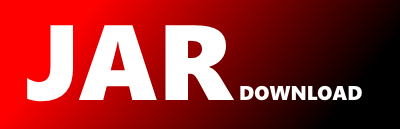
com.pulumi.azurenative.containerinstance.kotlin.inputs.ContainerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.containerinstance.kotlin.inputs
import com.pulumi.azurenative.containerinstance.inputs.ContainerArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* A container instance.
* @property command The commands to execute within the container instance in exec form.
* @property environmentVariables The environment variables to set in the container instance.
* @property image The name of the image used to create the container instance.
* @property livenessProbe The liveness probe.
* @property name The user-provided name of the container instance.
* @property ports The exposed ports on the container instance.
* @property readinessProbe The readiness probe.
* @property resources The resource requirements of the container instance.
* @property securityContext The container security properties.
* @property volumeMounts The volume mounts available to the container instance.
*/
public data class ContainerArgs(
public val command: Output>? = null,
public val environmentVariables: Output>? = null,
public val image: Output,
public val livenessProbe: Output? = null,
public val name: Output,
public val ports: Output>? = null,
public val readinessProbe: Output? = null,
public val resources: Output,
public val securityContext: Output? = null,
public val volumeMounts: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.containerinstance.inputs.ContainerArgs =
com.pulumi.azurenative.containerinstance.inputs.ContainerArgs.builder()
.command(command?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.environmentVariables(
environmentVariables?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.image(image.applyValue({ args0 -> args0 }))
.livenessProbe(livenessProbe?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.ports(ports?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.readinessProbe(readinessProbe?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resources(resources.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.securityContext(securityContext?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.volumeMounts(
volumeMounts?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ContainerArgs].
*/
@PulumiTagMarker
public class ContainerArgsBuilder internal constructor() {
private var command: Output>? = null
private var environmentVariables: Output>? = null
private var image: Output? = null
private var livenessProbe: Output? = null
private var name: Output? = null
private var ports: Output>? = null
private var readinessProbe: Output? = null
private var resources: Output? = null
private var securityContext: Output? = null
private var volumeMounts: Output>? = null
/**
* @param value The commands to execute within the container instance in exec form.
*/
@JvmName("sxudhsthnusdxqka")
public suspend fun command(`value`: Output>) {
this.command = value
}
@JvmName("arsguftxpbovseju")
public suspend fun command(vararg values: Output) {
this.command = Output.all(values.asList())
}
/**
* @param values The commands to execute within the container instance in exec form.
*/
@JvmName("ysmgggecxisipqkq")
public suspend fun command(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy