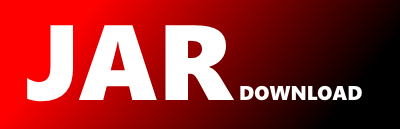
com.pulumi.azurenative.dataprotection.kotlin.BackupPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.dataprotection.kotlin
import com.pulumi.azurenative.dataprotection.BackupPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* BaseBackupPolicy resource
* Azure REST API version: 2023-01-01. Prior API version in Azure Native 1.x: 2021-01-01.
* Other available API versions: 2023-04-01-preview, 2023-05-01, 2023-06-01-preview, 2023-08-01-preview, 2023-11-01, 2023-12-01, 2024-02-01-preview, 2024-03-01, 2024-04-01.
* ## Example Usage
* ### CreateOrUpdate BackupPolicy
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var backupPolicy = new AzureNative.DataProtection.BackupPolicy("backupPolicy", new()
* {
* BackupPolicyName = "OSSDBPolicy",
* Properties = new AzureNative.DataProtection.Inputs.BackupPolicyArgs
* {
* DatasourceTypes = new[]
* {
* "OssDB",
* },
* ObjectType = "BackupPolicy",
* PolicyRules =
* {
* new AzureNative.DataProtection.Inputs.AzureBackupRuleArgs
* {
* BackupParameters = new AzureNative.DataProtection.Inputs.AzureBackupParamsArgs
* {
* BackupType = "Full",
* ObjectType = "AzureBackupParams",
* },
* DataStore = new AzureNative.DataProtection.Inputs.DataStoreInfoBaseArgs
* {
* DataStoreType = AzureNative.DataProtection.DataStoreTypes.VaultStore,
* ObjectType = "DataStoreInfoBase",
* },
* Name = "BackupWeekly",
* ObjectType = "AzureBackupRule",
* Trigger = new AzureNative.DataProtection.Inputs.ScheduleBasedTriggerContextArgs
* {
* ObjectType = "ScheduleBasedTriggerContext",
* Schedule = new AzureNative.DataProtection.Inputs.BackupScheduleArgs
* {
* RepeatingTimeIntervals = new[]
* {
* "R/2019-11-20T08:00:00-08:00/P1W",
* },
* },
* TaggingCriteria = new[]
* {
* new AzureNative.DataProtection.Inputs.TaggingCriteriaArgs
* {
* IsDefault = true,
* TagInfo = new AzureNative.DataProtection.Inputs.RetentionTagArgs
* {
* TagName = "Default",
* },
* TaggingPriority = 99,
* },
* new AzureNative.DataProtection.Inputs.TaggingCriteriaArgs
* {
* Criteria = new[]
* {
* new AzureNative.DataProtection.Inputs.ScheduleBasedBackupCriteriaArgs
* {
* DaysOfTheWeek = new[]
* {
* AzureNative.DataProtection.DayOfWeek.Sunday,
* },
* ObjectType = "ScheduleBasedBackupCriteria",
* ScheduleTimes = new[]
* {
* "2019-03-01T13:00:00Z",
* },
* },
* },
* IsDefault = false,
* TagInfo = new AzureNative.DataProtection.Inputs.RetentionTagArgs
* {
* TagName = "Weekly",
* },
* TaggingPriority = 20,
* },
* },
* },
* },
* new AzureNative.DataProtection.Inputs.AzureRetentionRuleArgs
* {
* IsDefault = true,
* Lifecycles = new[]
* {
* new AzureNative.DataProtection.Inputs.SourceLifeCycleArgs
* {
* DeleteAfter = new AzureNative.DataProtection.Inputs.AbsoluteDeleteOptionArgs
* {
* Duration = "P1W",
* ObjectType = "AbsoluteDeleteOption",
* },
* SourceDataStore = new AzureNative.DataProtection.Inputs.DataStoreInfoBaseArgs
* {
* DataStoreType = AzureNative.DataProtection.DataStoreTypes.VaultStore,
* ObjectType = "DataStoreInfoBase",
* },
* },
* },
* Name = "Default",
* ObjectType = "AzureRetentionRule",
* },
* new AzureNative.DataProtection.Inputs.AzureRetentionRuleArgs
* {
* IsDefault = false,
* Lifecycles = new[]
* {
* new AzureNative.DataProtection.Inputs.SourceLifeCycleArgs
* {
* DeleteAfter = new AzureNative.DataProtection.Inputs.AbsoluteDeleteOptionArgs
* {
* Duration = "P12W",
* ObjectType = "AbsoluteDeleteOption",
* },
* SourceDataStore = new AzureNative.DataProtection.Inputs.DataStoreInfoBaseArgs
* {
* DataStoreType = AzureNative.DataProtection.DataStoreTypes.VaultStore,
* ObjectType = "DataStoreInfoBase",
* },
* },
* },
* Name = "Weekly",
* ObjectType = "AzureRetentionRule",
* },
* },
* },
* ResourceGroupName = "000pikumar",
* VaultName = "PrivatePreviewVault",
* });
* });
* ```
* ```go
* package main
* import (
* dataprotection "github.com/pulumi/pulumi-azure-native-sdk/dataprotection/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := dataprotection.NewBackupPolicy(ctx, "backupPolicy", &dataprotection.BackupPolicyArgs{
* BackupPolicyName: pulumi.String("OSSDBPolicy"),
* Properties: &dataprotection.BackupPolicyTypeArgs{
* DatasourceTypes: pulumi.StringArray{
* pulumi.String("OssDB"),
* },
* ObjectType: pulumi.String("BackupPolicy"),
* PolicyRules: pulumi.Array{
* dataprotection.AzureBackupRule{
* BackupParameters: dataprotection.AzureBackupParams{
* BackupType: "Full",
* ObjectType: "AzureBackupParams",
* },
* DataStore: dataprotection.DataStoreInfoBase{
* DataStoreType: dataprotection.DataStoreTypesVaultStore,
* ObjectType: "DataStoreInfoBase",
* },
* Name: "BackupWeekly",
* ObjectType: "AzureBackupRule",
* Trigger: dataprotection.ScheduleBasedTriggerContext{
* ObjectType: "ScheduleBasedTriggerContext",
* Schedule: dataprotection.BackupSchedule{
* RepeatingTimeIntervals: []string{
* "R/2019-11-20T08:00:00-08:00/P1W",
* },
* },
* TaggingCriteria: []dataprotection.TaggingCriteria{
* {
* IsDefault: true,
* TagInfo: {
* TagName: "Default",
* },
* TaggingPriority: 99,
* },
* {
* Criteria: []dataprotection.ScheduleBasedBackupCriteria{
* {
* DaysOfTheWeek: []dataprotection.DayOfWeek{
* dataprotection.DayOfWeekSunday,
* },
* ObjectType: "ScheduleBasedBackupCriteria",
* ScheduleTimes: []string{
* "2019-03-01T13:00:00Z",
* },
* },
* },
* IsDefault: false,
* TagInfo: {
* TagName: "Weekly",
* },
* TaggingPriority: 20,
* },
* },
* },
* },
* dataprotection.AzureRetentionRule{
* IsDefault: true,
* Lifecycles: []dataprotection.SourceLifeCycle{
* {
* DeleteAfter: {
* Duration: "P1W",
* ObjectType: "AbsoluteDeleteOption",
* },
* SourceDataStore: {
* DataStoreType: dataprotection.DataStoreTypesVaultStore,
* ObjectType: "DataStoreInfoBase",
* },
* },
* },
* Name: "Default",
* ObjectType: "AzureRetentionRule",
* },
* dataprotection.AzureRetentionRule{
* IsDefault: false,
* Lifecycles: []dataprotection.SourceLifeCycle{
* {
* DeleteAfter: {
* Duration: "P12W",
* ObjectType: "AbsoluteDeleteOption",
* },
* SourceDataStore: {
* DataStoreType: dataprotection.DataStoreTypesVaultStore,
* ObjectType: "DataStoreInfoBase",
* },
* },
* },
* Name: "Weekly",
* ObjectType: "AzureRetentionRule",
* },
* },
* },
* ResourceGroupName: pulumi.String("000pikumar"),
* VaultName: pulumi.String("PrivatePreviewVault"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.dataprotection.BackupPolicy;
* import com.pulumi.azurenative.dataprotection.BackupPolicyArgs;
* import com.pulumi.azurenative.dataprotection.inputs.BackupPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var backupPolicy = new BackupPolicy("backupPolicy", BackupPolicyArgs.builder()
* .backupPolicyName("OSSDBPolicy")
* .properties(BackupPolicyArgs.builder()
* .datasourceTypes("OssDB")
* .objectType("BackupPolicy")
* .policyRules(
* AzureBackupRuleArgs.builder()
* .backupParameters(AzureBackupParamsArgs.builder()
* .backupType("Full")
* .objectType("AzureBackupParams")
* .build())
* .dataStore(DataStoreInfoBaseArgs.builder()
* .dataStoreType("VaultStore")
* .objectType("DataStoreInfoBase")
* .build())
* .name("BackupWeekly")
* .objectType("AzureBackupRule")
* .trigger(ScheduleBasedTriggerContextArgs.builder()
* .objectType("ScheduleBasedTriggerContext")
* .schedule(BackupScheduleArgs.builder()
* .repeatingTimeIntervals("R/2019-11-20T08:00:00-08:00/P1W")
* .build())
* .taggingCriteria(
* TaggingCriteriaArgs.builder()
* .isDefault(true)
* .tagInfo(RetentionTagArgs.builder()
* .tagName("Default")
* .build())
* .taggingPriority(99)
* .build(),
* TaggingCriteriaArgs.builder()
* .criteria(ScheduleBasedBackupCriteriaArgs.builder()
* .daysOfTheWeek("Sunday")
* .objectType("ScheduleBasedBackupCriteria")
* .scheduleTimes("2019-03-01T13:00:00Z")
* .build())
* .isDefault(false)
* .tagInfo(RetentionTagArgs.builder()
* .tagName("Weekly")
* .build())
* .taggingPriority(20)
* .build())
* .build())
* .build(),
* AzureRetentionRuleArgs.builder()
* .isDefault(true)
* .lifecycles(SourceLifeCycleArgs.builder()
* .deleteAfter(AbsoluteDeleteOptionArgs.builder()
* .duration("P1W")
* .objectType("AbsoluteDeleteOption")
* .build())
* .sourceDataStore(DataStoreInfoBaseArgs.builder()
* .dataStoreType("VaultStore")
* .objectType("DataStoreInfoBase")
* .build())
* .build())
* .name("Default")
* .objectType("AzureRetentionRule")
* .build(),
* AzureRetentionRuleArgs.builder()
* .isDefault(false)
* .lifecycles(SourceLifeCycleArgs.builder()
* .deleteAfter(AbsoluteDeleteOptionArgs.builder()
* .duration("P12W")
* .objectType("AbsoluteDeleteOption")
* .build())
* .sourceDataStore(DataStoreInfoBaseArgs.builder()
* .dataStoreType("VaultStore")
* .objectType("DataStoreInfoBase")
* .build())
* .build())
* .name("Weekly")
* .objectType("AzureRetentionRule")
* .build())
* .build())
* .resourceGroupName("000pikumar")
* .vaultName("PrivatePreviewVault")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:dataprotection:BackupPolicy OSSDBPolicy /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.DataProtection/backupVaults/{vaultName}/backupPolicies/{backupPolicyName}
* ```
* @property backupPolicyName Name of the policy
* @property properties BaseBackupPolicyResource properties
* @property resourceGroupName The name of the resource group. The name is case insensitive.
* @property vaultName The name of the backup vault.
*/
public data class BackupPolicyArgs(
public val backupPolicyName: Output? = null,
public val properties: Output? = null,
public val resourceGroupName: Output? = null,
public val vaultName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.dataprotection.BackupPolicyArgs =
com.pulumi.azurenative.dataprotection.BackupPolicyArgs.builder()
.backupPolicyName(backupPolicyName?.applyValue({ args0 -> args0 }))
.properties(properties?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.vaultName(vaultName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackupPolicyArgs].
*/
@PulumiTagMarker
public class BackupPolicyArgsBuilder internal constructor() {
private var backupPolicyName: Output? = null
private var properties:
Output? = null
private var resourceGroupName: Output? = null
private var vaultName: Output? = null
/**
* @param value Name of the policy
*/
@JvmName("dttxxcagcsqbqlmc")
public suspend fun backupPolicyName(`value`: Output) {
this.backupPolicyName = value
}
/**
* @param value BaseBackupPolicyResource properties
*/
@JvmName("ivjjmovatbuviwgh")
public suspend fun properties(`value`: Output) {
this.properties = value
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("qcnkslqplbtvfyeq")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value The name of the backup vault.
*/
@JvmName("nqespolsgnxqnvfw")
public suspend fun vaultName(`value`: Output) {
this.vaultName = value
}
/**
* @param value Name of the policy
*/
@JvmName("vghniobeswtfwvqu")
public suspend fun backupPolicyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backupPolicyName = mapped
}
/**
* @param value BaseBackupPolicyResource properties
*/
@JvmName("bvysoctydeoloyev")
public suspend fun properties(`value`: com.pulumi.azurenative.dataprotection.kotlin.inputs.BackupPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.properties = mapped
}
/**
* @param argument BaseBackupPolicyResource properties
*/
@JvmName("nutrlsqdrvhbkstj")
public suspend fun properties(argument: suspend com.pulumi.azurenative.dataprotection.kotlin.inputs.BackupPolicyArgsBuilder.() -> Unit) {
val toBeMapped =
com.pulumi.azurenative.dataprotection.kotlin.inputs.BackupPolicyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.properties = mapped
}
/**
* @param value The name of the resource group. The name is case insensitive.
*/
@JvmName("kivgvlfyklgshlok")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value The name of the backup vault.
*/
@JvmName("wfkkevljcrngysvv")
public suspend fun vaultName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vaultName = mapped
}
internal fun build(): BackupPolicyArgs = BackupPolicyArgs(
backupPolicyName = backupPolicyName,
properties = properties,
resourceGroupName = resourceGroupName,
vaultName = vaultName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy