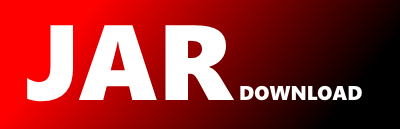
com.pulumi.azurenative.desktopvirtualization.kotlin.MSIXPackage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.desktopvirtualization.kotlin
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.MsixPackageApplicationsResponse
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.MsixPackageDependenciesResponse
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.SystemDataResponse
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.MsixPackageApplicationsResponse.Companion.toKotlin as msixPackageApplicationsResponseToKotlin
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.MsixPackageDependenciesResponse.Companion.toKotlin as msixPackageDependenciesResponseToKotlin
import com.pulumi.azurenative.desktopvirtualization.kotlin.outputs.SystemDataResponse.Companion.toKotlin as systemDataResponseToKotlin
/**
* Builder for [MSIXPackage].
*/
@PulumiTagMarker
public class MSIXPackageResourceBuilder internal constructor() {
public var name: String? = null
public var args: MSIXPackageArgs = MSIXPackageArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend MSIXPackageArgsBuilder.() -> Unit) {
val builder = MSIXPackageArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): MSIXPackage {
val builtJavaResource =
com.pulumi.azurenative.desktopvirtualization.MSIXPackage(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return MSIXPackage(builtJavaResource)
}
}
/**
* Schema for MSIX Package properties.
* Azure REST API version: 2022-09-09. Prior API version in Azure Native 1.x: 2021-02-01-preview.
* Other available API versions: 2022-10-14-preview, 2023-07-07-preview, 2023-09-05, 2023-10-04-preview, 2023-11-01-preview, 2024-01-16-preview, 2024-03-06-preview, 2024-04-03, 2024-04-08-preview.
* ## Example Usage
* ### MSIXPackage_Create
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using AzureNative = Pulumi.AzureNative;
* return await Deployment.RunAsync(() =>
* {
* var msixPackage = new AzureNative.DesktopVirtualization.MSIXPackage("msixPackage", new()
* {
* DisplayName = "displayname",
* HostPoolName = "hostpool1",
* ImagePath = "imagepath",
* IsActive = false,
* IsRegularRegistration = false,
* LastUpdated = "2008-09-22T14:01:54.9571247Z",
* MsixPackageFullName = "msixpackagefullname",
* PackageApplications = new[]
* {
* new AzureNative.DesktopVirtualization.Inputs.MsixPackageApplicationsArgs
* {
* AppId = "ApplicationId",
* AppUserModelID = "AppUserModelId",
* Description = "application-desc",
* FriendlyName = "friendlyname",
* IconImageName = "Apptile",
* RawIcon = "VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo",
* RawPng = "VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo",
* },
* },
* PackageDependencies = new[]
* {
* new AzureNative.DesktopVirtualization.Inputs.MsixPackageDependenciesArgs
* {
* DependencyName = "MsixTest_Dependency_Name",
* MinVersion = "version",
* Publisher = "PublishedName",
* },
* },
* PackageFamilyName = "MsixPackage_FamilyName",
* PackageName = "MsixPackage_name",
* PackageRelativePath = "packagerelativepath",
* ResourceGroupName = "resourceGroup1",
* Version = "version",
* });
* });
* ```
* ```go
* package main
* import (
* desktopvirtualization "github.com/pulumi/pulumi-azure-native-sdk/desktopvirtualization/v2"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := desktopvirtualization.NewMSIXPackage(ctx, "msixPackage", &desktopvirtualization.MSIXPackageArgs{
* DisplayName: pulumi.String("displayname"),
* HostPoolName: pulumi.String("hostpool1"),
* ImagePath: pulumi.String("imagepath"),
* IsActive: pulumi.Bool(false),
* IsRegularRegistration: pulumi.Bool(false),
* LastUpdated: pulumi.String("2008-09-22T14:01:54.9571247Z"),
* MsixPackageFullName: pulumi.String("msixpackagefullname"),
* PackageApplications: desktopvirtualization.MsixPackageApplicationsArray{
* &desktopvirtualization.MsixPackageApplicationsArgs{
* AppId: pulumi.String("ApplicationId"),
* AppUserModelID: pulumi.String("AppUserModelId"),
* Description: pulumi.String("application-desc"),
* FriendlyName: pulumi.String("friendlyname"),
* IconImageName: pulumi.String("Apptile"),
* RawIcon: pulumi.String("VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo"),
* RawPng: pulumi.String("VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo"),
* },
* },
* PackageDependencies: desktopvirtualization.MsixPackageDependenciesArray{
* &desktopvirtualization.MsixPackageDependenciesArgs{
* DependencyName: pulumi.String("MsixTest_Dependency_Name"),
* MinVersion: pulumi.String("version"),
* Publisher: pulumi.String("PublishedName"),
* },
* },
* PackageFamilyName: pulumi.String("MsixPackage_FamilyName"),
* PackageName: pulumi.String("MsixPackage_name"),
* PackageRelativePath: pulumi.String("packagerelativepath"),
* ResourceGroupName: pulumi.String("resourceGroup1"),
* Version: pulumi.String("version"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.desktopvirtualization.MSIXPackage;
* import com.pulumi.azurenative.desktopvirtualization.MSIXPackageArgs;
* import com.pulumi.azurenative.desktopvirtualization.inputs.MsixPackageApplicationsArgs;
* import com.pulumi.azurenative.desktopvirtualization.inputs.MsixPackageDependenciesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var msixPackage = new MSIXPackage("msixPackage", MSIXPackageArgs.builder()
* .displayName("displayname")
* .hostPoolName("hostpool1")
* .imagePath("imagepath")
* .isActive(false)
* .isRegularRegistration(false)
* .lastUpdated("2008-09-22T14:01:54.9571247Z")
* .msixPackageFullName("msixpackagefullname")
* .packageApplications(MsixPackageApplicationsArgs.builder()
* .appId("ApplicationId")
* .appUserModelID("AppUserModelId")
* .description("application-desc")
* .friendlyName("friendlyname")
* .iconImageName("Apptile")
* .rawIcon("VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo")
* .rawPng("VGhpcyBpcyBhIHN0cmluZyB0byBoYXNo")
* .build())
* .packageDependencies(MsixPackageDependenciesArgs.builder()
* .dependencyName("MsixTest_Dependency_Name")
* .minVersion("version")
* .publisher("PublishedName")
* .build())
* .packageFamilyName("MsixPackage_FamilyName")
* .packageName("MsixPackage_name")
* .packageRelativePath("packagerelativepath")
* .resourceGroupName("resourceGroup1")
* .version("version")
* .build());
* }
* }
* ```
* ## Import
* An existing resource can be imported using its type token, name, and identifier, e.g.
* ```sh
* $ pulumi import azure-native:desktopvirtualization:MSIXPackage hostpool1/MsixPackageFullName /subscriptions/{subscriptionId}/resourcegroups/{resourceGroupName}/providers/Microsoft.DesktopVirtualization/hostPools/{hostPoolName}/msixPackages/{msixPackageFullName}
* ```
*/
public class MSIXPackage internal constructor(
override val javaResource: com.pulumi.azurenative.desktopvirtualization.MSIXPackage,
) : KotlinCustomResource(javaResource, MSIXPackageMapper) {
/**
* User friendly Name to be displayed in the portal.
*/
public val displayName: Output?
get() = javaResource.displayName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* VHD/CIM image path on Network Share.
*/
public val imagePath: Output?
get() = javaResource.imagePath().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Make this version of the package the active one across the hostpool.
*/
public val isActive: Output?
get() = javaResource.isActive().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies how to register Package in feed.
*/
public val isRegularRegistration: Output?
get() = javaResource.isRegularRegistration().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Date Package was last updated, found in the appxmanifest.xml.
*/
public val lastUpdated: Output?
get() = javaResource.lastUpdated().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the resource
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* List of package applications.
*/
public val packageApplications: Output>?
get() = javaResource.packageApplications().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
msixPackageApplicationsResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* List of package dependencies.
*/
public val packageDependencies: Output>?
get() = javaResource.packageDependencies().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
msixPackageDependenciesResponseToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Package Family Name from appxmanifest.xml. Contains Package Name and Publisher name.
*/
public val packageFamilyName: Output?
get() = javaResource.packageFamilyName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Package Name from appxmanifest.xml.
*/
public val packageName: Output?
get() = javaResource.packageName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Relative Path to the package inside the image.
*/
public val packageRelativePath: Output?
get() = javaResource.packageRelativePath().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Metadata pertaining to creation and last modification of the resource.
*/
public val systemData: Output
get() = javaResource.systemData().applyValue({ args0 ->
args0.let({ args0 ->
systemDataResponseToKotlin(args0)
})
})
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* Package Version found in the appxmanifest.xml.
*/
public val version: Output?
get() = javaResource.version().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object MSIXPackageMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azurenative.desktopvirtualization.MSIXPackage::class == javaResource::class
override fun map(javaResource: Resource): MSIXPackage = MSIXPackage(
javaResource as
com.pulumi.azurenative.desktopvirtualization.MSIXPackage,
)
}
/**
* @see [MSIXPackage].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [MSIXPackage].
*/
public suspend fun msixPackage(name: String, block: suspend MSIXPackageResourceBuilder.() -> Unit): MSIXPackage {
val builder = MSIXPackageResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [MSIXPackage].
* @param name The _unique_ name of the resulting resource.
*/
public fun msixPackage(name: String): MSIXPackage {
val builder = MSIXPackageResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy