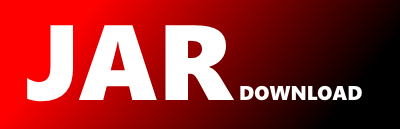
com.pulumi.azurenative.datafactory.kotlin.outputs.ODataLinkedServiceResponse.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datafactory.kotlin.outputs
import com.pulumi.core.Either
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* Open Data Protocol (OData) linked service.
* @property aadResourceId Specify the resource you are requesting authorization to use Directory. Type: string (or Expression with resultType string).
* @property aadServicePrincipalCredentialType Specify the credential type (key or cert) is used for service principal.
* @property annotations List of tags that can be used for describing the linked service.
* @property authHeaders The additional HTTP headers in the request to RESTful API used for authorization. Type: key value pairs (value should be string type).
* @property authenticationType Type of authentication used to connect to the OData service.
* @property azureCloudType Indicates the azure cloud type of the service principle auth. Allowed values are AzurePublic, AzureChina, AzureUsGovernment, AzureGermany. Default value is the data factory regions’ cloud type. Type: string (or Expression with resultType string).
* @property connectVia The integration runtime reference.
* @property description Linked service description.
* @property encryptedCredential The encrypted credential used for authentication. Credentials are encrypted using the integration runtime credential manager. Type: string.
* @property parameters Parameters for linked service.
* @property password Password of the OData service.
* @property servicePrincipalEmbeddedCert Specify the base64 encoded certificate of your application registered in Azure Active Directory. Type: string (or Expression with resultType string).
* @property servicePrincipalEmbeddedCertPassword Specify the password of your certificate if your certificate has a password and you are using AadServicePrincipal authentication. Type: string (or Expression with resultType string).
* @property servicePrincipalId Specify the application id of your application registered in Azure Active Directory. Type: string (or Expression with resultType string).
* @property servicePrincipalKey Specify the secret of your application registered in Azure Active Directory. Type: string (or Expression with resultType string).
* @property tenant Specify the tenant information (domain name or tenant ID) under which your application resides. Type: string (or Expression with resultType string).
* @property type Type of linked service.
* Expected value is 'OData'.
* @property url The URL of the OData service endpoint. Type: string (or Expression with resultType string).
* @property userName User name of the OData service. Type: string (or Expression with resultType string).
* @property version Version of the linked service.
*/
public data class ODataLinkedServiceResponse(
public val aadResourceId: Any? = null,
public val aadServicePrincipalCredentialType: String? = null,
public val annotations: List? = null,
public val authHeaders: Any? = null,
public val authenticationType: String? = null,
public val azureCloudType: Any? = null,
public val connectVia: IntegrationRuntimeReferenceResponse? = null,
public val description: String? = null,
public val encryptedCredential: String? = null,
public val parameters: Map? = null,
public val password: Either? = null,
public val servicePrincipalEmbeddedCert: Either? = null,
public val servicePrincipalEmbeddedCertPassword: Either? = null,
public val servicePrincipalId: Any? = null,
public val servicePrincipalKey: Either? = null,
public val tenant: Any? = null,
public val type: String,
public val url: Any,
public val userName: Any? = null,
public val version: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.datafactory.outputs.ODataLinkedServiceResponse): ODataLinkedServiceResponse = ODataLinkedServiceResponse(
aadResourceId = javaType.aadResourceId().map({ args0 -> args0 }).orElse(null),
aadServicePrincipalCredentialType = javaType.aadServicePrincipalCredentialType().map({ args0 ->
args0
}).orElse(null),
annotations = javaType.annotations().map({ args0 -> args0 }),
authHeaders = javaType.authHeaders().map({ args0 -> args0 }).orElse(null),
authenticationType = javaType.authenticationType().map({ args0 -> args0 }).orElse(null),
azureCloudType = javaType.azureCloudType().map({ args0 -> args0 }).orElse(null),
connectVia = javaType.connectVia().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.IntegrationRuntimeReferenceResponse.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
encryptedCredential = javaType.encryptedCredential().map({ args0 -> args0 }).orElse(null),
parameters = javaType.parameters().map({ args0 ->
args0.key.to(
args0.value.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.ParameterSpecificationResponse.Companion.toKotlin(args0)
}),
)
}).toMap(),
password = javaType.password().map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.AzureKeyVaultSecretReferenceResponse.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.SecureStringResponse.Companion.toKotlin(args0)
})
},
)
}).orElse(null),
servicePrincipalEmbeddedCert = javaType.servicePrincipalEmbeddedCert().map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.AzureKeyVaultSecretReferenceResponse.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.SecureStringResponse.Companion.toKotlin(args0)
})
},
)
}).orElse(null),
servicePrincipalEmbeddedCertPassword = javaType.servicePrincipalEmbeddedCertPassword().map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.AzureKeyVaultSecretReferenceResponse.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.SecureStringResponse.Companion.toKotlin(args0)
})
},
)
}).orElse(null),
servicePrincipalId = javaType.servicePrincipalId().map({ args0 -> args0 }).orElse(null),
servicePrincipalKey = javaType.servicePrincipalKey().map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.AzureKeyVaultSecretReferenceResponse.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datafactory.kotlin.outputs.SecureStringResponse.Companion.toKotlin(args0)
})
},
)
}).orElse(null),
tenant = javaType.tenant().map({ args0 -> args0 }).orElse(null),
type = javaType.type(),
url = javaType.url(),
userName = javaType.userName().map({ args0 -> args0 }).orElse(null),
version = javaType.version().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy