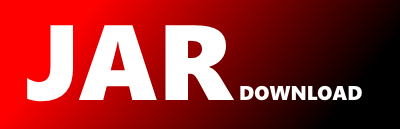
com.pulumi.azurenative.costmanagement.kotlin.Export.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.costmanagement.kotlin
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportDefinitionResponse
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportDeliveryInfoResponse
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportExecutionListResultResponse
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportScheduleResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportDefinitionResponse.Companion.toKotlin as exportDefinitionResponseToKotlin
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportDeliveryInfoResponse.Companion.toKotlin as exportDeliveryInfoResponseToKotlin
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportExecutionListResultResponse.Companion.toKotlin as exportExecutionListResultResponseToKotlin
import com.pulumi.azurenative.costmanagement.kotlin.outputs.ExportScheduleResponse.Companion.toKotlin as exportScheduleResponseToKotlin
/**
* Builder for [Export].
*/
@PulumiTagMarker
public class ExportResourceBuilder internal constructor() {
public var name: String? = null
public var args: ExportArgs = ExportArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ExportArgsBuilder.() -> Unit) {
val builder = ExportArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Export {
val builtJavaResource = com.pulumi.azurenative.costmanagement.Export(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Export(builtJavaResource)
}
}
/**
* An export resource.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2020-06-01.
* Other available API versions: 2019-10-01, 2023-04-01-preview, 2023-07-01-preview, 2023-08-01, 2023-09-01, 2023-11-01.
* ## Example Usage
* ### ExportCreateOrUpdateByBillingAccount
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "providers/Microsoft.Billing/billingAccounts/123456",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("providers/Microsoft.Billing/billingAccounts/123456"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("providers/Microsoft.Billing/billingAccounts/123456")
* .build());
* }
* }
* ```
* ### ExportCreateOrUpdateByDepartment
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "providers/Microsoft.Billing/billingAccounts/12/departments/1234",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("providers/Microsoft.Billing/billingAccounts/12/departments/1234"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("providers/Microsoft.Billing/billingAccounts/12/departments/1234")
* .build());
* }
* }
* ```
* ### ExportCreateOrUpdateByEnrollmentAccount
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "providers/Microsoft.Billing/billingAccounts/100/enrollmentAccounts/456",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("providers/Microsoft.Billing/billingAccounts/100/enrollmentAccounts/456"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("providers/Microsoft.Billing/billingAccounts/100/enrollmentAccounts/456")
* .build());
* }
* }
* ```
* ### ExportCreateOrUpdateByManagementGroup
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "providers/Microsoft.Management/managementGroups/TestMG",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("providers/Microsoft.Management/managementGroups/TestMG"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("providers/Microsoft.Management/managementGroups/TestMG")
* .build());
* }
* }
* ```
* ### ExportCreateOrUpdateByResourceGroup
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG")
* .build());
* }
* }
* ```
* ### ExportCreateOrUpdateBySubscription
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var export = new AzureNative.CostManagement.Export("export", new()
* {
* Definition = new AzureNative.CostManagement.Inputs.ExportDefinitionArgs
* {
* DataSet = new AzureNative.CostManagement.Inputs.ExportDatasetArgs
* {
* Configuration = new AzureNative.CostManagement.Inputs.ExportDatasetConfigurationArgs
* {
* Columns = new[]
* {
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity",
* },
* },
* Granularity = AzureNative.CostManagement.GranularityType.Daily,
* },
* Timeframe = AzureNative.CostManagement.TimeframeType.MonthToDate,
* Type = AzureNative.CostManagement.ExportType.ActualCost,
* },
* DeliveryInfo = new AzureNative.CostManagement.Inputs.ExportDeliveryInfoArgs
* {
* Destination = new AzureNative.CostManagement.Inputs.ExportDeliveryDestinationArgs
* {
* Container = "exports",
* ResourceId = "/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182",
* RootFolderPath = "ad-hoc",
* },
* },
* ExportName = "TestExport",
* Format = AzureNative.CostManagement.FormatType.Csv,
* Schedule = new AzureNative.CostManagement.Inputs.ExportScheduleArgs
* {
* Recurrence = AzureNative.CostManagement.RecurrenceType.Weekly,
* RecurrencePeriod = new AzureNative.CostManagement.Inputs.ExportRecurrencePeriodArgs
* {
* From = "2020-06-01T00:00:00Z",
* To = "2020-10-31T00:00:00Z",
* },
* Status = AzureNative.CostManagement.StatusType.Active,
* },
* Scope = "subscriptions/00000000-0000-0000-0000-000000000000",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewExport(ctx, "export", &costmanagement.ExportArgs{
* Definition: &costmanagement.ExportDefinitionArgs{
* DataSet: &costmanagement.ExportDatasetArgs{
* Configuration: &costmanagement.ExportDatasetConfigurationArgs{
* Columns: pulumi.StringArray{
* pulumi.String("Date"),
* pulumi.String("MeterId"),
* pulumi.String("ResourceId"),
* pulumi.String("ResourceLocation"),
* pulumi.String("Quantity"),
* },
* },
* Granularity: pulumi.String(costmanagement.GranularityTypeDaily),
* },
* Timeframe: pulumi.String(costmanagement.TimeframeTypeMonthToDate),
* Type: pulumi.String(costmanagement.ExportTypeActualCost),
* },
* DeliveryInfo: &costmanagement.ExportDeliveryInfoArgs{
* Destination: &costmanagement.ExportDeliveryDestinationArgs{
* Container: pulumi.String("exports"),
* ResourceId: pulumi.String("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182"),
* RootFolderPath: pulumi.String("ad-hoc"),
* },
* },
* ExportName: pulumi.String("TestExport"),
* Format: pulumi.String(costmanagement.FormatTypeCsv),
* Schedule: &costmanagement.ExportScheduleArgs{
* Recurrence: pulumi.String(costmanagement.RecurrenceTypeWeekly),
* RecurrencePeriod: &costmanagement.ExportRecurrencePeriodArgs{
* From: pulumi.String("2020-06-01T00:00:00Z"),
* To: pulumi.String("2020-10-31T00:00:00Z"),
* },
* Status: pulumi.String(costmanagement.StatusTypeActive),
* },
* Scope: pulumi.String("subscriptions/00000000-0000-0000-0000-000000000000"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.Export;
* import com.pulumi.azurenative.costmanagement.ExportArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDefinitionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDatasetConfigurationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryInfoArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportDeliveryDestinationArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportScheduleArgs;
* import com.pulumi.azurenative.costmanagement.inputs.ExportRecurrencePeriodArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var export = new Export("export", ExportArgs.builder()
* .definition(ExportDefinitionArgs.builder()
* .dataSet(ExportDatasetArgs.builder()
* .configuration(ExportDatasetConfigurationArgs.builder()
* .columns(
* "Date",
* "MeterId",
* "ResourceId",
* "ResourceLocation",
* "Quantity")
* .build())
* .granularity("Daily")
* .build())
* .timeframe("MonthToDate")
* .type("ActualCost")
* .build())
* .deliveryInfo(ExportDeliveryInfoArgs.builder()
* .destination(ExportDeliveryDestinationArgs.builder()
* .container("exports")
* .resourceId("/subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/MYDEVTESTRG/providers/Microsoft.Storage/storageAccounts/ccmeastusdiag182")
* .rootFolderPath("ad-hoc")
* .build())
* .build())
* .exportName("TestExport")
* .format("Csv")
* .schedule(ExportScheduleArgs.builder()
* .recurrence("Weekly")
* .recurrencePeriod(ExportRecurrencePeriodArgs.builder()
* .from("2020-06-01T00:00:00Z")
* .to("2020-10-31T00:00:00Z")
* .build())
* .status("Active")
* .build())
* .scope("subscriptions/00000000-0000-0000-0000-000000000000")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:costmanagement:Export TestExport /{scope}/providers/Microsoft.CostManagement/exports/{exportName}
* ```
*/
public class Export internal constructor(
override val javaResource: com.pulumi.azurenative.costmanagement.Export,
) : KotlinCustomResource(javaResource, ExportMapper) {
/**
* Has the definition for the export.
*/
public val definition: Output
get() = javaResource.definition().applyValue({ args0 ->
args0.let({ args0 ->
exportDefinitionResponseToKotlin(args0)
})
})
/**
* Has delivery information for the export.
*/
public val deliveryInfo: Output
get() = javaResource.deliveryInfo().applyValue({ args0 ->
args0.let({ args0 ->
exportDeliveryInfoResponseToKotlin(args0)
})
})
/**
* eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
*/
public val eTag: Output?
get() = javaResource.eTag().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The format of the export being delivered. Currently only 'Csv' is supported.
*/
public val format: Output?
get() = javaResource.format().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Resource name.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* If the export has an active schedule, provides an estimate of the next run time.
*/
public val nextRunTimeEstimate: Output
get() = javaResource.nextRunTimeEstimate().applyValue({ args0 -> args0 })
/**
* If set to true, exported data will be partitioned by size and placed in a blob directory together with a manifest file. Note: this option is currently available only for Microsoft Customer Agreement commerce scopes.
*/
public val partitionData: Output?
get() = javaResource.partitionData().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If requested, has the most recent run history for the export.
*/
public val runHistory: Output?
get() = javaResource.runHistory().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
exportExecutionListResultResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Has schedule information for the export.
*/
public val schedule: Output?
get() = javaResource.schedule().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
exportScheduleResponseToKotlin(args0)
})
}).orElse(null)
})
/**
* Resource type.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object ExportMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.costmanagement.Export::class == javaResource::class
override fun map(javaResource: Resource): Export = Export(
javaResource as
com.pulumi.azurenative.costmanagement.Export,
)
}
/**
* @see [Export].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Export].
*/
public suspend fun export(name: String, block: suspend ExportResourceBuilder.() -> Unit): Export {
val builder = ExportResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Export].
* @param name The _unique_ name of the resulting resource.
*/
public fun export(name: String): Export {
val builder = ExportResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy