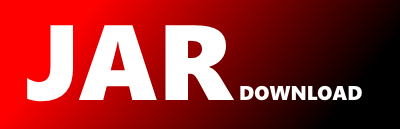
com.pulumi.azurenative.compute.kotlin.inputs.ImageDataDiskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin.inputs
import com.pulumi.azurenative.compute.inputs.ImageDataDiskArgs.builder
import com.pulumi.azurenative.compute.kotlin.enums.CachingTypes
import com.pulumi.azurenative.compute.kotlin.enums.StorageAccountTypes
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Describes a data disk.
* @property blobUri The Virtual Hard Disk.
* @property caching Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
* @property diskEncryptionSet Specifies the customer managed disk encryption set resource id for the managed image disk.
* @property diskSizeGB Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
* @property lun Specifies the logical unit number of the data disk. This value is used to identify data disks within the VM and therefore must be unique for each data disk attached to a VM.
* @property managedDisk The managedDisk.
* @property snapshot The snapshot.
* @property storageAccountType Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*/
public data class ImageDataDiskArgs(
public val blobUri: Output? = null,
public val caching: Output? = null,
public val diskEncryptionSet: Output? = null,
public val diskSizeGB: Output? = null,
public val lun: Output,
public val managedDisk: Output? = null,
public val snapshot: Output? = null,
public val storageAccountType: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.compute.inputs.ImageDataDiskArgs =
com.pulumi.azurenative.compute.inputs.ImageDataDiskArgs.builder()
.blobUri(blobUri?.applyValue({ args0 -> args0 }))
.caching(caching?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diskEncryptionSet(diskEncryptionSet?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diskSizeGB(diskSizeGB?.applyValue({ args0 -> args0 }))
.lun(lun.applyValue({ args0 -> args0 }))
.managedDisk(managedDisk?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.snapshot(snapshot?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.storageAccountType(
storageAccountType?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
).build()
}
/**
* Builder for [ImageDataDiskArgs].
*/
@PulumiTagMarker
public class ImageDataDiskArgsBuilder internal constructor() {
private var blobUri: Output? = null
private var caching: Output? = null
private var diskEncryptionSet: Output? = null
private var diskSizeGB: Output? = null
private var lun: Output? = null
private var managedDisk: Output? = null
private var snapshot: Output? = null
private var storageAccountType: Output>? = null
/**
* @param value The Virtual Hard Disk.
*/
@JvmName("ajkwpjyjhdtoqoci")
public suspend fun blobUri(`value`: Output) {
this.blobUri = value
}
/**
* @param value Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*/
@JvmName("rriaffamokflippa")
public suspend fun caching(`value`: Output) {
this.caching = value
}
/**
* @param value Specifies the customer managed disk encryption set resource id for the managed image disk.
*/
@JvmName("qxwudjlcnjseoybj")
public suspend fun diskEncryptionSet(`value`: Output) {
this.diskEncryptionSet = value
}
/**
* @param value Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*/
@JvmName("gisdpjyxjhpdrxcd")
public suspend fun diskSizeGB(`value`: Output) {
this.diskSizeGB = value
}
/**
* @param value Specifies the logical unit number of the data disk. This value is used to identify data disks within the VM and therefore must be unique for each data disk attached to a VM.
*/
@JvmName("avcqplyndmdmbuae")
public suspend fun lun(`value`: Output) {
this.lun = value
}
/**
* @param value The managedDisk.
*/
@JvmName("nsxjlhdtxogildvm")
public suspend fun managedDisk(`value`: Output) {
this.managedDisk = value
}
/**
* @param value The snapshot.
*/
@JvmName("jengctkuctudhtyt")
public suspend fun snapshot(`value`: Output) {
this.snapshot = value
}
/**
* @param value Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*/
@JvmName("pxnhaiyilfbvcedk")
public suspend fun storageAccountType(`value`: Output>) {
this.storageAccountType = value
}
/**
* @param value The Virtual Hard Disk.
*/
@JvmName("qkvrwxddsobpywsn")
public suspend fun blobUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blobUri = mapped
}
/**
* @param value Specifies the caching requirements. Possible values are: **None,** **ReadOnly,** **ReadWrite.** The default values are: **None for Standard storage. ReadOnly for Premium storage.**
*/
@JvmName("ixpsqnklmmaqapfg")
public suspend fun caching(`value`: CachingTypes?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caching = mapped
}
/**
* @param value Specifies the customer managed disk encryption set resource id for the managed image disk.
*/
@JvmName("stgjiwmuyqegttmg")
public suspend fun diskEncryptionSet(`value`: DiskEncryptionSetParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskEncryptionSet = mapped
}
/**
* @param argument Specifies the customer managed disk encryption set resource id for the managed image disk.
*/
@JvmName("hkfbdibctycepxya")
public suspend fun diskEncryptionSet(argument: suspend DiskEncryptionSetParametersArgsBuilder.() -> Unit) {
val toBeMapped = DiskEncryptionSetParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.diskEncryptionSet = mapped
}
/**
* @param value Specifies the size of empty data disks in gigabytes. This element can be used to overwrite the name of the disk in a virtual machine image. This value cannot be larger than 1023 GB.
*/
@JvmName("dhdloofyoeuwtvyo")
public suspend fun diskSizeGB(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskSizeGB = mapped
}
/**
* @param value Specifies the logical unit number of the data disk. This value is used to identify data disks within the VM and therefore must be unique for each data disk attached to a VM.
*/
@JvmName("kfltqoanhtlypfks")
public suspend fun lun(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.lun = mapped
}
/**
* @param value The managedDisk.
*/
@JvmName("ntrpfyxjdysyniqk")
public suspend fun managedDisk(`value`: SubResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedDisk = mapped
}
/**
* @param argument The managedDisk.
*/
@JvmName("vbmwqadseecdyhkb")
public suspend fun managedDisk(argument: suspend SubResourceArgsBuilder.() -> Unit) {
val toBeMapped = SubResourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.managedDisk = mapped
}
/**
* @param value The snapshot.
*/
@JvmName("bpxvxgshsnckokjw")
public suspend fun snapshot(`value`: SubResourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshot = mapped
}
/**
* @param argument The snapshot.
*/
@JvmName("hdvhhnlgegrmgvlr")
public suspend fun snapshot(argument: suspend SubResourceArgsBuilder.() -> Unit) {
val toBeMapped = SubResourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.snapshot = mapped
}
/**
* @param value Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*/
@JvmName("yurfnxjkgvwitams")
public suspend fun storageAccountType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageAccountType = mapped
}
/**
* @param value Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*/
@JvmName("qonikugomictnsex")
public fun storageAccountType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageAccountType = mapped
}
/**
* @param value Specifies the storage account type for the managed disk. NOTE: UltraSSD_LRS can only be used with data disks, it cannot be used with OS Disk.
*/
@JvmName("eftcgomimvlmjpuw")
public fun storageAccountType(`value`: StorageAccountTypes) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageAccountType = mapped
}
internal fun build(): ImageDataDiskArgs = ImageDataDiskArgs(
blobUri = blobUri,
caching = caching,
diskEncryptionSet = diskEncryptionSet,
diskSizeGB = diskSizeGB,
lun = lun ?: throw PulumiNullFieldException("lun"),
managedDisk = managedDisk,
snapshot = snapshot,
storageAccountType = storageAccountType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy