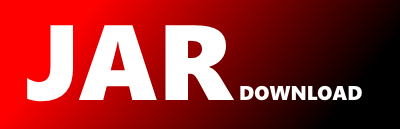
com.pulumi.azurenative.datafactory.kotlin.inputs.MongoDbV2SourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datafactory.kotlin.inputs
import com.pulumi.azurenative.datafactory.inputs.MongoDbV2SourceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A copy activity source for a MongoDB database.
* @property additionalColumns Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
* @property batchSize Specifies the number of documents to return in each batch of the response from MongoDB instance. In most cases, modifying the batch size will not affect the user or the application. This property's main purpose is to avoid hit the limitation of response size. Type: integer (or Expression with resultType integer).
* @property cursorMethods Cursor methods for Mongodb query
* @property disableMetricsCollection If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
* @property filter Specifies selection filter using query operators. To return all documents in a collection, omit this parameter or pass an empty document ({}). Type: string (or Expression with resultType string).
* @property maxConcurrentConnections The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
* @property queryTimeout Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
* @property sourceRetryCount Source retry count. Type: integer (or Expression with resultType integer).
* @property sourceRetryWait Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
* @property type Copy source type.
* Expected value is 'MongoDbV2Source'.
*/
public data class MongoDbV2SourceArgs(
public val additionalColumns: Output? = null,
public val batchSize: Output? = null,
public val cursorMethods: Output? = null,
public val disableMetricsCollection: Output? = null,
public val filter: Output? = null,
public val maxConcurrentConnections: Output? = null,
public val queryTimeout: Output? = null,
public val sourceRetryCount: Output? = null,
public val sourceRetryWait: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.datafactory.inputs.MongoDbV2SourceArgs =
com.pulumi.azurenative.datafactory.inputs.MongoDbV2SourceArgs.builder()
.additionalColumns(additionalColumns?.applyValue({ args0 -> args0 }))
.batchSize(batchSize?.applyValue({ args0 -> args0 }))
.cursorMethods(cursorMethods?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.disableMetricsCollection(disableMetricsCollection?.applyValue({ args0 -> args0 }))
.filter(filter?.applyValue({ args0 -> args0 }))
.maxConcurrentConnections(maxConcurrentConnections?.applyValue({ args0 -> args0 }))
.queryTimeout(queryTimeout?.applyValue({ args0 -> args0 }))
.sourceRetryCount(sourceRetryCount?.applyValue({ args0 -> args0 }))
.sourceRetryWait(sourceRetryWait?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MongoDbV2SourceArgs].
*/
@PulumiTagMarker
public class MongoDbV2SourceArgsBuilder internal constructor() {
private var additionalColumns: Output? = null
private var batchSize: Output? = null
private var cursorMethods: Output? = null
private var disableMetricsCollection: Output? = null
private var filter: Output? = null
private var maxConcurrentConnections: Output? = null
private var queryTimeout: Output? = null
private var sourceRetryCount: Output? = null
private var sourceRetryWait: Output? = null
private var type: Output? = null
/**
* @param value Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*/
@JvmName("hfcspoedskaycdel")
public suspend fun additionalColumns(`value`: Output) {
this.additionalColumns = value
}
/**
* @param value Specifies the number of documents to return in each batch of the response from MongoDB instance. In most cases, modifying the batch size will not affect the user or the application. This property's main purpose is to avoid hit the limitation of response size. Type: integer (or Expression with resultType integer).
*/
@JvmName("ipcsplolgwbelqpx")
public suspend fun batchSize(`value`: Output) {
this.batchSize = value
}
/**
* @param value Cursor methods for Mongodb query
*/
@JvmName("uvwvemgavfwnasip")
public suspend fun cursorMethods(`value`: Output) {
this.cursorMethods = value
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("bgbiyhaahmmpmuvs")
public suspend fun disableMetricsCollection(`value`: Output) {
this.disableMetricsCollection = value
}
/**
* @param value Specifies selection filter using query operators. To return all documents in a collection, omit this parameter or pass an empty document ({}). Type: string (or Expression with resultType string).
*/
@JvmName("nosasbbapitlhtga")
public suspend fun filter(`value`: Output) {
this.filter = value
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("falxijlntbayxlyd")
public suspend fun maxConcurrentConnections(`value`: Output) {
this.maxConcurrentConnections = value
}
/**
* @param value Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("eyufprrtnthxuuho")
public suspend fun queryTimeout(`value`: Output) {
this.queryTimeout = value
}
/**
* @param value Source retry count. Type: integer (or Expression with resultType integer).
*/
@JvmName("umgduntxtpnrdhtc")
public suspend fun sourceRetryCount(`value`: Output) {
this.sourceRetryCount = value
}
/**
* @param value Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("ltcasovuqmtattbr")
public suspend fun sourceRetryWait(`value`: Output) {
this.sourceRetryWait = value
}
/**
* @param value Copy source type.
* Expected value is 'MongoDbV2Source'.
*/
@JvmName("fuatahjjcnkmffel")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*/
@JvmName("oewkwpewmevoubru")
public suspend fun additionalColumns(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additionalColumns = mapped
}
/**
* @param value Specifies the number of documents to return in each batch of the response from MongoDB instance. In most cases, modifying the batch size will not affect the user or the application. This property's main purpose is to avoid hit the limitation of response size. Type: integer (or Expression with resultType integer).
*/
@JvmName("nkhlmpsruyvsurju")
public suspend fun batchSize(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.batchSize = mapped
}
/**
* @param value Cursor methods for Mongodb query
*/
@JvmName("crkcfybrlsfnbtpe")
public suspend fun cursorMethods(`value`: MongoDbCursorMethodsPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cursorMethods = mapped
}
/**
* @param argument Cursor methods for Mongodb query
*/
@JvmName("ddsvfjocgrjppxky")
public suspend fun cursorMethods(argument: suspend MongoDbCursorMethodsPropertiesArgsBuilder.() -> Unit) {
val toBeMapped = MongoDbCursorMethodsPropertiesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.cursorMethods = mapped
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("rhjgpysnoyjmpxam")
public suspend fun disableMetricsCollection(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableMetricsCollection = mapped
}
/**
* @param value Specifies selection filter using query operators. To return all documents in a collection, omit this parameter or pass an empty document ({}). Type: string (or Expression with resultType string).
*/
@JvmName("trxklxwrgrbgwqju")
public suspend fun filter(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filter = mapped
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("wocsaopjaqcnccky")
public suspend fun maxConcurrentConnections(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConcurrentConnections = mapped
}
/**
* @param value Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("wntmwimnstidedar")
public suspend fun queryTimeout(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryTimeout = mapped
}
/**
* @param value Source retry count. Type: integer (or Expression with resultType integer).
*/
@JvmName("vlwhijumtarytofc")
public suspend fun sourceRetryCount(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRetryCount = mapped
}
/**
* @param value Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("ahdaggenwhvgkmbv")
public suspend fun sourceRetryWait(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRetryWait = mapped
}
/**
* @param value Copy source type.
* Expected value is 'MongoDbV2Source'.
*/
@JvmName("aatgktmdynfmpjgo")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): MongoDbV2SourceArgs = MongoDbV2SourceArgs(
additionalColumns = additionalColumns,
batchSize = batchSize,
cursorMethods = cursorMethods,
disableMetricsCollection = disableMetricsCollection,
filter = filter,
maxConcurrentConnections = maxConcurrentConnections,
queryTimeout = queryTimeout,
sourceRetryCount = sourceRetryCount,
sourceRetryWait = sourceRetryWait,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy