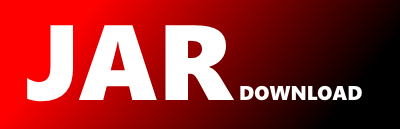
com.pulumi.azurenative.datafactory.kotlin.inputs.SapOpenHubSourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datafactory.kotlin.inputs
import com.pulumi.azurenative.datafactory.inputs.SapOpenHubSourceArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A copy activity source for SAP Business Warehouse Open Hub Destination source.
* @property additionalColumns Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
* @property baseRequestId The ID of request for delta loading. Once it is set, only data with requestId larger than the value of this property will be retrieved. The default value is 0. Type: integer (or Expression with resultType integer ).
* @property customRfcReadTableFunctionModule Specifies the custom RFC function module that will be used to read data from SAP Table. Type: string (or Expression with resultType string).
* @property disableMetricsCollection If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
* @property excludeLastRequest Whether to exclude the records of the last request. The default value is true. Type: boolean (or Expression with resultType boolean).
* @property maxConcurrentConnections The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
* @property queryTimeout Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
* @property sapDataColumnDelimiter The single character that will be used as delimiter passed to SAP RFC as well as splitting the output data retrieved. Type: string (or Expression with resultType string).
* @property sourceRetryCount Source retry count. Type: integer (or Expression with resultType integer).
* @property sourceRetryWait Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
* @property type Copy source type.
* Expected value is 'SapOpenHubSource'.
*/
public data class SapOpenHubSourceArgs(
public val additionalColumns: Output? = null,
public val baseRequestId: Output? = null,
public val customRfcReadTableFunctionModule: Output? = null,
public val disableMetricsCollection: Output? = null,
public val excludeLastRequest: Output? = null,
public val maxConcurrentConnections: Output? = null,
public val queryTimeout: Output? = null,
public val sapDataColumnDelimiter: Output? = null,
public val sourceRetryCount: Output? = null,
public val sourceRetryWait: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.datafactory.inputs.SapOpenHubSourceArgs =
com.pulumi.azurenative.datafactory.inputs.SapOpenHubSourceArgs.builder()
.additionalColumns(additionalColumns?.applyValue({ args0 -> args0 }))
.baseRequestId(baseRequestId?.applyValue({ args0 -> args0 }))
.customRfcReadTableFunctionModule(customRfcReadTableFunctionModule?.applyValue({ args0 -> args0 }))
.disableMetricsCollection(disableMetricsCollection?.applyValue({ args0 -> args0 }))
.excludeLastRequest(excludeLastRequest?.applyValue({ args0 -> args0 }))
.maxConcurrentConnections(maxConcurrentConnections?.applyValue({ args0 -> args0 }))
.queryTimeout(queryTimeout?.applyValue({ args0 -> args0 }))
.sapDataColumnDelimiter(sapDataColumnDelimiter?.applyValue({ args0 -> args0 }))
.sourceRetryCount(sourceRetryCount?.applyValue({ args0 -> args0 }))
.sourceRetryWait(sourceRetryWait?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SapOpenHubSourceArgs].
*/
@PulumiTagMarker
public class SapOpenHubSourceArgsBuilder internal constructor() {
private var additionalColumns: Output? = null
private var baseRequestId: Output? = null
private var customRfcReadTableFunctionModule: Output? = null
private var disableMetricsCollection: Output? = null
private var excludeLastRequest: Output? = null
private var maxConcurrentConnections: Output? = null
private var queryTimeout: Output? = null
private var sapDataColumnDelimiter: Output? = null
private var sourceRetryCount: Output? = null
private var sourceRetryWait: Output? = null
private var type: Output? = null
/**
* @param value Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*/
@JvmName("smwjweagcnxkpoga")
public suspend fun additionalColumns(`value`: Output) {
this.additionalColumns = value
}
/**
* @param value The ID of request for delta loading. Once it is set, only data with requestId larger than the value of this property will be retrieved. The default value is 0. Type: integer (or Expression with resultType integer ).
*/
@JvmName("awcjeoftybhkkshd")
public suspend fun baseRequestId(`value`: Output) {
this.baseRequestId = value
}
/**
* @param value Specifies the custom RFC function module that will be used to read data from SAP Table. Type: string (or Expression with resultType string).
*/
@JvmName("etgnpekquayfvsot")
public suspend fun customRfcReadTableFunctionModule(`value`: Output) {
this.customRfcReadTableFunctionModule = value
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("juhnwtcmbhkrbjvq")
public suspend fun disableMetricsCollection(`value`: Output) {
this.disableMetricsCollection = value
}
/**
* @param value Whether to exclude the records of the last request. The default value is true. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("unpnrckgpdaodnhq")
public suspend fun excludeLastRequest(`value`: Output) {
this.excludeLastRequest = value
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("nbmttvwfujerlsub")
public suspend fun maxConcurrentConnections(`value`: Output) {
this.maxConcurrentConnections = value
}
/**
* @param value Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("puxoqixlyavfycmw")
public suspend fun queryTimeout(`value`: Output) {
this.queryTimeout = value
}
/**
* @param value The single character that will be used as delimiter passed to SAP RFC as well as splitting the output data retrieved. Type: string (or Expression with resultType string).
*/
@JvmName("tfkkaijlnenkmrld")
public suspend fun sapDataColumnDelimiter(`value`: Output) {
this.sapDataColumnDelimiter = value
}
/**
* @param value Source retry count. Type: integer (or Expression with resultType integer).
*/
@JvmName("wiewtmnykgvxdgli")
public suspend fun sourceRetryCount(`value`: Output) {
this.sourceRetryCount = value
}
/**
* @param value Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("ftlcsmtsoiildpsl")
public suspend fun sourceRetryWait(`value`: Output) {
this.sourceRetryWait = value
}
/**
* @param value Copy source type.
* Expected value is 'SapOpenHubSource'.
*/
@JvmName("ffoystkcwpyslyyg")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Specifies the additional columns to be added to source data. Type: array of objects(AdditionalColumns) (or Expression with resultType array of objects).
*/
@JvmName("loejcubnmptpxucs")
public suspend fun additionalColumns(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.additionalColumns = mapped
}
/**
* @param value The ID of request for delta loading. Once it is set, only data with requestId larger than the value of this property will be retrieved. The default value is 0. Type: integer (or Expression with resultType integer ).
*/
@JvmName("ukfoycmrlppdhgna")
public suspend fun baseRequestId(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseRequestId = mapped
}
/**
* @param value Specifies the custom RFC function module that will be used to read data from SAP Table. Type: string (or Expression with resultType string).
*/
@JvmName("gjsejkhkokqvtmbm")
public suspend fun customRfcReadTableFunctionModule(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customRfcReadTableFunctionModule = mapped
}
/**
* @param value If true, disable data store metrics collection. Default is false. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("qvmvgjvbuasonjpb")
public suspend fun disableMetricsCollection(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.disableMetricsCollection = mapped
}
/**
* @param value Whether to exclude the records of the last request. The default value is true. Type: boolean (or Expression with resultType boolean).
*/
@JvmName("pdrxstobvxpvvvye")
public suspend fun excludeLastRequest(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.excludeLastRequest = mapped
}
/**
* @param value The maximum concurrent connection count for the source data store. Type: integer (or Expression with resultType integer).
*/
@JvmName("ydsgogrhlfkwasuy")
public suspend fun maxConcurrentConnections(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConcurrentConnections = mapped
}
/**
* @param value Query timeout. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("unsldighajdxjqdc")
public suspend fun queryTimeout(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.queryTimeout = mapped
}
/**
* @param value The single character that will be used as delimiter passed to SAP RFC as well as splitting the output data retrieved. Type: string (or Expression with resultType string).
*/
@JvmName("ssbthetfevfbhwuj")
public suspend fun sapDataColumnDelimiter(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sapDataColumnDelimiter = mapped
}
/**
* @param value Source retry count. Type: integer (or Expression with resultType integer).
*/
@JvmName("mvucreayiutomqes")
public suspend fun sourceRetryCount(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRetryCount = mapped
}
/**
* @param value Source retry wait. Type: string (or Expression with resultType string), pattern: ((\d+)\.)?(\d\d):(60|([0-5][0-9])):(60|([0-5][0-9])).
*/
@JvmName("hfrfgxyrtfweovsf")
public suspend fun sourceRetryWait(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceRetryWait = mapped
}
/**
* @param value Copy source type.
* Expected value is 'SapOpenHubSource'.
*/
@JvmName("mipxhulfgawqkiie")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): SapOpenHubSourceArgs = SapOpenHubSourceArgs(
additionalColumns = additionalColumns,
baseRequestId = baseRequestId,
customRfcReadTableFunctionModule = customRfcReadTableFunctionModule,
disableMetricsCollection = disableMetricsCollection,
excludeLastRequest = excludeLastRequest,
maxConcurrentConnections = maxConcurrentConnections,
queryTimeout = queryTimeout,
sapDataColumnDelimiter = sapDataColumnDelimiter,
sourceRetryCount = sourceRetryCount,
sourceRetryWait = sourceRetryWait,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy