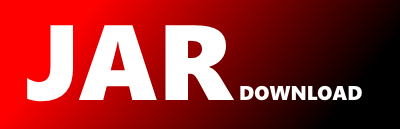
com.pulumi.azurenative.compute.kotlin.inputs.CloudServiceNetworkProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin.inputs
import com.pulumi.azurenative.compute.inputs.CloudServiceNetworkProfileArgs.builder
import com.pulumi.azurenative.compute.kotlin.enums.CloudServiceSlotType
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Network Profile for the cloud service.
* @property loadBalancerConfigurations List of Load balancer configurations. Cloud service can have up to two load balancer configurations, corresponding to a Public Load Balancer and an Internal Load Balancer.
* @property slotType Slot type for the cloud service.
* Possible values are
**Production**
**Staging**
* If not specified, the default value is Production.
* @property swappableCloudService The id reference of the cloud service containing the target IP with which the subject cloud service can perform a swap. This property cannot be updated once it is set. The swappable cloud service referred by this id must be present otherwise an error will be thrown.
*/
public data class CloudServiceNetworkProfileArgs(
public val loadBalancerConfigurations: Output>? = null,
public val slotType: Output>? = null,
public val swappableCloudService: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.compute.inputs.CloudServiceNetworkProfileArgs =
com.pulumi.azurenative.compute.inputs.CloudServiceNetworkProfileArgs.builder()
.loadBalancerConfigurations(
loadBalancerConfigurations?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.slotType(
slotType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.swappableCloudService(
swappableCloudService?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [CloudServiceNetworkProfileArgs].
*/
@PulumiTagMarker
public class CloudServiceNetworkProfileArgsBuilder internal constructor() {
private var loadBalancerConfigurations: Output>? = null
private var slotType: Output>? = null
private var swappableCloudService: Output? = null
/**
* @param value List of Load balancer configurations. Cloud service can have up to two load balancer configurations, corresponding to a Public Load Balancer and an Internal Load Balancer.
*/
@JvmName("uqrthxivhvbasfmy")
public suspend fun loadBalancerConfigurations(`value`: Output>) {
this.loadBalancerConfigurations = value
}
@JvmName("bmvusdefjyjvfdyo")
public suspend fun loadBalancerConfigurations(vararg values: Output) {
this.loadBalancerConfigurations = Output.all(values.asList())
}
/**
* @param values List of Load balancer configurations. Cloud service can have up to two load balancer configurations, corresponding to a Public Load Balancer and an Internal Load Balancer.
*/
@JvmName("nyvoasorlipjudma")
public suspend fun loadBalancerConfigurations(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy