptionSet(ctx, "diskEncryptionSet", &compute.DiskEncryptionSetArgs{
* ActiveKey: &compute.KeyForDiskEncryptionSetArgs{
* KeyUrl: pulumi.String("https://myvaultdifferentsub.vault-int.azure-int.net/keys/{key}"),
* },
* DiskEncryptionSetName: pulumi.String("myDiskEncryptionSet"),
* EncryptionType: pulumi.String(compute.DiskEncryptionSetTypeEncryptionAtRestWithCustomerKey),
* Identity: &compute.EncryptionSetIdentityArgs{
* Type: pulumi.String(compute.DiskEncryptionSetIdentityTypeSystemAssigned),
* },
* Location: pulumi.String("West US"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.DiskEncryptionSet;
* import com.pulumi.azurenative.compute.DiskEncryptionSetArgs;
* import com.pulumi.azurenative.compute.inputs.KeyForDiskEncryptionSetArgs;
* import com.pulumi.azurenative.compute.inputs.EncryptionSetIdentityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var diskEncryptionSet = new DiskEncryptionSet("diskEncryptionSet", DiskEncryptionSetArgs.builder()
* .activeKey(KeyForDiskEncryptionSetArgs.builder()
* .keyUrl("https://myvaultdifferentsub.vault-int.azure-int.net/keys/{key}")
* .build())
* .diskEncryptionSetName("myDiskEncryptionSet")
* .encryptionType("EncryptionAtRestWithCustomerKey")
* .identity(EncryptionSetIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .build());
* }
* }
* ```
* ### Create a disk encryption set.
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var diskEncryptionSet = new AzureNative.Compute.DiskEncryptionSet("diskEncryptionSet", new()
* {
* ActiveKey = new AzureNative.Compute.Inputs.KeyForDiskEncryptionSetArgs
* {
* KeyUrl = "https://myvmvault.vault-int.azure-int.net/keys/{key}",
* SourceVault = new AzureNative.Compute.Inputs.SourceVaultArgs
* {
* Id = "/subscriptions/{subscriptionId}/resourceGroups/myResourceGroup/providers/Microsoft.KeyVault/vaults/myVMVault",
* },
* },
* DiskEncryptionSetName = "myDiskEncryptionSet",
* EncryptionType = AzureNative.Compute.DiskEncryptionSetType.EncryptionAtRestWithCustomerKey,
* Identity = new AzureNative.Compute.Inputs.EncryptionSetIdentityArgs
* {
* Type = AzureNative.Compute.DiskEncryptionSetIdentityType.SystemAssigned,
* },
* Location = "West US",
* ResourceGroupName = "myResourceGroup",
* });
* });
* ```
* ```go
* package main
* import (
* compute "github.com/pulumi/pulumi-azure-native-sdk/compute/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewDiskEncryptionSet(ctx, "diskEncryptionSet", &compute.DiskEncryptionSetArgs{
* ActiveKey: &compute.KeyForDiskEncryptionSetArgs{
* KeyUrl: pulumi.String("https://myvmvault.vault-int.azure-int.net/keys/{key}"),
* SourceVault: &compute.SourceVaultArgs{
* Id: pulumi.String("/subscriptions/{subscriptionId}/resourceGroups/myResourceGroup/providers/Microsoft.KeyVault/vaults/myVMVault"),
* },
* },
* DiskEncryptionSetName: pulumi.String("myDiskEncryptionSet"),
* EncryptionType: pulumi.String(compute.DiskEncryptionSetTypeEncryptionAtRestWithCustomerKey),
* Identity: &compute.EncryptionSetIdentityArgs{
* Type: pulumi.String(compute.DiskEncryptionSetIdentityTypeSystemAssigned),
* },
* Location: pulumi.String("West US"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.compute.DiskEncryptionSet;
* import com.pulumi.azurenative.compute.DiskEncryptionSetArgs;
* import com.pulumi.azurenative.compute.inputs.KeyForDiskEncryptionSetArgs;
* import com.pulumi.azurenative.compute.inputs.SourceVaultArgs;
* import com.pulumi.azurenative.compute.inputs.EncryptionSetIdentityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var diskEncryptionSet = new DiskEncryptionSet("diskEncryptionSet", DiskEncryptionSetArgs.builder()
* .activeKey(KeyForDiskEncryptionSetArgs.builder()
* .keyUrl("https://myvmvault.vault-int.azure-int.net/keys/{key}")
* .sourceVault(SourceVaultArgs.builder()
* .id("/subscriptions/{subscriptionId}/resourceGroups/myResourceGroup/providers/Microsoft.KeyVault/vaults/myVMVault")
* .build())
* .build())
* .diskEncryptionSetName("myDiskEncryptionSet")
* .encryptionType("EncryptionAtRestWithCustomerKey")
* .identity(EncryptionSetIdentityArgs.builder()
* .type("SystemAssigned")
* .build())
* .location("West US")
* .resourceGroupName("myResourceGroup")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:compute:DiskEncryptionSet myDiskEncryptionSet /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Compute/diskEncryptionSets/{diskEncryptionSetName}
* ```
* @property activeKey The key vault key which is currently used by this disk encryption set.
* @property diskEncryptionSetName The name of the disk encryption set that is being created. The name can't be changed after the disk encryption set is created. Supported characters for the name are a-z, A-Z, 0-9, _ and -. The maximum name length is 80 characters.
* @property encryptionType The type of key used to encrypt the data of the disk.
* @property federatedClientId Multi-tenant application client id to access key vault in a different tenant. Setting the value to 'None' will clear the property.
* @property identity The managed identity for the disk encryption set. It should be given permission on the key vault before it can be used to encrypt disks.
* @property location Resource location
* @property resourceGroupName The name of the resource group.
* @property rotationToLatestKeyVersionEnabled Set this flag to true to enable auto-updating of this disk encryption set to the latest key version.
* @property tags Resource tags
*/
public data class DiskEncryptionSetArgs(
public val activeKey: Output? = null,
public val diskEncryptionSetName: Output? = null,
public val encryptionType: Output>? = null,
public val federatedClientId: Output? = null,
public val identity: Output? = null,
public val location: Output? = null,
public val resourceGroupName: Output? = null,
public val rotationToLatestKeyVersionEnabled: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy