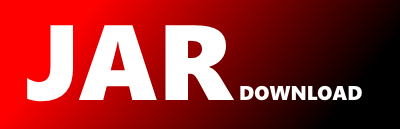
com.pulumi.azurenative.compute.kotlin.inputs.AdditionalUnattendContentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin.inputs
import com.pulumi.azurenative.compute.inputs.AdditionalUnattendContentArgs.builder
import com.pulumi.azurenative.compute.kotlin.enums.ComponentNames
import com.pulumi.azurenative.compute.kotlin.enums.PassNames
import com.pulumi.azurenative.compute.kotlin.enums.SettingNames
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Specifies additional XML formatted information that can be included in the Unattend.xml file, which is used by Windows Setup. Contents are defined by setting name, component name, and the pass in which the content is applied.
* @property componentName The component name. Currently, the only allowable value is Microsoft-Windows-Shell-Setup.
* @property content Specifies the XML formatted content that is added to the unattend.xml file for the specified path and component. The XML must be less than 4KB and must include the root element for the setting or feature that is being inserted.
* @property passName The pass name. Currently, the only allowable value is OobeSystem.
* @property settingName Specifies the name of the setting to which the content applies. Possible values are: FirstLogonCommands and AutoLogon.
*/
public data class AdditionalUnattendContentArgs(
public val componentName: Output? = null,
public val content: Output? = null,
public val passName: Output? = null,
public val settingName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.compute.inputs.AdditionalUnattendContentArgs =
com.pulumi.azurenative.compute.inputs.AdditionalUnattendContentArgs.builder()
.componentName(componentName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.content(content?.applyValue({ args0 -> args0 }))
.passName(passName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.settingName(settingName?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AdditionalUnattendContentArgs].
*/
@PulumiTagMarker
public class AdditionalUnattendContentArgsBuilder internal constructor() {
private var componentName: Output? = null
private var content: Output? = null
private var passName: Output? = null
private var settingName: Output? = null
/**
* @param value The component name. Currently, the only allowable value is Microsoft-Windows-Shell-Setup.
*/
@JvmName("fvkxhkkuuxtikoby")
public suspend fun componentName(`value`: Output) {
this.componentName = value
}
/**
* @param value Specifies the XML formatted content that is added to the unattend.xml file for the specified path and component. The XML must be less than 4KB and must include the root element for the setting or feature that is being inserted.
*/
@JvmName("dukjasmoedidltsp")
public suspend fun content(`value`: Output) {
this.content = value
}
/**
* @param value The pass name. Currently, the only allowable value is OobeSystem.
*/
@JvmName("ejbimfqcgwxixqdf")
public suspend fun passName(`value`: Output) {
this.passName = value
}
/**
* @param value Specifies the name of the setting to which the content applies. Possible values are: FirstLogonCommands and AutoLogon.
*/
@JvmName("bfykcdosnaylyems")
public suspend fun settingName(`value`: Output) {
this.settingName = value
}
/**
* @param value The component name. Currently, the only allowable value is Microsoft-Windows-Shell-Setup.
*/
@JvmName("wegnqqmoxaniglxa")
public suspend fun componentName(`value`: ComponentNames?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.componentName = mapped
}
/**
* @param value Specifies the XML formatted content that is added to the unattend.xml file for the specified path and component. The XML must be less than 4KB and must include the root element for the setting or feature that is being inserted.
*/
@JvmName("jjpuwvkjwbkivfdj")
public suspend fun content(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.content = mapped
}
/**
* @param value The pass name. Currently, the only allowable value is OobeSystem.
*/
@JvmName("ffecmfycqwmwgeao")
public suspend fun passName(`value`: PassNames?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.passName = mapped
}
/**
* @param value Specifies the name of the setting to which the content applies. Possible values are: FirstLogonCommands and AutoLogon.
*/
@JvmName("vbejsvhtlksoajsq")
public suspend fun settingName(`value`: SettingNames?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.settingName = mapped
}
internal fun build(): AdditionalUnattendContentArgs = AdditionalUnattendContentArgs(
componentName = componentName,
content = content,
passName = passName,
settingName = settingName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy