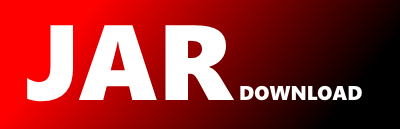
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.GetAccountResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datalakeanalytics.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A Data Lake Analytics account object, containing all information associated with the named Data Lake Analytics account.
* @property accountId The unique identifier associated with this Data Lake Analytics account.
* @property computePolicies The list of compute policies associated with this account.
* @property creationTime The account creation time.
* @property currentTier The commitment tier in use for the current month.
* @property dataLakeStoreAccounts The list of Data Lake Store accounts associated with this account.
* @property debugDataAccessLevel The current state of the DebugDataAccessLevel for this account.
* @property defaultDataLakeStoreAccount The default Data Lake Store account associated with this account.
* @property defaultDataLakeStoreAccountType The type of the default Data Lake Store account associated with this account.
* @property endpoint The full CName endpoint for this account.
* @property firewallAllowAzureIps The current state of allowing or disallowing IPs originating within Azure through the firewall. If the firewall is disabled, this is not enforced.
* @property firewallRules The list of firewall rules associated with this account.
* @property firewallState The current state of the IP address firewall for this account.
* @property hiveMetastores The list of hiveMetastores associated with this account.
* @property id The resource identifier.
* @property lastModifiedTime The account last modified time.
* @property location The resource location.
* @property maxActiveJobCountPerUser The maximum supported active jobs under the account at the same time.
* @property maxDegreeOfParallelism The maximum supported degree of parallelism for this account.
* @property maxDegreeOfParallelismPerJob The maximum supported degree of parallelism per job for this account.
* @property maxJobCount The maximum supported jobs running under the account at the same time.
* @property maxJobRunningTimeInMin The maximum supported active jobs under the account at the same time.
* @property maxQueuedJobCountPerUser The maximum supported jobs queued under the account at the same time.
* @property minPriorityPerJob The minimum supported priority per job for this account.
* @property name The resource name.
* @property newTier The commitment tier for the next month.
* @property provisioningState The provisioning status of the Data Lake Analytics account.
* @property publicDataLakeStoreAccounts The list of Data Lake Store accounts associated with this account.
* @property queryStoreRetention The number of days that job metadata is retained.
* @property state The state of the Data Lake Analytics account.
* @property storageAccounts The list of Azure Blob Storage accounts associated with this account.
* @property systemMaxDegreeOfParallelism The system defined maximum supported degree of parallelism for this account, which restricts the maximum value of parallelism the user can set for the account.
* @property systemMaxJobCount The system defined maximum supported jobs running under the account at the same time, which restricts the maximum number of running jobs the user can set for the account.
* @property tags The resource tags.
* @property type The resource type.
* @property virtualNetworkRules The list of virtualNetwork rules associated with this account.
*/
public data class GetAccountResult(
public val accountId: String,
public val computePolicies: List,
public val creationTime: String,
public val currentTier: String,
public val dataLakeStoreAccounts: List,
public val debugDataAccessLevel: String,
public val defaultDataLakeStoreAccount: String,
public val defaultDataLakeStoreAccountType: String,
public val endpoint: String,
public val firewallAllowAzureIps: String? = null,
public val firewallRules: List,
public val firewallState: String? = null,
public val hiveMetastores: List,
public val id: String,
public val lastModifiedTime: String,
public val location: String,
public val maxActiveJobCountPerUser: Int,
public val maxDegreeOfParallelism: Int? = null,
public val maxDegreeOfParallelismPerJob: Int? = null,
public val maxJobCount: Int? = null,
public val maxJobRunningTimeInMin: Int,
public val maxQueuedJobCountPerUser: Int,
public val minPriorityPerJob: Int,
public val name: String,
public val newTier: String? = null,
public val provisioningState: String,
public val publicDataLakeStoreAccounts: List? = null,
public val queryStoreRetention: Int? = null,
public val state: String,
public val storageAccounts: List,
public val systemMaxDegreeOfParallelism: Int,
public val systemMaxJobCount: Int,
public val tags: Map,
public val type: String,
public val virtualNetworkRules: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azurenative.datalakeanalytics.outputs.GetAccountResult): GetAccountResult = GetAccountResult(
accountId = javaType.accountId(),
computePolicies = javaType.computePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.ComputePolicyResponse.Companion.toKotlin(args0)
})
}),
creationTime = javaType.creationTime(),
currentTier = javaType.currentTier(),
dataLakeStoreAccounts = javaType.dataLakeStoreAccounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.DataLakeStoreAccountInformationResponse.Companion.toKotlin(args0)
})
}),
debugDataAccessLevel = javaType.debugDataAccessLevel(),
defaultDataLakeStoreAccount = javaType.defaultDataLakeStoreAccount(),
defaultDataLakeStoreAccountType = javaType.defaultDataLakeStoreAccountType(),
endpoint = javaType.endpoint(),
firewallAllowAzureIps = javaType.firewallAllowAzureIps().map({ args0 -> args0 }).orElse(null),
firewallRules = javaType.firewallRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.FirewallRuleResponse.Companion.toKotlin(args0)
})
}),
firewallState = javaType.firewallState().map({ args0 -> args0 }).orElse(null),
hiveMetastores = javaType.hiveMetastores().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.HiveMetastoreResponse.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
lastModifiedTime = javaType.lastModifiedTime(),
location = javaType.location(),
maxActiveJobCountPerUser = javaType.maxActiveJobCountPerUser(),
maxDegreeOfParallelism = javaType.maxDegreeOfParallelism().map({ args0 -> args0 }).orElse(null),
maxDegreeOfParallelismPerJob = javaType.maxDegreeOfParallelismPerJob().map({ args0 ->
args0
}).orElse(null),
maxJobCount = javaType.maxJobCount().map({ args0 -> args0 }).orElse(null),
maxJobRunningTimeInMin = javaType.maxJobRunningTimeInMin(),
maxQueuedJobCountPerUser = javaType.maxQueuedJobCountPerUser(),
minPriorityPerJob = javaType.minPriorityPerJob(),
name = javaType.name(),
newTier = javaType.newTier().map({ args0 -> args0 }).orElse(null),
provisioningState = javaType.provisioningState(),
publicDataLakeStoreAccounts = javaType.publicDataLakeStoreAccounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.DataLakeStoreAccountInformationResponse.Companion.toKotlin(args0)
})
}),
queryStoreRetention = javaType.queryStoreRetention().map({ args0 -> args0 }).orElse(null),
state = javaType.state(),
storageAccounts = javaType.storageAccounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.StorageAccountInformationResponse.Companion.toKotlin(args0)
})
}),
systemMaxDegreeOfParallelism = javaType.systemMaxDegreeOfParallelism(),
systemMaxJobCount = javaType.systemMaxJobCount(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
type = javaType.type(),
virtualNetworkRules = javaType.virtualNetworkRules().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azurenative.datalakeanalytics.kotlin.outputs.VirtualNetworkRuleResponse.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy