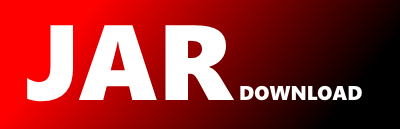
com.pulumi.azurenative.devices.kotlin.inputs.RoutingPropertiesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-native-kotlin Show documentation
Show all versions of pulumi-azure-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azurenative.devices.kotlin.inputs
import com.pulumi.azurenative.devices.inputs.RoutingPropertiesArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The routing related properties of the IoT hub. See: https://docs.microsoft.com/azure/iot-hub/iot-hub-devguide-messaging
* @property endpoints The properties related to the custom endpoints to which your IoT hub routes messages based on the routing rules. A maximum of 10 custom endpoints are allowed across all endpoint types for paid hubs and only 1 custom endpoint is allowed across all endpoint types for free hubs.
* @property enrichments The list of user-provided enrichments that the IoT hub applies to messages to be delivered to built-in and custom endpoints. See: https://aka.ms/telemetryoneventgrid
* @property fallbackRoute The properties of the route that is used as a fall-back route when none of the conditions specified in the 'routes' section are met. This is an optional parameter. When this property is not set, the messages which do not meet any of the conditions specified in the 'routes' section get routed to the built-in eventhub endpoint.
* @property routes The list of user-provided routing rules that the IoT hub uses to route messages to built-in and custom endpoints. A maximum of 100 routing rules are allowed for paid hubs and a maximum of 5 routing rules are allowed for free hubs.
*/
public data class RoutingPropertiesArgs(
public val endpoints: Output? = null,
public val enrichments: Output>? = null,
public val fallbackRoute: Output? = null,
public val routes: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azurenative.devices.inputs.RoutingPropertiesArgs =
com.pulumi.azurenative.devices.inputs.RoutingPropertiesArgs.builder()
.endpoints(endpoints?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.enrichments(
enrichments?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.fallbackRoute(fallbackRoute?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.routes(
routes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RoutingPropertiesArgs].
*/
@PulumiTagMarker
public class RoutingPropertiesArgsBuilder internal constructor() {
private var endpoints: Output? = null
private var enrichments: Output>? = null
private var fallbackRoute: Output? = null
private var routes: Output>? = null
/**
* @param value The properties related to the custom endpoints to which your IoT hub routes messages based on the routing rules. A maximum of 10 custom endpoints are allowed across all endpoint types for paid hubs and only 1 custom endpoint is allowed across all endpoint types for free hubs.
*/
@JvmName("oluhvfmwoxexlgpf")
public suspend fun endpoints(`value`: Output) {
this.endpoints = value
}
/**
* @param value The list of user-provided enrichments that the IoT hub applies to messages to be delivered to built-in and custom endpoints. See: https://aka.ms/telemetryoneventgrid
*/
@JvmName("hywssmkkrokxadrp")
public suspend fun enrichments(`value`: Output>) {
this.enrichments = value
}
@JvmName("jeewsihytvscncak")
public suspend fun enrichments(vararg values: Output) {
this.enrichments = Output.all(values.asList())
}
/**
* @param values The list of user-provided enrichments that the IoT hub applies to messages to be delivered to built-in and custom endpoints. See: https://aka.ms/telemetryoneventgrid
*/
@JvmName("krudhguijaqsmqqi")
public suspend fun enrichments(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy