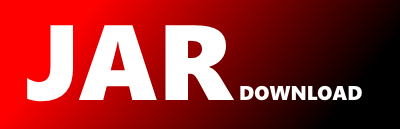
com.pulumi.azurenative.datafactory.kotlin.inputs.AzureBlobFSReadSettingsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datafactory.kotlin.inputs
import com.pulumi.azurenative.datafactory.inputs.AzureBlobFSReadSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Azure blobFS read settings.
* @property deleteFilesAfterCompletion Indicates whether the source files need to be deleted after copy completion. Default is false. Type: boolean (or Expression with resultType boolean).
* @property disableMetricsCollection If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
* @property enablePartitionDiscovery Indicates whether to enable partition discovery. Type: boolean (or Expression with resultType boolean).
* @property fileListPath Point to a text file that lists each file (relative path to the path configured in the dataset) that you want to copy. Type: string (or Expression with resultType string).
* @property maxConcurrentConnections The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
* @property modifiedDatetimeEnd The end of file's modified datetime. Type: string (or Expression with resultType string).
* @property modifiedDatetimeStart The start of file's modified datetime. Type: string (or Expression with resultType string).
* @property partitionRootPath Specify the root path where partition discovery starts from. Type: string (or Expression with resultType string).
* @property recursive If true, files under the folder path will be read recursively. Default is true. Type: boolean (or Expression with resultType boolean).
* @property type The read setting type.
* Expected value is 'AzureBlobFSReadSettings'.
* @property wildcardFileName Azure blobFS wildcardFileName. Type: string (or Expression with resultType string).
* @property wildcardFolderPath Azure blobFS wildcardFolderPath. Type: string (or Expression with resultType string).
*/
public data class AzureBlobFSReadSettingsArgs(
public val deleteFilesAfterCompletion: Output? = null,
public val disableMetricsCollection: Output? = null,
public val enablePartitionDiscovery: Output? = null,
public val fileListPath: Output? = null,
public val maxConcurrentConnections: Output? = null,
public val modifiedDatetimeEnd: Output? = null,
public val modifiedDatetimeStart: Output? = null,
public val partitionRootPath: Output? = null,
public val recursive: Output? = null,
public val type: Output,
public val wildcardFileName: Output? = null,
public val wildcardFolderPath: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.datafactory.inputs.AzureBlobFSReadSettingsArgs =
com.pulumi.azurenative.datafactory.inputs.AzureBlobFSReadSettingsArgs.builder()
.deleteFilesAfterCompletion(deleteFilesAfterCompletion?.applyValue({ args0 -> args0 }))
.disableMetricsCollection(disableMetricsCollection?.applyValue({ args0 -> args0 }))
.enablePartitionDiscovery(enablePartitionDiscovery?.applyValue({ args0 -> args0 }))
.fileListPath(fileListPath?.applyValue({ args0 -> args0 }))
.maxConcurrentConnections(maxConcurrentConnections?.applyValue({ args0 -> args0 }))
.modifiedDatetimeEnd(modifiedDatetimeEnd?.applyValue({ args0 -> args0 }))
.modifiedDatetimeStart(modifiedDatetimeStart?.applyValue({ args0 -> args0 }))
.partitionRootPath(partitionRootPath?.applyValue({ args0 -> args0 }))
.recursive(recursive?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 }))
.wildcardFileName(wildcardFileName?.applyValue({ args0 -> args0 }))
.wildcardFolderPath(wildcardFolderPath?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AzureBlobFSReadSettingsArgs].
*/
@PulumiTagMarker
public class AzureBlobFSReadSettingsArgsBuilder internal constructor() {
private var deleteFilesAfterCompletion: Output? = null
private var disableMetricsCollection: Output? = null
private var enablePartitionDiscovery: Output? = null
private var fileListPath: Output? = null
private var maxConcurrentConnections: Output? = null
private var modifiedDatetimeEnd: Output? = null
private var modifiedDatetimeStart: Output? = null
private var partitionRootPath: Output? = null
private var recursive: Output? = null
private var type: Output? = null
private var wildcardFileName: Output? = null
private var wildcardFolderPath: Output? = null
/**
* @param value Indicates whether the source files need to be deleted after copy completion. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("mqlqdpibvdfhitep")
public suspend fun deleteFilesAfterCompletion(`value`: Output) {
this.deleteFilesAfterCompletion = value
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("scxscdtqlnrifbmx")
public suspend fun disableMetricsCollection(`value`: Output) {
this.disableMetricsCollection = value
}
/**
* @param value Indicates whether to enable partition discovery. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("rnqyemhesfnieoon")
public suspend fun enablePartitionDiscovery(`value`: Output) {
this.enablePartitionDiscovery = value
}
/**
* @param value Point to a text file that lists each file (relative path to the path configured in the dataset) that you want to copy. Type: string (or Expression with resultType string).
*/
@JvmName("mywutvgetiayyrcl")
public suspend fun fileListPath(`value`: Output) {
this.fileListPath = value
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("vglskceigwjcpbve")
public suspend fun maxConcurrentConnections(`value`: Output) {
this.maxConcurrentConnections = value
}
/**
* @param value The end of file's modified datetime. Type: string (or Expression with resultType string).
*/
@JvmName("reipbyoimxlfnyyo")
public suspend fun modifiedDatetimeEnd(`value`: Output) {
this.modifiedDatetimeEnd = value
}
/**
* @param value The start of file's modified datetime. Type: string (or Expression with resultType string).
*/
@JvmName("fwdqxbucfbfafxtc")
public suspend fun modifiedDatetimeStart(`value`: Output) {
this.modifiedDatetimeStart = value
}
/**
* @param value Specify the root path where partition discovery starts from. Type: string (or Expression with resultType string).
*/
@JvmName("fmysoauussnpodcp")
public suspend fun partitionRootPath(`value`: Output) {
this.partitionRootPath = value
}
/**
* @param value If true, files under the folder path will be read recursively. Default is true. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("ryesfsrxbekkuali")
public suspend fun recursive(`value`: Output) {
this.recursive = value
}
/**
* @param value The read setting type.
* Expected value is 'AzureBlobFSReadSettings'.
*/
@JvmName("wvhsrieqkauspegs")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Azure blobFS wildcardFileName. Type: string (or Expression with resultType string).
*/
@JvmName("xeshyfueqwmnaucw")
public suspend fun wildcardFileName(`value`: Output) {
this.wildcardFileName = value
}
/**
* @param value Azure blobFS wildcardFolderPath. Type: string (or Expression with resultType string).
*/
@JvmName("tlxolggtfgagowbc")
public suspend fun wildcardFolderPath(`value`: Output) {
this.wildcardFolderPath = value
}
/**
* @param value Indicates whether the source files need to be deleted after copy completion. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("qpkoirlfkjoqvuuk")
public suspend fun deleteFilesAfterCompletion(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteFilesAfterCompletion = mapped
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("esbiokqnqhokillk")
public suspend fun disableMetricsCollection(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableMetricsCollection = mapped
}
/**
* @param value Indicates whether to enable partition discovery. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("lgrikqaebihfonvp")
public suspend fun enablePartitionDiscovery(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePartitionDiscovery = mapped
}
/**
* @param value Point to a text file that lists each file (relative path to the path configured in the dataset) that you want to copy. Type: string (or Expression with resultType string).
*/
@JvmName("olmfklvnswutfitv")
public suspend fun fileListPath(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fileListPath = mapped
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("wlddjcnigiqocrok")
public suspend fun maxConcurrentConnections(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConcurrentConnections = mapped
}
/**
* @param value The end of file's modified datetime. Type: string (or Expression with resultType string).
*/
@JvmName("tfoqrcallbeatidx")
public suspend fun modifiedDatetimeEnd(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.modifiedDatetimeEnd = mapped
}
/**
* @param value The start of file's modified datetime. Type: string (or Expression with resultType string).
*/
@JvmName("frhpegyiyfgstgym")
public suspend fun modifiedDatetimeStart(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.modifiedDatetimeStart = mapped
}
/**
* @param value Specify the root path where partition discovery starts from. Type: string (or Expression with resultType string).
*/
@JvmName("ddfhwdortxteohcn")
public suspend fun partitionRootPath(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partitionRootPath = mapped
}
/**
* @param value If true, files under the folder path will be read recursively. Default is true. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("qwxqliucpuukyebn")
public suspend fun recursive(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recursive = mapped
}
/**
* @param value The read setting type.
* Expected value is 'AzureBlobFSReadSettings'.
*/
@JvmName("qjlsyqbqykwcbjjd")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value Azure blobFS wildcardFileName. Type: string (or Expression with resultType string).
*/
@JvmName("tffdnjqfouaaymay")
public suspend fun wildcardFileName(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wildcardFileName = mapped
}
/**
* @param value Azure blobFS wildcardFolderPath. Type: string (or Expression with resultType string).
*/
@JvmName("khoilmbbxtfdjpcm")
public suspend fun wildcardFolderPath(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.wildcardFolderPath = mapped
}
internal fun build(): AzureBlobFSReadSettingsArgs = AzureBlobFSReadSettingsArgs(
deleteFilesAfterCompletion = deleteFilesAfterCompletion,
disableMetricsCollection = disableMetricsCollection,
enablePartitionDiscovery = enablePartitionDiscovery,
fileListPath = fileListPath,
maxConcurrentConnections = maxConcurrentConnections,
modifiedDatetimeEnd = modifiedDatetimeEnd,
modifiedDatetimeStart = modifiedDatetimeStart,
partitionRootPath = partitionRootPath,
recursive = recursive,
type = type ?: throw PulumiNullFieldException("type"),
wildcardFileName = wildcardFileName,
wildcardFolderPath = wildcardFolderPath,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy