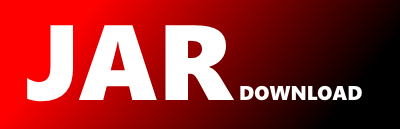
com.pulumi.azurenative.datalakestore.kotlin.DatalakestoreFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datalakestore.kotlin
import com.pulumi.azurenative.datalakestore.DatalakestoreFunctions.getAccountPlain
import com.pulumi.azurenative.datalakestore.DatalakestoreFunctions.getFirewallRulePlain
import com.pulumi.azurenative.datalakestore.DatalakestoreFunctions.getTrustedIdProviderPlain
import com.pulumi.azurenative.datalakestore.DatalakestoreFunctions.getVirtualNetworkRulePlain
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetAccountPlainArgs
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetAccountPlainArgsBuilder
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetFirewallRulePlainArgs
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetFirewallRulePlainArgsBuilder
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetTrustedIdProviderPlainArgs
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetTrustedIdProviderPlainArgsBuilder
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetVirtualNetworkRulePlainArgs
import com.pulumi.azurenative.datalakestore.kotlin.inputs.GetVirtualNetworkRulePlainArgsBuilder
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetAccountResult
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetFirewallRuleResult
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetTrustedIdProviderResult
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetVirtualNetworkRuleResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetAccountResult.Companion.toKotlin as getAccountResultToKotlin
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetFirewallRuleResult.Companion.toKotlin as getFirewallRuleResultToKotlin
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetTrustedIdProviderResult.Companion.toKotlin as getTrustedIdProviderResultToKotlin
import com.pulumi.azurenative.datalakestore.kotlin.outputs.GetVirtualNetworkRuleResult.Companion.toKotlin as getVirtualNetworkRuleResultToKotlin
public object DatalakestoreFunctions {
/**
* Gets the specified Data Lake Store account.
* Azure REST API version: 2016-11-01.
* @param argument null
* @return Data Lake Store account information.
*/
public suspend fun getAccount(argument: GetAccountPlainArgs): GetAccountResult =
getAccountResultToKotlin(getAccountPlain(argument.toJava()).await())
/**
* @see [getAccount].
* @param accountName The name of the Data Lake Store account.
* @param resourceGroupName The name of the Azure resource group.
* @return Data Lake Store account information.
*/
public suspend fun getAccount(accountName: String, resourceGroupName: String): GetAccountResult {
val argument = GetAccountPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
)
return getAccountResultToKotlin(getAccountPlain(argument.toJava()).await())
}
/**
* @see [getAccount].
* @param argument Builder for [com.pulumi.azurenative.datalakestore.kotlin.inputs.GetAccountPlainArgs].
* @return Data Lake Store account information.
*/
public suspend fun getAccount(argument: suspend GetAccountPlainArgsBuilder.() -> Unit): GetAccountResult {
val builder = GetAccountPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getAccountResultToKotlin(getAccountPlain(builtArgument.toJava()).await())
}
/**
* Gets the specified Data Lake Store firewall rule.
* Azure REST API version: 2016-11-01.
* @param argument null
* @return Data Lake Store firewall rule information.
*/
public suspend fun getFirewallRule(argument: GetFirewallRulePlainArgs): GetFirewallRuleResult =
getFirewallRuleResultToKotlin(getFirewallRulePlain(argument.toJava()).await())
/**
* @see [getFirewallRule].
* @param accountName The name of the Data Lake Store account.
* @param firewallRuleName The name of the firewall rule to retrieve.
* @param resourceGroupName The name of the Azure resource group.
* @return Data Lake Store firewall rule information.
*/
public suspend fun getFirewallRule(
accountName: String,
firewallRuleName: String,
resourceGroupName: String,
): GetFirewallRuleResult {
val argument = GetFirewallRulePlainArgs(
accountName = accountName,
firewallRuleName = firewallRuleName,
resourceGroupName = resourceGroupName,
)
return getFirewallRuleResultToKotlin(getFirewallRulePlain(argument.toJava()).await())
}
/**
* @see [getFirewallRule].
* @param argument Builder for [com.pulumi.azurenative.datalakestore.kotlin.inputs.GetFirewallRulePlainArgs].
* @return Data Lake Store firewall rule information.
*/
public suspend fun getFirewallRule(argument: suspend GetFirewallRulePlainArgsBuilder.() -> Unit): GetFirewallRuleResult {
val builder = GetFirewallRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getFirewallRuleResultToKotlin(getFirewallRulePlain(builtArgument.toJava()).await())
}
/**
* Gets the specified Data Lake Store trusted identity provider.
* Azure REST API version: 2016-11-01.
* @param argument null
* @return Data Lake Store trusted identity provider information.
*/
public suspend fun getTrustedIdProvider(argument: GetTrustedIdProviderPlainArgs): GetTrustedIdProviderResult =
getTrustedIdProviderResultToKotlin(getTrustedIdProviderPlain(argument.toJava()).await())
/**
* @see [getTrustedIdProvider].
* @param accountName The name of the Data Lake Store account.
* @param resourceGroupName The name of the Azure resource group.
* @param trustedIdProviderName The name of the trusted identity provider to retrieve.
* @return Data Lake Store trusted identity provider information.
*/
public suspend fun getTrustedIdProvider(
accountName: String,
resourceGroupName: String,
trustedIdProviderName: String,
): GetTrustedIdProviderResult {
val argument = GetTrustedIdProviderPlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
trustedIdProviderName = trustedIdProviderName,
)
return getTrustedIdProviderResultToKotlin(getTrustedIdProviderPlain(argument.toJava()).await())
}
/**
* @see [getTrustedIdProvider].
* @param argument Builder for [com.pulumi.azurenative.datalakestore.kotlin.inputs.GetTrustedIdProviderPlainArgs].
* @return Data Lake Store trusted identity provider information.
*/
public suspend fun getTrustedIdProvider(argument: suspend GetTrustedIdProviderPlainArgsBuilder.() -> Unit): GetTrustedIdProviderResult {
val builder = GetTrustedIdProviderPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getTrustedIdProviderResultToKotlin(getTrustedIdProviderPlain(builtArgument.toJava()).await())
}
/**
* Gets the specified Data Lake Store virtual network rule.
* Azure REST API version: 2016-11-01.
* @param argument null
* @return Data Lake Store virtual network rule information.
*/
public suspend fun getVirtualNetworkRule(argument: GetVirtualNetworkRulePlainArgs): GetVirtualNetworkRuleResult =
getVirtualNetworkRuleResultToKotlin(getVirtualNetworkRulePlain(argument.toJava()).await())
/**
* @see [getVirtualNetworkRule].
* @param accountName The name of the Data Lake Store account.
* @param resourceGroupName The name of the Azure resource group.
* @param virtualNetworkRuleName The name of the virtual network rule to retrieve.
* @return Data Lake Store virtual network rule information.
*/
public suspend fun getVirtualNetworkRule(
accountName: String,
resourceGroupName: String,
virtualNetworkRuleName: String,
): GetVirtualNetworkRuleResult {
val argument = GetVirtualNetworkRulePlainArgs(
accountName = accountName,
resourceGroupName = resourceGroupName,
virtualNetworkRuleName = virtualNetworkRuleName,
)
return getVirtualNetworkRuleResultToKotlin(getVirtualNetworkRulePlain(argument.toJava()).await())
}
/**
* @see [getVirtualNetworkRule].
* @param argument Builder for [com.pulumi.azurenative.datalakestore.kotlin.inputs.GetVirtualNetworkRulePlainArgs].
* @return Data Lake Store virtual network rule information.
*/
public suspend fun getVirtualNetworkRule(argument: suspend GetVirtualNetworkRulePlainArgsBuilder.() -> Unit): GetVirtualNetworkRuleResult {
val builder = GetVirtualNetworkRulePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getVirtualNetworkRuleResultToKotlin(getVirtualNetworkRulePlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy