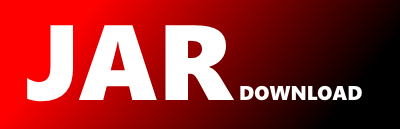
com.pulumi.azurenative.deviceregistry.kotlin.inputs.EventArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.deviceregistry.kotlin.inputs
import com.pulumi.azurenative.deviceregistry.inputs.EventArgs.builder
import com.pulumi.azurenative.deviceregistry.kotlin.enums.EventsObservabilityMode
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Defines the event properties.
* @property capabilityId The path to the type definition of the capability (e.g. DTMI, OPC UA information model node id, etc.), for example dtmi:com:example:Robot:_contents:__prop1;1.
* @property eventConfiguration Protocol-specific configuration for the event. For OPC UA, this could include configuration like, publishingInterval, samplingInterval, and queueSize.
* @property eventNotifier The address of the notifier of the event in the asset (e.g. URL) so that a client can access the event on the asset.
* @property name The name of the event.
* @property observabilityMode An indication of how the event should be mapped to OpenTelemetry.
*/
public data class EventArgs(
public val capabilityId: Output? = null,
public val eventConfiguration: Output? = null,
public val eventNotifier: Output,
public val name: Output? = null,
public val observabilityMode: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.deviceregistry.inputs.EventArgs =
com.pulumi.azurenative.deviceregistry.inputs.EventArgs.builder()
.capabilityId(capabilityId?.applyValue({ args0 -> args0 }))
.eventConfiguration(eventConfiguration?.applyValue({ args0 -> args0 }))
.eventNotifier(eventNotifier.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.observabilityMode(
observabilityMode?.applyValue({ args0 ->
args0.transform(
{ args0 -> args0 },
{ args0 -> args0.let({ args0 -> args0.toJava() }) },
)
}),
).build()
}
/**
* Builder for [EventArgs].
*/
@PulumiTagMarker
public class EventArgsBuilder internal constructor() {
private var capabilityId: Output? = null
private var eventConfiguration: Output? = null
private var eventNotifier: Output? = null
private var name: Output? = null
private var observabilityMode: Output>? = null
/**
* @param value The path to the type definition of the capability (e.g. DTMI, OPC UA information model node id, etc.), for example dtmi:com:example:Robot:_contents:__prop1;1.
*/
@JvmName("xoccrgcbakbyetod")
public suspend fun capabilityId(`value`: Output) {
this.capabilityId = value
}
/**
* @param value Protocol-specific configuration for the event. For OPC UA, this could include configuration like, publishingInterval, samplingInterval, and queueSize.
*/
@JvmName("lffdxlrhaxtlqrci")
public suspend fun eventConfiguration(`value`: Output) {
this.eventConfiguration = value
}
/**
* @param value The address of the notifier of the event in the asset (e.g. URL) so that a client can access the event on the asset.
*/
@JvmName("uibhjjtwstmxjgvn")
public suspend fun eventNotifier(`value`: Output) {
this.eventNotifier = value
}
/**
* @param value The name of the event.
*/
@JvmName("efxxmqfnvhabwmua")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value An indication of how the event should be mapped to OpenTelemetry.
*/
@JvmName("xdkaarbxwnilldxb")
public suspend fun observabilityMode(`value`: Output>) {
this.observabilityMode = value
}
/**
* @param value The path to the type definition of the capability (e.g. DTMI, OPC UA information model node id, etc.), for example dtmi:com:example:Robot:_contents:__prop1;1.
*/
@JvmName("ogfsygkpleybyncp")
public suspend fun capabilityId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.capabilityId = mapped
}
/**
* @param value Protocol-specific configuration for the event. For OPC UA, this could include configuration like, publishingInterval, samplingInterval, and queueSize.
*/
@JvmName("fthsohxdeucblcab")
public suspend fun eventConfiguration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventConfiguration = mapped
}
/**
* @param value The address of the notifier of the event in the asset (e.g. URL) so that a client can access the event on the asset.
*/
@JvmName("tmhcjnbpnkcvtqxo")
public suspend fun eventNotifier(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.eventNotifier = mapped
}
/**
* @param value The name of the event.
*/
@JvmName("mthtovbqianfrqpc")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value An indication of how the event should be mapped to OpenTelemetry.
*/
@JvmName("vsyajoavvgtbajia")
public suspend fun observabilityMode(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.observabilityMode = mapped
}
/**
* @param value An indication of how the event should be mapped to OpenTelemetry.
*/
@JvmName("xiipntjcyyfomvis")
public fun observabilityMode(`value`: String) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.observabilityMode = mapped
}
/**
* @param value An indication of how the event should be mapped to OpenTelemetry.
*/
@JvmName("jlhpplcqyqhfjjqw")
public fun observabilityMode(`value`: EventsObservabilityMode) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.observabilityMode = mapped
}
internal fun build(): EventArgs = EventArgs(
capabilityId = capabilityId,
eventConfiguration = eventConfiguration,
eventNotifier = eventNotifier ?: throw PulumiNullFieldException("eventNotifier"),
name = name,
observabilityMode = observabilityMode,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy