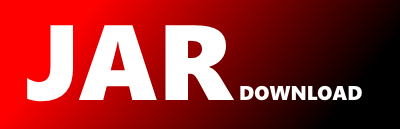
com.pulumi.azurenative.databox.kotlin.DataboxFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.databox.kotlin
import com.pulumi.azurenative.databox.DataboxFunctions.getJobPlain
import com.pulumi.azurenative.databox.DataboxFunctions.listJobCredentialsPlain
import com.pulumi.azurenative.databox.kotlin.inputs.GetJobPlainArgs
import com.pulumi.azurenative.databox.kotlin.inputs.GetJobPlainArgsBuilder
import com.pulumi.azurenative.databox.kotlin.inputs.ListJobCredentialsPlainArgs
import com.pulumi.azurenative.databox.kotlin.inputs.ListJobCredentialsPlainArgsBuilder
import com.pulumi.azurenative.databox.kotlin.outputs.GetJobResult
import com.pulumi.azurenative.databox.kotlin.outputs.ListJobCredentialsResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.databox.kotlin.outputs.GetJobResult.Companion.toKotlin as getJobResultToKotlin
import com.pulumi.azurenative.databox.kotlin.outputs.ListJobCredentialsResult.Companion.toKotlin as listJobCredentialsResultToKotlin
public object DataboxFunctions {
/**
* Gets information about the specified job.
* Azure REST API version: 2022-12-01.
* Other available API versions: 2019-09-01, 2023-03-01, 2023-12-01, 2024-02-01-preview, 2024-03-01-preview.
* @param argument null
* @return Job Resource.
*/
public suspend fun getJob(argument: GetJobPlainArgs): GetJobResult =
getJobResultToKotlin(getJobPlain(argument.toJava()).await())
/**
* @see [getJob].
* @param expand $expand is supported on details parameter for job, which provides details on the job stages.
* @param jobName The name of the job Resource within the specified resource group. job names must be between 3 and 24 characters in length and use any alphanumeric and underscore only
* @param resourceGroupName The Resource Group Name
* @return Job Resource.
*/
public suspend fun getJob(
expand: String? = null,
jobName: String,
resourceGroupName: String,
): GetJobResult {
val argument = GetJobPlainArgs(
expand = expand,
jobName = jobName,
resourceGroupName = resourceGroupName,
)
return getJobResultToKotlin(getJobPlain(argument.toJava()).await())
}
/**
* @see [getJob].
* @param argument Builder for [com.pulumi.azurenative.databox.kotlin.inputs.GetJobPlainArgs].
* @return Job Resource.
*/
public suspend fun getJob(argument: suspend GetJobPlainArgsBuilder.() -> Unit): GetJobResult {
val builder = GetJobPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getJobResultToKotlin(getJobPlain(builtArgument.toJava()).await())
}
/**
* This method gets the unencrypted secrets related to the job.
* Azure REST API version: 2022-12-01.
* Other available API versions: 2019-09-01, 2023-03-01, 2023-12-01, 2024-02-01-preview, 2024-03-01-preview.
* @param argument null
* @return List of unencrypted credentials for accessing device.
*/
public suspend fun listJobCredentials(argument: ListJobCredentialsPlainArgs): ListJobCredentialsResult =
listJobCredentialsResultToKotlin(listJobCredentialsPlain(argument.toJava()).await())
/**
* @see [listJobCredentials].
* @param jobName The name of the job Resource within the specified resource group. job names must be between 3 and 24 characters in length and use any alphanumeric and underscore only
* @param resourceGroupName The Resource Group Name
* @return List of unencrypted credentials for accessing device.
*/
public suspend fun listJobCredentials(jobName: String, resourceGroupName: String): ListJobCredentialsResult {
val argument = ListJobCredentialsPlainArgs(
jobName = jobName,
resourceGroupName = resourceGroupName,
)
return listJobCredentialsResultToKotlin(listJobCredentialsPlain(argument.toJava()).await())
}
/**
* @see [listJobCredentials].
* @param argument Builder for [com.pulumi.azurenative.databox.kotlin.inputs.ListJobCredentialsPlainArgs].
* @return List of unencrypted credentials for accessing device.
*/
public suspend fun listJobCredentials(argument: suspend ListJobCredentialsPlainArgsBuilder.() -> Unit): ListJobCredentialsResult {
val builder = ListJobCredentialsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return listJobCredentialsResultToKotlin(listJobCredentialsPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy