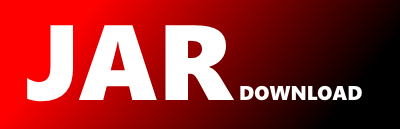
com.pulumi.azurenative.datamigration.kotlin.inputs.PostgreSqlConnectionInfoArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.datamigration.kotlin.inputs
import com.pulumi.azurenative.datamigration.inputs.PostgreSqlConnectionInfoArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Information for connecting to PostgreSQL server
* @property databaseName Name of the database
* @property encryptConnection Whether to encrypt the connection
* @property password Password credential.
* @property port Port for Server
* @property serverName Name of the server
* @property trustServerCertificate Whether to trust the server certificate
* @property type Type of connection info
* Expected value is 'PostgreSqlConnectionInfo'.
* @property userName User name
*/
public data class PostgreSqlConnectionInfoArgs(
public val databaseName: Output? = null,
public val encryptConnection: Output? = null,
public val password: Output? = null,
public val port: Output,
public val serverName: Output,
public val trustServerCertificate: Output? = null,
public val type: Output,
public val userName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.datamigration.inputs.PostgreSqlConnectionInfoArgs =
com.pulumi.azurenative.datamigration.inputs.PostgreSqlConnectionInfoArgs.builder()
.databaseName(databaseName?.applyValue({ args0 -> args0 }))
.encryptConnection(encryptConnection?.applyValue({ args0 -> args0 }))
.password(password?.applyValue({ args0 -> args0 }))
.port(port.applyValue({ args0 -> args0 }))
.serverName(serverName.applyValue({ args0 -> args0 }))
.trustServerCertificate(trustServerCertificate?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 }))
.userName(userName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PostgreSqlConnectionInfoArgs].
*/
@PulumiTagMarker
public class PostgreSqlConnectionInfoArgsBuilder internal constructor() {
private var databaseName: Output? = null
private var encryptConnection: Output? = null
private var password: Output? = null
private var port: Output? = null
private var serverName: Output? = null
private var trustServerCertificate: Output? = null
private var type: Output? = null
private var userName: Output? = null
/**
* @param value Name of the database
*/
@JvmName("omrocapifoqkxlvm")
public suspend fun databaseName(`value`: Output) {
this.databaseName = value
}
/**
* @param value Whether to encrypt the connection
*/
@JvmName("dsvxowtumrifptgu")
public suspend fun encryptConnection(`value`: Output) {
this.encryptConnection = value
}
/**
* @param value Password credential.
*/
@JvmName("nrsuosrdoabmssgv")
public suspend fun password(`value`: Output) {
this.password = value
}
/**
* @param value Port for Server
*/
@JvmName("dtevtpbvjrfhblbb")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Name of the server
*/
@JvmName("hopbdddwdcojahcv")
public suspend fun serverName(`value`: Output) {
this.serverName = value
}
/**
* @param value Whether to trust the server certificate
*/
@JvmName("tqpwwtdeutawgakm")
public suspend fun trustServerCertificate(`value`: Output) {
this.trustServerCertificate = value
}
/**
* @param value Type of connection info
* Expected value is 'PostgreSqlConnectionInfo'.
*/
@JvmName("forrmvglqoxhwqon")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value User name
*/
@JvmName("yfnocihtdinsneby")
public suspend fun userName(`value`: Output) {
this.userName = value
}
/**
* @param value Name of the database
*/
@JvmName("uyuldbrvmybbudsd")
public suspend fun databaseName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseName = mapped
}
/**
* @param value Whether to encrypt the connection
*/
@JvmName("rnlbhbsjouhlkujk")
public suspend fun encryptConnection(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptConnection = mapped
}
/**
* @param value Password credential.
*/
@JvmName("rvfjxhlmaupymnfc")
public suspend fun password(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.password = mapped
}
/**
* @param value Port for Server
*/
@JvmName("oxamfvywrxhesmad")
public suspend fun port(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value Name of the server
*/
@JvmName("hsjtomwlhbqrltpo")
public suspend fun serverName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.serverName = mapped
}
/**
* @param value Whether to trust the server certificate
*/
@JvmName("btiysfnctxiqxoix")
public suspend fun trustServerCertificate(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trustServerCertificate = mapped
}
/**
* @param value Type of connection info
* Expected value is 'PostgreSqlConnectionInfo'.
*/
@JvmName("hhbrartnyelrgsfl")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value User name
*/
@JvmName("xrajsikvlcbadree")
public suspend fun userName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.userName = mapped
}
internal fun build(): PostgreSqlConnectionInfoArgs = PostgreSqlConnectionInfoArgs(
databaseName = databaseName,
encryptConnection = encryptConnection,
password = password,
port = port ?: throw PulumiNullFieldException("port"),
serverName = serverName ?: throw PulumiNullFieldException("serverName"),
trustServerCertificate = trustServerCertificate,
type = type ?: throw PulumiNullFieldException("type"),
userName = userName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy