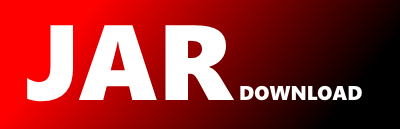
com.pulumi.azurenative.costmanagement.kotlin.ScheduledActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.costmanagement.kotlin
import com.pulumi.azurenative.costmanagement.ScheduledActionArgs.builder
import com.pulumi.azurenative.costmanagement.kotlin.enums.ScheduledActionKind
import com.pulumi.azurenative.costmanagement.kotlin.enums.ScheduledActionStatus
import com.pulumi.azurenative.costmanagement.kotlin.inputs.FileDestinationArgs
import com.pulumi.azurenative.costmanagement.kotlin.inputs.FileDestinationArgsBuilder
import com.pulumi.azurenative.costmanagement.kotlin.inputs.NotificationPropertiesArgs
import com.pulumi.azurenative.costmanagement.kotlin.inputs.NotificationPropertiesArgsBuilder
import com.pulumi.azurenative.costmanagement.kotlin.inputs.SchedulePropertiesArgs
import com.pulumi.azurenative.costmanagement.kotlin.inputs.SchedulePropertiesArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Scheduled action definition.
* Azure REST API version: 2023-03-01. Prior API version in Azure Native 1.x: 2022-04-01-preview.
* Other available API versions: 2023-04-01-preview, 2023-07-01-preview, 2023-08-01, 2023-09-01, 2023-11-01.
* ## Example Usage
* ### CreateOrUpdatePrivateScheduledAction
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var scheduledAction = new AzureNative.CostManagement.ScheduledAction("scheduledAction", new()
* {
* DisplayName = "Monthly Cost By Resource",
* Kind = AzureNative.CostManagement.ScheduledActionKind.Email,
* Name = "monthlyCostByResource",
* Notification = new AzureNative.CostManagement.Inputs.NotificationPropertiesArgs
* {
* Subject = "Cost by resource this month",
* To = new[]
* {
* "[email protected]",
* "[email protected]",
* },
* },
* Schedule = new AzureNative.CostManagement.Inputs.SchedulePropertiesArgs
* {
* DaysOfWeek = new[]
* {
* AzureNative.CostManagement.DaysOfWeek.Monday,
* },
* EndDate = "2021-06-19T22:21:51.1287144Z",
* Frequency = AzureNative.CostManagement.ScheduleFrequency.Monthly,
* HourOfDay = 10,
* StartDate = "2020-06-19T22:21:51.1287144Z",
* WeeksOfMonth = new[]
* {
* AzureNative.CostManagement.WeeksOfMonth.First,
* AzureNative.CostManagement.WeeksOfMonth.Third,
* },
* },
* Status = AzureNative.CostManagement.ScheduledActionStatus.Enabled,
* ViewId = "/providers/Microsoft.CostManagement/views/swaggerExample",
* });
* });
* ```
* ```go
* package main
* import (
* costmanagement "github.com/pulumi/pulumi-azure-native-sdk/costmanagement/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := costmanagement.NewScheduledAction(ctx, "scheduledAction", &costmanagement.ScheduledActionArgs{
* DisplayName: pulumi.String("Monthly Cost By Resource"),
* Kind: pulumi.String(costmanagement.ScheduledActionKindEmail),
* Name: pulumi.String("monthlyCostByResource"),
* Notification: &costmanagement.NotificationPropertiesArgs{
* Subject: pulumi.String("Cost by resource this month"),
* To: pulumi.StringArray{
* pulumi.String("[email protected]"),
* pulumi.String("[email protected]"),
* },
* },
* Schedule: &costmanagement.SchedulePropertiesArgs{
* DaysOfWeek: pulumi.StringArray{
* pulumi.String(costmanagement.DaysOfWeekMonday),
* },
* EndDate: pulumi.String("2021-06-19T22:21:51.1287144Z"),
* Frequency: pulumi.String(costmanagement.ScheduleFrequencyMonthly),
* HourOfDay: pulumi.Int(10),
* StartDate: pulumi.String("2020-06-19T22:21:51.1287144Z"),
* WeeksOfMonth: pulumi.StringArray{
* pulumi.String(costmanagement.WeeksOfMonthFirst),
* pulumi.String(costmanagement.WeeksOfMonthThird),
* },
* },
* Status: pulumi.String(costmanagement.ScheduledActionStatusEnabled),
* ViewId: pulumi.String("/providers/Microsoft.CostManagement/views/swaggerExample"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.costmanagement.ScheduledAction;
* import com.pulumi.azurenative.costmanagement.ScheduledActionArgs;
* import com.pulumi.azurenative.costmanagement.inputs.NotificationPropertiesArgs;
* import com.pulumi.azurenative.costmanagement.inputs.SchedulePropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var scheduledAction = new ScheduledAction("scheduledAction", ScheduledActionArgs.builder()
* .displayName("Monthly Cost By Resource")
* .kind("Email")
* .name("monthlyCostByResource")
* .notification(NotificationPropertiesArgs.builder()
* .subject("Cost by resource this month")
* .to(
* "[email protected]",
* "[email protected]")
* .build())
* .schedule(SchedulePropertiesArgs.builder()
* .daysOfWeek("Monday")
* .endDate("2021-06-19T22:21:51.1287144Z")
* .frequency("Monthly")
* .hourOfDay(10)
* .startDate("2020-06-19T22:21:51.1287144Z")
* .weeksOfMonth(
* "First",
* "Third")
* .build())
* .status("Enabled")
* .viewId("/providers/Microsoft.CostManagement/views/swaggerExample")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:costmanagement:ScheduledAction monthlyCostByResource /providers/Microsoft.CostManagement/scheduledActions/{name}
* ```
* @property displayName Scheduled action name.
* @property fileDestination Destination format of the view data. This is optional.
* @property kind Kind of the scheduled action.
* @property name Scheduled action name.
* @property notification Notification properties based on scheduled action kind.
* @property notificationEmail Email address of the point of contact that should get the unsubscribe requests and notification emails.
* @property schedule Schedule of the scheduled action.
* @property scope For private scheduled action(Create or Update), scope will be empty.
For shared scheduled action(Create or Update By Scope), Cost Management scope can be 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
* @property status Status of the scheduled action.
* @property viewId Cost analysis viewId used for scheduled action. For example, '/providers/Microsoft.CostManagement/views/swaggerExample'
*/
public data class ScheduledActionArgs(
public val displayName: Output? = null,
public val fileDestination: Output? = null,
public val kind: Output>? = null,
public val name: Output? = null,
public val notification: Output? = null,
public val notificationEmail: Output? = null,
public val schedule: Output? = null,
public val scope: Output? = null,
public val status: Output>? = null,
public val viewId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.costmanagement.ScheduledActionArgs =
com.pulumi.azurenative.costmanagement.ScheduledActionArgs.builder()
.displayName(displayName?.applyValue({ args0 -> args0 }))
.fileDestination(fileDestination?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kind(
kind?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.notification(notification?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.notificationEmail(notificationEmail?.applyValue({ args0 -> args0 }))
.schedule(schedule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scope(scope?.applyValue({ args0 -> args0 }))
.status(
status?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.viewId(viewId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScheduledActionArgs].
*/
@PulumiTagMarker
public class ScheduledActionArgsBuilder internal constructor() {
private var displayName: Output? = null
private var fileDestination: Output? = null
private var kind: Output>? = null
private var name: Output? = null
private var notification: Output? = null
private var notificationEmail: Output? = null
private var schedule: Output? = null
private var scope: Output? = null
private var status: Output>? = null
private var viewId: Output? = null
/**
* @param value Scheduled action name.
*/
@JvmName("ktkoimrgtcpojeld")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Destination format of the view data. This is optional.
*/
@JvmName("mjtbvbbyljeartew")
public suspend fun fileDestination(`value`: Output) {
this.fileDestination = value
}
/**
* @param value Kind of the scheduled action.
*/
@JvmName("yitcdxobxvwodwvo")
public suspend fun kind(`value`: Output>) {
this.kind = value
}
/**
* @param value Scheduled action name.
*/
@JvmName("xycbvspiqpqedjmt")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Notification properties based on scheduled action kind.
*/
@JvmName("vrpeqlafkyfajdgo")
public suspend fun notification(`value`: Output) {
this.notification = value
}
/**
* @param value Email address of the point of contact that should get the unsubscribe requests and notification emails.
*/
@JvmName("fkhmjapxkevcxkuj")
public suspend fun notificationEmail(`value`: Output) {
this.notificationEmail = value
}
/**
* @param value Schedule of the scheduled action.
*/
@JvmName("opdwtlvuadrlqsgf")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value For private scheduled action(Create or Update), scope will be empty.
For shared scheduled action(Create or Update By Scope), Cost Management scope can be 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*/
@JvmName("wnujlrbfervuylff")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value Status of the scheduled action.
*/
@JvmName("rxvduljlyuawspmy")
public suspend fun status(`value`: Output>) {
this.status = value
}
/**
* @param value Cost analysis viewId used for scheduled action. For example, '/providers/Microsoft.CostManagement/views/swaggerExample'
*/
@JvmName("mqnpatienodgigdr")
public suspend fun viewId(`value`: Output) {
this.viewId = value
}
/**
* @param value Scheduled action name.
*/
@JvmName("uoludbbisgkeggtu")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value Destination format of the view data. This is optional.
*/
@JvmName("fkkbpevvqmefpuof")
public suspend fun fileDestination(`value`: FileDestinationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileDestination = mapped
}
/**
* @param argument Destination format of the view data. This is optional.
*/
@JvmName("pbwermfsohcixvtt")
public suspend fun fileDestination(argument: suspend FileDestinationArgsBuilder.() -> Unit) {
val toBeMapped = FileDestinationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fileDestination = mapped
}
/**
* @param value Kind of the scheduled action.
*/
@JvmName("maqklhgpsmpjlirb")
public suspend fun kind(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Kind of the scheduled action.
*/
@JvmName("qfogsogiydehhntn")
public fun kind(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Kind of the scheduled action.
*/
@JvmName("gwblrhiwtbpwqpqx")
public fun kind(`value`: ScheduledActionKind) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Scheduled action name.
*/
@JvmName("wtqrownogtmbajdo")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Notification properties based on scheduled action kind.
*/
@JvmName("rovtbtnjugxopxqs")
public suspend fun notification(`value`: NotificationPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notification = mapped
}
/**
* @param argument Notification properties based on scheduled action kind.
*/
@JvmName("dyemqtcatcdjqcty")
public suspend fun notification(argument: suspend NotificationPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = NotificationPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.notification = mapped
}
/**
* @param value Email address of the point of contact that should get the unsubscribe requests and notification emails.
*/
@JvmName("pqnnuraklwyconpb")
public suspend fun notificationEmail(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notificationEmail = mapped
}
/**
* @param value Schedule of the scheduled action.
*/
@JvmName("xiwfbgqpobkibjjy")
public suspend fun schedule(`value`: SchedulePropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param argument Schedule of the scheduled action.
*/
@JvmName("wrtidsqvbqdrctpl")
public suspend fun schedule(argument: suspend SchedulePropertiesArgsBuilder.() -> Unit) {
val toBeMapped = SchedulePropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.schedule = mapped
}
/**
* @param value For private scheduled action(Create or Update), scope will be empty.
For shared scheduled action(Create or Update By Scope), Cost Management scope can be 'subscriptions/{subscriptionId}' for subscription scope, 'subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}' for resourceGroup scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}' for Billing Account scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/departments/{departmentId}' for Department scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/enrollmentAccounts/{enrollmentAccountId}' for EnrollmentAccount scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/billingProfiles/{billingProfileId}' for BillingProfile scope, 'providers/Microsoft.Billing/billingAccounts/{billingAccountId}/invoiceSections/{invoiceSectionId}' for InvoiceSection scope, '/providers/Microsoft.CostManagement/externalBillingAccounts/{externalBillingAccountName}' for ExternalBillingAccount scope, and '/providers/Microsoft.CostManagement/externalSubscriptions/{externalSubscriptionName}' for ExternalSubscription scope.
*/
@JvmName("rfyqcmxdxpbcnywf")
public suspend fun scope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scope = mapped
}
/**
* @param value Status of the scheduled action.
*/
@JvmName("rabteviqdcfgorhx")
public suspend fun status(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value Status of the scheduled action.
*/
@JvmName("bshwshiqyjatompg")
public fun status(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value Status of the scheduled action.
*/
@JvmName("gxvolyppmihgmbfr")
public fun status(`value`: ScheduledActionStatus) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param value Cost analysis viewId used for scheduled action. For example, '/providers/Microsoft.CostManagement/views/swaggerExample'
*/
@JvmName("behuiveudmogqndk")
public suspend fun viewId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.viewId = mapped
}
internal fun build(): ScheduledActionArgs = ScheduledActionArgs(
displayName = displayName,
fileDestination = fileDestination,
kind = kind,
name = name,
notification = notification,
notificationEmail = notificationEmail,
schedule = schedule,
scope = scope,
status = status,
viewId = viewId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy