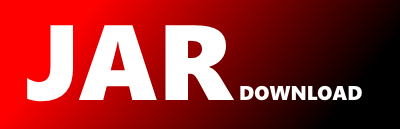
com.pulumi.azurenative.costmanagement.kotlin.outputs.GetBudgetResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.costmanagement.kotlin.outputs
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
/**
* A budget resource.
* @property amount The total amount of cost to track with the budget.
* Supported for CategoryType(s): Cost.
* Required for CategoryType(s): Cost.
* @property category The category of the budget.
* - 'Cost' defines a Budget.
* - 'ReservationUtilization' defines a Reservation Utilization Alert Rule.
* @property currentSpend The current amount of cost which is being tracked for a budget.
* Supported for CategoryType(s): Cost.
* @property eTag eTag of the resource. To handle concurrent update scenario, this field will be used to determine whether the user is updating the latest version or not.
* @property filter May be used to filter budgets by user-specified dimensions and/or tags.
* Supported for CategoryType(s): Cost, ReservationUtilization.
* @property forecastSpend The forecasted cost which is being tracked for a budget.
* Supported for CategoryType(s): Cost.
* @property id Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
* @property name The name of the resource
* @property notifications Dictionary of notifications associated with the budget.
* Supported for CategoryType(s): Cost, ReservationUtilization.
* - Constraints for **CategoryType: Cost** - Budget can have up to 5 notifications with thresholdType: Actual and 5 notifications with thresholdType: Forecasted.
* - Constraints for **CategoryType: ReservationUtilization** - Only one notification allowed. thresholdType is not applicable.
* @property timeGrain The time covered by a budget. Tracking of the amount will be reset based on the time grain.
* Supported for CategoryType(s): Cost, ReservationUtilization.
* Supported timeGrainTypes for **CategoryType: Cost**
* - Monthly
* - Quarterly
* - Annually
* - BillingMonth*
* - BillingQuarter*
* - BillingAnnual*
* *only supported for Web Direct customers.
* Supported timeGrainTypes for **CategoryType: ReservationUtilization**
* - Last7Days
* - Last30Days
* Required for CategoryType(s): Cost, ReservationUtilization.
* @property timePeriod The time period that defines the active period of the budget. The budget will evaluate data on or after the startDate and will expire on the endDate.
* Supported for CategoryType(s): Cost, ReservationUtilization.
* Required for CategoryType(s): Cost, ReservationUtilization.
* @property type The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public data class GetBudgetResult(
public val amount: Double? = null,
public val category: String,
public val currentSpend: CurrentSpendResponse,
public val eTag: String? = null,
public val filter: BudgetFilterResponse? = null,
public val forecastSpend: ForecastSpendResponse,
public val id: String,
public val name: String,
public val notifications: Map? = null,
public val timeGrain: String,
public val timePeriod: BudgetTimePeriodResponse,
public val type: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.costmanagement.outputs.GetBudgetResult): GetBudgetResult = GetBudgetResult(
amount = javaType.amount().map({ args0 -> args0 }).orElse(null),
category = javaType.category(),
currentSpend = javaType.currentSpend().let({ args0 ->
com.pulumi.azurenative.costmanagement.kotlin.outputs.CurrentSpendResponse.Companion.toKotlin(args0)
}),
eTag = javaType.eTag().map({ args0 -> args0 }).orElse(null),
filter = javaType.filter().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.costmanagement.kotlin.outputs.BudgetFilterResponse.Companion.toKotlin(args0)
})
}).orElse(null),
forecastSpend = javaType.forecastSpend().let({ args0 ->
com.pulumi.azurenative.costmanagement.kotlin.outputs.ForecastSpendResponse.Companion.toKotlin(args0)
}),
id = javaType.id(),
name = javaType.name(),
notifications = javaType.notifications().map({ args0 ->
args0.key.to(
args0.value.let({ args0 ->
com.pulumi.azurenative.costmanagement.kotlin.outputs.NotificationResponse.Companion.toKotlin(args0)
}),
)
}).toMap(),
timeGrain = javaType.timeGrain(),
timePeriod = javaType.timePeriod().let({ args0 ->
com.pulumi.azurenative.costmanagement.kotlin.outputs.BudgetTimePeriodResponse.Companion.toKotlin(args0)
}),
type = javaType.type(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy