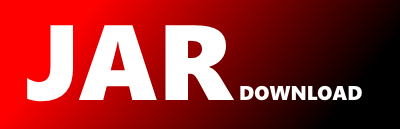
com.pulumi.azurenative.customerinsights.kotlin.ConnectorMappingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.customerinsights.kotlin
import com.pulumi.azurenative.customerinsights.ConnectorMappingArgs.builder
import com.pulumi.azurenative.customerinsights.kotlin.enums.ConnectorTypes
import com.pulumi.azurenative.customerinsights.kotlin.enums.EntityTypes
import com.pulumi.azurenative.customerinsights.kotlin.inputs.ConnectorMappingPropertiesArgs
import com.pulumi.azurenative.customerinsights.kotlin.inputs.ConnectorMappingPropertiesArgsBuilder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The connector mapping resource format.
* Azure REST API version: 2017-04-26. Prior API version in Azure Native 1.x: 2017-04-26.
* ## Example Usage
* ### ConnectorMappings_CreateOrUpdate
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var connectorMapping = new AzureNative.CustomerInsights.ConnectorMapping("connectorMapping", new()
* {
* ConnectorName = "testConnector8858",
* Description = "Test mapping",
* DisplayName = "testMapping12491",
* EntityType = AzureNative.CustomerInsights.EntityTypes.Interaction,
* EntityTypeName = "TestInteractionType2967",
* HubName = "sdkTestHub",
* MappingName = "testMapping12491",
* MappingProperties = new AzureNative.CustomerInsights.Inputs.ConnectorMappingPropertiesArgs
* {
* Availability = new AzureNative.CustomerInsights.Inputs.ConnectorMappingAvailabilityArgs
* {
* Frequency = AzureNative.CustomerInsights.FrequencyTypes.Hour,
* Interval = 5,
* },
* CompleteOperation = new AzureNative.CustomerInsights.Inputs.ConnectorMappingCompleteOperationArgs
* {
* CompletionOperationType = AzureNative.CustomerInsights.CompletionOperationTypes.DeleteFile,
* DestinationFolder = "fakePath",
* },
* ErrorManagement = new AzureNative.CustomerInsights.Inputs.ConnectorMappingErrorManagementArgs
* {
* ErrorLimit = 10,
* ErrorManagementType = AzureNative.CustomerInsights.ErrorManagementTypes.StopImport,
* },
* FileFilter = "unknown",
* FolderPath = "http://sample.dne/file",
* Format = new AzureNative.CustomerInsights.Inputs.ConnectorMappingFormatArgs
* {
* ColumnDelimiter = "|",
* FormatType = AzureNative.CustomerInsights.FormatTypes.TextFormat,
* },
* HasHeader = false,
* Structure = new[]
* {
* new AzureNative.CustomerInsights.Inputs.ConnectorMappingStructureArgs
* {
* ColumnName = "unknown1",
* IsEncrypted = false,
* PropertyName = "unknwon1",
* },
* new AzureNative.CustomerInsights.Inputs.ConnectorMappingStructureArgs
* {
* ColumnName = "unknown2",
* IsEncrypted = true,
* PropertyName = "unknwon2",
* },
* },
* },
* ResourceGroupName = "TestHubRG",
* });
* });
* ```
* ```go
* package main
* import (
* customerinsights "github.com/pulumi/pulumi-azure-native-sdk/customerinsights/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := customerinsights.NewConnectorMapping(ctx, "connectorMapping", &customerinsights.ConnectorMappingArgs{
* ConnectorName: pulumi.String("testConnector8858"),
* Description: pulumi.String("Test mapping"),
* DisplayName: pulumi.String("testMapping12491"),
* EntityType: customerinsights.EntityTypesInteraction,
* EntityTypeName: pulumi.String("TestInteractionType2967"),
* HubName: pulumi.String("sdkTestHub"),
* MappingName: pulumi.String("testMapping12491"),
* MappingProperties: &customerinsights.ConnectorMappingPropertiesArgs{
* Availability: &customerinsights.ConnectorMappingAvailabilityArgs{
* Frequency: customerinsights.FrequencyTypesHour,
* Interval: pulumi.Int(5),
* },
* CompleteOperation: &customerinsights.ConnectorMappingCompleteOperationArgs{
* CompletionOperationType: customerinsights.CompletionOperationTypesDeleteFile,
* DestinationFolder: pulumi.String("fakePath"),
* },
* ErrorManagement: &customerinsights.ConnectorMappingErrorManagementArgs{
* ErrorLimit: pulumi.Int(10),
* ErrorManagementType: customerinsights.ErrorManagementTypesStopImport,
* },
* FileFilter: pulumi.String("unknown"),
* FolderPath: pulumi.String("http://sample.dne/file"),
* Format: &customerinsights.ConnectorMappingFormatArgs{
* ColumnDelimiter: pulumi.String("|"),
* FormatType: customerinsights.FormatTypesTextFormat,
* },
* HasHeader: pulumi.Bool(false),
* Structure: customerinsights.ConnectorMappingStructureArray{
* &customerinsights.ConnectorMappingStructureArgs{
* ColumnName: pulumi.String("unknown1"),
* IsEncrypted: pulumi.Bool(false),
* PropertyName: pulumi.String("unknwon1"),
* },
* &customerinsights.ConnectorMappingStructureArgs{
* ColumnName: pulumi.String("unknown2"),
* IsEncrypted: pulumi.Bool(true),
* PropertyName: pulumi.String("unknwon2"),
* },
* },
* },
* ResourceGroupName: pulumi.String("TestHubRG"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.customerinsights.ConnectorMapping;
* import com.pulumi.azurenative.customerinsights.ConnectorMappingArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ConnectorMappingPropertiesArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ConnectorMappingAvailabilityArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ConnectorMappingCompleteOperationArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ConnectorMappingErrorManagementArgs;
* import com.pulumi.azurenative.customerinsights.inputs.ConnectorMappingFormatArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var connectorMapping = new ConnectorMapping("connectorMapping", ConnectorMappingArgs.builder()
* .connectorName("testConnector8858")
* .description("Test mapping")
* .displayName("testMapping12491")
* .entityType("Interaction")
* .entityTypeName("TestInteractionType2967")
* .hubName("sdkTestHub")
* .mappingName("testMapping12491")
* .mappingProperties(ConnectorMappingPropertiesArgs.builder()
* .availability(ConnectorMappingAvailabilityArgs.builder()
* .frequency("Hour")
* .interval(5)
* .build())
* .completeOperation(ConnectorMappingCompleteOperationArgs.builder()
* .completionOperationType("DeleteFile")
* .destinationFolder("fakePath")
* .build())
* .errorManagement(ConnectorMappingErrorManagementArgs.builder()
* .errorLimit(10)
* .errorManagementType("StopImport")
* .build())
* .fileFilter("unknown")
* .folderPath("http://sample.dne/file")
* .format(ConnectorMappingFormatArgs.builder()
* .columnDelimiter("|")
* .formatType("TextFormat")
* .build())
* .hasHeader(false)
* .structure(
* ConnectorMappingStructureArgs.builder()
* .columnName("unknown1")
* .isEncrypted(false)
* .propertyName("unknwon1")
* .build(),
* ConnectorMappingStructureArgs.builder()
* .columnName("unknown2")
* .isEncrypted(true)
* .propertyName("unknwon2")
* .build())
* .build())
* .resourceGroupName("TestHubRG")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:customerinsights:ConnectorMapping sdkTestHub/testConnector8858/testMapping12491 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.CustomerInsights/hubs/{hubName}/connectors/{connectorName}/mappings/{mappingName}
* ```
* @property connectorName The name of the connector.
* @property connectorType Type of connector.
* @property description The description of the connector mapping.
* @property displayName Display name for the connector mapping.
* @property entityType Defines which entity type the file should map to.
* @property entityTypeName The mapping entity name.
* @property hubName The name of the hub.
* @property mappingName The name of the connector mapping.
* @property mappingProperties The properties of the mapping.
* @property resourceGroupName The name of the resource group.
*/
public data class ConnectorMappingArgs(
public val connectorName: Output? = null,
public val connectorType: Output>? = null,
public val description: Output? = null,
public val displayName: Output? = null,
public val entityType: Output? = null,
public val entityTypeName: Output? = null,
public val hubName: Output? = null,
public val mappingName: Output? = null,
public val mappingProperties: Output? = null,
public val resourceGroupName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.customerinsights.ConnectorMappingArgs =
com.pulumi.azurenative.customerinsights.ConnectorMappingArgs.builder()
.connectorName(connectorName?.applyValue({ args0 -> args0 }))
.connectorType(
connectorType?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.displayName(displayName?.applyValue({ args0 -> args0 }))
.entityType(entityType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.entityTypeName(entityTypeName?.applyValue({ args0 -> args0 }))
.hubName(hubName?.applyValue({ args0 -> args0 }))
.mappingName(mappingName?.applyValue({ args0 -> args0 }))
.mappingProperties(mappingProperties?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectorMappingArgs].
*/
@PulumiTagMarker
public class ConnectorMappingArgsBuilder internal constructor() {
private var connectorName: Output? = null
private var connectorType: Output>? = null
private var description: Output? = null
private var displayName: Output? = null
private var entityType: Output? = null
private var entityTypeName: Output? = null
private var hubName: Output? = null
private var mappingName: Output? = null
private var mappingProperties: Output? = null
private var resourceGroupName: Output? = null
/**
* @param value The name of the connector.
*/
@JvmName("akbumdrrwxfvnxxo")
public suspend fun connectorName(`value`: Output) {
this.connectorName = value
}
/**
* @param value Type of connector.
*/
@JvmName("halbqcsjsywobcqc")
public suspend fun connectorType(`value`: Output>) {
this.connectorType = value
}
/**
* @param value The description of the connector mapping.
*/
@JvmName("wwjbwfbovaioyxnh")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Display name for the connector mapping.
*/
@JvmName("jskajxbbqarxyhsw")
public suspend fun displayName(`value`: Output) {
this.displayName = value
}
/**
* @param value Defines which entity type the file should map to.
*/
@JvmName("rmingjqqxlunhjrg")
public suspend fun entityType(`value`: Output) {
this.entityType = value
}
/**
* @param value The mapping entity name.
*/
@JvmName("tpapvxkqthijarbj")
public suspend fun entityTypeName(`value`: Output) {
this.entityTypeName = value
}
/**
* @param value The name of the hub.
*/
@JvmName("pjkkuckooetxqmoj")
public suspend fun hubName(`value`: Output) {
this.hubName = value
}
/**
* @param value The name of the connector mapping.
*/
@JvmName("kskhvcljgmrhbkku")
public suspend fun mappingName(`value`: Output) {
this.mappingName = value
}
/**
* @param value The properties of the mapping.
*/
@JvmName("jlugikbpiblybflm")
public suspend fun mappingProperties(`value`: Output) {
this.mappingProperties = value
}
/**
* @param value The name of the resource group.
*/
@JvmName("xaymgutyaukdnwvn")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the connector.
*/
@JvmName("bmrppfmxvfsixmur")
public suspend fun connectorName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorName = mapped
}
/**
* @param value Type of connector.
*/
@JvmName("ifbiabwyrvmfmlug")
public suspend fun connectorType(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectorType = mapped
}
/**
* @param value Type of connector.
*/
@JvmName("fecmmrhooiqknjql")
public fun connectorType(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.connectorType = mapped
}
/**
* @param value Type of connector.
*/
@JvmName("dwviltrlplaimqmk")
public fun connectorType(`value`: ConnectorTypes) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.connectorType = mapped
}
/**
* @param value The description of the connector mapping.
*/
@JvmName("peccvjrovsyadlkv")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Display name for the connector mapping.
*/
@JvmName("vkhexxgkvogrrmyq")
public suspend fun displayName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayName = mapped
}
/**
* @param value Defines which entity type the file should map to.
*/
@JvmName("jbbovjcrgqlgsilj")
public suspend fun entityType(`value`: EntityTypes?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityType = mapped
}
/**
* @param value The mapping entity name.
*/
@JvmName("anhgyjtkdlovmqox")
public suspend fun entityTypeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.entityTypeName = mapped
}
/**
* @param value The name of the hub.
*/
@JvmName("brnafckqqbrdowqg")
public suspend fun hubName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hubName = mapped
}
/**
* @param value The name of the connector mapping.
*/
@JvmName("bssyjolhgqladntu")
public suspend fun mappingName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mappingName = mapped
}
/**
* @param value The properties of the mapping.
*/
@JvmName("enrkpdgbcprxkgfs")
public suspend fun mappingProperties(`value`: ConnectorMappingPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mappingProperties = mapped
}
/**
* @param argument The properties of the mapping.
*/
@JvmName("stmkfaebhbqwhgir")
public suspend fun mappingProperties(argument: suspend ConnectorMappingPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = ConnectorMappingPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.mappingProperties = mapped
}
/**
* @param value The name of the resource group.
*/
@JvmName("bbidteuxosgaquvw")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
internal fun build(): ConnectorMappingArgs = ConnectorMappingArgs(
connectorName = connectorName,
connectorType = connectorType,
description = description,
displayName = displayName,
entityType = entityType,
entityTypeName = entityTypeName,
hubName = hubName,
mappingName = mappingName,
mappingProperties = mappingProperties,
resourceGroupName = resourceGroupName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy