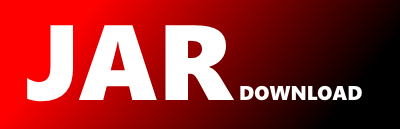
com.pulumi.azurenative.compute.kotlin.inputs.VirtualMachineScaleSetExtensionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.compute.kotlin.inputs
import com.pulumi.azurenative.compute.inputs.VirtualMachineScaleSetExtensionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Describes a Virtual Machine Scale Set Extension.
* @property autoUpgradeMinorVersion Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
* @property enableAutomaticUpgrade Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
* @property forceUpdateTag If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
* @property name The name of the extension.
* @property protectedSettings The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
* @property protectedSettingsFromKeyVault The extensions protected settings that are passed by reference, and consumed from key vault
* @property provisionAfterExtensions Collection of extension names after which this extension needs to be provisioned.
* @property publisher The name of the extension handler publisher.
* @property settings Json formatted public settings for the extension.
* @property suppressFailures Indicates whether failures stemming from the extension will be suppressed (Operational failures such as not connecting to the VM will not be suppressed regardless of this value). The default is false.
* @property type Specifies the type of the extension; an example is "CustomScriptExtension".
* @property typeHandlerVersion Specifies the version of the script handler.
*/
public data class VirtualMachineScaleSetExtensionArgs(
public val autoUpgradeMinorVersion: Output? = null,
public val enableAutomaticUpgrade: Output? = null,
public val forceUpdateTag: Output? = null,
public val name: Output? = null,
public val protectedSettings: Output? = null,
public val protectedSettingsFromKeyVault: Output? = null,
public val provisionAfterExtensions: Output>? = null,
public val publisher: Output? = null,
public val settings: Output? = null,
public val suppressFailures: Output? = null,
public val type: Output? = null,
public val typeHandlerVersion: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.compute.inputs.VirtualMachineScaleSetExtensionArgs =
com.pulumi.azurenative.compute.inputs.VirtualMachineScaleSetExtensionArgs.builder()
.autoUpgradeMinorVersion(autoUpgradeMinorVersion?.applyValue({ args0 -> args0 }))
.enableAutomaticUpgrade(enableAutomaticUpgrade?.applyValue({ args0 -> args0 }))
.forceUpdateTag(forceUpdateTag?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.protectedSettings(protectedSettings?.applyValue({ args0 -> args0 }))
.protectedSettingsFromKeyVault(
protectedSettingsFromKeyVault?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.provisionAfterExtensions(
provisionAfterExtensions?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
)
.publisher(publisher?.applyValue({ args0 -> args0 }))
.settings(settings?.applyValue({ args0 -> args0 }))
.suppressFailures(suppressFailures?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 }))
.typeHandlerVersion(typeHandlerVersion?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [VirtualMachineScaleSetExtensionArgs].
*/
@PulumiTagMarker
public class VirtualMachineScaleSetExtensionArgsBuilder internal constructor() {
private var autoUpgradeMinorVersion: Output? = null
private var enableAutomaticUpgrade: Output? = null
private var forceUpdateTag: Output? = null
private var name: Output? = null
private var protectedSettings: Output? = null
private var protectedSettingsFromKeyVault: Output? = null
private var provisionAfterExtensions: Output>? = null
private var publisher: Output? = null
private var settings: Output? = null
private var suppressFailures: Output? = null
private var type: Output? = null
private var typeHandlerVersion: Output? = null
/**
* @param value Indicates whether the extension should use a newer minor version if one is available at deployment time. Once deployed, however, the extension will not upgrade minor versions unless redeployed, even with this property set to true.
*/
@JvmName("rjsuwntckxwpgejp")
public suspend fun autoUpgradeMinorVersion(`value`: Output) {
this.autoUpgradeMinorVersion = value
}
/**
* @param value Indicates whether the extension should be automatically upgraded by the platform if there is a newer version of the extension available.
*/
@JvmName("nljwlhxyfdjclyur")
public suspend fun enableAutomaticUpgrade(`value`: Output) {
this.enableAutomaticUpgrade = value
}
/**
* @param value If a value is provided and is different from the previous value, the extension handler will be forced to update even if the extension configuration has not changed.
*/
@JvmName("fpnlqvbldcxptreb")
public suspend fun forceUpdateTag(`value`: Output) {
this.forceUpdateTag = value
}
/**
* @param value The name of the extension.
*/
@JvmName("kihopbgqvfngiqft")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The extension can contain either protectedSettings or protectedSettingsFromKeyVault or no protected settings at all.
*/
@JvmName("epvgserhcelljhlv")
public suspend fun protectedSettings(`value`: Output) {
this.protectedSettings = value
}
/**
* @param value The extensions protected settings that are passed by reference, and consumed from key vault
*/
@JvmName("qsvdtrorlmqrkfgw")
public suspend fun protectedSettingsFromKeyVault(`value`: Output) {
this.protectedSettingsFromKeyVault = value
}
/**
* @param value Collection of extension names after which this extension needs to be provisioned.
*/
@JvmName("bcmvymdovrrsonwv")
public suspend fun provisionAfterExtensions(`value`: Output>) {
this.provisionAfterExtensions = value
}
@JvmName("rifwmvptgoetjyvf")
public suspend fun provisionAfterExtensions(vararg values: Output) {
this.provisionAfterExtensions = Output.all(values.asList())
}
/**
* @param values Collection of extension names after which this extension needs to be provisioned.
*/
@JvmName("fjiuwhcwaorxquda")
public suspend fun provisionAfterExtensions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy