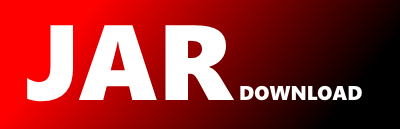
com.pulumi.azurenative.datafactory.kotlin.inputs.AzureMLLinkedServiceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datafactory.kotlin.inputs
import com.pulumi.azurenative.datafactory.inputs.AzureMLLinkedServiceArgs.builder
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Azure ML Studio Web Service linked service.
* @property annotations List of tags that can be used for describing the linked service.
* @property apiKey The API key for accessing the Azure ML model endpoint.
* @property authentication Type of authentication (Required to specify MSI) used to connect to AzureML. Type: string (or Expression with resultType string).
* @property connectVia The integration runtime reference.
* @property description Linked service description.
* @property encryptedCredential The encrypted credential used for authentication. Credentials are encrypted using the integration runtime credential manager. Type: string.
* @property mlEndpoint The Batch Execution REST URL for an Azure ML Studio Web Service endpoint. Type: string (or Expression with resultType string).
* @property parameters Parameters for linked service.
* @property servicePrincipalId The ID of the service principal used to authenticate against the ARM-based updateResourceEndpoint of an Azure ML Studio web service. Type: string (or Expression with resultType string).
* @property servicePrincipalKey The key of the service principal used to authenticate against the ARM-based updateResourceEndpoint of an Azure ML Studio web service.
* @property tenant The name or ID of the tenant to which the service principal belongs. Type: string (or Expression with resultType string).
* @property type Type of linked service.
* Expected value is 'AzureML'.
* @property updateResourceEndpoint The Update Resource REST URL for an Azure ML Studio Web Service endpoint. Type: string (or Expression with resultType string).
* @property version Version of the linked service.
*/
public data class AzureMLLinkedServiceArgs(
public val annotations: Output>? = null,
public val apiKey: Output>,
public val authentication: Output? = null,
public val connectVia: Output? = null,
public val description: Output? = null,
public val encryptedCredential: Output? = null,
public val mlEndpoint: Output,
public val parameters: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy