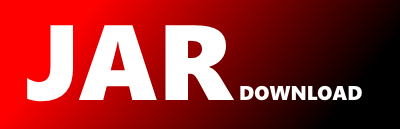
com.pulumi.azurenative.containerregistry.kotlin.TaskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.containerregistry.kotlin
import com.pulumi.azurenative.containerregistry.TaskArgs.builder
import com.pulumi.azurenative.containerregistry.kotlin.enums.TaskStatus
import com.pulumi.azurenative.containerregistry.kotlin.inputs.AgentPropertiesArgs
import com.pulumi.azurenative.containerregistry.kotlin.inputs.AgentPropertiesArgsBuilder
import com.pulumi.azurenative.containerregistry.kotlin.inputs.CredentialsArgs
import com.pulumi.azurenative.containerregistry.kotlin.inputs.CredentialsArgsBuilder
import com.pulumi.azurenative.containerregistry.kotlin.inputs.IdentityPropertiesArgs
import com.pulumi.azurenative.containerregistry.kotlin.inputs.IdentityPropertiesArgsBuilder
import com.pulumi.azurenative.containerregistry.kotlin.inputs.PlatformPropertiesArgs
import com.pulumi.azurenative.containerregistry.kotlin.inputs.PlatformPropertiesArgsBuilder
import com.pulumi.azurenative.containerregistry.kotlin.inputs.TriggerPropertiesArgs
import com.pulumi.azurenative.containerregistry.kotlin.inputs.TriggerPropertiesArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The task that has the ARM resource and task properties.
* The task will have all information to schedule a run against it.
* Azure REST API version: 2019-06-01-preview. Prior API version in Azure Native 1.x: 2019-06-01-preview.
* Other available API versions: 2018-09-01, 2019-04-01.
* ## Example Usage
* ### Tasks_Create
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var task = new AzureNative.ContainerRegistry.Task("task", new()
* {
* AgentConfiguration = new AzureNative.ContainerRegistry.Inputs.AgentPropertiesArgs
* {
* Cpu = 2,
* },
* Identity = new AzureNative.ContainerRegistry.Inputs.IdentityPropertiesArgs
* {
* Type = AzureNative.ContainerRegistry.ResourceIdentityType.SystemAssigned,
* },
* IsSystemTask = false,
* Location = "eastus",
* LogTemplate = "acr/tasks:{{.Run.OS}}",
* Platform = new AzureNative.ContainerRegistry.Inputs.PlatformPropertiesArgs
* {
* Architecture = AzureNative.ContainerRegistry.Architecture.Amd64,
* Os = AzureNative.ContainerRegistry.OS.Linux,
* },
* RegistryName = "myRegistry",
* ResourceGroupName = "myResourceGroup",
* Status = AzureNative.ContainerRegistry.TaskStatus.Enabled,
* Step = new AzureNative.ContainerRegistry.Inputs.DockerBuildStepArgs
* {
* Arguments = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = false,
* Name = "mytestargument",
* Value = "mytestvalue",
* },
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = true,
* Name = "mysecrettestargument",
* Value = "mysecrettestvalue",
* },
* },
* ContextPath = "src",
* DockerFilePath = "src/DockerFile",
* ImageNames = new[]
* {
* "azurerest:testtag",
* },
* IsPushEnabled = true,
* NoCache = false,
* Type = "Docker",
* },
* Tags =
* {
* { "testkey", "value" },
* },
* TaskName = "mytTask",
* Trigger = new AzureNative.ContainerRegistry.Inputs.TriggerPropertiesArgs
* {
* BaseImageTrigger = new AzureNative.ContainerRegistry.Inputs.BaseImageTriggerArgs
* {
* BaseImageTriggerType = AzureNative.ContainerRegistry.BaseImageTriggerType.Runtime,
* Name = "myBaseImageTrigger",
* UpdateTriggerEndpoint = "https://user:[email protected]?token=foo",
* UpdateTriggerPayloadType = AzureNative.ContainerRegistry.UpdateTriggerPayloadType.Token,
* },
* SourceTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.SourceTriggerArgs
* {
* Name = "mySourceTrigger",
* SourceRepository = new AzureNative.ContainerRegistry.Inputs.SourcePropertiesArgs
* {
* Branch = "master",
* RepositoryUrl = "https://github.com/Azure/azure-rest-api-specs",
* SourceControlAuthProperties = new AzureNative.ContainerRegistry.Inputs.AuthInfoArgs
* {
* Token = "xxxxx",
* TokenType = AzureNative.ContainerRegistry.TokenType.PAT,
* },
* SourceControlType = AzureNative.ContainerRegistry.SourceControlType.Github,
* },
* SourceTriggerEvents = new[]
* {
* AzureNative.ContainerRegistry.SourceTriggerEvent.Commit,
* },
* },
* },
* TimerTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.TimerTriggerArgs
* {
* Name = "myTimerTrigger",
* Schedule = "30 9 * * 1-5",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* containerregistry "github.com/pulumi/pulumi-azure-native-sdk/containerregistry/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerregistry.NewTask(ctx, "task", &containerregistry.TaskArgs{
* AgentConfiguration: &containerregistry.AgentPropertiesArgs{
* Cpu: pulumi.Int(2),
* },
* Identity: &containerregistry.IdentityPropertiesArgs{
* Type: containerregistry.ResourceIdentityTypeSystemAssigned,
* },
* IsSystemTask: pulumi.Bool(false),
* Location: pulumi.String("eastus"),
* LogTemplate: pulumi.String("acr/tasks:{{.Run.OS}}"),
* Platform: &containerregistry.PlatformPropertiesArgs{
* Architecture: pulumi.String(containerregistry.ArchitectureAmd64),
* Os: pulumi.String(containerregistry.OSLinux),
* },
* RegistryName: pulumi.String("myRegistry"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Status: pulumi.String(containerregistry.TaskStatusEnabled),
* Step: &containerregistry.DockerBuildStepArgs{
* Arguments: containerregistry.ArgumentArray{
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(false),
* Name: pulumi.String("mytestargument"),
* Value: pulumi.String("mytestvalue"),
* },
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(true),
* Name: pulumi.String("mysecrettestargument"),
* Value: pulumi.String("mysecrettestvalue"),
* },
* },
* ContextPath: pulumi.String("src"),
* DockerFilePath: pulumi.String("src/DockerFile"),
* ImageNames: pulumi.StringArray{
* pulumi.String("azurerest:testtag"),
* },
* IsPushEnabled: pulumi.Bool(true),
* NoCache: pulumi.Bool(false),
* Type: pulumi.String("Docker"),
* },
* Tags: pulumi.StringMap{
* "testkey": pulumi.String("value"),
* },
* TaskName: pulumi.String("mytTask"),
* Trigger: &containerregistry.TriggerPropertiesArgs{
* BaseImageTrigger: &containerregistry.BaseImageTriggerArgs{
* BaseImageTriggerType: pulumi.String(containerregistry.BaseImageTriggerTypeRuntime),
* Name: pulumi.String("myBaseImageTrigger"),
* UpdateTriggerEndpoint: pulumi.String("https://user:[email protected]?token=foo"),
* UpdateTriggerPayloadType: pulumi.String(containerregistry.UpdateTriggerPayloadTypeToken),
* },
* SourceTriggers: containerregistry.SourceTriggerArray{
* &containerregistry.SourceTriggerArgs{
* Name: pulumi.String("mySourceTrigger"),
* SourceRepository: &containerregistry.SourcePropertiesArgs{
* Branch: pulumi.String("master"),
* RepositoryUrl: pulumi.String("https://github.com/Azure/azure-rest-api-specs"),
* SourceControlAuthProperties: &containerregistry.AuthInfoArgs{
* Token: pulumi.String("xxxxx"),
* TokenType: pulumi.String(containerregistry.TokenTypePAT),
* },
* SourceControlType: pulumi.String(containerregistry.SourceControlTypeGithub),
* },
* SourceTriggerEvents: pulumi.StringArray{
* pulumi.String(containerregistry.SourceTriggerEventCommit),
* },
* },
* },
* TimerTriggers: containerregistry.TimerTriggerArray{
* &containerregistry.TimerTriggerArgs{
* Name: pulumi.String("myTimerTrigger"),
* Schedule: pulumi.String("30 9 * * 1-5"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned")
* .build())
* .isSystemTask(false)
* .location("eastus")
* .logTemplate("acr/tasks:{{.Run.OS}}")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:[email protected]?token=foo")
* .updateTriggerPayloadType("Token")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
* }
* }
* ```
* ### Tasks_Create_QuickTask
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var task = new AzureNative.ContainerRegistry.Task("task", new()
* {
* IsSystemTask = true,
* Location = "eastus",
* LogTemplate = "acr/tasks:{{.Run.OS}}",
* RegistryName = "myRegistry",
* ResourceGroupName = "myResourceGroup",
* Status = AzureNative.ContainerRegistry.TaskStatus.Enabled,
* Tags =
* {
* { "testkey", "value" },
* },
* TaskName = "quicktask",
* });
* });
* ```
* ```go
* package main
* import (
* containerregistry "github.com/pulumi/pulumi-azure-native-sdk/containerregistry/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerregistry.NewTask(ctx, "task", &containerregistry.TaskArgs{
* IsSystemTask: pulumi.Bool(true),
* Location: pulumi.String("eastus"),
* LogTemplate: pulumi.String("acr/tasks:{{.Run.OS}}"),
* RegistryName: pulumi.String("myRegistry"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Status: pulumi.String(containerregistry.TaskStatusEnabled),
* Tags: pulumi.StringMap{
* "testkey": pulumi.String("value"),
* },
* TaskName: pulumi.String("quicktask"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .isSystemTask(true)
* .location("eastus")
* .logTemplate("acr/tasks:{{.Run.OS}}")
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .tags(Map.of("testkey", "value"))
* .taskName("quicktask")
* .build());
* }
* }
* ```
* ### Tasks_Create_WithSystemAndUserIdentities
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var task = new AzureNative.ContainerRegistry.Task("task", new()
* {
* AgentConfiguration = new AzureNative.ContainerRegistry.Inputs.AgentPropertiesArgs
* {
* Cpu = 2,
* },
* Identity = new AzureNative.ContainerRegistry.Inputs.IdentityPropertiesArgs
* {
* Type = AzureNative.ContainerRegistry.ResourceIdentityType.SystemAssigned_UserAssigned,
* UserAssignedIdentities =
* {
* { "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", null },
* },
* },
* IsSystemTask = false,
* Location = "eastus",
* Platform = new AzureNative.ContainerRegistry.Inputs.PlatformPropertiesArgs
* {
* Architecture = AzureNative.ContainerRegistry.Architecture.Amd64,
* Os = AzureNative.ContainerRegistry.OS.Linux,
* },
* RegistryName = "myRegistry",
* ResourceGroupName = "myResourceGroup",
* Status = AzureNative.ContainerRegistry.TaskStatus.Enabled,
* Step = new AzureNative.ContainerRegistry.Inputs.DockerBuildStepArgs
* {
* Arguments = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = false,
* Name = "mytestargument",
* Value = "mytestvalue",
* },
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = true,
* Name = "mysecrettestargument",
* Value = "mysecrettestvalue",
* },
* },
* ContextPath = "src",
* DockerFilePath = "src/DockerFile",
* ImageNames = new[]
* {
* "azurerest:testtag",
* },
* IsPushEnabled = true,
* NoCache = false,
* Type = "Docker",
* },
* Tags =
* {
* { "testkey", "value" },
* },
* TaskName = "mytTask",
* Trigger = new AzureNative.ContainerRegistry.Inputs.TriggerPropertiesArgs
* {
* BaseImageTrigger = new AzureNative.ContainerRegistry.Inputs.BaseImageTriggerArgs
* {
* BaseImageTriggerType = AzureNative.ContainerRegistry.BaseImageTriggerType.Runtime,
* Name = "myBaseImageTrigger",
* UpdateTriggerEndpoint = "https://user:[email protected]?token=foo",
* UpdateTriggerPayloadType = AzureNative.ContainerRegistry.UpdateTriggerPayloadType.Default,
* },
* SourceTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.SourceTriggerArgs
* {
* Name = "mySourceTrigger",
* SourceRepository = new AzureNative.ContainerRegistry.Inputs.SourcePropertiesArgs
* {
* Branch = "master",
* RepositoryUrl = "https://github.com/Azure/azure-rest-api-specs",
* SourceControlAuthProperties = new AzureNative.ContainerRegistry.Inputs.AuthInfoArgs
* {
* Token = "xxxxx",
* TokenType = AzureNative.ContainerRegistry.TokenType.PAT,
* },
* SourceControlType = AzureNative.ContainerRegistry.SourceControlType.Github,
* },
* SourceTriggerEvents = new[]
* {
* AzureNative.ContainerRegistry.SourceTriggerEvent.Commit,
* },
* },
* },
* TimerTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.TimerTriggerArgs
* {
* Name = "myTimerTrigger",
* Schedule = "30 9 * * 1-5",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* containerregistry "github.com/pulumi/pulumi-azure-native-sdk/containerregistry/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerregistry.NewTask(ctx, "task", &containerregistry.TaskArgs{
* AgentConfiguration: &containerregistry.AgentPropertiesArgs{
* Cpu: pulumi.Int(2),
* },
* Identity: &containerregistry.IdentityPropertiesArgs{
* Type: containerregistry.ResourceIdentityType_SystemAssigned_UserAssigned,
* UserAssignedIdentities: containerregistry.UserIdentityPropertiesMap{
* "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2": nil,
* },
* },
* IsSystemTask: pulumi.Bool(false),
* Location: pulumi.String("eastus"),
* Platform: &containerregistry.PlatformPropertiesArgs{
* Architecture: pulumi.String(containerregistry.ArchitectureAmd64),
* Os: pulumi.String(containerregistry.OSLinux),
* },
* RegistryName: pulumi.String("myRegistry"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Status: pulumi.String(containerregistry.TaskStatusEnabled),
* Step: &containerregistry.DockerBuildStepArgs{
* Arguments: containerregistry.ArgumentArray{
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(false),
* Name: pulumi.String("mytestargument"),
* Value: pulumi.String("mytestvalue"),
* },
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(true),
* Name: pulumi.String("mysecrettestargument"),
* Value: pulumi.String("mysecrettestvalue"),
* },
* },
* ContextPath: pulumi.String("src"),
* DockerFilePath: pulumi.String("src/DockerFile"),
* ImageNames: pulumi.StringArray{
* pulumi.String("azurerest:testtag"),
* },
* IsPushEnabled: pulumi.Bool(true),
* NoCache: pulumi.Bool(false),
* Type: pulumi.String("Docker"),
* },
* Tags: pulumi.StringMap{
* "testkey": pulumi.String("value"),
* },
* TaskName: pulumi.String("mytTask"),
* Trigger: &containerregistry.TriggerPropertiesArgs{
* BaseImageTrigger: &containerregistry.BaseImageTriggerArgs{
* BaseImageTriggerType: pulumi.String(containerregistry.BaseImageTriggerTypeRuntime),
* Name: pulumi.String("myBaseImageTrigger"),
* UpdateTriggerEndpoint: pulumi.String("https://user:[email protected]?token=foo"),
* UpdateTriggerPayloadType: pulumi.String(containerregistry.UpdateTriggerPayloadTypeDefault),
* },
* SourceTriggers: containerregistry.SourceTriggerArray{
* &containerregistry.SourceTriggerArgs{
* Name: pulumi.String("mySourceTrigger"),
* SourceRepository: &containerregistry.SourcePropertiesArgs{
* Branch: pulumi.String("master"),
* RepositoryUrl: pulumi.String("https://github.com/Azure/azure-rest-api-specs"),
* SourceControlAuthProperties: &containerregistry.AuthInfoArgs{
* Token: pulumi.String("xxxxx"),
* TokenType: pulumi.String(containerregistry.TokenTypePAT),
* },
* SourceControlType: pulumi.String(containerregistry.SourceControlTypeGithub),
* },
* SourceTriggerEvents: pulumi.StringArray{
* pulumi.String(containerregistry.SourceTriggerEventCommit),
* },
* },
* },
* TimerTriggers: containerregistry.TimerTriggerArray{
* &containerregistry.TimerTriggerArgs{
* Name: pulumi.String("myTimerTrigger"),
* Schedule: pulumi.String("30 9 * * 1-5"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned, UserAssigned")
* .userAssignedIdentities(Map.of("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", ))
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:[email protected]?token=foo")
* .updateTriggerPayloadType("Default")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
* }
* }
* ```
* ### Tasks_Create_WithUserIdentities
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var task = new AzureNative.ContainerRegistry.Task("task", new()
* {
* AgentConfiguration = new AzureNative.ContainerRegistry.Inputs.AgentPropertiesArgs
* {
* Cpu = 2,
* },
* Identity = new AzureNative.ContainerRegistry.Inputs.IdentityPropertiesArgs
* {
* Type = AzureNative.ContainerRegistry.ResourceIdentityType.UserAssigned,
* UserAssignedIdentities =
* {
* { "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity1", null },
* { "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", null },
* },
* },
* IsSystemTask = false,
* Location = "eastus",
* Platform = new AzureNative.ContainerRegistry.Inputs.PlatformPropertiesArgs
* {
* Architecture = AzureNative.ContainerRegistry.Architecture.Amd64,
* Os = AzureNative.ContainerRegistry.OS.Linux,
* },
* RegistryName = "myRegistry",
* ResourceGroupName = "myResourceGroup",
* Status = AzureNative.ContainerRegistry.TaskStatus.Enabled,
* Step = new AzureNative.ContainerRegistry.Inputs.DockerBuildStepArgs
* {
* Arguments = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = false,
* Name = "mytestargument",
* Value = "mytestvalue",
* },
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = true,
* Name = "mysecrettestargument",
* Value = "mysecrettestvalue",
* },
* },
* ContextPath = "src",
* DockerFilePath = "src/DockerFile",
* ImageNames = new[]
* {
* "azurerest:testtag",
* },
* IsPushEnabled = true,
* NoCache = false,
* Type = "Docker",
* },
* Tags =
* {
* { "testkey", "value" },
* },
* TaskName = "mytTask",
* Trigger = new AzureNative.ContainerRegistry.Inputs.TriggerPropertiesArgs
* {
* BaseImageTrigger = new AzureNative.ContainerRegistry.Inputs.BaseImageTriggerArgs
* {
* BaseImageTriggerType = AzureNative.ContainerRegistry.BaseImageTriggerType.Runtime,
* Name = "myBaseImageTrigger",
* UpdateTriggerEndpoint = "https://user:[email protected]?token=foo",
* UpdateTriggerPayloadType = AzureNative.ContainerRegistry.UpdateTriggerPayloadType.Default,
* },
* SourceTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.SourceTriggerArgs
* {
* Name = "mySourceTrigger",
* SourceRepository = new AzureNative.ContainerRegistry.Inputs.SourcePropertiesArgs
* {
* Branch = "master",
* RepositoryUrl = "https://github.com/Azure/azure-rest-api-specs",
* SourceControlAuthProperties = new AzureNative.ContainerRegistry.Inputs.AuthInfoArgs
* {
* Token = "xxxxx",
* TokenType = AzureNative.ContainerRegistry.TokenType.PAT,
* },
* SourceControlType = AzureNative.ContainerRegistry.SourceControlType.Github,
* },
* SourceTriggerEvents = new[]
* {
* AzureNative.ContainerRegistry.SourceTriggerEvent.Commit,
* },
* },
* },
* TimerTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.TimerTriggerArgs
* {
* Name = "myTimerTrigger",
* Schedule = "30 9 * * 1-5",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* containerregistry "github.com/pulumi/pulumi-azure-native-sdk/containerregistry/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerregistry.NewTask(ctx, "task", &containerregistry.TaskArgs{
* AgentConfiguration: &containerregistry.AgentPropertiesArgs{
* Cpu: pulumi.Int(2),
* },
* Identity: &containerregistry.IdentityPropertiesArgs{
* Type: containerregistry.ResourceIdentityTypeUserAssigned,
* UserAssignedIdentities: containerregistry.UserIdentityPropertiesMap{
* "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity1": nil,
* "/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2": nil,
* },
* },
* IsSystemTask: pulumi.Bool(false),
* Location: pulumi.String("eastus"),
* Platform: &containerregistry.PlatformPropertiesArgs{
* Architecture: pulumi.String(containerregistry.ArchitectureAmd64),
* Os: pulumi.String(containerregistry.OSLinux),
* },
* RegistryName: pulumi.String("myRegistry"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Status: pulumi.String(containerregistry.TaskStatusEnabled),
* Step: &containerregistry.DockerBuildStepArgs{
* Arguments: containerregistry.ArgumentArray{
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(false),
* Name: pulumi.String("mytestargument"),
* Value: pulumi.String("mytestvalue"),
* },
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(true),
* Name: pulumi.String("mysecrettestargument"),
* Value: pulumi.String("mysecrettestvalue"),
* },
* },
* ContextPath: pulumi.String("src"),
* DockerFilePath: pulumi.String("src/DockerFile"),
* ImageNames: pulumi.StringArray{
* pulumi.String("azurerest:testtag"),
* },
* IsPushEnabled: pulumi.Bool(true),
* NoCache: pulumi.Bool(false),
* Type: pulumi.String("Docker"),
* },
* Tags: pulumi.StringMap{
* "testkey": pulumi.String("value"),
* },
* TaskName: pulumi.String("mytTask"),
* Trigger: &containerregistry.TriggerPropertiesArgs{
* BaseImageTrigger: &containerregistry.BaseImageTriggerArgs{
* BaseImageTriggerType: pulumi.String(containerregistry.BaseImageTriggerTypeRuntime),
* Name: pulumi.String("myBaseImageTrigger"),
* UpdateTriggerEndpoint: pulumi.String("https://user:[email protected]?token=foo"),
* UpdateTriggerPayloadType: pulumi.String(containerregistry.UpdateTriggerPayloadTypeDefault),
* },
* SourceTriggers: containerregistry.SourceTriggerArray{
* &containerregistry.SourceTriggerArgs{
* Name: pulumi.String("mySourceTrigger"),
* SourceRepository: &containerregistry.SourcePropertiesArgs{
* Branch: pulumi.String("master"),
* RepositoryUrl: pulumi.String("https://github.com/Azure/azure-rest-api-specs"),
* SourceControlAuthProperties: &containerregistry.AuthInfoArgs{
* Token: pulumi.String("xxxxx"),
* TokenType: pulumi.String(containerregistry.TokenTypePAT),
* },
* SourceControlType: pulumi.String(containerregistry.SourceControlTypeGithub),
* },
* SourceTriggerEvents: pulumi.StringArray{
* pulumi.String(containerregistry.SourceTriggerEventCommit),
* },
* },
* },
* TimerTriggers: containerregistry.TimerTriggerArray{
* &containerregistry.TimerTriggerArgs{
* Name: pulumi.String("myTimerTrigger"),
* Schedule: pulumi.String("30 9 * * 1-5"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("UserAssigned")
* .userAssignedIdentities(Map.ofEntries(
* Map.entry("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity1", ),
* Map.entry("/subscriptions/f9d7ebed-adbd-4cb4-b973-aaf82c136138/resourcegroups/myResourceGroup1/providers/Microsoft.ManagedIdentity/userAssignedIdentities/identity2", )
* ))
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .updateTriggerEndpoint("https://user:[email protected]?token=foo")
* .updateTriggerPayloadType("Default")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
* }
* }
* ```
* ### Tasks_Create_WithUserIdentities_WithSystemIdentity
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var task = new AzureNative.ContainerRegistry.Task("task", new()
* {
* AgentConfiguration = new AzureNative.ContainerRegistry.Inputs.AgentPropertiesArgs
* {
* Cpu = 2,
* },
* Identity = new AzureNative.ContainerRegistry.Inputs.IdentityPropertiesArgs
* {
* Type = AzureNative.ContainerRegistry.ResourceIdentityType.SystemAssigned,
* },
* IsSystemTask = false,
* Location = "eastus",
* Platform = new AzureNative.ContainerRegistry.Inputs.PlatformPropertiesArgs
* {
* Architecture = AzureNative.ContainerRegistry.Architecture.Amd64,
* Os = AzureNative.ContainerRegistry.OS.Linux,
* },
* RegistryName = "myRegistry",
* ResourceGroupName = "myResourceGroup",
* Status = AzureNative.ContainerRegistry.TaskStatus.Enabled,
* Step = new AzureNative.ContainerRegistry.Inputs.DockerBuildStepArgs
* {
* Arguments = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = false,
* Name = "mytestargument",
* Value = "mytestvalue",
* },
* new AzureNative.ContainerRegistry.Inputs.ArgumentArgs
* {
* IsSecret = true,
* Name = "mysecrettestargument",
* Value = "mysecrettestvalue",
* },
* },
* ContextPath = "src",
* DockerFilePath = "src/DockerFile",
* ImageNames = new[]
* {
* "azurerest:testtag",
* },
* IsPushEnabled = true,
* NoCache = false,
* Type = "Docker",
* },
* Tags =
* {
* { "testkey", "value" },
* },
* TaskName = "mytTask",
* Trigger = new AzureNative.ContainerRegistry.Inputs.TriggerPropertiesArgs
* {
* BaseImageTrigger = new AzureNative.ContainerRegistry.Inputs.BaseImageTriggerArgs
* {
* BaseImageTriggerType = AzureNative.ContainerRegistry.BaseImageTriggerType.Runtime,
* Name = "myBaseImageTrigger",
* },
* SourceTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.SourceTriggerArgs
* {
* Name = "mySourceTrigger",
* SourceRepository = new AzureNative.ContainerRegistry.Inputs.SourcePropertiesArgs
* {
* Branch = "master",
* RepositoryUrl = "https://github.com/Azure/azure-rest-api-specs",
* SourceControlAuthProperties = new AzureNative.ContainerRegistry.Inputs.AuthInfoArgs
* {
* Token = "xxxxx",
* TokenType = AzureNative.ContainerRegistry.TokenType.PAT,
* },
* SourceControlType = AzureNative.ContainerRegistry.SourceControlType.Github,
* },
* SourceTriggerEvents = new[]
* {
* AzureNative.ContainerRegistry.SourceTriggerEvent.Commit,
* },
* },
* },
* TimerTriggers = new[]
* {
* new AzureNative.ContainerRegistry.Inputs.TimerTriggerArgs
* {
* Name = "myTimerTrigger",
* Schedule = "30 9 * * 1-5",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* containerregistry "github.com/pulumi/pulumi-azure-native-sdk/containerregistry/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerregistry.NewTask(ctx, "task", &containerregistry.TaskArgs{
* AgentConfiguration: &containerregistry.AgentPropertiesArgs{
* Cpu: pulumi.Int(2),
* },
* Identity: &containerregistry.IdentityPropertiesArgs{
* Type: containerregistry.ResourceIdentityTypeSystemAssigned,
* },
* IsSystemTask: pulumi.Bool(false),
* Location: pulumi.String("eastus"),
* Platform: &containerregistry.PlatformPropertiesArgs{
* Architecture: pulumi.String(containerregistry.ArchitectureAmd64),
* Os: pulumi.String(containerregistry.OSLinux),
* },
* RegistryName: pulumi.String("myRegistry"),
* ResourceGroupName: pulumi.String("myResourceGroup"),
* Status: pulumi.String(containerregistry.TaskStatusEnabled),
* Step: &containerregistry.DockerBuildStepArgs{
* Arguments: containerregistry.ArgumentArray{
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(false),
* Name: pulumi.String("mytestargument"),
* Value: pulumi.String("mytestvalue"),
* },
* &containerregistry.ArgumentArgs{
* IsSecret: pulumi.Bool(true),
* Name: pulumi.String("mysecrettestargument"),
* Value: pulumi.String("mysecrettestvalue"),
* },
* },
* ContextPath: pulumi.String("src"),
* DockerFilePath: pulumi.String("src/DockerFile"),
* ImageNames: pulumi.StringArray{
* pulumi.String("azurerest:testtag"),
* },
* IsPushEnabled: pulumi.Bool(true),
* NoCache: pulumi.Bool(false),
* Type: pulumi.String("Docker"),
* },
* Tags: pulumi.StringMap{
* "testkey": pulumi.String("value"),
* },
* TaskName: pulumi.String("mytTask"),
* Trigger: &containerregistry.TriggerPropertiesArgs{
* BaseImageTrigger: &containerregistry.BaseImageTriggerArgs{
* BaseImageTriggerType: pulumi.String(containerregistry.BaseImageTriggerTypeRuntime),
* Name: pulumi.String("myBaseImageTrigger"),
* },
* SourceTriggers: containerregistry.SourceTriggerArray{
* &containerregistry.SourceTriggerArgs{
* Name: pulumi.String("mySourceTrigger"),
* SourceRepository: &containerregistry.SourcePropertiesArgs{
* Branch: pulumi.String("master"),
* RepositoryUrl: pulumi.String("https://github.com/Azure/azure-rest-api-specs"),
* SourceControlAuthProperties: &containerregistry.AuthInfoArgs{
* Token: pulumi.String("xxxxx"),
* TokenType: pulumi.String(containerregistry.TokenTypePAT),
* },
* SourceControlType: pulumi.String(containerregistry.SourceControlTypeGithub),
* },
* SourceTriggerEvents: pulumi.StringArray{
* pulumi.String(containerregistry.SourceTriggerEventCommit),
* },
* },
* },
* TimerTriggers: containerregistry.TimerTriggerArray{
* &containerregistry.TimerTriggerArgs{
* Name: pulumi.String("myTimerTrigger"),
* Schedule: pulumi.String("30 9 * * 1-5"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.containerregistry.Task;
* import com.pulumi.azurenative.containerregistry.TaskArgs;
* import com.pulumi.azurenative.containerregistry.inputs.AgentPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.IdentityPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.PlatformPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.TriggerPropertiesArgs;
* import com.pulumi.azurenative.containerregistry.inputs.BaseImageTriggerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var task = new Task("task", TaskArgs.builder()
* .agentConfiguration(AgentPropertiesArgs.builder()
* .cpu(2)
* .build())
* .identity(IdentityPropertiesArgs.builder()
* .type("SystemAssigned")
* .build())
* .isSystemTask(false)
* .location("eastus")
* .platform(PlatformPropertiesArgs.builder()
* .architecture("amd64")
* .os("Linux")
* .build())
* .registryName("myRegistry")
* .resourceGroupName("myResourceGroup")
* .status("Enabled")
* .step(DockerBuildStepArgs.builder()
* .arguments(
* ArgumentArgs.builder()
* .isSecret(false)
* .name("mytestargument")
* .value("mytestvalue")
* .build(),
* ArgumentArgs.builder()
* .isSecret(true)
* .name("mysecrettestargument")
* .value("mysecrettestvalue")
* .build())
* .contextPath("src")
* .dockerFilePath("src/DockerFile")
* .imageNames("azurerest:testtag")
* .isPushEnabled(true)
* .noCache(false)
* .type("Docker")
* .build())
* .tags(Map.of("testkey", "value"))
* .taskName("mytTask")
* .trigger(TriggerPropertiesArgs.builder()
* .baseImageTrigger(BaseImageTriggerArgs.builder()
* .baseImageTriggerType("Runtime")
* .name("myBaseImageTrigger")
* .build())
* .sourceTriggers(SourceTriggerArgs.builder()
* .name("mySourceTrigger")
* .sourceRepository(SourcePropertiesArgs.builder()
* .branch("master")
* .repositoryUrl("https://github.com/Azure/azure-rest-api-specs")
* .sourceControlAuthProperties(AuthInfoArgs.builder()
* .token("xxxxx")
* .tokenType("PAT")
* .build())
* .sourceControlType("Github")
* .build())
* .sourceTriggerEvents("commit")
* .build())
* .timerTriggers(TimerTriggerArgs.builder()
* .name("myTimerTrigger")
* .schedule("30 9 * * 1-5")
* .build())
* .build())
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:containerregistry:Task myTask /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.ContainerRegistry/registries/{registryName}/tasks/{taskName}
* ```
* @property agentConfiguration The machine configuration of the run agent.
* @property agentPoolName The dedicated agent pool for the task.
* @property credentials The properties that describes a set of credentials that will be used when this run is invoked.
* @property identity Identity for the resource.
* @property isSystemTask The value of this property indicates whether the task resource is system task or not.
* @property location The location of the resource. This cannot be changed after the resource is created.
* @property logTemplate The template that describes the repository and tag information for run log artifact.
* @property platform The platform properties against which the run has to happen.
* @property registryName The name of the container registry.
* @property resourceGroupName The name of the resource group to which the container registry belongs.
* @property status The current status of task.
* @property step The properties of a task step.
* @property tags The tags of the resource.
* @property taskName The name of the container registry task.
* @property timeout Run timeout in seconds.
* @property trigger The properties that describe all triggers for the task.
*/
public data class TaskArgs(
public val agentConfiguration: Output? = null,
public val agentPoolName: Output? = null,
public val credentials: Output? = null,
public val identity: Output? = null,
public val isSystemTask: Output? = null,
public val location: Output? = null,
public val logTemplate: Output? = null,
public val platform: Output? = null,
public val registryName: Output? = null,
public val resourceGroupName: Output? = null,
public val status: Output>? = null,
public val step: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy