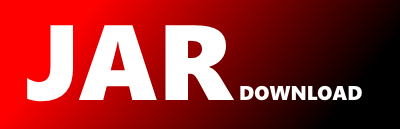
com.pulumi.azurenative.containerservice.kotlin.inputs.SysctlConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.containerservice.kotlin.inputs
import com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Sysctl settings for Linux agent nodes.
* @property fsAioMaxNr Sysctl setting fs.aio-max-nr.
* @property fsFileMax Sysctl setting fs.file-max.
* @property fsInotifyMaxUserWatches Sysctl setting fs.inotify.max_user_watches.
* @property fsNrOpen Sysctl setting fs.nr_open.
* @property kernelThreadsMax Sysctl setting kernel.threads-max.
* @property netCoreNetdevMaxBacklog Sysctl setting net.core.netdev_max_backlog.
* @property netCoreOptmemMax Sysctl setting net.core.optmem_max.
* @property netCoreRmemDefault Sysctl setting net.core.rmem_default.
* @property netCoreRmemMax Sysctl setting net.core.rmem_max.
* @property netCoreSomaxconn Sysctl setting net.core.somaxconn.
* @property netCoreWmemDefault Sysctl setting net.core.wmem_default.
* @property netCoreWmemMax Sysctl setting net.core.wmem_max.
* @property netIpv4IpLocalPortRange Sysctl setting net.ipv4.ip_local_port_range.
* @property netIpv4NeighDefaultGcThresh1 Sysctl setting net.ipv4.neigh.default.gc_thresh1.
* @property netIpv4NeighDefaultGcThresh2 Sysctl setting net.ipv4.neigh.default.gc_thresh2.
* @property netIpv4NeighDefaultGcThresh3 Sysctl setting net.ipv4.neigh.default.gc_thresh3.
* @property netIpv4TcpFinTimeout Sysctl setting net.ipv4.tcp_fin_timeout.
* @property netIpv4TcpKeepaliveProbes Sysctl setting net.ipv4.tcp_keepalive_probes.
* @property netIpv4TcpKeepaliveTime Sysctl setting net.ipv4.tcp_keepalive_time.
* @property netIpv4TcpMaxSynBacklog Sysctl setting net.ipv4.tcp_max_syn_backlog.
* @property netIpv4TcpMaxTwBuckets Sysctl setting net.ipv4.tcp_max_tw_buckets.
* @property netIpv4TcpTwReuse Sysctl setting net.ipv4.tcp_tw_reuse.
* @property netIpv4TcpkeepaliveIntvl Sysctl setting net.ipv4.tcp_keepalive_intvl.
* @property netNetfilterNfConntrackBuckets Sysctl setting net.netfilter.nf_conntrack_buckets.
* @property netNetfilterNfConntrackMax Sysctl setting net.netfilter.nf_conntrack_max.
* @property vmMaxMapCount Sysctl setting vm.max_map_count.
* @property vmSwappiness Sysctl setting vm.swappiness.
* @property vmVfsCachePressure Sysctl setting vm.vfs_cache_pressure.
*/
public data class SysctlConfigArgs(
public val fsAioMaxNr: Output? = null,
public val fsFileMax: Output? = null,
public val fsInotifyMaxUserWatches: Output? = null,
public val fsNrOpen: Output? = null,
public val kernelThreadsMax: Output? = null,
public val netCoreNetdevMaxBacklog: Output? = null,
public val netCoreOptmemMax: Output? = null,
public val netCoreRmemDefault: Output? = null,
public val netCoreRmemMax: Output? = null,
public val netCoreSomaxconn: Output? = null,
public val netCoreWmemDefault: Output? = null,
public val netCoreWmemMax: Output? = null,
public val netIpv4IpLocalPortRange: Output? = null,
public val netIpv4NeighDefaultGcThresh1: Output? = null,
public val netIpv4NeighDefaultGcThresh2: Output? = null,
public val netIpv4NeighDefaultGcThresh3: Output? = null,
public val netIpv4TcpFinTimeout: Output? = null,
public val netIpv4TcpKeepaliveProbes: Output? = null,
public val netIpv4TcpKeepaliveTime: Output? = null,
public val netIpv4TcpMaxSynBacklog: Output? = null,
public val netIpv4TcpMaxTwBuckets: Output? = null,
public val netIpv4TcpTwReuse: Output? = null,
public val netIpv4TcpkeepaliveIntvl: Output? = null,
public val netNetfilterNfConntrackBuckets: Output? = null,
public val netNetfilterNfConntrackMax: Output? = null,
public val vmMaxMapCount: Output? = null,
public val vmSwappiness: Output? = null,
public val vmVfsCachePressure: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs =
com.pulumi.azurenative.containerservice.inputs.SysctlConfigArgs.builder()
.fsAioMaxNr(fsAioMaxNr?.applyValue({ args0 -> args0 }))
.fsFileMax(fsFileMax?.applyValue({ args0 -> args0 }))
.fsInotifyMaxUserWatches(fsInotifyMaxUserWatches?.applyValue({ args0 -> args0 }))
.fsNrOpen(fsNrOpen?.applyValue({ args0 -> args0 }))
.kernelThreadsMax(kernelThreadsMax?.applyValue({ args0 -> args0 }))
.netCoreNetdevMaxBacklog(netCoreNetdevMaxBacklog?.applyValue({ args0 -> args0 }))
.netCoreOptmemMax(netCoreOptmemMax?.applyValue({ args0 -> args0 }))
.netCoreRmemDefault(netCoreRmemDefault?.applyValue({ args0 -> args0 }))
.netCoreRmemMax(netCoreRmemMax?.applyValue({ args0 -> args0 }))
.netCoreSomaxconn(netCoreSomaxconn?.applyValue({ args0 -> args0 }))
.netCoreWmemDefault(netCoreWmemDefault?.applyValue({ args0 -> args0 }))
.netCoreWmemMax(netCoreWmemMax?.applyValue({ args0 -> args0 }))
.netIpv4IpLocalPortRange(netIpv4IpLocalPortRange?.applyValue({ args0 -> args0 }))
.netIpv4NeighDefaultGcThresh1(netIpv4NeighDefaultGcThresh1?.applyValue({ args0 -> args0 }))
.netIpv4NeighDefaultGcThresh2(netIpv4NeighDefaultGcThresh2?.applyValue({ args0 -> args0 }))
.netIpv4NeighDefaultGcThresh3(netIpv4NeighDefaultGcThresh3?.applyValue({ args0 -> args0 }))
.netIpv4TcpFinTimeout(netIpv4TcpFinTimeout?.applyValue({ args0 -> args0 }))
.netIpv4TcpKeepaliveProbes(netIpv4TcpKeepaliveProbes?.applyValue({ args0 -> args0 }))
.netIpv4TcpKeepaliveTime(netIpv4TcpKeepaliveTime?.applyValue({ args0 -> args0 }))
.netIpv4TcpMaxSynBacklog(netIpv4TcpMaxSynBacklog?.applyValue({ args0 -> args0 }))
.netIpv4TcpMaxTwBuckets(netIpv4TcpMaxTwBuckets?.applyValue({ args0 -> args0 }))
.netIpv4TcpTwReuse(netIpv4TcpTwReuse?.applyValue({ args0 -> args0 }))
.netIpv4TcpkeepaliveIntvl(netIpv4TcpkeepaliveIntvl?.applyValue({ args0 -> args0 }))
.netNetfilterNfConntrackBuckets(netNetfilterNfConntrackBuckets?.applyValue({ args0 -> args0 }))
.netNetfilterNfConntrackMax(netNetfilterNfConntrackMax?.applyValue({ args0 -> args0 }))
.vmMaxMapCount(vmMaxMapCount?.applyValue({ args0 -> args0 }))
.vmSwappiness(vmSwappiness?.applyValue({ args0 -> args0 }))
.vmVfsCachePressure(vmVfsCachePressure?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SysctlConfigArgs].
*/
@PulumiTagMarker
public class SysctlConfigArgsBuilder internal constructor() {
private var fsAioMaxNr: Output? = null
private var fsFileMax: Output? = null
private var fsInotifyMaxUserWatches: Output? = null
private var fsNrOpen: Output? = null
private var kernelThreadsMax: Output? = null
private var netCoreNetdevMaxBacklog: Output? = null
private var netCoreOptmemMax: Output? = null
private var netCoreRmemDefault: Output? = null
private var netCoreRmemMax: Output? = null
private var netCoreSomaxconn: Output? = null
private var netCoreWmemDefault: Output? = null
private var netCoreWmemMax: Output? = null
private var netIpv4IpLocalPortRange: Output? = null
private var netIpv4NeighDefaultGcThresh1: Output? = null
private var netIpv4NeighDefaultGcThresh2: Output? = null
private var netIpv4NeighDefaultGcThresh3: Output? = null
private var netIpv4TcpFinTimeout: Output? = null
private var netIpv4TcpKeepaliveProbes: Output? = null
private var netIpv4TcpKeepaliveTime: Output? = null
private var netIpv4TcpMaxSynBacklog: Output? = null
private var netIpv4TcpMaxTwBuckets: Output? = null
private var netIpv4TcpTwReuse: Output? = null
private var netIpv4TcpkeepaliveIntvl: Output? = null
private var netNetfilterNfConntrackBuckets: Output? = null
private var netNetfilterNfConntrackMax: Output? = null
private var vmMaxMapCount: Output? = null
private var vmSwappiness: Output? = null
private var vmVfsCachePressure: Output? = null
/**
* @param value Sysctl setting fs.aio-max-nr.
*/
@JvmName("kpgjfroaktkrxoaw")
public suspend fun fsAioMaxNr(`value`: Output) {
this.fsAioMaxNr = value
}
/**
* @param value Sysctl setting fs.file-max.
*/
@JvmName("oipojltqfaoakche")
public suspend fun fsFileMax(`value`: Output) {
this.fsFileMax = value
}
/**
* @param value Sysctl setting fs.inotify.max_user_watches.
*/
@JvmName("bhjvulrbcqmdrheg")
public suspend fun fsInotifyMaxUserWatches(`value`: Output) {
this.fsInotifyMaxUserWatches = value
}
/**
* @param value Sysctl setting fs.nr_open.
*/
@JvmName("djlrhuwfevbnpksg")
public suspend fun fsNrOpen(`value`: Output) {
this.fsNrOpen = value
}
/**
* @param value Sysctl setting kernel.threads-max.
*/
@JvmName("tlmxfykbmfqojgjk")
public suspend fun kernelThreadsMax(`value`: Output) {
this.kernelThreadsMax = value
}
/**
* @param value Sysctl setting net.core.netdev_max_backlog.
*/
@JvmName("hutdyxhguklgxlsf")
public suspend fun netCoreNetdevMaxBacklog(`value`: Output) {
this.netCoreNetdevMaxBacklog = value
}
/**
* @param value Sysctl setting net.core.optmem_max.
*/
@JvmName("fwkexxmgpddslfxj")
public suspend fun netCoreOptmemMax(`value`: Output) {
this.netCoreOptmemMax = value
}
/**
* @param value Sysctl setting net.core.rmem_default.
*/
@JvmName("euakftcrqaocurtc")
public suspend fun netCoreRmemDefault(`value`: Output) {
this.netCoreRmemDefault = value
}
/**
* @param value Sysctl setting net.core.rmem_max.
*/
@JvmName("yrgtxyjrhqcxhtcb")
public suspend fun netCoreRmemMax(`value`: Output) {
this.netCoreRmemMax = value
}
/**
* @param value Sysctl setting net.core.somaxconn.
*/
@JvmName("dmqyeqssncsmqlbn")
public suspend fun netCoreSomaxconn(`value`: Output) {
this.netCoreSomaxconn = value
}
/**
* @param value Sysctl setting net.core.wmem_default.
*/
@JvmName("iknpmekmqoabuhhq")
public suspend fun netCoreWmemDefault(`value`: Output) {
this.netCoreWmemDefault = value
}
/**
* @param value Sysctl setting net.core.wmem_max.
*/
@JvmName("iobxtbgpaoqprgyc")
public suspend fun netCoreWmemMax(`value`: Output) {
this.netCoreWmemMax = value
}
/**
* @param value Sysctl setting net.ipv4.ip_local_port_range.
*/
@JvmName("cvdhyplajnwglcef")
public suspend fun netIpv4IpLocalPortRange(`value`: Output) {
this.netIpv4IpLocalPortRange = value
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh1.
*/
@JvmName("gemhjxkdaoklpsnd")
public suspend fun netIpv4NeighDefaultGcThresh1(`value`: Output) {
this.netIpv4NeighDefaultGcThresh1 = value
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh2.
*/
@JvmName("foccthfcvafgbqny")
public suspend fun netIpv4NeighDefaultGcThresh2(`value`: Output) {
this.netIpv4NeighDefaultGcThresh2 = value
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh3.
*/
@JvmName("amxmvgowwvkmuhgd")
public suspend fun netIpv4NeighDefaultGcThresh3(`value`: Output) {
this.netIpv4NeighDefaultGcThresh3 = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_fin_timeout.
*/
@JvmName("wnhmrvwbspxvcxdv")
public suspend fun netIpv4TcpFinTimeout(`value`: Output) {
this.netIpv4TcpFinTimeout = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_probes.
*/
@JvmName("kbennwvuqkycnjab")
public suspend fun netIpv4TcpKeepaliveProbes(`value`: Output) {
this.netIpv4TcpKeepaliveProbes = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_time.
*/
@JvmName("vfjslkjmyjltwptp")
public suspend fun netIpv4TcpKeepaliveTime(`value`: Output) {
this.netIpv4TcpKeepaliveTime = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_max_syn_backlog.
*/
@JvmName("bvvdkodskiwxoquu")
public suspend fun netIpv4TcpMaxSynBacklog(`value`: Output) {
this.netIpv4TcpMaxSynBacklog = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_max_tw_buckets.
*/
@JvmName("raxoibgnovvwilds")
public suspend fun netIpv4TcpMaxTwBuckets(`value`: Output) {
this.netIpv4TcpMaxTwBuckets = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_tw_reuse.
*/
@JvmName("gkdcfedbyrgpdygt")
public suspend fun netIpv4TcpTwReuse(`value`: Output) {
this.netIpv4TcpTwReuse = value
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_intvl.
*/
@JvmName("byjbtjcagehuehiq")
public suspend fun netIpv4TcpkeepaliveIntvl(`value`: Output) {
this.netIpv4TcpkeepaliveIntvl = value
}
/**
* @param value Sysctl setting net.netfilter.nf_conntrack_buckets.
*/
@JvmName("fwgvalbwvbdbkxkp")
public suspend fun netNetfilterNfConntrackBuckets(`value`: Output) {
this.netNetfilterNfConntrackBuckets = value
}
/**
* @param value Sysctl setting net.netfilter.nf_conntrack_max.
*/
@JvmName("eruoabuoqkvmuxwv")
public suspend fun netNetfilterNfConntrackMax(`value`: Output) {
this.netNetfilterNfConntrackMax = value
}
/**
* @param value Sysctl setting vm.max_map_count.
*/
@JvmName("ibfjlqnfjsjtugqf")
public suspend fun vmMaxMapCount(`value`: Output) {
this.vmMaxMapCount = value
}
/**
* @param value Sysctl setting vm.swappiness.
*/
@JvmName("ttncwhfwtftvejco")
public suspend fun vmSwappiness(`value`: Output) {
this.vmSwappiness = value
}
/**
* @param value Sysctl setting vm.vfs_cache_pressure.
*/
@JvmName("cosffrothtsagcpc")
public suspend fun vmVfsCachePressure(`value`: Output) {
this.vmVfsCachePressure = value
}
/**
* @param value Sysctl setting fs.aio-max-nr.
*/
@JvmName("lohdctwbvyipwaqy")
public suspend fun fsAioMaxNr(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fsAioMaxNr = mapped
}
/**
* @param value Sysctl setting fs.file-max.
*/
@JvmName("bcqspggombvcnpob")
public suspend fun fsFileMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fsFileMax = mapped
}
/**
* @param value Sysctl setting fs.inotify.max_user_watches.
*/
@JvmName("ycqbspbgehbhgxee")
public suspend fun fsInotifyMaxUserWatches(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fsInotifyMaxUserWatches = mapped
}
/**
* @param value Sysctl setting fs.nr_open.
*/
@JvmName("yrtwtblumbxosjwp")
public suspend fun fsNrOpen(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fsNrOpen = mapped
}
/**
* @param value Sysctl setting kernel.threads-max.
*/
@JvmName("dncwvvkbigmkwomq")
public suspend fun kernelThreadsMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kernelThreadsMax = mapped
}
/**
* @param value Sysctl setting net.core.netdev_max_backlog.
*/
@JvmName("ptwtnqpebggnpbxa")
public suspend fun netCoreNetdevMaxBacklog(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreNetdevMaxBacklog = mapped
}
/**
* @param value Sysctl setting net.core.optmem_max.
*/
@JvmName("mncscevbkmgjuuuh")
public suspend fun netCoreOptmemMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreOptmemMax = mapped
}
/**
* @param value Sysctl setting net.core.rmem_default.
*/
@JvmName("ilojsmgfibdqhcal")
public suspend fun netCoreRmemDefault(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreRmemDefault = mapped
}
/**
* @param value Sysctl setting net.core.rmem_max.
*/
@JvmName("kisomgldbsimchel")
public suspend fun netCoreRmemMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreRmemMax = mapped
}
/**
* @param value Sysctl setting net.core.somaxconn.
*/
@JvmName("bpmhepetoohwfhqw")
public suspend fun netCoreSomaxconn(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreSomaxconn = mapped
}
/**
* @param value Sysctl setting net.core.wmem_default.
*/
@JvmName("ydlgobqgyuwwltig")
public suspend fun netCoreWmemDefault(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreWmemDefault = mapped
}
/**
* @param value Sysctl setting net.core.wmem_max.
*/
@JvmName("hrhijnsshrqyrnxf")
public suspend fun netCoreWmemMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netCoreWmemMax = mapped
}
/**
* @param value Sysctl setting net.ipv4.ip_local_port_range.
*/
@JvmName("ojwnyugejfpfexiw")
public suspend fun netIpv4IpLocalPortRange(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4IpLocalPortRange = mapped
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh1.
*/
@JvmName("wgyepmtglqumputf")
public suspend fun netIpv4NeighDefaultGcThresh1(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4NeighDefaultGcThresh1 = mapped
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh2.
*/
@JvmName("hargsigscogaohxg")
public suspend fun netIpv4NeighDefaultGcThresh2(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4NeighDefaultGcThresh2 = mapped
}
/**
* @param value Sysctl setting net.ipv4.neigh.default.gc_thresh3.
*/
@JvmName("fpvxagnccaiftjmy")
public suspend fun netIpv4NeighDefaultGcThresh3(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4NeighDefaultGcThresh3 = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_fin_timeout.
*/
@JvmName("kstwsprjuujmyiwt")
public suspend fun netIpv4TcpFinTimeout(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpFinTimeout = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_probes.
*/
@JvmName("fqnmbjssgytcamyq")
public suspend fun netIpv4TcpKeepaliveProbes(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpKeepaliveProbes = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_time.
*/
@JvmName("aacxfgctdtfrcagm")
public suspend fun netIpv4TcpKeepaliveTime(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpKeepaliveTime = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_max_syn_backlog.
*/
@JvmName("odojtcayrwsmknfa")
public suspend fun netIpv4TcpMaxSynBacklog(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpMaxSynBacklog = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_max_tw_buckets.
*/
@JvmName("uudgxwdnmmyitckk")
public suspend fun netIpv4TcpMaxTwBuckets(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpMaxTwBuckets = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_tw_reuse.
*/
@JvmName("agiglggohccviaqu")
public suspend fun netIpv4TcpTwReuse(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpTwReuse = mapped
}
/**
* @param value Sysctl setting net.ipv4.tcp_keepalive_intvl.
*/
@JvmName("fupkcrvrynhtsjux")
public suspend fun netIpv4TcpkeepaliveIntvl(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netIpv4TcpkeepaliveIntvl = mapped
}
/**
* @param value Sysctl setting net.netfilter.nf_conntrack_buckets.
*/
@JvmName("uouyntoocfipywjp")
public suspend fun netNetfilterNfConntrackBuckets(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netNetfilterNfConntrackBuckets = mapped
}
/**
* @param value Sysctl setting net.netfilter.nf_conntrack_max.
*/
@JvmName("tpdudddjtrcnlicu")
public suspend fun netNetfilterNfConntrackMax(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.netNetfilterNfConntrackMax = mapped
}
/**
* @param value Sysctl setting vm.max_map_count.
*/
@JvmName("idrwivcexcodhxua")
public suspend fun vmMaxMapCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vmMaxMapCount = mapped
}
/**
* @param value Sysctl setting vm.swappiness.
*/
@JvmName("musrkpvaisodnunh")
public suspend fun vmSwappiness(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vmSwappiness = mapped
}
/**
* @param value Sysctl setting vm.vfs_cache_pressure.
*/
@JvmName("cpdqgnpybqohxruo")
public suspend fun vmVfsCachePressure(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vmVfsCachePressure = mapped
}
internal fun build(): SysctlConfigArgs = SysctlConfigArgs(
fsAioMaxNr = fsAioMaxNr,
fsFileMax = fsFileMax,
fsInotifyMaxUserWatches = fsInotifyMaxUserWatches,
fsNrOpen = fsNrOpen,
kernelThreadsMax = kernelThreadsMax,
netCoreNetdevMaxBacklog = netCoreNetdevMaxBacklog,
netCoreOptmemMax = netCoreOptmemMax,
netCoreRmemDefault = netCoreRmemDefault,
netCoreRmemMax = netCoreRmemMax,
netCoreSomaxconn = netCoreSomaxconn,
netCoreWmemDefault = netCoreWmemDefault,
netCoreWmemMax = netCoreWmemMax,
netIpv4IpLocalPortRange = netIpv4IpLocalPortRange,
netIpv4NeighDefaultGcThresh1 = netIpv4NeighDefaultGcThresh1,
netIpv4NeighDefaultGcThresh2 = netIpv4NeighDefaultGcThresh2,
netIpv4NeighDefaultGcThresh3 = netIpv4NeighDefaultGcThresh3,
netIpv4TcpFinTimeout = netIpv4TcpFinTimeout,
netIpv4TcpKeepaliveProbes = netIpv4TcpKeepaliveProbes,
netIpv4TcpKeepaliveTime = netIpv4TcpKeepaliveTime,
netIpv4TcpMaxSynBacklog = netIpv4TcpMaxSynBacklog,
netIpv4TcpMaxTwBuckets = netIpv4TcpMaxTwBuckets,
netIpv4TcpTwReuse = netIpv4TcpTwReuse,
netIpv4TcpkeepaliveIntvl = netIpv4TcpkeepaliveIntvl,
netNetfilterNfConntrackBuckets = netNetfilterNfConntrackBuckets,
netNetfilterNfConntrackMax = netNetfilterNfConntrackMax,
vmMaxMapCount = vmMaxMapCount,
vmSwappiness = vmSwappiness,
vmVfsCachePressure = vmVfsCachePressure,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy